探索Android布局:LinearLayout与RelativeLayout
发布时间: 2024-03-09 04:07:06 阅读量: 51 订阅数: 28 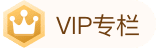
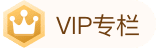
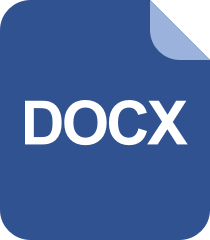
基于STM32单片机的激光雕刻机控制系统设计-含详细步骤和代码
# 1. 布局基础介绍
## 1.1 什么是Android布局?
在Android开发中,布局是用来定义界面上各个控件的摆放位置和大小的方式。布局文件通常使用XML来描述,通过控制布局文件中的各种属性,可以实现对界面的设计和排版。
布局主要用于界面的结构和外观设计,是构建用户界面的基础。
## 1.2 布局在Android开发中的重要性
布局在Android开发中起着至关重要的作用。一个合适的布局可以使界面看起来更加美观,用户体验更加友好,同时也能提升App的性能和响应速度。因此,对于Android开发者来说,熟练掌握各种布局方式,选择合适的布局方式对于提升App的质量至关重要。
# 2. LinearLayout布局深入探讨
LinearLayout是Android中最常用的布局之一,它能够实现简单且有效的布局设计。接下来将深入探讨LinearLayout的特性和用法。
### 2.1 LinearLayout的基本特点
LinearLayout是一个线性布局,其中的子视图可以按照水平或垂直方向排列。可以设置权重来控制子视图在布局中的相对大小,非常灵活。
```java
// 示例代码:垂直布局
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Item 1" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Item 2" />
</LinearLayout>
```
### 2.2 LinearLayout中的垂直布局
垂直布局是指子视图按照垂直方向排列,从上到下依次显示。可以通过`android:orientation="vertical"`来设置为垂直布局。
```java
// 示例代码:垂直布局
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2" />
</LinearLayout>
```
### 2.3 LinearLayout中的水平布局
水平布局是指子视图按照水平方向排列,从左到右依次显示。可以通过`android:orientation="horizontal"`来设置为水平布局。
```java
// 示例代码:水平布局
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 1" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button 2" />
</LinearLayout>
```
通过以上示例,我们可以看到LinearLayout的灵活性和易用性。在实际开发中,根据布局需要选择合适的方向进行布局设计,能够更好地实现页面效果。
# 3. RelativeLayout布局详解
RelativeLayout是Android中非常常用的布局方式,相比LinearLayout更加灵活,能够实现更加复杂的布局结构。在这一章节中,我们将深入探讨RelativeLayout的特点、优势,以及如何使用RelativeLayout实现复杂的布局。
#### 3.1 RelativeLayout的特点和优势
RelativeLayout的特点主要有以下几点:
- 1. 相对定位:RelativeLayout中的子视图会根据相互之间的位置关系进行排列。
- 2. 灵活性:相比LinearLayout,RelativeLayout能够更灵活地对子视图进行定位和布局。
- 3. 嵌套支持:RelativeLayout支持嵌套布局,可以实现更加复杂的界面设计。
优势:
- 1. 灵活性高:可以根据需要自由定位子视图的位置。
- 2. 支持子视图之间的相对关系:可以通过相对定位控制子视图之间的显示顺序和位置。
- 3. 适用于复杂布局:适用于需要复杂布局结构的页面设计。
#### 3.2 使用RelativeLayout实现复杂布局
下面是一个使用RelativeLayout实现复杂布局的示例代码,其中展示了如何相对定位两个TextView和一个ImageView:
```java
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView 1"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"/>
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView 2"
android:layout_below="@id/textView1"
android:layout_alignStart="@id/textView1"/>
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher_foreground"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"/>
</RelativeLayout>
```
#### 3.3 RelativeLayout中常用的属性和方法
在RelativeLayout中,常用的属性和方法有:
- android:layout_alignParentTop:将子视图与父视图顶部对齐。
- android:layout_alignStart:将子视图与指定视图的起始位置对齐。
- android:layout_below:将子视图放置在指定视图的下方。
- android:layout_centerInParent:将子视图居中显示在父视图中心等。
以上是RelativeLayout布局详解的内容,希望对你有所帮助。
# 4. LinearLayout与RelativeLayout的比较
在Android布局中,LinearLayout和RelativeLayout是两种常用的布局方式。它们各自具有特点和优势,下面我们将对它们进行比较分析,以便开发者能够更好地选择合适的布局方式。
#### 4.1 性能对比
线性布局方式(LinearLayout)通常比相对布局方式(RelativeLayout)更高效。这是因为RelativeLayout需要进行多次测量和布局来确定视图的位置,而LinearLayout则在单次测量中完成对子视图的布置。
#### 4.2 灵活性对比
在布局的灵活性方面,RelativeLayout通常比LinearLayout更加灵活。RelativeLayout允许开发者按照视图之间的相对位置进行布局,可以更容易实现复杂的布局结构,而LinearLayout则局限于线性的布局方式。
#### 4.3 使用场景选择
一般来说,对于线性的简单布局,使用LinearLayout会更加高效和方便。而对于复杂且需要灵活性的布局,RelativeLayout则是更好的选择。
以上是LinearLayout与RelativeLayout在性能、灵活性和使用场景方面的比较。在实际开发中,开发者需要根据具体需求和布局复杂程度来选择合适的布局方式。
接下来,我们将通过示例代码和实际案例进一步验证这些比较结果。
# 5. 最佳实践:如何选择合适的布局方式
在Android开发中,选择合适的布局方式至关重要。下面将介绍一些最佳实践,以帮助开发者更好地选择适合的布局方式。
#### 5.1 根据需求选择合适的布局方式
在选择布局方式时,首先要根据页面的布局需求来确定使用LinearLayout还是RelativeLayout。如果页面结构比较简单,且布局是线性的(如垂直或水平排列),那么使用LinearLayout可能更加合适。如果页面结构比较复杂,需要控件之间有相对位置关系,那么RelativeLayout可能更为适用。
#### 5.2 优化布局结构提升性能
在布局过程中,为了提升性能,开发者可以考虑以下几点优化方法:
- **避免过深的布局层级:** 减少嵌套层级,可以减少布局解析和绘制所消耗的时间。
- **使用合适的布局方式:** 根据实际需求选择最合适的布局方式,避免不必要的嵌套和复杂性。
- **合理使用布局属性:** 使用布局属性来控制控件的位置和大小,避免使用过多的固定尺寸,提高布局的灵活性和适应性。
通过以上最佳实践,开发者可以更好地选择合适的布局方式,并优化布局结构以提升应用性能。
以上是关于如何选择合适的布局方式的建议,希望对你有所帮助。
# 6. 实战案例分享
在本节中,我们将分享两个实战案例,分别使用LinearLayout和RelativeLayout来实现页面布局。通过这两个案例,我们可以更好地理解和掌握LinearLayout和RelativeLayout的具体应用场景,以及它们在实际开发中的灵活运用。
#### 6.1 使用LinearLayout实现简单页面布局
```java
// 代码示例
public class LinearLayoutActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_linear_layout);
// 布局文件:activity_linear_layout.xml
// 使用LinearLayout实现简单页面布局
LinearLayout linearLayout = findViewById(R.id.linear_layout);
// 添加TextView
TextView textView1 = new TextView(this);
textView1.setText("Hello,");
linearLayout.addView(textView1);
TextView textView2 = new TextView(this);
textView2.setText("World!");
linearLayout.addView(textView2);
}
}
```
**代码总结:** 上述代码演示了如何使用LinearLayout在Android中实现简单的页面布局。通过在布局文件中定义LinearLayout,并在Java代码中动态添加TextView,实现了简单的线性布局效果。
**结果说明:** 运行该示例程序后,将在页面上看到“Hello,”和“World!”两个文本依次水平排列的效果。
#### 6.2 使用RelativeLayout解决复杂页面布局问题
```java
// 代码示例
public class RelativeLayoutActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_relative_layout);
// 布局文件:activity_relative_layout.xml
// 使用RelativeLayout解决复杂页面布局问题
RelativeLayout relativeLayout = findViewById(R.id.relative_layout);
// 添加ImageView
ImageView imageView = new ImageView(this);
imageView.setImageResource(R.drawable.image);
RelativeLayout.LayoutParams params = new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.WRAP_CONTENT,
RelativeLayout.LayoutParams.WRAP_CONTENT
);
params.addRule(RelativeLayout.ALIGN_PARENT_RIGHT);
relativeLayout.addView(imageView, params);
}
}
```
**代码总结:** 以上代码展示了如何使用RelativeLayout在Android中解决复杂页面布局问题。通过在布局文件中定义RelativeLayout,并在Java代码中动态添加ImageView,并设置其在父布局中的相对位置,实现了复杂页面布局的效果。
**结果说明:** 运行该示例程序后,将在页面上看到一个图片被放置在右侧的效果。
通过以上两个实战案例的分享,我们可以更好地理解LinearLayout和RelativeLayout的灵活运用方式,以及它们分别在简单和复杂页面布局中的作用和优势。
0
0
相关推荐
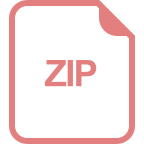