与Spring集成:使用MyBatis进行数据访问
发布时间: 2023-12-08 14:13:08 阅读量: 27 订阅数: 49 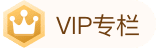
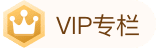
# 1. 介绍Spring和MyBatis
## 1.1 Spring和MyBatis的概述
Spring是一个开源的Java框架,提供了广泛的功能和特性,包括IoC(控制反转)和AOP(面向切面编程),以及对事务管理、数据访问、Web开发等方面的支持。
MyBatis是一种持久层框架,它可以简化数据库访问的代码编写,并提供了强大的SQL映射功能。它可以将数据库中的记录映射到Java对象中,并且提供了一套灵活的机制来执行SQL语句。
Spring和MyBatis的结合可以充分发挥它们各自的优势,使得数据访问变得更加简洁和高效。
## 1.2 如何在项目中使用Spring和MyBatis
在项目中使用Spring和MyBatis的组合,通常需要进行以下几个步骤:
1. 添加Spring和MyBatis的依赖库到项目中,可以使用Maven或Gradle进行管理。
2. 配置Spring的ApplicationContext,通常在一个XML文件中定义Bean的配置信息,包括数据源的配置、事务管理的配置等。
3. 配置MyBatis的SqlSessionFactory,通过配置文件或Java代码来创建一个SqlSessionFactory对象,用于管理数据库会话。
4. 创建实体类,用于映射数据库表的记录。
5. 编写MyBatis的Mapper接口,定义访问数据库的方法。
6. 编写MyBatis的映射文件,将SQL语句与Mapper接口的方法进行映射。
7. 使用Spring的依赖注入机制,将Mapper接口注入到Service层或Controller层中,以便在业务逻辑中使用。
8. 使用Spring的事务管理机制,对需要进行事务管理的方法进行标注,以保证数据的一致性和完整性。
## 1.3 Spring和MyBatis集成的优势
集成Spring和MyBatis可以带来以下几个优势:
1. 简化数据访问的代码:使用MyBatis可以避免手动编写JDBC的代码,而使用Spring可以进一步简化配置和管理。
2. 提供灵活的事务管理:Spring提供了声明式事务管理的机制,可以根据需要配置事务的传播行为和隔离级别,并且可以灵活地控制事务的边界。
3. 充分利用Spring的特性:Spring提供了许多特性,如依赖注入、AOP等,可以进一步简化开发和提高代码的可维护性。
4. 可以与其他Spring组件集成:由于Spring的开放性和扩展性,可以方便地集成其他的Spring组件,如Spring MVC、Spring Security等,从而构建更加完整和可扩展的应用。
接下来的章节将详细介绍如何配置Spring和MyBatis,以及如何使用它们进行数据访问。
# 2. 配置Spring和MyBatis
### 2.1 配置Spring的ApplicationContext
在使用Spring和MyBatis进行集成之前,首先需要配置Spring的ApplicationContext。ApplicationContext是Spring的核心容器,负责管理和组织Bean的创建和配置。
在项目中创建一个XML配置文件,命名为"applicationContext.xml",并添加以下内容:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<!-- 配置扫描包 -->
<context:component-scan base-package="com.example.myproject" />
<!-- 配置数据源 -->
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/myprojectdb" />
<property name="username" value="root" />
<property name="password" value="password" />
</bean>
<!-- 配置Spring和MyBatis的整合 -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="mapperLocations" value="classpath*:mapper/*.xml" />
</bean>
<!-- 配置MyBatis的Mapper接口扫描 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.myproject.mapper" />
</bean>
<!-- 配置事务管理器 -->
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<!-- 启用Spring的声明式事务管理 -->
<tx:annotation-driven transaction-manager="transactionManager" />
</beans>
```
在上面的配置文件中,我们首先使用`<context:component-scan>`标签配置了需要扫描的基础包,以使Spring能够自动检测和实例化我们的Bean。
然后,我们配置了数据源(DataSource),这里使用的是MySQL数据库,并提供了连接信息。
接着,我们使用`<bean>`标签配置了Spring和MyBatis的整合,通过`<property>`子元素将数据源注入到了SqlSessionFactoryBean中,并指定了Mapper映射文件的位置。
接下来,我们使用`<bean>`标签配置了Mapper接口的扫描器,指定了Mapper接口所在的包。
最后,通过`<bean>`标签配置了事务管理器,并启用了Spring的声明式事务管理。
### 2.2 配置MyBatis的SqlSessionFactory
在使用MyBatis进行数据访问时,需要配置SqlSessionFactory,该对象是MyBatis的核心工厂类,负责创建SqlSession。
在Spring的配置文件"applicationContext.xml"中,我们已经配置了SqlSessionFactory,具体配置如下:
```xml
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="mapperLocations" value="classpath*:mapper/*.xml" />
</bean>
```
在上述配置中,我们指定了数据源(dataSource)和Mapper映射文件(mapperLocations)的位置。
### 2.3 数据库连接配置
在Spring的配置文件"applicationContext.xml"中,我们已经配置了数据源(dataSource),具体配置如下:
```xml
<bean id="dataSource" class="org.springframework.jdbc.datasour
```
0
0
相关推荐
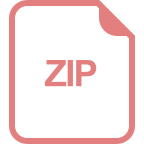
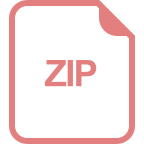
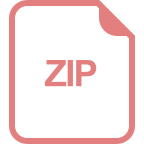
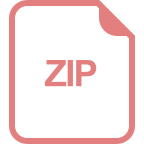
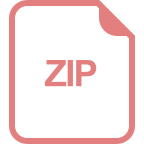
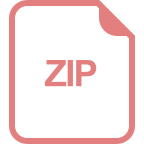