MATLAB矩阵设计模式精髓:应用设计模式提升矩阵操作代码的可重用性和可维护性
发布时间: 2024-06-08 04:47:47 阅读量: 79 订阅数: 53 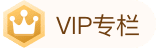
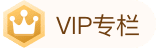
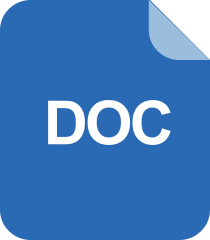
MATLAB矩阵操作设计
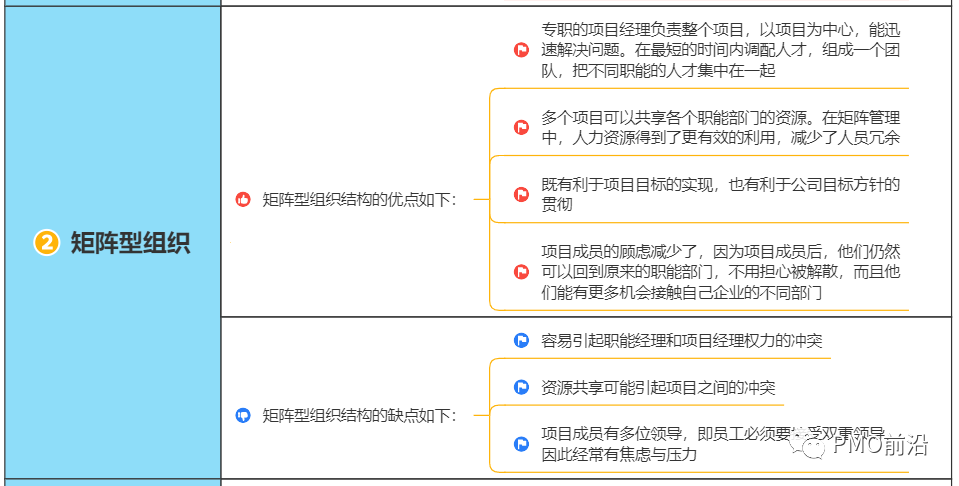
# 1. MATLAB矩阵操作基础**
MATLAB中的矩阵是数据组织的基本单位,它是一个二维数组,由行和列组成。MATLAB提供了丰富的矩阵操作函数,涵盖了从基本算术运算到高级线性代数操作等各种功能。
矩阵操作的基本语法遵循MATLAB的点语法。例如,矩阵A和B的加法操作表示为:`C = A + B`,其中C是结果矩阵。MATLAB还支持矩阵索引,允许访问矩阵中的特定元素或子矩阵。
MATLAB中矩阵操作的效率至关重要。MATLAB使用稀疏矩阵和向量化等技术来优化性能。稀疏矩阵用于表示包含大量零元素的矩阵,而向量化则通过使用内建函数来避免显式循环,从而提高代码效率。
# 2. 设计模式在矩阵操作中的应用
设计模式是一组可重用的解决方案,用于解决软件开发中常见的编程问题。在 MATLAB 矩阵操作中,设计模式可以帮助我们提高代码的可重用性、可维护性和可扩展性。本章将介绍三种在矩阵操作中常用的设计模式:策略模式、工厂模式和单例模式。
### 2.1 策略模式:实现矩阵操作的动态选择
**2.1.1 策略模式的原理和实现**
策略模式是一种行为设计模式,它允许我们动态地选择算法或策略来执行特定任务。在 MATLAB 矩阵操作中,我们可以使用策略模式来实现矩阵操作的动态选择。
策略模式的实现通常涉及以下步骤:
1. 定义一个抽象策略接口,它定义了所有策略必须实现的公共方法。
2. 创建具体策略类,它们实现了抽象策略接口中的方法,并提供了不同的实现。
3. 创建一个上下文类,它持有对策略对象的引用,并使用策略对象来执行矩阵操作。
**2.1.2 策略模式在矩阵操作中的应用实例**
考虑一个示例,其中我们想要实现一个矩阵求逆函数,该函数可以根据不同的求逆算法(例如,高斯消去法或奇异值分解)动态地选择求逆算法。
```
% 定义抽象策略接口
interface InverseStrategy
method invert(A)
end
% 创建具体策略类(高斯消去法)
class GaussInverseStrategy < InverseStrategy
method invert(A)
% 高斯消去法求逆
[L, U] = lu(A);
invA = U \ (L \ eye(size(A)));
return invA;
end
end
% 创建具体策略类(奇异值分解)
class SVDInverseStrategy < InverseStrategy
method invert(A)
% 奇异值分解求逆
[U, S, V] = svd(A);
invA = V * diag(1 ./ S) * U';
return invA;
end
end
% 创建上下文类
class MatrixInverter
private strategy
function MatrixInverter(strategy)
this.strategy = strategy;
end
function invA = invert(A)
% 使用策略对象求逆矩阵
invA = this.strategy.invert(A);
end
end
% 使用策略模式动态选择求逆算法
A = randn(5);
gaussStrategy = GaussInverseStrategy();
svdStrategy = SVDInverseStrategy();
inverter1 = MatrixInverter(gaussStrategy);
invA1 = inverter1.invert(A);
inverter2 = MatrixInverter(svdStrategy);
invA2 = inverter2.invert(A);
```
### 2.2 工厂模式:创建矩阵对象的可重用机制
**2.2.1 工厂模式的原理和实现**
工厂模式是一种创建模式,它允许我们创建对象而不指定其具体类。在 MATLAB 矩阵操作中,我们可以使用工厂模式来创建矩阵对象,而无需指定其具体类型(例如,实矩阵、复矩阵或稀疏矩阵)。
工厂模式的实现通常涉及以下步骤:
1. 定义一个抽象工厂类,它定义了创建不同类型对象的公共方法。
2. 创建具体工厂类,它们实现了抽象工厂类中的方法,并提供了不同类型的对象的创建。
3. 创建一个客户端类,它使用工厂对象来创建矩阵对象。
**2.2.2 工厂模式在矩阵操作中的应用实例**
考虑一个示例,其中我们想要实现一个矩阵创建函数,该函数可以根据不同的矩阵类型(例如,实矩阵、复矩阵或稀疏矩阵)动态地创建矩阵对象。
```
% 定义抽象工厂类
interface MatrixFactory
method createRealMatrix(rows, cols)
method createComplexMatrix(rows, cols)
method createSparseMatrix(rows, cols)
end
% 创建具体工厂类(实矩阵)
class RealMatrixFactory < MatrixFactory
method createRealMatrix(rows, cols)
% 创建实矩阵
A = randn(rows, cols);
return A;
end
end
% 创建具体工厂类(复矩阵)
class ComplexMatrixFactory < MatrixFactory
method createComplexMatrix(rows, cols)
% 创建复矩阵
A = randn(rows, cols) + 1i * randn(rows, cols);
return A;
end
end
% 创建具体工厂类(稀疏矩阵)
class SparseMatrixFactory < MatrixFactory
method createSparseMatrix(rows, cols)
% 创建稀疏矩阵
A = sprandn(rows, cols, 0.1);
return A;
end
end
% 创建客户端类
class MatrixCreator
private factory
function MatrixCreator(factory)
this.factory = factory;
end
function A = createMatrix(type, rows, cols)
% 使用工厂对象创建矩阵
switch type
case 'real'
A = this.factory.createRealMatrix(rows, cols);
case 'complex'
A = this.factory.createComplexMatrix(rows, cols);
case 'sparse'
A = this.factory.createSparseMatrix(rows, cols);
otherwise
error('Invalid matrix type');
end
end
end
% 使用工厂模式动态创建矩阵对象
realFactory = RealMatrixFactory();
complexFactory = ComplexMatrixFactory();
sparseFactory = SparseMatrixFactory();
creator1 = MatrixCreator(realFactory);
A1 = creator1.createMatrix('real', 5, 5);
creator2 = MatrixCr
```
0
0
相关推荐







