MySQL数据库数据转JSON实战指南:5步搞定数据转换
发布时间: 2024-08-04 07:42:49 阅读量: 36 订阅数: 31 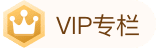
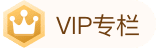
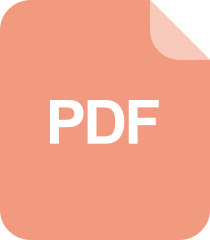
使用python将mysql数据库的数据转换为json数据的方法

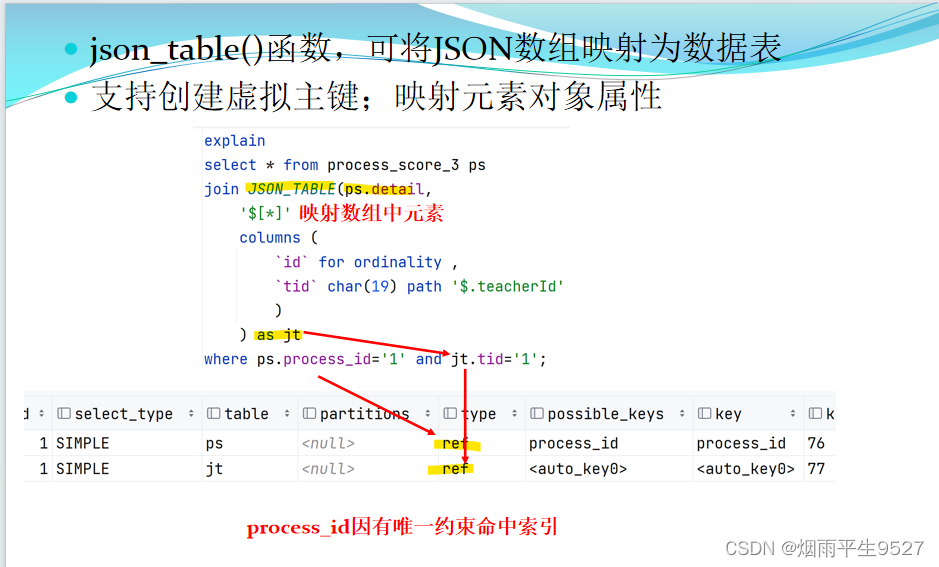
# 1. MySQL数据库和JSON简介**
**1.1 MySQL数据库**
MySQL是一种流行的关系型数据库管理系统(RDBMS),以其高性能、可靠性和可扩展性而闻名。它广泛用于各种应用程序,从小型网站到大型企业系统。MySQL使用结构化查询语言(SQL)进行数据操作和管理。
**1.2 JSON**
JSON(JavaScript对象表示法)是一种轻量级数据交换格式,基于JavaScript对象语法。它是一种独立于语言的数据表示方式,用于在不同的系统和应用程序之间传输数据。JSON以其可读性、易解析性和可扩展性而著称。
# 2. 数据转换理论基础
### 2.1 JSON数据结构与MySQL数据结构对比
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,广泛用于Web开发和数据传输。其数据结构主要包括对象和数组,其中对象由键值对组成,数组由有序元素组成。
MySQL是一种关系型数据库管理系统,其数据结构主要包括表、行和列。表由行组成,行由列组成。列具有特定的数据类型,如整数、字符串、日期等。
**对比表**
| 特征 | JSON | MySQL |
|---|---|---|
| 数据结构 | 对象、数组 | 表、行、列 |
| 数据类型 | 无固定类型 | 固定类型 |
| 嵌套 | 支持嵌套对象和数组 | 支持嵌套表和子查询 |
| 键 | 对象键为字符串 | 表列名为字符串 |
| 值 | 对象值可以是任何类型 | 表列值必须符合指定的数据类型 |
| 顺序 | 数组元素有顺序 | 表行和列无顺序 |
### 2.2 数据转换算法与优化策略
数据转换算法是指将MySQL数据结构转换为JSON数据结构的具体方法。常见的算法包括:
- **递归算法:**深度遍历MySQL数据结构,递归地将子结构转换为JSON对象或数组。
- **迭代算法:**使用循环逐层遍历MySQL数据结构,逐个将数据元素转换为JSON对象或数组。
- **流式算法:**将MySQL数据结构作为流式数据处理,边读取边转换,提高效率。
**优化策略**
- **选择合适的算法:**根据数据结构的复杂度和转换需求,选择最合适的算法。
- **并行处理:**将大数据集拆分为多个子数据集,并行转换,提高整体效率。
- **缓存机制:**将转换后的JSON数据缓存起来,避免重复转换,提高性能。
- **数据类型转换:**根据JSON数据结构的要求,将MySQL数据类型转换为相应的JSON数据类型。
- **数据过滤:**根据需要,过滤掉不需要转换的数据,减少转换开销。
**代码示例**
```python
# 使用递归算法将MySQL查询结果转换为JSON对象
def mysql_to_json_recursive(cursor):
rows = cursor.fetchall()
json_data = []
for row in rows:
json_obj = {}
for i, col in enumerate(cursor.description):
json_obj[col[0]] = row[i]
json_data.append(json_obj)
return json_data
```
**逻辑分析**
该代码使用递归算法将MySQL查询结果转换为JSON对象。它遍历查询结果中的每一行,并为每一行创建一个JSON对象。JSON对象的键是查询结果中列的名称,值是查询结果中相应列的值。
**参数说明**
* `cursor`:MySQL查询结果游标。
# 3. 数据转换实践操作
### 3.1 使用MySQL内置函数进行数据转换
MySQL内置了多种函数,可以方便地将MySQL数据转换为JSON格式。常用的函数包括:
- **JSON_OBJECT()函数:**将一组键值对转换为JSON对象。
- **JSON_ARRAY()函数:**将一组值转换为JSON数组。
#### 3.1.1 JSON_OBJECT()函数
**语法:**
```sql
JSON_OBJECT(key1, value1, key2, value2, ...)
```
**参数:**
- **key1, key2, ...:**键名,类型为字符串。
- **value1, value2, ...:**键值,可以是任何数据类型。
**示例:**
将以下表数据转换为JSON对象:
| id | name | age |
|---|---|---|
| 1 | John | 25 |
| 2 | Mary | 30 |
```sql
SELECT JSON_OBJECT('id', id, 'name', name, 'age', age) AS json_data
FROM table_name;
```
**结果:**
```json
{"id": 1, "name": "John", "age": 25}
```
#### 3.1.2 JSON_ARRAY()函数
**语法:**
```sql
JSON_ARRAY(value1, value2, ...)
```
**参数:**
- **value1, value2, ...:**数组元素,可以是任何数据类型。
**示例:**
将以下表数据转换为JSON数组:
| id | name |
|---|---|
| 1 | John |
| 2 | Mary |
```sql
SELECT JSON_ARRAY(id, name) AS json_data
FROM table_name;
```
**结果:**
```json
[1, "John", 2, "Mary"]
```
### 3.2 使用第三方工具进行数据转换
除了MySQL内置函数,还可以使用第三方工具进行数据转换。常用的工具包括:
- **mysqldump命令:**MySQL官方提供的命令行工具,可以将数据库数据导出为多种格式,包括JSON。
- **jq命令:**一个命令行工具,专门用于处理JSON数据,可以方便地对JSON数据进行转换和查询。
#### 3.2.1 mysqldump命令
**语法:**
```
mysqldump [options] database_name table_name > output_file.json
```
**参数:**
- **database_name:**要导出的数据库名称。
- **table_name:**要导出的表名称。
- **output_file.json:**输出JSON文件的文件名。
**示例:**
将`table_name`表数据导出为JSON文件:
```
mysqldump -u root -p database_name table_name > table_name.json
```
#### 3.2.2 jq命令
**语法:**
```
jq 'filter' input_file.json
```
**参数:**
- **filter:**用于过滤和转换JSON数据的表达式。
- **input_file.json:**输入JSON文件的文件名。
**示例:**
提取JSON文件中所有`name`字段的值:
```
jq '.[].name' input_file.json
```
# 4. 数据转换高级应用
### 4.1 批量数据转换优化
#### 4.1.1 并行处理技术
当需要转换大量数据时,采用并行处理技术可以显著提升效率。MySQL提供了`PARALLEL`选项,允许在多个线程上并行执行查询。
```sql
SELECT * FROM table_name
WHERE condition
PARALLEL 4;
```
其中,`PARALLEL 4`表示使用4个线程并行执行查询。并行线程数应根据服务器硬件资源和数据量合理设置,过多线程反而会降低性能。
#### 4.1.2 缓存机制优化
MySQL使用查询缓存来存储最近执行过的查询结果,以提高后续相同查询的执行效率。对于频繁执行的数据转换查询,可以考虑使用`SQL_CACHE`关键字强制使用查询缓存。
```sql
SELECT /*+ SQL_CACHE */ * FROM table_name
WHERE condition;
```
不过,需要注意的是,查询缓存可能会导致数据不一致问题,因此应谨慎使用。
### 4.2 复杂数据结构转换
#### 4.2.1 嵌套数据结构转换
嵌套数据结构是指包含其他数据结构的数据结构。例如,一个JSON对象可以包含其他JSON对象或数组。
```json
{
"name": "John Doe",
"address": {
"street": "123 Main Street",
"city": "Anytown",
"state": "CA",
"zip": "12345"
},
"phone_numbers": [
"555-123-4567",
"555-234-5678"
]
}
```
要将嵌套数据结构转换为MySQL数据,可以采用递归的方式。首先将外层数据结构转换为MySQL表,然后依次将内层数据结构转换为子表。
#### 4.2.2 多表关联数据转换
多表关联数据是指多个表之间存在关联关系的数据。例如,订单表和订单明细表之间存在一对多的关联关系。
```sql
CREATE TABLE orders (
order_id INT NOT NULL,
customer_id INT NOT NULL,
order_date DATETIME NOT NULL,
PRIMARY KEY (order_id)
);
CREATE TABLE order_details (
order_id INT NOT NULL,
product_id INT NOT NULL,
quantity INT NOT NULL,
PRIMARY KEY (order_id, product_id),
FOREIGN KEY (order_id) REFERENCES orders (order_id)
);
```
要将多表关联数据转换为JSON,可以采用嵌套查询的方式。首先查询主表数据,然后依次查询关联表数据,并将其嵌套到主表数据中。
```sql
SELECT * FROM orders o
LEFT JOIN order_details od ON o.order_id = od.order_id;
```
# 5. 数据转换实战案例
### 5.1 电商订单数据转JSON
**场景描述:**
需要将电商平台的订单数据转换成JSON格式,以便于后续的分析和处理。订单数据包含订单号、商品名称、数量、单价、总价等信息。
**数据转换步骤:**
1. **查询订单数据:**
```sql
SELECT
order_id,
product_name,
quantity,
unit_price,
total_price
FROM orders;
```
2. **使用JSON_OBJECT()函数构建JSON对象:**
```sql
SELECT
JSON_OBJECT(
'order_id', order_id,
'product_name', product_name,
'quantity', quantity,
'unit_price', unit_price,
'total_price', total_price
) AS order_json
FROM orders;
```
3. **结果展示:**
执行上述查询后,将得到如下JSON结果:
```json
{
"order_id": 1,
"product_name": "iPhone 13 Pro Max",
"quantity": 1,
"unit_price": 1299,
"total_price": 1299
}
```
### 5.2 用户信息数据转JSON
**场景描述:**
需要将用户平台的用户信息数据转换成JSON格式,以便于后续的个性化推荐和营销活动。用户信息数据包含用户ID、姓名、年龄、性别、职业等信息。
**数据转换步骤:**
1. **查询用户信息:**
```sql
SELECT
user_id,
name,
age,
gender,
occupation
FROM users;
```
2. **使用JSON_OBJECT()函数构建JSON对象:**
```sql
SELECT
JSON_OBJECT(
'user_id', user_id,
'name', name,
'age', age,
'gender', gender,
'occupation', occupation
) AS user_json
FROM users;
```
3. **结果展示:**
执行上述查询后,将得到如下JSON结果:
```json
{
"user_id": 1,
"name": "John Doe",
"age": 30,
"gender": "male",
"occupation": "software engineer"
}
```
# 6. 数据转换常见问题与解决方案
### 6.1 数据转换失败原因分析
在进行数据转换时,可能会遇到各种各样的错误和问题。以下是一些常见的数据转换失败原因:
- **数据类型不匹配:**MySQL数据类型与JSON数据类型不匹配,导致转换失败。例如,MySQL中的整数类型无法直接转换为JSON中的浮点数类型。
- **数据格式不正确:**JSON数据格式不正确,导致转换失败。例如,缺少引号、逗号或大括号。
- **内存不足:**转换大量数据时,可能会遇到内存不足的问题,导致转换失败。
- **权限不足:**用户没有足够的权限执行数据转换操作,导致转换失败。
- **语法错误:**转换语句中存在语法错误,导致转换失败。
### 6.2 数据转换性能优化建议
为了提高数据转换的性能,可以采用以下优化建议:
- **使用索引:**在涉及到大量数据的转换操作中,使用索引可以显著提高查询速度。
- **批量处理:**将大量数据分批处理,而不是一次性转换所有数据,可以减少内存消耗和提高性能。
- **使用并行处理:**在支持并行处理的数据库系统中,可以利用多核CPU的优势,并行执行转换操作。
- **优化转换算法:**选择合适的转换算法,可以提高转换效率。例如,对于复杂的数据结构转换,可以使用递归算法。
- **缓存机制:**对于经常需要转换的数据,可以将转换结果缓存起来,避免重复转换。
0
0
相关推荐
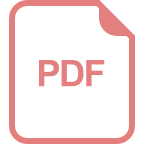
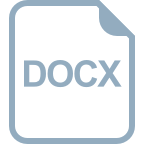
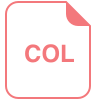
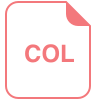
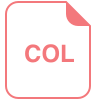
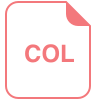
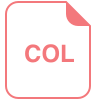
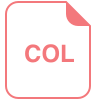