实现Spring Boot中的文件上传与下载
发布时间: 2024-05-01 15:12:03 阅读量: 4 订阅数: 11 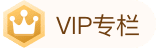
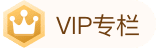
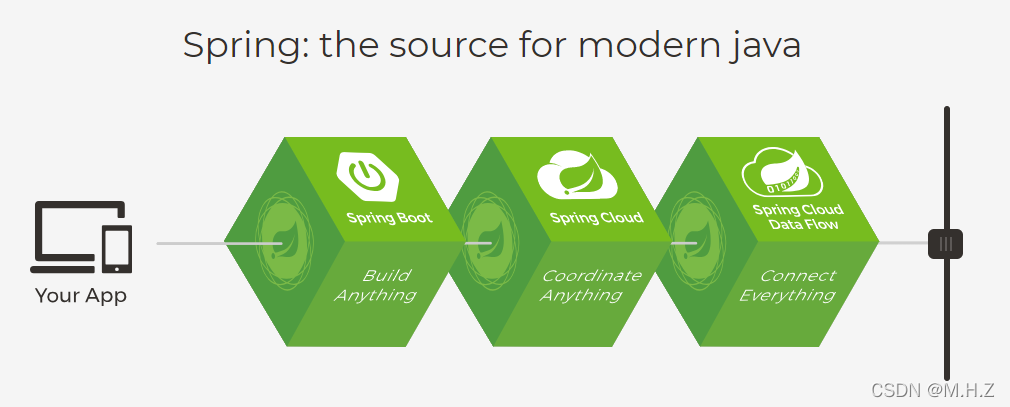
# 1. Spring Boot文件上传与下载概述**
文件上传和下载是Web应用程序中常见的操作。Spring Boot是一个流行的Java框架,它提供了对文件上传和下载的强大支持。本章将概述Spring Boot文件上传和下载功能,并介绍其基本原理和配置。
# 2. 文件上传
### 2.1 文件上传原理
#### 2.1.1 HTTP文件上传协议
HTTP文件上传协议是客户端与服务器之间传输文件的规范。它使用`multipart/form-data`作为请求的`Content-Type`,将文件内容和表单数据一起发送给服务器。
**请求报文格式:**
```
POST /upload HTTP/1.1
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Length: 1048576
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="file"; filename="test.txt"
Content-Type: text/plain
This is a test file.
------WebKitFormBoundary7MA4YWxkTrZu0gW--
```
#### 2.1.2 Spring Boot文件上传处理流程
Spring Boot通过`MultipartResolver`组件处理文件上传请求,其处理流程如下:
1. 客户端发送文件上传请求。
2. Spring Boot使用`MultipartResolver`解析请求,将文件内容和表单数据分离。
3. Spring Boot将文件内容保存到指定路径。
4. Spring Boot返回文件上传结果。
### 2.2 Spring Boot文件上传配置
#### 2.2.1 文件上传路径配置
通过`spring.servlet.multipart.location`属性配置文件上传路径,默认为`./uploads`。
```
spring.servlet.multipart.location=/tmp/uploads
```
#### 2.2.2 文件大小限制配置
通过`spring.servlet.multipart.max-file-size`和`spring.servlet.multipart.max-request-size`属性配置文件大小限制,单位为字节。
```
# 单个文件最大大小
spring.servlet.multipart.max-file-size=10MB
# 请求中所有文件总大小
spring.servlet.multipart.max-request-size=100MB
```
### 2.3 文件上传实践
#### 2.3.1 单文件上传
**请求报文:**
```
POST /upload HTTP/1.1
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Length: 1048576
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="file"; filename="test.txt"
Content-Type: text/plain
This is a test file.
------WebKitFormBoundary7MA4YWxkTrZu0gW--
```
**Controller代码:**
```java
@PostMapping("/upload")
public ResponseEntity<String> uploadFile(@RequestParam("file") MultipartFile file) {
// 保存文件
String path = saveFile(file);
// 返回上传结果
return ResponseEntity.ok("File uploaded successfully: " + path);
}
```
#### 2.3.2 多文件上传
**请求报文:**
```
POST /upload HTTP/1.1
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Length: 1048576
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="files"; filename="test1.txt"
Content-Type: text/plain
This is a test file 1.
------WebKitFormBoundary7MA4YWxkTrZu0gW
Content-Disposition: form-data; name="files"; filename="test2.txt"
Content-Type: text/plain
This is a test file 2.
------WebKitFormBoundary7MA4YWxkTrZu0gW--
```
**Controller代码:**
```java
@PostMapping("/upload")
public ResponseEntity<String> uploadFiles(@RequestParam("files") MultipartFile[] files) {
// 保存文件
List<String> paths = new ArrayList<>();
for (MultipartFile file : files) {
paths.add(saveFile(file));
}
// 返回上传结果
return ResponseEntity.ok("Files uploaded successfully: " + paths);
}
```
# 3. 文件下载
### 3.1 文件下载原理
#### 3.1.1 HTTP文件下载协议
文件下载是通过HTTP协议进行的,主要涉及以下几个HTTP请求头:
* `Content-Type`: 指定文件的MIME类型,如`application/octet-stream`。
* `Content-Disposition`: 指定文件的下载方式,如`attachment; filename=filename.ext`。
* `Content-Length`: 指定文件的长度,以字节为单位。
#### 3.1.2 Spring Boot文件下载处理流程
Spring Boot的文件下载处理流程如下:
1. 客户端发送一个HTTP GET请求,请求下载指定文件。
2. Spring Boot控制器接收请求,并根据请求路径获取要下载的文件。
3. Spring Boot使用`ResponseEntity`对象设置文件下载的HTTP响应头,包括`Content-Type`、`Content-Disposition`和`Content-Length`。
4. Spring Boot将文件内容写入HTTP响应体,并发送给客户端。
5. 客户端接收文件内容,并将其保存到本地。
### 3.2 Spring Boot文件下载配置
#### 3.2.1 文件下载路径配置
通过`spring.servlet.multipart.location`属性可以配置文件下载的临时路径,默认情况下,文件将下载到系统临时目录。
```properties
# 配置文件
```
0
0
相关推荐
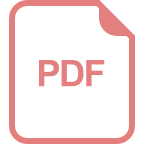
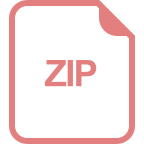
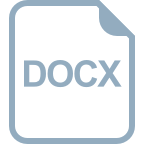





