使用Spring Boot快速搭建JavaWeb应用
发布时间: 2023-12-08 14:12:12 阅读量: 46 订阅数: 22 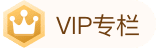
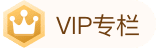
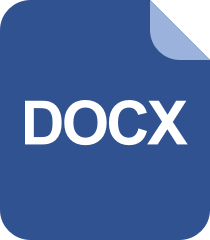
使用 Spring Boot 快速构建 Spring 框架应用
## 1. Introduction to Spring Boot
### 1.1 What is Spring Boot
Spring Boot is a popular Java-based framework for building standalone, production-grade Spring-based applications. It aims to simplify the development process and provide a faster way to set up, configure, and run Java applications. Spring Boot eliminates the need for a lot of boilerplate code and configuration, allowing developers to quickly create and deploy applications.
### 1.2 Why use Spring Boot for JavaWeb development
Spring Boot is well-suited for JavaWeb development due to its ability to streamline the setup and development process. It offers a convention-over-configuration approach, which reduces the need for manual configuration and boilerplate code. Additionally, Spring Boot provides built-in support for embedding servlet containers, making it easy to package and deploy web applications.
### 1.3 Advantages of using Spring Boot
- Simplified configuration and setup
- Built-in support for embedding servlet containers
- Elimination of boilerplate code
- Seamless integration with other Spring projects
## 2. Getting started with Spring Boot
### 2.1 Installing Spring Boot
To install Spring Boot, you can use either Maven or Gradle build tools. For Maven, you need to add the Spring Boot starter parent to your project's pom.xml file. For Gradle, you can include the Spring Boot plugin in your build.gradle file. Additionally, you can download Spring Boot CLI for command-line interface-based development.
### 2.2 Setting up the development environment
You can use any Java IDE such as IntelliJ IDEA, Eclipse, or NetBeans for Spring Boot development. These IDEs provide support for Spring Boot application development and make it easy to manage dependencies, run/debug applications, and package them for deployment.
### 2.3 Creating a new Spring Boot project
## 3. Building a JavaWeb application with Spring Boot
In this chapter, we will learn how to build a JavaWeb application using Spring Boot. We will cover the basic structure of a Spring Boot application, configuration options, creating controllers, and testing the application.
### 3.1 Understanding the structure of a Spring Boot application
A Spring Boot application follows a specific directory structure that helps organize the code in a modular way. The main components of a Spring Boot application are as follows:
- **src/main/java:** This directory contains the Java source code for the application.
- **src/main/resources:** This directory contains the application configuration files and static resources.
- **src/test/java:** This directory contains the test classes for the application.
- **src/test/resources:** This directory contains the configuration and resources required for testing.
### 3.2 Configuring the application properties
Spring Boot allows us to configure the application properties easily using the application.properties or application.yml file. These files are typically located in the src/main/resources directory. We can define various properties such as server port, database connection details, logging configuration, etc. in these files.
Here is an example of configuring the server port in the application.properties file:
```properties
# Configure the server port
server.port=8080
```
### 3.3 Creating controllers and configuring routes
Controllers handle the incoming HTTP requests and define the routes for the application. Spring Boot provides annotations such as @RestController and @RequestMapping to easily create controllers and configure routes.
Here is an example of creating a simple controller:
```java
@RestController
@RequestMapping("/api")
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, world!";
}
}
```
In this example, the controller is mapped to the "/api" path, and the "/hello" route returns the string "Hello, world!".
### 3.4 Building and testing the application
To build the Spring Boot application, we can use Maven or Gradle. These build tools provide commands to compile the source code, package the application, and run tests.
To run the application, we can use the `mvn spring-boot:run` command if we are using Maven. For Gradle, we can use the `./gradlew bootRun` command.
To test the application, we can use the Spring Boot testing framework and popular testing libraries such as JUnit and Mockito. We can write unit tests for the controllers, services, and repositories to ensure the application behaves as expected.
Here is an example of a simple unit test for the controller we defined earlier:
```java
@RunWith(SpringRunner.class)
@WebMvcTest(HelloController.class)
public class HelloControllerTests {
@Autowired
private MockMvc mockMvc;
@Test
public void testHello() throws Exception {
m
```
0
0
相关推荐






