快速排序算法探秘
发布时间: 2024-04-08 21:30:41 阅读量: 16 订阅数: 14 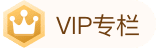
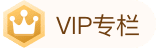
# 1. 【快速排序算法探秘】
## 一、引言
快速排序(Quick Sort)是一种高效的排序算法,由英国计算机科学家 Tony Hoare 在 1960 年提出。它是一个分治的算法,通过将数组分割成较小的子数组来递归地排序。快速排序的基本思想是选择一个基准数(pivot),将比基准数小的数放在其左边,大的数放在右边,然后对左右两个子数组分别进行排序,最终数组变得有序。相比于其他排序算法,快速排序的平均时间复杂度为 O(nlogn),且具有原地排序和不稳定性的特点。
在本文中,我们将探讨快速排序算法的原理、实现方式、优化手段以及时间复杂度分析,帮助读者更深入地理解这一经典的排序算法。接下来,让我们一起揭开快速排序算法的神秘面纱!
# 2. 快速排序算法的实现
快速排序(Quick Sort)是一种常用且高效的排序算法,它基于分治的思想,通过递归(或者迭代)将原始数组分割成较小的子数组,在保证子数组有序的基础上,通过“分而治之”的策略,最终使整个数组有序。
### 递归方式实现快速排序
在递归方式中,我们首先选择一个基准元素(通常选择数组中的第一个元素),然后将小于基准元素的元素移到基准元素的左边,将大于基准元素的元素移到基准元素的右边,最后将基准元素插入到两个子数组的中间位置。然后,对左右两个子数组进行递归排序,直到整个数组有序。
下面是Python代码实现递归方式的快速排序算法:
```python
def quick_sort_recursive(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[0]
less_than_pivot = [x for x in arr[1:] if x <= pivot]
greater_than_pivot = [x for x in arr[1:] if x > pivot]
return quick_sort_recursive(less_than_pivot) + [pivot] + quick_sort_recursive(greater_than_pivot)
# 示例
arr = [3, 6, 8, 10, 1, 2, 1]
sorted_arr = quick_sort_recursive(arr)
print(sorted_arr)
```
### 迭代方式实现快速排序
迭代方式的快速排序通常使用栈(Stack)来模拟递归过程,通过循环来实现。同样需要选定基准元素,并根据基准元素进行元素的划分和交换,直到整个数组有序。
下面是Java代码实现迭代方式的快速排序算法:
```java
public class QuickSortIteration {
public static void quickSortIterative(int[] arr, int low, int high) {
// 使用栈模拟递归过程
Stack<Integer> stack = new Stack<>();
stack.push(low);
stack.push(high);
while (!stack.isEmpty()) {
high = stack.pop();
low = stack.pop();
int pi = partition(arr, low, high);
if (pi - 1 > low) {
stack.push(low);
stack.push(pi - 1);
}
if (pi + 1 < high) {
stack.push(pi + 1);
stack.push(high);
}
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
```
0
0
相关推荐
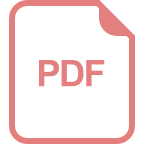
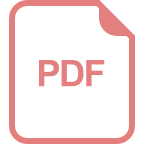





