AVR单片机C程序设计与高级数据结构:链表、队列和栈的深入理解
发布时间: 2024-07-07 04:25:22 阅读量: 72 订阅数: 23 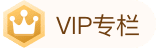
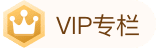
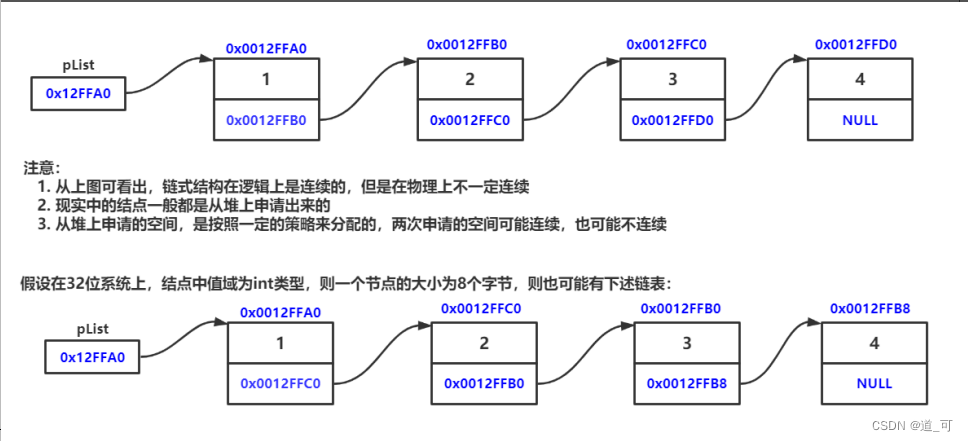
# 1. AVR单片机C程序设计基础**
AVR单片机C程序设计是嵌入式系统开发中重要的基础,它提供了对AVR单片机硬件和软件资源的低级控制。本章将介绍AVR单片机C程序设计的语法、数据类型、运算符、控制语句和函数等基本概念,为后续章节的高级数据结构和程序设计实践奠定基础。
**1.1 AVR单片机简介**
AVR单片机是一种8位RISC微控制器,具有低功耗、高性能和丰富的片上外设等特点。本章将介绍AVR单片机的架构、指令集和存储器模型,帮助读者理解AVR单片机的底层工作原理。
**1.2 C语言基础**
C语言是一种广泛应用于嵌入式系统开发的高级编程语言。本章将介绍C语言的基本语法、数据类型、运算符、控制语句和函数,为读者提供理解AVR单片机C程序设计的必要基础。
# 2. 高级数据结构理论
### 2.1 链表
#### 2.1.1 链表的概念和结构
链表是一种非连续的线性数据结构,其元素通过指针连接起来。每个链表元素称为一个节点,包含数据和指向下一个节点的指针。链表的第一个节点称为头节点,最后一个节点的指针指向空(NULL)。
**链表结构:**
```
struct Node {
int data;
struct Node *next;
};
```
#### 2.1.2 链表的插入、删除和查找
**插入:**
* 在指定位置插入:找到插入位置的前一个节点,将新节点插入到该节点之后。
* 在链表头部插入:将新节点指向头节点,并将头节点更新为新节点。
**删除:**
* 删除指定位置的节点:找到要删除节点的前一个节点,将该节点的指针指向要删除节点的下一个节点。
* 删除链表头部节点:将头节点更新为下一个节点。
**查找:**
* 顺序查找:从头节点开始,逐个节点比较,直到找到目标节点。
* 链表中使用哨兵节点(一个值为特殊标记的额外节点)可以简化查找过程。
### 2.2 队列
#### 2.2.1 队列的概念和结构
队列是一种先进先出(FIFO)的数据结构,其元素通过指针连接起来。队列的第一个元素称为队头,最后一个元素称为队尾。
**队列结构:**
```
struct Queue {
struct Node *front;
struct Node *rear;
};
```
#### 2.2.2 队列的入队、出队和判断空满
**入队:**
* 将新元素添加到队列尾部。
* 更新队列尾部指针指向新元素。
**出队:**
* 从队列头部删除元素。
* 更新队列头部指针指向下一个元素。
**判断空满:**
* 队列为空:队列头部和尾部指针都指向空(NULL)。
* 队列已满:队列尾部指针指向最后一个元素,且该元素的下一个指针指向空(NULL)。
### 2.3 栈
#### 2.3.1 栈的概念和结构
栈是一种后进先出(LIFO)的数据结构,其元素通过指针连接起来。栈的顶部称为栈顶,底部称为栈底。
**栈结构:**
```
struct Stack {
struct Node *top;
};
```
#### 2.3.2 栈的压栈、弹栈和判断空满
**压栈:**
* 将新元素添加到栈顶。
* 更新栈顶指针指向新元素。
**弹栈:**
* 从栈顶删除元素。
* 更新栈顶指针指向下一个元素。
**判断空满:**
* 栈为空:栈顶指针指向空(NULL)。
* 栈已满:栈顶指针指向最后一个元素,且该元素的下一个指针指向空(NULL)。
# 3.1 链表的实现
#### 3.1.1 链表的创建和初始化
链表的创建和初始化涉及到链表头结点的分配和初始化。链表头结点是一个特殊的节点,它不存储任何实际数据,而是指向链表中第一个实际节点。
```c
struct node {
int data;
struct node *next;
};
struct node *head = NULL;
```
在上面的代码中,`head`是一个指向链表头结点的指针,最初被初始化为`NULL`,表示链表为空。
#### 3.1.2 链表的插入、删除和查找
**插入**
在链表中插入一个新节点涉及到以下步骤:
1. 分配一个新的节点。
2. 将新节点的数据成员初始化为要插入的数据。
3. 将新节点的`next`指针指向当前链表的最后一个节点。
4. 将链表头结点的`next`指针指向新节点。
```c
void insert_node(int data) {
struct node *new_node = (struct node *)malloc(sizeof(struct node));
new_node->data = data;
new_node->next = head;
head = new_node;
}
```
**删除**
从链表中删除一个节点涉及到以下步骤:
1. 找到要删除的节点的前一个节点。
2. 将前一个节点的`next`指针指向要删除节点的下一个节点。
3. 释放要删除的节点的内存。
```c
void delete_node(int data) {
struct node *current_node = head;
struct node *previous_node
```
0
0
相关推荐
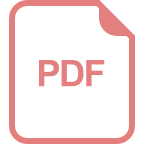
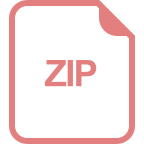
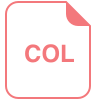
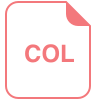
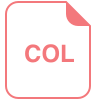
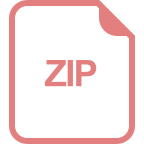
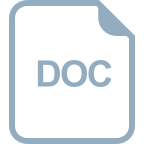
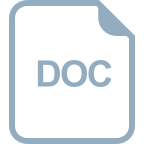