C++异常处理标准化:遵循C++11最佳实践的实践(技术总结)
发布时间: 2024-12-10 01:10:33 阅读量: 23 订阅数: 17 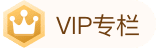
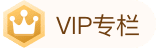
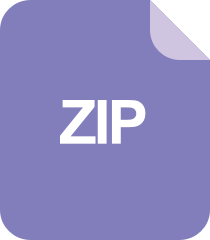
C++16《C++编程规范 规则、准则与最佳实践》)
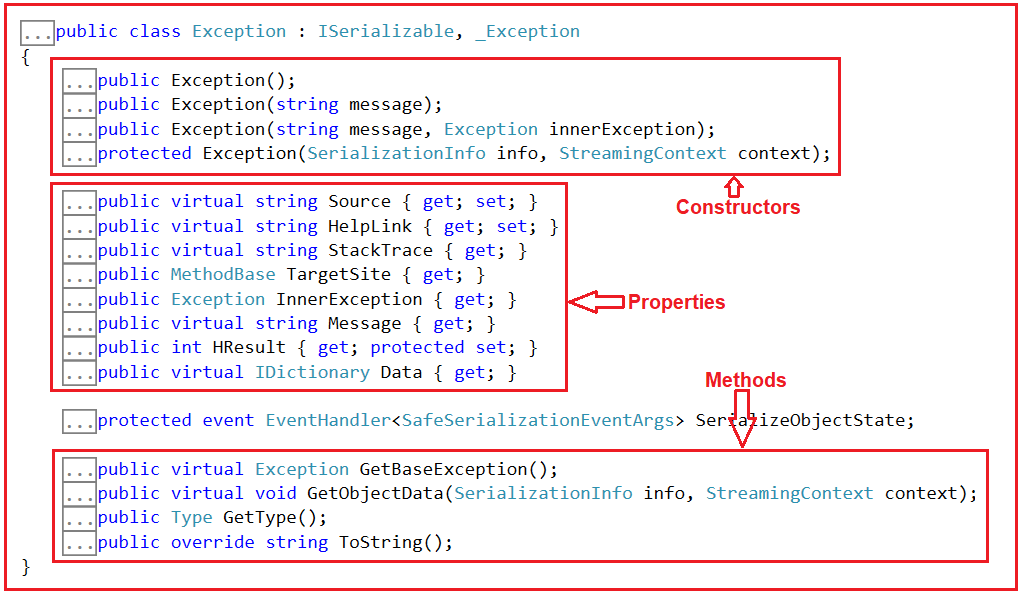
# 1. C++异常处理概述
C++是一种具有丰富特性的编程语言,异常处理是其核心组成部分之一。在C++中,异常处理允许程序在运行时处理错误和异常情况,从而避免程序崩溃,并提供更精确的错误恢复机制。异常处理的基本思想是将通常的错误处理代码与正常代码逻辑分离,使得程序的结构更加清晰,同时保证资源的正确释放。
异常处理涉及的关键概念包括异常对象、throw语句、try块、catch块以及栈展开(stack unwinding)。异常对象是当错误或异常状况发生时被创建的,用来传递错误信息的对象;throw语句用于抛出异常;try块中包含可能会抛出异常的代码;catch块则用于捕获并处理特定类型的异常。
本章将简要介绍C++异常处理的基本原则和用法,为读者在后续章节中深入理解C++11及以后版本中异常处理的机制与最佳实践打下坚实基础。
# 2. C++11异常处理机制详解
## 2.1 异常处理基础
异常处理是C++中用于处理程序运行时错误的标准机制。C++11对异常处理做了重要的扩展和改进,它使得异常处理更加强大和灵活。在这一部分,我们将深入探讨C++11中的异常处理基础,包括throw语句和try-catch块的使用。
### 2.1.1 throw语句的使用
`throw`语句被用来抛出异常。在C++中,当你遇到了一个错误或者一个不能处理的问题时,你可以使用`throw`语句抛出一个异常。这个异常可以是一个基本数据类型,也可以是一个对象。`throw`语句可以带参数,这样就相当于抛出了一个异常对象。
```cpp
throw std::runtime_error("Error message");
```
上述代码中,`throw`语句抛出了一个`std::runtime_error`类型的异常对象,其中包含了错误信息"Error message"。
**参数说明:**
- `std::runtime_error`: 这是一个标准异常类,用于报告运行时错误,如检查到的逻辑错误。
- `"Error message"`: 这是传递给`std::runtime_error`构造函数的字符串参数,用于提供错误信息。
### 2.1.2 try-catch块的构建
在程序中使用`try-catch`块来捕获和处理异常。`try`块中包含可能会抛出异常的代码。如果`try`块中的代码抛出了异常,则会被`catch`块捕获并处理。
```cpp
try {
// 可能抛出异常的代码
throw std::runtime_error("Example error");
} catch (const std::exception& e) {
// 处理异常
std::cerr << "Caught exception: " << e.what() << std::endl;
}
```
在这个示例中,如果`try`块中抛出了一个`std::runtime_error`异常,那么`catch`块将会捕获并处理它。`catch`块中的`e.what()`函数将会输出异常对象提供的错误信息。
**参数说明:**
- `catch (const std::exception& e)`: 这个`catch`块捕获了`std::exception`类型的异常,异常对象以引用的方式传入`e`。这是一个标准的异常类,它要求所有派生异常类都必须提供`what()`成员函数。
- `e.what()`: 这是调用异常对象的`what()`成员函数,返回一个描述错误信息的字符串。
## 2.2 标准异常类的结构与用法
C++的标准库提供了一系列的异常类,它们构成了一个异常类的层次结构。程序员也可以根据需要自定义异常类,来实现更加具体和丰富的错误处理。
### 2.2.1 标准异常类层次结构
C++标准异常类的层次结构以`std::exception`为基类,派生出不同的异常类来表示各种不同的错误类型。这些异常类包括但不限于`std::runtime_error`、`std::logic_error`、`std::invalid_argument`等。
```mermaid
graph TD;
std::exception --> std::logic_error
std::exception --> std::runtime_error
std::exception --> std::bad_alloc
std::exception --> std::bad_cast
std::exception --> std::bad_typeid
std::exception --> std::bad_function_call
std::exception --> |...|
std::runtime_error --> std::overflow_error
std::runtime_error --> std::range_error
std::runtime_error --> |...|
std::logic_error --> std::out_of_range
std::logic_error --> std::invalid_argument
std::logic_error --> std::length_error
std::logic_error --> |...|
```
在这个mermaid流程图中,我们可以看到标准异常类构成了一个层次结构。例如,`std::exception`是基类,它派生出了`std::runtime_error`和`std::logic_error`,它们又进一步派生出更具体的异常类,如`std::out_of_range`和`std::invalid_argument`。
### 2.2.2 自定义异常类的继承与使用
自定义异常类通常是通过继承一个标准异常类来实现的。通过继承,自定义异常类可以拥有标准异常类的所有成员函数,并且可以增加自己的特定功能。
```cpp
#include <stdexcept>
#include <string>
class MyCustomException : public std::runtime_error {
public:
MyCustomException(const std::string& message)
: std::runtime_error(message) {}
};
try {
throw MyCustomException("My custom error occurred");
} catch (const MyCustomException& e) {
std::cerr << "Caught my custom exception: " << e.what() << std::endl;
}
```
在这个代码示例中,`MyCustomException`类继承自`std::runtime_error`。当我们抛出`MyCustomException`异常时,它被`catch`块捕获并输出了错误信息。
**参数说明:**
- `MyCustomException(const std::string& message)`: 构造函数,接受一个字符串参数,将错误信息传递给基类构造函数`std::runtime_error`。
- `std::cerr << "Caught my custom exception: " << e.what() << std::endl;`: 输出捕获到的自定义异常信息,使用`std::cerr`进行错误输出,调用`what()`函数获取错误信息。
## 2.3 异常安全性的概念和实现
异常安全性是衡量程序在遭遇异常时保持状态一致性的能力。C++11引入了异常安全性保证的概念,帮助开发者编写能可靠处理异常的代码。
### 2.3.1 异常安全性基础
异常安全性主要分为三种保证:基本保证(basic guarantee)、强保证(strong guarantee)和不抛出保证(no-throw guarantee)。
- 基本保证:当异常被抛出时,程序资源(如内存、文件描述符)不会泄露,对象保持在有效的状态。
- 强保证:当异常被抛出时,程序状态不改变。如果操作失败,则撤销已经做的所有修改。
- 不抛出保证:函数保证不会抛出异常,它总是成功执行或报告错误。
### 2.3.2 实现异常安全代码的策略
要实现异常安全代码,开发者应当采取一些策略,如使用RAII(Resource Acquisition Is Initialization)管理资源,以及编写异常安全的函数。
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <stdexcept>
class FileRAII {
public:
FileRAII(const std::string& filename) {
file.open(filename, std::ios::binary | std::ios::out);
if (!file) {
throw std::runtime_error("Can't open file");
}
}
~FileRAII() {
if (file) {
file.close();
}
}
void write(const std::string& data) {
if (file) {
file << data;
}
}
private:
std::ofstream file;
};
void writeToFile(const std::string& filename, const std::string& data) {
FileRAII file(filename);
file.write(data);
// 函数结束时,file对象会自动调用析构函数
}
int main() {
try {
writeToFile("output.txt", "Test data");
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个示例中,`FileRAII`类使用RAII原则管理文件资源。当`FileRAII`对象被创建时,它尝试打开文件,并在对象被销毁时自动关闭文件。这样可以确保当异常发生时,文件资源不会泄露,实现了基本保证。
**参数说明:**
- `FileRAII(const std::string& filename)`: 构造函数,尝试打开一个文件。
- `~FileRAII()`: 析构函数,确保文件被关闭。
- `
0
0
相关推荐
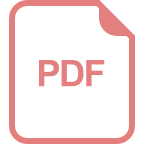
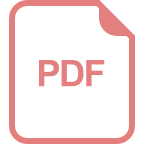
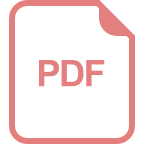
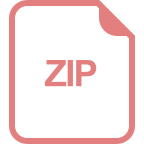
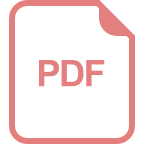
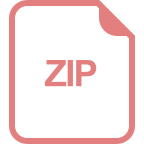
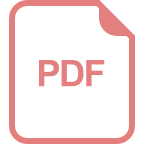
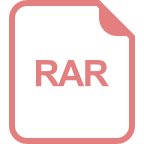