探索AngularJS4中的指令和组件
发布时间: 2023-12-17 06:01:43 阅读量: 13 订阅数: 18 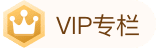
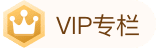
# 章节一:AngularJS4 简介
## 1.1 什么是AngularJS4
AngularJS4是一款流行的前端JavaScript框架,由Google维护和开发。它基于MVC(Model-View-Controller)架构,用于构建动态、单页面Web应用程序。
## 1.2 AngularJS4 的特性和优势
AngularJS4具有以下特性和优势:
- 双向数据绑定:实现视图和模型之间的自动同步
- 模块化开发:通过模块化的方式构建大型应用
- 依赖注入:方便模块间的解耦和组件的复用
- 指令和组件:用于创建可复用的UI组件
- 强大的HTTP支持:简化与服务器端的数据交互
- 跨平台兼容性:可以在多种平台上运行
## 章节二:AngularJS4 中的指令
在AngularJS4中,指令是一种特殊的HTML属性,用于扩展HTML标记的功能。通过指令,我们可以在HTML中添加自定义的行为和交互效果。本章将介绍AngularJS4中指令的概念、使用方法以及自定义指令的开发。
### 2.1 指令的概念和作用
指令是AngularJS4的核心概念之一,它允许我们通过自定义HTML元素、属性、类名或注释来扩展HTML的功能。指令可以用于实现各种功能,例如表单验证、数据绑定、事件处理等。通过指令,我们可以将一些通用的功能封装成可以重复利用的组件,从而提高代码的可维护性和复用性。
### 2.2 内置指令的使用方法
AngularJS4提供了一系列内置指令,可以直接在HTML中使用。常用的内置指令包括ng-app、ng-init、ng-model、ng-bind等。下面是一些常用指令的使用方法:
#### ng-app 指令
ng-app指令用于指定AngularJS应用的根元素。在HTML中使用ng-app指令后,AngularJS会自动初始化应用并进行编译和数据绑定。
```html
<!DOCTYPE html>
<html ng-app="myApp">
...
</html>
```
#### ng-model 指令
ng-model指令用于实现数据绑定,将表单元素的值与作用域中的变量进行双向绑定。
```html
<input type="text" ng-model="name">
<p>{{name}}</p>
```
#### ng-repeat 指令
ng-repeat指令用于循环渲染HTML元素,根据作用域中的数组或对象进行重复。
```html
<ul>
<li ng-repeat="item in items">{{item}}</li>
</ul>
```
### 2.3 自定义指令的开发和使用
除了使用内置指令,我们还可以开发自定义指令来实现特定的功能。通过自定义指令,我们可以封装一些通用的组件,并在HTML中以标签形式使用。
```javascript
// 定义一个自定义指令
app.directive('myDirective', function() {
return {
restrict: 'E',
template: '<div>自定义指令的内容</div>',
link: function(scope, element, attrs) {
// 指令的逻辑处理
}
};
});
```
```html
<!-- 在HTML中使用自定义指令 -->
<my-directive></my-directive>
```
自定义指令使用`directive`方法进行定义,指定指令的名称、限定符(restrict)、模板(template)和链接函数(link)。限定符指定指令可以使用的方式,常用的限定符有`E`代表元素、`A`代表属性、`C`代表类名、`M`代表注释。模板定义了指令渲染的HTML内容,链接函数用于处理指令的逻辑。
### 章节三:AngularJS4 中的组件
在AngularJS4中,组件是构建用户界面的基本单位,它封装了一段特定功能的界面元素,并负责控制其行为。在本章节中,我们将深入探讨AngularJS4中的组件相关知识,包括组件的概念和结构、组件之间的通信方式以及模块化思维下的组件设计。让我们一起来了解和掌握AngularJS4中的组件使用。
#### 3.1 组件的概念和结构
在AngularJS4中,组件是通过@Component装饰器来定义的。一个典型的组件由组件类、模板和元数据组成。
以下是一个简单的组件示例:
```typescript
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<h1>Hello, {{name}}!</h1>
`
})
export class AppComponent {
name = 'AngularJS4';
}
```
在上面的示例中,@Component装饰器用于定义一个组件,其中包括selector(组件的选择器)、template(组件的模板)等元数据。AppComponent类是组件的类定义,包括组件的行为逻辑和数据。
#### 3.2 组件之间的通信方式
在AngularJS4中,组件之间可以通过输入属性和输出属性进行通信。
- 输入属性:通过@Input装饰器可以将数据从父组件传递到子组件。
```typescript
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<p>{{message}}</h1>
`
})
export class ChildComponent {
@Input() message: string;
}
```
父组件中使用子组件时可以这样传递数据:
```html
<app-child [message]="'Hello from Parent'"></app-child>
```
- 输出属性:通过@Output装饰器和EventEmitter可以将数据从子组件传递到父组件。
```typescript
import { Component, EventEmitter, Output } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<button (click)="sendMessage()">Send Message</button>
`
})
export class ChildComponent {
@Output() messageSent = new EventEmitter<string>();
sendMessage() {
this.messageSent.emit('Hello from Child');
}
}
```
父组件中使用子组件时可以这样接收数据:
```html
<app-child (messageSent)="receiveMessage($event)"></app-child>
```
#### 3.3 模块化思维下的组件设计
在AngularJS4中,我们可以通过模块化的方式设计和组织组件,使得应用的结构更加清晰和易于维护。
比如可以将相关联的组件放在同一个模块下:
```typescript
// product.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { ProductListComponent } from './product-list.component';
import { ProductDetailComponent } from './product-detail.component';
@NgModule({
declarations: [ProductListComponent, ProductDetailComponent],
imports: [CommonModule]
})
export class ProductModule {}
```
在上面的示例中,ProductListComponent和ProductDetailComponent被放在了同一个ProductModule模块下,便于统一管理和导入。
## 章节四:AngularJS4 中常用的指令和组件
在 AngularJS4 中,指令和组件是非常常用的功能模块,能够帮助开发者快速构建丰富的交互界面和功能。本章将介绍 AngularJS4 中常用的指令和组件,包括表单相关的指令和组件、路由指令和组件,以及 HTTP 请求和响应指令和组件的详细内容。
### 4.1 表单相关的指令和组件
表单是 Web 应用中常见的交互界面,AngularJS4 提供了丰富的表单相关指令和组件,帮助开发者轻松实现输入验证、数据绑定等功能。常见的表单指令包括 `ngForm`、`ngModel`、`ngSubmit` 等,而常见的表单组件包括 `input`、`select`、`textarea` 等。
以下是一个简单的示例,演示了如何使用 AngularJS4 中的表单相关指令和组件:
```javascript
import { Component } from '@angular/core';
@Component({
selector: 'app-form-example',
template: `
<form #myForm="ngForm" (ngSubmit)="onSubmit(myForm)">
<div>
<label for="name">Name:</label>
<input type="text" id="name" name="name" ngModel>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" ngModel>
</div>
<button type="submit">Submit</button>
</form>
`
})
export class FormExampleComponent {
onSubmit(form: NgForm) {
if (form.valid) {
// 处理表单提交逻辑
}
}
}
```
在上述示例中,`ngForm` 指令用于包裹整个表单,而 `ngModel` 指令用于实现数据双向绑定。当用户提交表单时,会触发 `ngSubmit` 事件,调用 `onSubmit` 方法进行表单验证和提交逻辑处理。
### 4.2 路由指令和组件
在 AngularJS4 中,路由是实现单页面应用 (SPA) 的重要组成部分,可以帮助开发者实现页面间的切换和导航。AngularJS4 提供了丰富的路由指令和组件,包括 `routerLink`、`router-outlet`、`RouterModule` 等,开发者可以通过这些指令和组件进行路由配置和实现页面跳转。
以下是一个简单的示例,演示了如何使用 AngularJS4 中的路由指令和组件:
```javascript
import { Component } from '@angular/core';
@Component({
selector: 'app-router-example',
template: `
<a routerLink="/home">Home</a>
<a routerLink="/about">About</a>
<router-outlet></router-outlet>
`
})
export class RouterExampleComponent {
// 路由配置等逻辑
}
```
在上述示例中,通过 `routerLink` 指令可以实现页面间的跳转,而 `router-outlet` 组件则用于显示当前路由对应的组件内容。
### 4.3 HTTP 请求和响应指令和组件
在实际的 Web 应用开发中,经常需要和后端服务器进行数据交互,AngularJS4 提供了丰富的 HTTP 请求和响应指令和组件,帮助开发者发送 HTTP 请求、处理响应并展示数据。
以下是一个简单的示例,演示了如何使用 AngularJS4 中的 HTTP 请求和响应指令和组件:
```javascript
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-http-example',
template: `
<button (click)="getData()">Get Data</button>
<div>{{ responseData }}</div>
`
})
export class HttpExampleComponent {
responseData: any;
constructor(private http: HttpClient) {}
getData() {
this.http.get('https://api.example.com/data').subscribe((data) => {
this.responseData = data;
});
}
}
```
在上述示例中,通过 `HttpClient` 进行 HTTP 请求,并利用 `subscribe` 方法处理响应数据并展示在页面上。
### 章节五:AngularJS4 中的指令和组件实践
在本章中,我们将通过实例演示如何在AngularJS4中使用指令和组件来实践具体的功能和场景。我们将从构建一个简单的 TodoList 应用开始,然后探讨如何利用指令和组件实现数据可视化,最后使用指令和组件进行表单验证和数据绑定。
#### 5.1 构建一个简单的 TodoList 应用
首先我们来构建一个简单的 TodoList 应用,具体步骤如下:
1. 创建一个新的 AngularJS4 项目,可以使用 Angular CLI 进行快速搭建。
2. 在应用目录中创建一个新的组件,命名为 `todo-list`,并在组件的模板中展示一个输入框和一个按钮,用于添加新的 todo。
```typescript
// todo-list.component.html
<input type="text" [(ngModel)]="newTodo" placeholder="请输入新的 todo">
<button (click)="addTodo()">添加</button>
```
3. 在组件的 TypeScript 文件中,定义相关的数据和方法。
```typescript
// todo-list.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-todo-list',
templateUrl: './todo-list.component.html',
styleUrls: ['./todo-list.component.css']
})
export class TodoListComponent {
todos: string[] = [];
newTodo: string;
addTodo() {
this.todos.push(this.newTodo);
this.newTodo = '';
}
}
```
4. 在应用的根组件模板中使用 `todo-list` 组件。
```html
<!-- app.component.html -->
<h1>TodoList 应用</h1>
<app-todo-list></app-todo-list>
```
通过以上步骤,我们已经成功构建了一个简单的 TodoList 应用。用户可以在输入框中输入新的 todo,点击按钮后,新的 todo 会被添加到列表中,如下图所示:
#### 5.2 利用指令和组件实现数据可视化
在实际项目中,我们常常需要将数据可视化展示给用户,比如通过图表展示数据统计结果。在AngularJS4中,我们可以利用指令和组件来实现这样的功能。
首先,我们需要选择一个合适的图表库,比如 `Chart.js`。然后,按照该图表库的使用说明,安装并引入相关的依赖。
接下来,我们创建一个新的指令,命名为 `chart`,并在指令的代码中使用 `Chart.js` 绘制图表。
```typescript
// chart.directive.ts
import { Directive, ElementRef, Input, OnInit } from '@angular/core';
import * as Chart from 'chart.js';
@Directive({
selector: '[appChart]'
})
export class ChartDirective implements OnInit {
@Input('appChart') data: any;
@Input() options: any;
private chart: any;
constructor(private el: ElementRef) {}
ngOnInit() {
const ctx = this.el.nativeElement.getContext('2d');
this.chart = new Chart(ctx, {
type: 'bar',
data: this.data,
options: this.options
});
}
}
```
在使用该指令的组件中,我们可以通过输入属性传入需要展示的数据和图表配置。
```html
<!-- chart.component.html -->
<canvas appChart [data]="data" [options]="options"></canvas>
```
```typescript
// chart.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-chart',
templateUrl: './chart.component.html',
styleUrls: ['./chart.component.css']
})
export class ChartComponent {
data = {
labels: ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange'],
datasets: [{
label: '# of Votes',
data: [12, 19, 3, 5, 2, 3],
backgroundColor: [
'rgba(255, 99, 132, 0.2)',
'rgba(54, 162, 235, 0.2)',
'rgba(255, 206, 86, 0.2)',
'rgba(75, 192, 192, 0.2)',
'rgba(153, 102, 255, 0.2)',
'rgba(255, 159, 64, 0.2)'
],
borderColor: [
'rgba(255, 99, 132, 1)',
'rgba(54, 162, 235, 1)',
'rgba(255, 206, 86, 1)',
'rgba(75, 192, 192, 1)',
'rgba(153, 102, 255, 1)',
'rgba(255, 159, 64, 1)'
],
borderWidth: 1
}]
};
options = {
scales: {
y: {
beginAtZero: true
}
}
};
}
```
通过以上步骤,我们已经成功利用指令和组件实现了数据可视化的功能。用户可以在组件中看到一个柱状图,展示了相应数据的统计结果。
#### 5.3 使用指令和组件进行表单验证和数据绑定
在表单处理方面,AngularJS4提供了丰富的指令和组件,可以帮助我们实现数据绑定和表单验证。
首先,我们来展示如何实现数据双向绑定和表单验证。我们创建一个新的组件,命名为 `user-profile`,并在组件的模板中展示一个表单,包含用户名和密码两个输入框。
```html
<!-- user-profile.component.html -->
<form>
<label for="username">用户名:</label>
<input id="username" type="text" [(ngModel)]="username" required>
<div *ngIf="username.touched && username.errors?.required" class="error">用户名不能为空</div>
<label for="password">密码:</label>
<input id="password" type="password" [(ngModel)]="password" required>
<div *ngIf="password.touched && password.errors?.required" class="error">密码不能为空</div>
</form>
```
在组件的 TypeScript 文件中,我们需要定义相关的数据和方法。
```typescript
// user-profile.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-user-profile',
templateUrl: './user-profile.component.html',
styleUrls: ['./user-profile.component.css']
})
export class UserProfileComponent {
username: string;
password: string;
}
```
通过以上步骤,我们已经成功实现了数据双向绑定和基本的表单验证。用户在输入框中输入用户名和密码后,如果输入框为空,会展示相应的错误信息。
除了数据绑定和表单验证,AngularJS4还提供了更多复杂的表单处理功能,比如动态表单的生成和校验、自定义表单控件等。在具体的项目中,我们可以根据需求选择和使用相应的指令和组件。
# 章节六:AngularJS4 中指令和组件的优化和最佳实践
在使用 AngularJS4 中的指令和组件时,我们需要注意一些优化和最佳实践的原则,以提高应用的性能和开发效率。本章将介绍一些常用的优化技巧、组件和指令的复用方法以及最佳实践和规范。
## 6.1 性能优化技巧
在开发 AngularJS4 应用时,我们可以采取一些性能优化技巧来提高应用的加载速度和运行效率。下面列举了一些常用的性能优化技巧:
1. 使用 AOT 编译:Ahead of Time (AOT) 编译可以将 Angular 应用的代码提前编译成优化的 JavaScript 代码,以减少加载时间。
2. 懒加载模块:将应用模块按需加载,只有在需要时才进行加载,可以减少初始加载时间。
3. 使用 ChangeDetectionStrategy.OnPush 策略:通过设置组件的变化检测策略为 OnPush,可以减少变化检测的频率,提高性能。
4. 使用 TrackBy 函数:在使用 ngFor 指令循环渲染列表时,使用 TrackBy 函数来提供稳定的唯一标识符,减少不必要的 DOM 更新。
5. 避免过多的绑定表达式:减少双向绑定和复杂的绑定表达式可以提高应用的性能。
6. 使用 Pure Pipes:在自定义管道时,可以设置纯函数的 Pure 属性,只有在输入变化时才进行计算。
## 6.2 组件和指令的复用
在开发 AngularJS4 应用时,合理利用组件和指令的复用可以提高代码的可维护性和开发效率。
1. 组件的复用:将常用的 UI 元素或功能封装成组件,通过输入属性和输出事件来定制组件的行为和样式。
2. 指令的复用:将常用的 DOM 操作封装成指令,通过在 HTML 元素上应用指令来实现特定的功能。
3. 使用模块化思维:将组件和指令按照功能模块化的方式进行组织,可以提高代码的可维护性和复用性。
4. 使用 Angular Material 等第三方组件库:可以使用成熟的第三方组件库来快速构建应用界面,并提供一致的用户体验。
## 6.3 最佳实践和规范
在开发 AngularJS4 应用时,遵循一些最佳实践和规范可以提高代码的可读性和可维护性。
1. 遵循 Angular 风格指南:Angular 官方提供了一套规范和最佳实践,包括命名规范、文件组织结构、代码风格等。
2. 使用 TypeScript:使用 TypeScript 来开发 AngularJS4 应用,可以提供更强的类型检查和代码提示。
3. 使用 Angular CLI:使用 Angular CLI 工具来快速创建项目、生成组件和指令等,可以提高开发效率。
4. 使用单向数据流:避免在组件之间直接共享状态,使用单向数据流的方式来传递和管理数据。
0
0
相关推荐
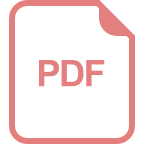





