posixpath实战演练:创建自定义路径操作工具的技巧分享
发布时间: 2024-10-02 01:01:04 阅读量: 29 订阅数: 35 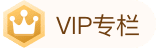
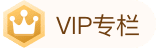
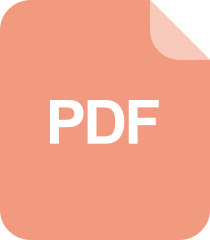
计算机视觉实战演练:算法与应用_思维导图1
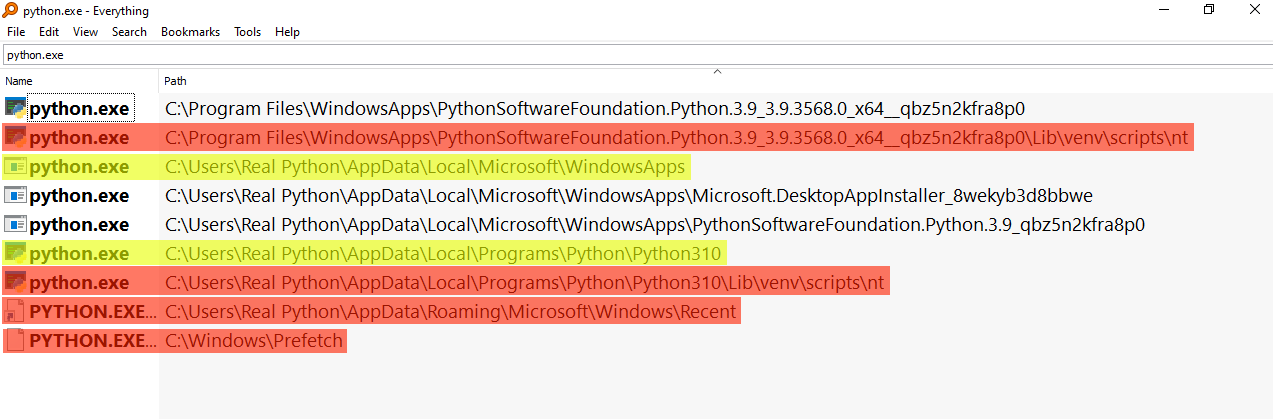
# 1. POSIX路径标准概述
## POSIX路径标准定义
POSIX(Portable Operating System Interface)路径标准定义了一组规范,用以确保操作系统之间在文件系统路径操作方面的兼容性。这些标准涉及路径的构造、分解、连接、比较、规范化和查询,为开发人员提供了一套共同遵循的路径操作规则。
## 路径操作的重要性
路径操作是文件系统管理的基础,影响着数据的存取效率和系统的安全性。良好的路径管理可以提高开发效率,确保应用的可移植性,以及避免潜在的安全漏洞。
## POSIX路径与现代应用
在多平台应用开发中,遵循POSIX路径标准对于确保应用在不同操作系统中的兼容性和稳定性至关重要。无论是本地开发还是云服务部署,掌握并正确使用POSIX路径操作都是开发者的必备技能之一。
# 2. POSIX路径的基本操作
### 2.1 路径的构建与分解
路径是文件系统中文件或目录的位置表示。在POSIX兼容的系统中,路径可以是绝对路径也可以是相对路径。绝对路径从根目录(`/`)开始,而相对路径则是相对于当前工作目录。
#### 2.1.1 构建绝对路径与相对路径
绝对路径的构建通常用于指定文件或目录的全局位置。构建时,需要确保路径字符串以根目录`/`开始,后面跟随目录层级结构。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <limits.h>
int main() {
char absolutePath[PATH_MAX];
// 构建绝对路径到当前工作目录
if (getcwd(absolutePath, sizeof(absolutePath)) == NULL) {
perror("getcwd");
return EXIT_FAILURE;
}
printf("Current working directory's absolute path: %s\n", absolutePath);
return EXIT_SUCCESS;
}
```
相对路径通常用于在当前工作目录下导航,不需要`/`作为前缀。例如,如果当前目录是`/home/user`,相对路径`Documents/report.txt`指向`/home/user/Documents/report.txt`。
#### 2.1.2 分解路径组件
分解路径组件是为了从给定的路径中提取出单独的目录或文件名。例如,使用`strtok`和`strchr`函数来分离路径中的各个部分。
```c
#include <stdio.h>
#include <string.h>
int main() {
const char *path = "/home/user/Documents/report.txt";
char *token, *str = strdup(path);
char *components[10]; // 假设路径最多有10个组件
int count = 0;
token = strtok(str, "/");
while (token != NULL) {
components[count++] = token;
token = strtok(NULL, "/");
}
printf("Path components:\n");
for (int i = 0; i < count; ++i) {
printf("%d: %s\n", i + 1, components[i]);
}
free(str);
return EXIT_SUCCESS;
}
```
### 2.2 路径的连接与比较
连接路径片段与比较路径的相等性是文件系统操作中常见的需求。
#### 2.2.1 连接路径片段
连接两个或多个路径片段时,要确保不会出现如`../`这样的路径遍历序列,从而引起安全问题。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char* path_join(const char *left, const char *right) {
const int len = strlen(left) + strlen(right) + 2;
char *path = malloc(len);
if (!path) {
perror("malloc");
exit(EXIT_FAILURE);
}
// 使用snprintf安全地连接路径
snprintf(path, len, "%s/%s", left, right);
return path;
}
int main() {
const char *left = "/home/user";
const char *right = "Documents/report.txt";
char *fullPath = path_join(left, right);
printf("Joined path: %s\n", fullPath);
free(fullPath);
return EXIT_SUCCESS;
}
```
#### 2.2.2 比较路径的相等性
比较两个路径是否相等,需要考虑到符号链接和相对路径的问题。下面示例展示如何使用`realpath`函数来解析符号链接并比较两个路径。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int paths_equal(const char *path1, const char *path2) {
char *realpath1, *realpath2;
realpath1 = realpath(path1, NULL);
if (!realpath1) {
perror("realpath");
return 0;
}
realpath2 = realpath(path2, NULL);
if (!realpath2) {
perror("realpath");
free(realpath1);
return 0;
}
int result = strcmp(realpath1, realpath2);
free(realpath1);
free(realpath2);
return result == 0;
}
int main() {
const char *path1 = "Documents/report.txt";
const char *path2 = "/home/user/Documents/report.txt";
if (paths_equal(path1, path2)) {
printf("The paths are equal.\n");
} else {
printf("The paths are not equal.\n");
}
return EXIT_SUCCESS;
}
```
### 2.3 路径的规范化与查询
路径规范化用于去除路径中不必要的部分,如`./`或`../`,查询路径信息时,常常需要获取到特定路径属性。
#### 2.3.1 规范化路径表示
规范化路径有助于统一路径的表现形式,保证其在系统中的唯一性。
```c
#include <stdio.h>
#include <stdlib.h>
#include <libgen.h>
int main() {
char path[PATH_MAX];
// 获取当前工作目录的规范路径
if (getcwd(path, sizeof(path)) == NULL) {
perror("getcwd");
return EXIT_FAILURE;
}
printf("Canonical path: %s\n", path);
// 使用realpath获取文件的规范绝对路径
char *realPath = realpath("Documents/report.txt", NULL);
if (realPath) {
printf("Real path: %s\n", realPath);
free(realPath);
} else {
perror("realpath");
}
return EXIT_SUCCESS;
}
```
#### 2.3.2 查询路径信息
查询路径信息,如文件类型、权限、所有者等,通常使用`stat`或`lstat`函数。
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
void print_file_info(const char *path) {
struct stat fileInfo;
if (stat(path, &fileInfo) == -1) {
perror("stat");
return;
}
printf("***\n", path);
printf("Size: %ld bytes\n", fileInfo.st_size);
printf("Permissions: %o\n", fileInfo.st_mode & S_IRWXU);
printf("Last modified: %ld\n", fileInfo.st_mtime);
}
int main() {
print_file_info("Documents/report.txt");
return EXIT_SUCCESS;
}
```
通过使用这些基本的路径操作,开发者可以有效地管理和处理文件系统中的文件和目录,确保其应用的跨平台兼容性和性能。
# 3. 自定义POSIX路径操作工具的构建
构建一个自定义的POSIX路径操作工具不仅需要对POSIX路径标准有深刻的理解,还需要在实际应用中对其进行扩展和优化。本章将深入探讨如何设计这样一个工具的架构,实现其主要功能,并提供测试用例来验证工具的有效性。
## 3.1 设计自定义路径工具的架构
在设计自定义路径工具的
0
0
相关推荐
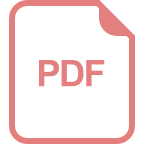
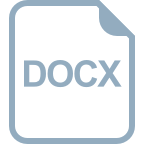
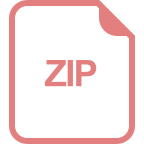
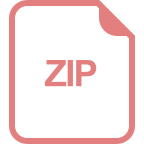
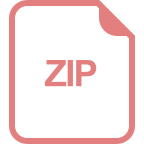
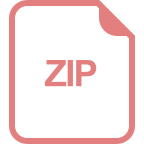
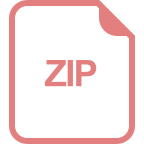