【Qt Creator配置OpenCV环境指南】:从零基础到项目实战,打造高效开发环境
发布时间: 2024-08-06 19:34:06 阅读量: 22 订阅数: 15 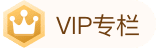
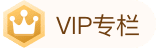
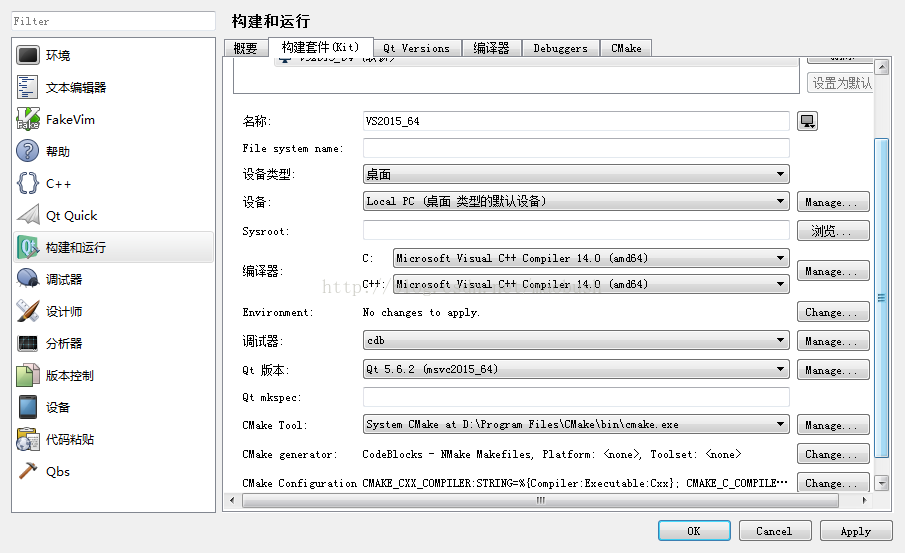
# 1. Qt Creator简介及环境搭建
Qt Creator是一款功能强大的跨平台集成开发环境(IDE),专为开发Qt应用程序而设计。它提供了一系列工具和功能,简化了开发过程,包括:
- 代码编辑器:支持语法高亮、自动补全和代码导航。
- 调试器:用于调试应用程序,查找错误并分析性能。
- 项目管理:管理项目文件、构建设置和版本控制。
- UI设计器:用于设计和预览图形用户界面(GUI)。
# 2. OpenCV基础知识及Qt Creator集成
### 2.1 OpenCV概述及安装
**OpenCV概述**
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉和机器学习库,广泛应用于图像处理、视频分析、计算机视觉和机器学习等领域。它提供了丰富的函数和算法,涵盖图像处理、特征提取、目标检测、机器学习等方面。
**OpenCV安装**
OpenCV的安装因操作系统而异。以下是一些常见操作系统的安装步骤:
* **Windows:**
* 下载OpenCV安装包(https://opencv.org/releases/)
* 运行安装程序并按照提示进行安装
* **macOS:**
* 使用Homebrew安装:`brew install opencv`
* 使用MacPorts安装:`sudo port install opencv`
* **Linux:**
* 使用apt-get安装(Ubuntu/Debian):`sudo apt-get install libopencv-dev`
* 使用yum安装(CentOS/Red Hat):`sudo yum install opencv-devel`
### 2.2 Qt Creator中集成OpenCV
**Qt Creator集成OpenCV步骤**
将OpenCV集成到Qt Creator中需要进行以下步骤:
1. **配置Qt Creator:**
* 打开Qt Creator,转到“工具”->“选项”
* 在“构建和运行”->“套件”中,添加OpenCV库的路径
2. **添加OpenCV头文件:**
* 在项目中,右键单击“头文件”文件夹,选择“添加新”->“头文件”
* 输入OpenCV头文件路径(例如:`#include <opencv2/opencv.hpp>`)
3. **链接OpenCV库:**
* 在项目中,右键单击“库”文件夹,选择“添加库”
* 选择OpenCV库(例如:`opencv_core`、`opencv_imgproc`)
4. **验证集成:**
* 在项目中,创建一个新的源文件(`.cpp`)
* 使用OpenCV函数编写代码,例如:`cv::Mat image = cv::imread("image.jpg");`
* 构建并运行项目,验证OpenCV是否已成功集成
**代码示例**
```cpp
#include <opencv2/opencv.hpp>
int main() {
// 读取图像
cv::Mat image = cv::imread("image.jpg");
// 显示图像
cv::imshow("Image", image);
// 等待用户输入
cv::waitKey(0);
return 0;
}
```
**代码逻辑分析**
* `cv::imread("image.jpg")`:读取图像文件并将其存储在`image`矩阵中。
* `cv::imshow("Image", image)`:显示图像并将其命名为“Image”。
* `cv::waitKey(0)`:等待用户按任意键关闭图像窗口。
# 3. 图像处理基础
### 3.1.1 图像读取与显示
#### 图像读取
在 Qt Creator 中读取图像,可以使用 `QImage` 类。`QImage` 提供了多种图像格式的读取方法,例如:
```cpp
QImage image = QImage("image.jpg");
```
其中,`image.jpg` 为要读取的图像文件路径。
#### 图像显示
读取图像后,可以通过 `QLabel` 类显示图像。`QLabel` 提供了 `setPixmap()` 方法来设置图像:
```cpp
QLabel label;
label.setPixmap(QPixmap::fromImage(image));
```
### 3.1.2 图像转换与处理
#### 图像转换
Qt Creator 提供了多种图像转换方法,例如:
- `convertTo()`:将图像转换为指定的格式,如 `QImage::Format_RGB32`。
- `scaled()`:缩放图像到指定的大小。
- `rotated()`:旋转图像到指定的角度。
#### 图像处理
Qt Creator 还提供了图像处理方法,例如:
- `blur()`:模糊图像。
- `sharpen()`:锐化图像。
- `threshold()`:阈值化图像。
#### 代码示例
以下代码示例演示了图像读取、显示、转换和处理:
```cpp
// 图像读取
QImage image = QImage("image.jpg");
// 图像显示
QLabel label;
label.setPixmap(QPixmap::fromImage(image));
// 图像转换
QImage convertedImage = image.convertTo(QImage::Format_RGB32);
// 图像处理
QImage blurredImage = convertedImage.blurred();
QImage sharpenedImage = convertedImage.sharpened();
```
#### 逻辑分析
上述代码首先读取图像,然后将其显示在标签中。接着,将图像转换为 RGB32 格式,并对其进行模糊和锐化处理。
#### 参数说明
- `QImage::Format_RGB32`:指定图像格式为 32 位 RGB。
- `blurred()`:模糊图像的半径,默认为 1。
- `sharpened()`:锐化图像的半径,默认为 1。
# 4.1 视频处理与分析
### 4.1.1 视频读取与播放
**视频读取**
在 Qt Creator 中使用 OpenCV 读取视频文件,可以使用 `VideoCapture` 类。`VideoCapture` 类的构造函数接受一个视频文件路径作为参数,用于打开视频文件。
```cpp
VideoCapture videoCapture("path/to/video.mp4");
```
**视频播放**
要播放视频,可以使用 `VideoWriter` 类。`VideoWriter` 类的构造函数接受一个视频文件路径、视频编码器、帧率和帧大小作为参数。
```cpp
VideoWriter videoWriter("path/to/output.mp4", VideoWriter::fourcc('M', 'J', 'P', 'G'), 30, Size(640, 480));
```
**逐帧读取视频**
要逐帧读取视频,可以使用 `read()` 方法。`read()` 方法返回一个布尔值,指示是否成功读取帧。如果成功读取帧,则返回 `true`,否则返回 `false`。
```cpp
Mat frame;
while (videoCapture.read(frame)) {
// 处理帧
}
```
### 4.1.2 视频分析与跟踪
**运动检测**
运动检测是视频分析中的一项基本任务。它涉及检测视频中运动的区域。可以使用背景减除算法来检测运动。
```cpp
Mat background;
Ptr<BackgroundSubtractor> bgSubtractor = createBackgroundSubtractorMOG2();
while (videoCapture.read(frame)) {
bgSubtractor->apply(frame, foregroundMask);
// 处理前景掩码
}
```
**目标跟踪**
目标跟踪涉及跟踪视频中移动对象的运动。可以使用多种算法进行目标跟踪,例如 Kalman 滤波器和 Mean-Shift 算法。
```cpp
Ptr<Tracker> tracker = TrackerKCF::create();
Rect2d bbox;
while (videoCapture.read(frame)) {
if (tracker->init(frame, bbox)) {
while (videoCapture.read(frame)) {
tracker->update(frame, bbox);
// 处理边界框
}
}
}
```
**事件检测**
事件检测涉及检测视频中发生的特定事件。可以使用基于规则的算法或机器学习算法来检测事件。
```cpp
Mat eventMask;
Ptr<EventDetector> eventDetector = createEventDetector("event_type");
while (videoCapture.read(frame)) {
eventDetector->detect(frame, eventMask);
// 处理事件掩码
}
```
# 5. Qt Creator开发OpenCV项目优化
### 5.1 性能优化技巧
#### 5.1.1 代码优化
- **避免不必要的内存分配和释放:**使用智能指针或引用计数,避免频繁的内存分配和释放操作。
- **优化循环:**使用范围循环、并行循环或 SIMD 指令优化循环性能。
- **使用缓存:**将经常访问的数据存储在缓存中,减少内存访问次数。
- **重用对象:**避免重复创建对象,重用已创建的对象。
- **使用适当的数据结构:**根据数据访问模式选择合适的容器或数据结构,优化查找和遍历性能。
#### 5.1.2 算法优化
- **选择高效的算法:**研究并选择适合特定任务的高效算法。
- **并行化算法:**利用多核处理器或 GPU 并行化算法,提高计算速度。
- **减少数据冗余:**避免重复计算相同的数据,通过缓存或优化算法减少冗余。
- **利用图像处理库:**利用 OpenCV 等图像处理库提供的优化算法,避免重复实现。
- **优化图像格式:**选择合适的图像格式,如 JPEG、PNG 或 WebP,以优化图像加载和处理性能。
### 5.2 调试与故障排除
#### 5.2.1 常见问题及解决方法
| 问题 | 解决方法 |
|---|---|
| OpenCV 库未找到 | 确保 OpenCV 库已正确安装并添加到 Qt Creator 的构建路径中。 |
| 图像加载失败 | 检查图像文件路径是否正确,并确保图像格式受 OpenCV 支持。 |
| 视频播放卡顿 | 优化视频解码器设置,确保硬件加速可用,并减少视频分辨率或帧率。 |
| 算法结果不准确 | 检查算法参数是否正确,并尝试使用不同的算法或优化数据预处理。 |
| 应用程序崩溃 | 检查代码中是否存在内存泄漏或未处理的异常,并使用调试器进行故障排除。 |
#### 5.2.2 调试工具与技巧
- **Qt Creator 调试器:**使用 Qt Creator 内置的调试器,设置断点、检查变量和调用堆栈。
- **GDB 调试器:**使用 GDB 调试器进行更高级的调试,如反汇编和内存转储。
- **日志记录:**在代码中添加日志语句,记录重要事件和错误信息,便于故障排除。
- **单元测试:**编写单元测试,隔离和测试代码的特定部分,帮助识别错误和提高代码稳定性。
- **性能分析工具:**使用 Qt Creator 的性能分析工具或其他第三方工具,分析代码性能瓶颈并进行优化。
# 6.1 人脸识别系统
### Qt Creator与OpenCV人脸识别系统开发
人脸识别系统是一种利用计算机视觉技术识别和验证人脸身份的系统。在Qt Creator中,我们可以使用OpenCV库开发人脸识别系统。
**流程图:**
```mermaid
graph LR
subgraph 人脸识别系统
Qt Creator[Qt Creator] --> OpenCV[OpenCV]
OpenCV[OpenCV] --> Haar级联分类器[Haar级联分类器]
Haar级联分类器[Haar级联分类器] --> 人脸检测[人脸检测]
人脸检测[人脸检测] --> 特征提取[特征提取]
特征提取[特征提取] --> 人脸识别[人脸识别]
end
```
**步骤:**
1. **Haar级联分类器训练:**使用OpenCV的Haar级联分类器训练器训练人脸检测模型。
2. **人脸检测:**使用训练好的模型在输入图像中检测人脸。
3. **特征提取:**从检测到的人脸中提取特征,例如人脸几何特征、纹理特征等。
4. **人脸识别:**使用特征匹配算法,将提取的特征与已知人脸数据库进行匹配,识别出人脸身份。
**代码示例:**
```cpp
#include <opencv2/opencv.hpp>
using namespace cv;
int main() {
// 1. Haar级联分类器训练
CascadeClassifier face_cascade;
face_cascade.load("haarcascade_frontalface_default.xml");
// 2. 人脸检测
Mat image = imread("input.jpg");
std::vector<Rect> faces;
face_cascade.detectMultiScale(image, faces);
// 3. 特征提取
std::vector<Mat> face_features;
for (const Rect& face : faces) {
Mat face_roi = image(face);
face_features.push_back(face_roi);
}
// 4. 人脸识别
Ptr<FaceRecognizer> recognizer = createEigenFaceRecognizer();
recognizer->train(face_features, std::vector<int>(face_features.size(), 0));
int label = recognizer->predict(face_features[0]);
std::cout << "识别结果:" << label << std::endl;
return 0;
}
```
0
0
相关推荐
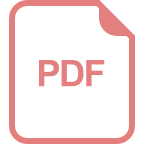
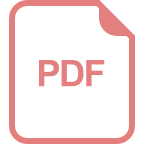





