Java编程高手的工具箱:复杂度分析工具使用攻略,打造无懈可击的代码
发布时间: 2024-08-30 04:11:18 阅读量: 92 订阅数: 40 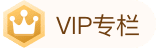
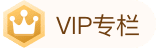
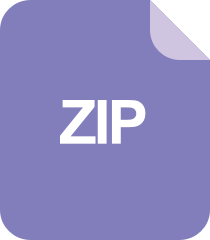
java+sql server项目之科帮网计算机配件报价系统源代码.zip
.png)
# 1. 代码复杂度分析的理论基础
代码复杂度分析是衡量软件质量的一个重要指标,尤其在维护和优化大型系统时更是不可或缺。本章将介绍复杂度分析的相关理论基础,为后文使用各种工具进行代码复杂度分析打下理论基石。
## 理论背景
在软件工程领域,"复杂度"通常指的是算法或程序在解决问题时所需的基本操作数量。它由两部分组成:时间复杂度和空间复杂度。时间复杂度反映了程序运行所需的时间量,而空间复杂度则关注程序运行时占用的存储空间。
## 复杂度的度量
复杂度的度量一般通过大O符号表示,例如O(n), O(log n), O(n^2)等,其中n表示输入规模。不同的算法根据问题的性质,其复杂度也会有所不同。理解这些基本概念对于后续分析工具的应用至关重要。
## 实际意义
复杂度分析在实际开发中非常有用,它可以帮助开发者识别出潜在的性能瓶颈。通过优化算法复杂度,可以提高程序运行效率,降低资源消耗,从而提升整体软件性能。这是建立在理论基础上,进一步深入实践的前提。
# 2. Java代码复杂度分析工具综述
## 2.1 静态代码分析工具简介
### 2.1.1 工具的定义和作用
静态代码分析工具是用于检测源代码中潜在错误、漏洞和代码质量相关问题的软件。这些工具能够识别程序代码中的语法规则错误、编码标准违规以及不安全的编码实践等问题,无需实际执行代码。它们通常是集成开发环境(IDE)或持续集成(CI)系统的一部分。
静态分析工具对于防止错误进入生产环境,维护代码质量和一致性具有至关重要的作用。这些工具能够帮助开发人员提前发现和解决问题,从而提高软件的可靠性,并减少后期修复问题的成本。
### 2.1.2 常见静态分析工具的对比
市场上存在多种静态代码分析工具,其中一些被广泛应用于Java开发中。以下是几种主流静态分析工具的对比:
| 工具名称 | 描述 | 特点 |
|--------------|--------------------------------------------------------------|----------------------------------------------------------|
| PMD | 高度可配置,支持多种规则集。可以集成到IDE和CI中。 | 提供了广泛的规则来检测潜在的问题,易于扩展。 |
| Checkstyle | 强调编码风格和格式化,拥有灵活的配置选项。 | 非常适合于保证代码风格的一致性。 |
| FindBugs | 专注于查找代码中的bug模式,通过字节码分析实现。 | 强调发现潜在的运行时错误。 |
| SonarQube | 不仅限于静态分析,还提供动态分析和代码质量管理平台。 | 集成了多种语言的分析工具,并提供了丰富的度量和报告功能。 |
## 2.2 动态代码分析工具简介
### 2.2.1 工具的运行原理
动态代码分析工具在程序运行时检查代码,因此能够检测到静态工具无法发现的问题,例如内存泄漏、竞态条件、死锁等。这些工具通常通过附加到运行中的应用程序来收集信息,分析程序在执行过程中的行为。
### 2.2.2 主要动态分析工具的特点
| 工具名称 | 描述 | 特点 |
|--------------|--------------------------------------------------------------|----------------------------------------------------------|
| JProfiler | 提供详细的性能分析数据,如内存使用、CPU使用和线程分析。 | 支持多种平台和JVM语言,适用于性能调优和问题诊断。 |
| VisualVM | 是一个多功能的监控和故障排除工具。 | 提供了丰富的监控视图和插件,几乎可以分析所有Java应用。 |
| Java Mission Control | 用于监控Java虚拟机性能的工具包。 | 提供了高级的JVM监控和诊断功能,适用于生产环境。 |
## 2.3 代码复杂度评估标准
### 2.3.1 时间复杂度和空间复杂度
时间复杂度和空间复杂度是衡量算法运行效率的两个主要标准。时间复杂度指的是执行算法所需的计算步骤数量,它描述了算法执行时间随输入规模增长的变化趋势。空间复杂度指的是算法执行所需的额外空间量。
### 2.3.2 复杂度评估的实用方法
复杂度评估通常通过大O表示法来描述,例如:
- O(1):常数时间复杂度,表示操作的时间不依赖于输入数据的大小。
- O(log n):对数时间复杂度,表示操作的时间随输入数据的对数线性增长。
- O(n):线性时间复杂度,表示操作的时间与输入数据大小成正比。
- O(n log n):通常出现在排序算法中,表示操作的时间与输入数据大小成对数关系。
- O(n^2):二次时间复杂度,常见于嵌套循环。
复杂度评估可以结合具体的数据结构和算法类型,评估其在不同情况下的表现,帮助我们选择或改进算法,达到优化性能的目的。
# 3. 主流Java复杂度分析工具的深度实践
### 3.1 PMD和Checkstyle工具应用
#### 3.1.1 规则集的配置和使用
PMD和Checkstyle是Java开发者中广泛使用的两个静态代码分析工具,它们有助于维持代码风格的一致性,并识别潜在的代码问题。PMD专注于找出未使用的变量、空的catch块、不必要的对象创建等潜在问题,而Checkstyle专注于代码格式和编程规范的遵守。
配置PMD的规则集通常涉及编辑`rulesets.xml`文件,该文件定义了
0
0
相关推荐
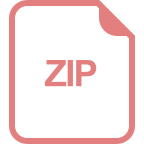
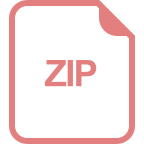
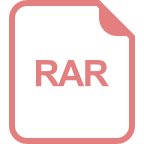
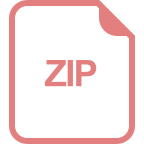
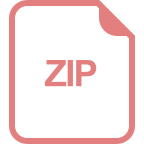