实现碰撞检测和物理效果的方法
发布时间: 2024-02-10 20:57:07 阅读量: 46 订阅数: 50 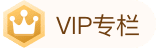
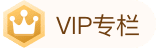
# 1. 引言
## 1.1 问题背景
在计算机图形学、游戏开发、虚拟现实等领域中,碰撞检测和物理效果模拟是非常重要的技术。在实现真实感的物理动作和交互性时,需要准确地检测物体之间的碰撞,并模拟物体的运动、碰撞、弹射等物理效果。
## 1.2 碰撞检测和物理效果的重要性
碰撞检测是计算机图形学中的一个关键问题,在许多应用中都有着广泛的应用。无论是游戏中的角色与障碍物碰撞、虚拟环境中的物体交互、还是CAD系统中的模型检测,都需要高效准确地进行碰撞检测。
同时,物理效果模拟也是虚拟环境、游戏开发等领域不可或缺的一部分。通过对物体的运动、碰撞、弹性等效果进行准确模拟,可以提升用户体验,使场景更加逼真。
因此,对于碰撞检测和物理效果的深入研究和实践应用具有重要意义。接下来的章节将对碰撞检测基础、物理效果模拟、高级碰撞检测技术、物理效果优化与性能提升等方面展开详细讨论。
# 2. 碰撞检测基础
在计算机图形学和游戏开发中,碰撞检测是一个非常重要的技术。通过检测物体之间是否发生碰撞,我们能够实现各种交互效果和物理模拟。本章将介绍一些常见的碰撞检测方法和算法,包括AABB碰撞检测、球体碰撞检测、网格碰撞检测以及碰撞检测算法的选择与优化。
### 2.1 AABB碰撞检测
AABB(Axis-Aligned Bounding Box)是一种以坐标轴对齐的包围盒,它可以用轴对齐的最小矩形来简单地表示一个物体的范围。AABB碰撞检测即判断两个包围盒是否相交。
```python
class AABB:
def __init__(self, x, y, z, width, height, depth):
self.x = x
self.y = y
self.z = z
self.width = width
self.height = height
self.depth = depth
def intersects(self, other):
if (self.x < other.x + other.width and
self.x + self.width > other.x and
self.y < other.y + other.height and
self.y + self.height > other.y and
self.z < other.z + other.depth and
self.z + self.depth > other.z):
return True
return False
```
上述代码展示了一个简单的AABB类的实现,其中`intersects()`方法用于判断两个包围盒是否相交。通过比较两个包围盒在X、Y、Z轴上的范围是否有重叠,我们可以得出碰撞检测的结果。
### 2.2 球体碰撞检测
球体碰撞检测也是常见的一种碰撞检测方法。对于两个球体,如果它们的距离小于它们的半径之和,那么它们就发生了碰撞。
```java
public class Sphere {
private Vector3D position;
private float radius;
public Sphere(Vector3D position, float radius) {
this.position = position;
this.radius = radius;
}
public boolean intersects(Sphere other) {
float distance = position.distanceTo(other.position);
return distance < (radius + other.radius);
}
}
```
上述代码展示了一个简单的Sphere类的实现,其中`intersects()`方法用于判断两个球体是否相交。通过计算两个球体中心点之间的距离,我们可以判断碰撞是否发生。
### 2.3 网格碰撞检测
网格碰撞检测适用于复杂的模型碰撞检测。在网格碰撞检测中,我们将模型分解为一个个三角形,并对每个三角形进行碰撞检测。
```javascript
class Mesh {
constructor(vertices, triangles) {
this.vertices = vertices;
this.triangles = triangles;
}
intersects(other) {
for (let i = 0; i < this.triangles.length; i++) {
let triangle = this.triangles[i];
let vertices = [
this.vertices[triangle[0]],
this.vertices[triangle[1]],
this.vertices[triangle[2]]
];
if (triangleIntersectsTriangle(vertices, other)) {
return true;
}
}
return false;
}
}
function triangleIntersectsTriangle(triangle1, triangle2) {
// 碰撞检测方法的实现
}
```
上述代码展示了一个简单的Mesh类的实现,其中`intersects()`方法用于判断模型和其他物体是否相交。在每个三角形上进行碰撞检测,如果存在相交的情况,则判断碰撞发生。
### 2.4 碰撞检测算法的选择与优化
在实际应用中,我们需要根据具体的场景选择合适的碰撞检测算法。AABB碰撞检测适用于简单的物体,而球体碰撞检测适用于大部分情况。对于复杂的模型,网格碰撞检测是更好的选择,但相对来说计算量较大。因此,在选用碰撞检测算法时需要权衡计算性能和准确性。此外,我们还可以通过空间分割技术如包围盒层次结构(Bounding
0
0
相关推荐
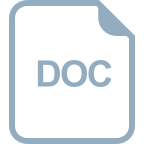
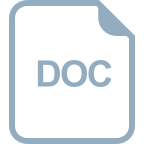
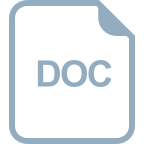
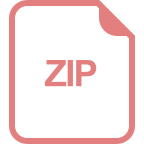
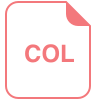
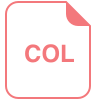
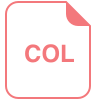
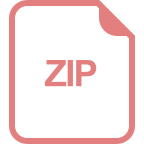
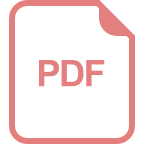