OpenCV视频转图片:从帧到图像的转换指南,揭秘幕后机制,提升效率
发布时间: 2024-08-13 18:22:52 阅读量: 11 订阅数: 12 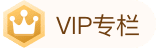
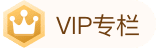
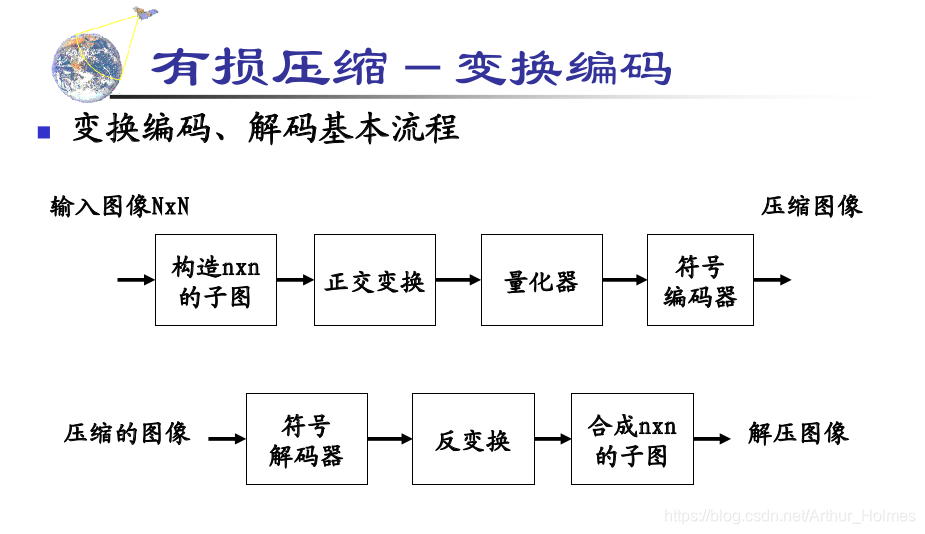
# 1. OpenCV视频转图片概述
OpenCV(Open Source Computer Vision Library)是一个开源的计算机视觉库,提供了一系列图像和视频处理算法。视频转图片是计算机视觉中一项常见的任务,涉及从视频中提取单个帧并将其保存为图像。
OpenCV提供了多种视频帧提取算法,包括基于时间间隔的提取和基于事件检测的提取。基于时间间隔的提取以固定间隔从视频中提取帧,而基于事件检测的提取则在检测到特定事件(例如运动或对象)时提取帧。
# 2. OpenCV视频帧提取理论
### 2.1 视频帧的概念和属性
视频帧是视频序列中连续的图像,它代表了视频在特定时间点的视觉内容。每个帧都是一个独立的图像,但与相邻帧一起播放时,它们会产生运动的错觉。
视频帧的属性包括:
- **帧率 (FPS):**每秒显示的帧数,决定了视频的流畅度。
- **分辨率:**帧的宽度和高度,决定了视频的清晰度。
- **颜色深度:**每像素存储的颜色位数,决定了视频的色彩范围。
- **编码格式:**用于存储和传输视频数据的格式,如 H.264、MPEG-4 等。
### 2.2 视频帧提取算法
视频帧提取算法用于从视频中提取帧。有两种主要类型的帧提取算法:
#### 2.2.1 基于时间间隔的帧提取
这种算法以固定的时间间隔从视频中提取帧。它简单易用,但可能会导致帧率不均匀,尤其是在视频中动作快速变化时。
#### 2.2.2 基于事件检测的帧提取
这种算法使用运动检测或其他事件检测技术来识别视频中感兴趣的帧。它可以提取出更相关的帧,但计算成本更高。
### 2.3 帧提取优化策略
为了提高视频帧提取的效率,可以使用以下优化策略:
#### 2.3.1 并行处理
通过使用多线程或多进程,可以并行处理帧提取任务,从而提高整体性能。
#### 2.3.2 缓存机制
通过将已提取的帧缓存起来,可以避免重复提取相同帧,从而减少计算开销。
# 3.1 视频帧提取代码实现
#### 3.1.1 视频读取和初始化
```python
import cv2
# 打开视频文件
cap = cv2.VideoCapture("video.mp4")
# 检查视频是否打开成功
if not cap.isOpened():
print("Error: Could not open video file.")
exit()
# 获取视频帧率
fps = cap.get(cv2.CAP_PROP_FPS)
# 获取视频帧宽和帧高
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# 逐帧读取视频
while True:
# 读取下一帧
ret, frame = cap.read()
# 如果读取到最后一帧,退出循环
if not ret:
break
```
#### 3.1.2 帧提取循环
在视频读取和初始化之后,进入帧提取循环。循环会不断读取视频帧,直到读取到最后一帧。
```python
# 帧计数器
frame_count = 0
# 提取间隔(以帧为单位)
interval = 10
# 提取帧
while True:
# 跳过不符合提取间隔的帧
if frame_count % interval != 0:
frame_count += 1
continue
# 提取当前帧
frame_extracted = frame
# 保存提取的帧
cv2.imwrite("frame_{}.jpg".format(frame_count), frame_extracted)
# 帧计数器加 1
frame_count += 1
# 如果读取到最后一帧,退出循环
if not ret:
break
```
#### 3.1.3 帧保存
提取的帧以 JPEG 格式保存到文件中。文件名使用 "frame_{frame_count}.jpg" 的格式,其中 {frame_count} 为帧计数器。
### 3.2 帧提取性能优化实践
#### 3.2.1 多线程并行
视频帧提取是一个耗时的过程。为了提高性能,可以使用多线程并行处理。
```python
import threading
# 创建线程池
pool = ThreadPool(4)
# 帧提取任务
def extract_frame(frame_count, frame):
# 提取帧
frame_extracted = frame
# 保存提取的帧
cv2.imwrite("frame_{}.jpg".format(frame_count), frame_extracted)
# 逐帧读取视频
while True:
# 读取下一帧
ret, frame = cap.read()
# 如果读取到最后一帧,退出循环
if not ret:
break
# 创建任务并添加到线程池
task = pool.submit(extract_frame, frame_count, frame)
# 帧计数器加 1
frame_count += 1
# 等待所有任务完成
pool.join()
```
#### 3.2.2 内存优化
在帧提取过程中,会占用大量的内存。为了优化内存使用,可以使用缓存机制。
```python
# 帧缓存
frame_cache = {}
# 帧提取循环
while True:
# 读取下一帧
ret, frame = cap.read()
# 如果读取到最后一帧,退出循环
if not ret:
break
# 帧计数器
frame_count = cap.get(cv2.CAP_PROP_POS_FRAMES)
# 检查帧是否已缓存
if frame_count in frame_cache:
# 从缓存中获取帧
frame = frame_cache[frame_count]
else:
# 提取帧
frame_extracted = frame
# 将帧添加到缓存中
frame_cache[frame_count] = frame_extracted
# 保存提取的帧
cv2.imwrite("frame_{}.jpg".format(frame_count), frame_extracted)
```
# 4. OpenCV图像转换理论
### 4.1 图像格式和数据结构
#### 4.1.1 图像格式
图像格式决定了图像数据的存储方式和组织结构。常见图像格式包括:
| 格式 | 特点 |
|---|---|
| JPEG | 有损压缩,适用于照片和自然图像 |
| PNG | 无损压缩,适用于图形和文本 |
| TIFF | 无损压缩,适用于高品质图像 |
| BMP | 无压缩,适用于简单图像 |
#### 4.1.2 图像数据结构
图像数据结构描述了图像中像素数据的组织方式。常见图像数据结构包括:
| 数据结构 | 特点 |
|---|---|
| 单通道 | 每个像素只有一个通道(灰度值) |
| 多通道 | 每个像素有多个通道(RGB、RGBA 等) |
| 位图 | 每个像素用一个或多个位表示 |
| 栅格 | 图像被划分为规则的网格,每个网格包含一个像素值 |
### 4.2 图像转换算法
图像转换算法用于将图像从一种格式或数据结构转换为另一种。常见图像转换算法包括:
#### 4.2.1 颜色空间转换
颜色空间转换算法将图像从一种颜色空间(如 RGB)转换为另一种(如 HSV)。
```python
import cv2
# 将 RGB 图像转换为 HSV 图像
hsv = cv2.cvtColor(rgb_image, cv2.COLOR_RGB2HSV)
# 参数说明:
# - rgb_image:输入 RGB 图像
# - cv2.COLOR_RGB2HSV:颜色空间转换代码
```
#### 4.2.2 尺寸缩放
尺寸缩放算法将图像调整为新的尺寸。
```python
import cv2
# 将图像缩小到一半
resized_image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
# 参数说明:
# - image:输入图像
# - (0, 0):输出图像尺寸,0 表示保持原始宽高比
# - fx、fy:缩放因子
```
#### 4.2.3 格式转换
格式转换算法将图像从一种格式转换为另一种。
```python
import cv2
# 将 PNG 图像转换为 JPEG 图像
jpeg_image = cv2.imwrite('output.jpg', png_image)
# 参数说明:
# - 'output.jpg':输出 JPEG 图像路径
# - png_image:输入 PNG 图像
```
### 4.3 图像转换优化策略
#### 4.3.1 硬件加速
硬件加速利用图形处理单元(GPU)来加速图像转换操作。
```python
import cv2
# 使用 CUDA 加速图像转换
cv2.setUseOptimized(True)
```
#### 4.3.2 算法选择
根据图像转换需求选择合适的算法可以提高效率。例如,对于图像缩放,双线性插值算法比最近邻插值算法提供更好的质量。
# 5. OpenCV图像转换实践
### 5.1 图像转换代码实现
#### 5.1.1 图像读取和初始化
图像转换的第一步是读取和初始化图像。OpenCV提供了`cv2.imread()`函数来读取图像文件。该函数接受图像文件的路径作为参数,并返回一个NumPy数组,其中包含图像数据。
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
```
#### 5.1.2 图像转换操作
图像读取后,可以使用OpenCV提供的各种函数对其进行转换。这些函数包括:
* **颜色空间转换:**将图像从一种颜色空间转换为另一种颜色空间,例如从BGR转换为RGB或HSV。
* **尺寸缩放:**调整图像的大小,使其具有不同的宽度和高度。
* **格式转换:**将图像从一种格式转换为另一种格式,例如从JPEG转换为PNG。
以下代码示例演示了如何执行这些转换:
```python
# 颜色空间转换:BGR -> RGB
rgb_image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# 尺寸缩放:将图像缩小到一半
scaled_image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
# 格式转换:JPEG -> PNG
cv2.imwrite('image.png', scaled_image)
```
#### 5.1.3 图像保存
转换后的图像可以使用`cv2.imwrite()`函数保存到文件中。该函数接受图像数据和输出文件路径作为参数。
```python
# 保存转换后的图像
cv2.imwrite('converted_image.jpg', rgb_image)
```
### 5.2 图像转换性能优化实践
图像转换操作可以是计算密集型的,尤其是在处理大图像时。以下是一些优化图像转换性能的策略:
#### 5.2.1 图像缓存
图像缓存涉及将图像数据存储在内存中,以便在需要时快速访问。这可以减少从磁盘读取图像的开销,从而提高性能。
```python
# 将图像缓存到内存中
image_cache = {}
# 从缓存中获取图像
image = image_cache.get('image.jpg')
# 如果图像不在缓存中,则从磁盘读取
if image is None:
image = cv2.imread('image.jpg')
image_cache['image.jpg'] = image
```
#### 5.2.2 懒加载
懒加载是一种技术,它只在需要时加载数据。在图像转换的情况下,这意味着仅在需要转换图像时才将其从磁盘读取。
```python
# 懒加载图像
def get_image(path):
if path not in image_cache:
image_cache[path] = cv2.imread(path)
return image_cache[path]
# 转换图像
image = get_image('image.jpg')
```
# 6.1 总结
OpenCV视频转图片技术在图像处理、视频分析、计算机视觉等领域有着广泛的应用。通过深入了解视频帧提取和图像转换的理论和实践,我们可以有效地处理视频数据,提取有价值的信息。
**视频帧提取**
* 基于时间间隔和事件检测的帧提取算法提供了灵活的选择,以满足不同的应用需求。
* 并行处理和缓存机制等优化策略可以显著提高帧提取效率。
**图像转换**
* 颜色空间转换、尺寸缩放和格式转换等图像转换操作是图像处理中的基本操作。
* 硬件加速、算法选择等优化策略可以优化图像转换性能。
**应用**
OpenCV视频转图片技术在以下应用中发挥着至关重要的作用:
* **视频监控:**从视频中提取帧以进行运动检测和目标识别。
* **视频分析:**提取关键帧以进行内容分析和摘要生成。
* **计算机视觉:**将视频分解为图像以进行对象检测、跟踪和识别。
## 6.2 展望
OpenCV视频转图片技术仍在不断发展,未来有望取得进一步的进步:
* **实时处理:**优化算法和利用硬件加速技术,实现视频帧提取和图像转换的实时处理。
* **人工智能集成:**将人工智能技术与OpenCV相结合,实现更智能、更自动化的视频处理任务。
* **云计算:**利用云计算平台的弹性和可扩展性,处理大规模视频数据。
0
0
相关推荐
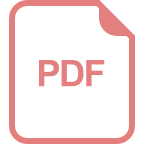
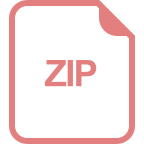
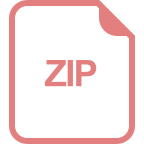





