深入剖析单片机数据类型与运算:数据表示和处理的奥秘
发布时间: 2024-07-08 23:47:57 阅读量: 95 订阅数: 36 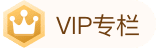
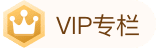
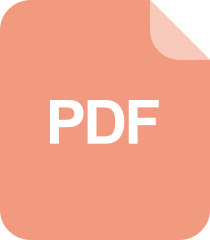
C51单片机功能模块和Keil C数据类型
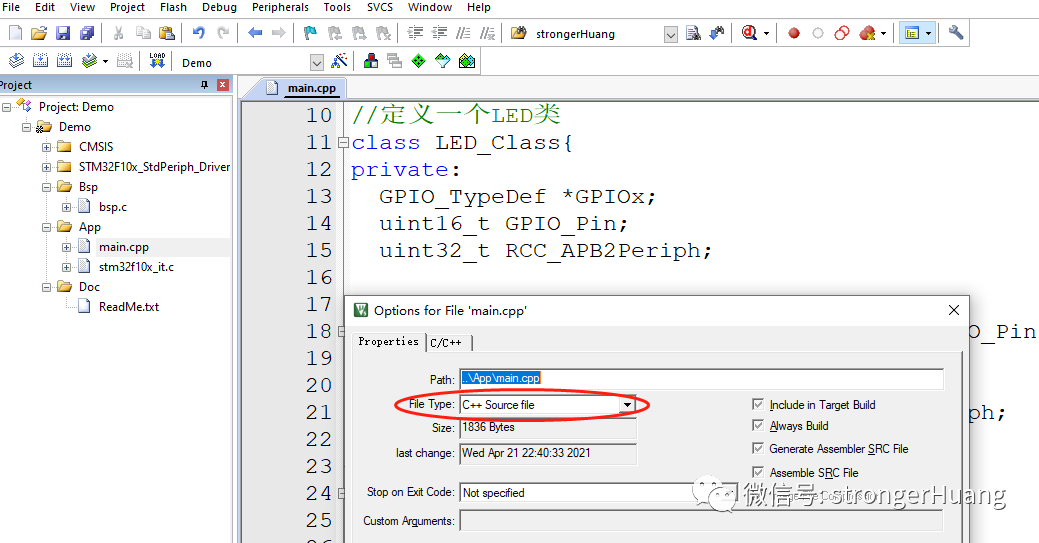
# 1. 单片机数据类型概述**
单片机数据类型定义了数据在内存中的表示方式和处理规则。它们决定了数据的大小、范围和精度,并影响运算和存储效率。单片机常用的数据类型包括:
* 整数类型:有符号整数(可以表示负值)和无符号整数(只能表示正值)
* 浮点类型:单精度浮点数(精度较低)和双精度浮点数(精度较高)
# 2. 数据表示与存储
### 2.1 整数数据类型
#### 2.1.1 有符号整数
有符号整数使用二进制补码表示,其中最高位(MSB)表示符号位,0 表示正数,1 表示负数。其余位表示整数的绝对值。
**代码块:**
```c
int8_t signed_integer = -127;
```
**逻辑分析:**
* `signed_integer` 是一个 8 位有符号整数变量。
* `-127` 的二进制补码表示为 `10000001`。
* 最高位为 1,表示负数。
* 剩余 7 位表示绝对值 127。
#### 2.1.2 无符号整数
无符号整数不使用符号位,所有位都用于表示非负整数。
**代码块:**
```c
uint8_t unsigned_integer = 255;
```
**逻辑分析:**
* `unsigned_integer` 是一个 8 位无符号整数变量。
* `255` 的二进制表示为 `11111111`。
* 由于没有符号位,所有位都表示非负整数。
### 2.2 浮点数据类型
浮点数据类型用于表示实数,使用科学计数法表示。
#### 2.2.1 单精度浮点数
单精度浮点数使用 32 位表示,其中:
* 1 位符号位
* 8 位指数位
* 23 位尾数位
**代码块:**
```c
float single_precision_float = 3.14159265;
```
**逻辑分析:**
* `single_precision_float` 是一个 32 位单精度浮点数变量。
* `3.14159265` 的二进制表示为 `01000000010010010000111111011010`。
* 符号位为 0,表示正数。
* 指数位为 `01000000`,表示指数为 127。
* 尾数位为 `10010010000111111011010`。
#### 2.2.2 双精度浮点数
双精度浮点数使用 64 位表示,其中:
* 1 位符号位
* 11 位指数位
* 52 位尾数位
**代码块:**
```c
double double_precision_float = 1.234567890123456789;
```
**逻辑分析:**
* `double_precision_float` 是一个 64 位双精度浮点数变量。
* `1.234567890123456789` 的二进制表示为 `0100000000000000000000000000000000000000000000000000000000000000`。
* 符号位为 0,表示正数。
* 指数位为 `01000000000`,表示指数为 1023。
* 尾数位为 `0000000000000000000000000000000000000000000000000000000000000000`。
# 3. 运算符与表达式
### 3.1 算术运算符
算术运算符用于执行算术运算,包括加法、减法、乘法、除法、取模、自增和自减。
#### 3.1.1 加法、减法、乘法、除法
| 运算符 | 描述 |
|---|---|
| + | 加法 |
| - | 减法 |
| * | 乘法 |
| / | 除法 |
**代码块:**
```c
int a = 10;
int b = 5;
int sum = a + b; // sum = 15
int difference = a - b; // difference = 5
int product = a * b; // product = 50
int quotient = a / b; // quotient = 2
```
**逻辑分析:**
* `a + b` 计算 `a` 和 `b` 的和,结果存储在 `sum` 中。
* `a - b` 计算 `a` 和 `b` 的差,结果存储在 `difference` 中。
* `a * b` 计算 `a` 和 `b` 的积,结果存储在 `product` 中。
* `a / b` 计算 `a` 除以 `b` 的商,结果存储在 `quotient` 中。
#### 3.1.2 取模、自增、自减
| 运算符 | 描述 |
|---|---|
| % | 取模 |
| ++ | 自增 |
| -- | 自减 |
**代码块:**
```c
int a = 10;
int b = 5;
int remainder = a % b; // remainder = 0
int incremented = ++a; // incremented = 11
int decremented = --b; // decremented = 4
```
**逻辑分析:**
* `a % b` 计算 `a` 除以 `b` 的余数,结果存储在 `remainder` 中。
* `++a` 将 `a` 的值加 1,结果存储在 `incremented` 中。
* `--b` 将 `b` 的值减 1,结果存储在 `decremented` 中。
### 3.2 逻辑运算符
逻辑运算符用于执行逻辑运算,包括与运算、或运算、异或运算和非运算。
#### 3.2.1 与运算、或运算、异或运算
| 运算符 | 描述 |
|---|---|
| & | 与运算 |
| | | 或运算 |
| ^ | 异或运算 |
**代码块:**
```c
bool a = true;
bool b = false;
bool andResult = a & b; // andResult = false
bool orResult = a | b; // orResult = true
bool xorResult = a ^ b; // xorResult = true
```
**逻辑分析:**
* `a & b` 计算 `a` 和 `b` 的与运算,结果为 `false`,因为两个操作数都为 `false`。
* `a | b` 计算 `a` 和 `b` 的或运算,结果为 `true`,因为至少一个操作数为 `true`。
* `a ^ b` 计算 `a` 和 `b` 的异或运算,结果为 `true`,因为两个操作数不同。
#### 3.2.2 非运算
| 运算符 | 描述 |
|---|---|
| ! | 非运算 |
**代码块:**
```c
bool a = true;
bool notA = !a; // notA = false
```
**逻辑分析:**
* `!a` 计算 `a` 的非运算,结果为 `false`,因为 `a` 为 `true`。
### 3.3 关系运算符
关系运算符用于比较两个值,并返回一个布尔值(`true` 或 `false`)。
#### 3.3.1 等于、不等于、大于、小于
| 运算符 | 描述 |
|---|---|
| == | 等于 |
| != | 不等于 |
| > | 大于 |
| < | 小于 |
**代码块:**
```c
int a = 10;
int b = 5;
bool isEqual = a == b; // isEqual = false
bool isNotEqual = a != b; // isNotEqual = true
bool isGreaterThan = a > b; // isGreaterThan = true
bool isLessThan = a < b; // isLessThan = false
```
**逻辑分析:**
* `a == b` 比较 `a` 和 `b` 是否相等,结果为 `false`。
* `a != b` 比较 `a` 和 `b` 是否不相等,结果为 `true`。
* `a > b` 比较 `a` 是否大于 `b`,结果为 `true`。
* `a < b` 比较 `a` 是否小于 `b`,结果为 `false`。
#### 3.3.2 大于等于、小于等于
| 运算符 | 描述 |
|---|---|
| >= | 大于等于 |
| <= | 小于等于 |
**代码块:**
```c
int a = 10;
int b = 5;
bool isGreaterThanOrEqual = a >= b; // isGreaterThanOrEqual = true
bool isLessThanOrEqual = a <= b; // isLessThanOrEqual = false
```
**逻辑分析:**
* `a >= b` 比较 `a` 是否大于或等于 `b`,结果为 `true`。
* `a <= b` 比较 `a` 是否小于或等于 `b`,结果为 `false`。
# 4. 数据转换与类型转换
在单片机编程中,数据转换和类型转换是数据处理中的重要环节。它们允许在不同数据类型之间进行转换,以满足程序的不同需求。本章将深入剖析数据转换和类型转换的机制,并介绍相关的函数和操作。
### 4.1 数据类型转换
数据类型转换是指将一种数据类型转换为另一种数据类型。单片机支持多种数据类型转换,包括整数类型之间的转换、浮点类型之间的转换以及整数与浮点之间的转换。
#### 4.1.1 整数类型之间的转换
整数类型之间的转换可以通过强制类型转换运算符 `(type)` 来实现。例如,将 8 位有符号整数 `int8_t` 转换为 16 位无符号整数 `uint16_t` 可以使用以下代码:
```c
uint16_t value = (uint16_t)int8_value;
```
需要注意的是,在进行整数类型转换时,可能会发生数据溢出或截断。例如,将一个 16 位有符号整数转换为 8 位有符号整数时,如果值超出 8 位有符号整数的范围,则会发生截断,导致数据丢失。
#### 4.1.2 浮点类型之间的转换
浮点类型之间的转换也使用强制类型转换运算符 `(type)`。例如,将单精度浮点数 `float` 转换为双精度浮点数 `double` 可以使用以下代码:
```c
double value = (double)float_value;
```
与整数类型转换类似,浮点类型转换也可能发生精度损失。例如,将一个双精度浮点数转换为单精度浮点数时,由于单精度浮点数的精度较低,可能会导致精度损失。
#### 4.1.3 整数与浮点之间的转换
整数与浮点之间的转换需要使用特定的转换函数。例如,将 16 位有符号整数 `int16_t` 转换为单精度浮点数 `float` 可以使用以下代码:
```c
float value = (float)int16_value;
```
同样,将单精度浮点数 `float` 转换为 16 位有符号整数 `int16_t` 可以使用以下代码:
```c
int16_t value = (int16_t)float_value;
```
在进行整数与浮点之间的转换时,需要注意数据范围和精度。例如,将一个浮点数转换为整数时,可能会发生舍入或截断,导致数据精度损失。
### 4.2 类型转换函数
除了强制类型转换运算符之外,单片机还提供了专门的类型转换函数。这些函数可以提供更灵活和安全的类型转换操作。
#### 4.2.1 整数类型转换函数
整数类型转换函数可以将一种整数类型转换为另一种整数类型。例如,`atoi()` 函数可以将字符串转换为整数,`itoa()` 函数可以将整数转换为字符串。
```c
int value = atoi("123");
char buffer[10];
itoa(value, buffer, 10);
```
#### 4.2.2 浮点类型转换函数
浮点类型转换函数可以将一种浮点类型转换为另一种浮点类型。例如,`atof()` 函数可以将字符串转换为浮点数,`ftoa()` 函数可以将浮点数转换为字符串。
```c
float value = atof("123.45");
char buffer[10];
ftoa(value, buffer, 10);
```
类型转换函数提供了更丰富的功能,可以满足不同场景下的类型转换需求。
# 5. 数据处理与优化**
**5.1 数据排序算法**
数据排序是将一组数据按照特定顺序排列的过程。在单片机系统中,常见的排序算法包括冒泡排序和快速排序。
**5.1.1 冒泡排序**
冒泡排序是一种简单易懂的排序算法。其原理是逐个比较相邻元素,如果顺序不正确,则交换这两个元素。重复这一过程,直到所有元素都按顺序排列。
```c
void bubbleSort(int arr[], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
```
**代码逻辑分析:**
* 外层循环 `i` 表示当前比较的元素下标。
* 内层循环 `j` 表示与当前元素比较的元素下标。
* 如果 `arr[j]` 大于 `arr[j + 1]`,则交换这两个元素。
**5.1.2 快速排序**
快速排序是一种高效的排序算法。其原理是选取一个基准元素,将数组划分为两部分:比基准元素小的元素和比基准元素大的元素。然后递归地对这两部分进行排序。
```c
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pivot = partition(arr, low, high);
quickSort(arr, low, pivot - 1);
quickSort(arr, pivot + 1, high);
}
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return (i + 1);
}
```
**代码逻辑分析:**
* `partition` 函数选取数组最后一个元素作为基准元素,将数组划分为两部分。
* `quickSort` 函数递归地对这两部分进行排序。
**5.2 数据结构**
数据结构是组织和存储数据的形式。在单片机系统中,常用的数据结构包括数组和链表。
**5.2.1 数组**
数组是一种线性数据结构,它存储一系列具有相同数据类型的元素。每个元素都有一个唯一的索引,可以通过索引访问。
```c
int arr[10];
```
**5.2.2 链表**
链表是一种非线性数据结构,它由一系列节点组成。每个节点包含数据和指向下一个节点的指针。
```c
struct Node {
int data;
struct Node *next;
};
```
**5.3 存储优化技术**
存储优化技术可以提高单片机系统的存储效率。常用的技术包括内存管理和缓存机制。
**5.3.1 内存管理**
内存管理是管理单片机系统内存资源的过程。它包括内存分配、回收和保护。
**5.3.2 缓存机制**
缓存机制是一种将经常访问的数据存储在高速缓存中的技术。这可以减少对主存储器的访问,从而提高系统性能。
# 6. 实践应用与案例分析
### 6.1 数据采集与处理
#### 6.1.1 传感器数据采集
在单片机系统中,传感器是获取外部环境信息的重要手段。通过使用各种传感器,单片机可以采集温度、湿度、光照强度、加速度等物理量。
**代码示例:**
```c
#include <stdio.h>
#include <stdlib.h>
#include "sensor.h"
int main() {
// 初始化传感器
sensor_init();
// 循环采集数据
while (1) {
// 读取温度传感器数据
float temperature = sensor_read_temperature();
// 读取湿度传感器数据
float humidity = sensor_read_humidity();
// 打印采集到的数据
printf("温度:%.2f℃\n", temperature);
printf("湿度:%.2f%%\n", humidity);
}
return 0;
}
```
#### 6.1.2 数据过滤与处理
采集到的传感器数据可能存在噪声或异常值,需要进行过滤和处理才能得到准确可靠的数据。常用的数据过滤方法包括:
- **移动平均滤波:**对多个连续数据点求平均值,平滑数据。
- **中值滤波:**对多个连续数据点排序,取中间值。
- **卡尔曼滤波:**一种递归滤波算法,可以根据历史数据和当前测量值估计状态。
**代码示例:**
```c
#include <stdio.h>
#include <stdlib.h>
#include "filter.h"
int main() {
// 初始化滤波器
filter_init();
// 循环采集和处理数据
while (1) {
// 读取传感器数据
float data = sensor_read_data();
// 滤波处理数据
float filtered_data = filter_process(data);
// 打印处理后的数据
printf("原始数据:%.2f\n", data);
printf("滤波后数据:%.2f\n", filtered_data);
}
return 0;
}
```
0
0
相关推荐







