React Native中的动画效果实现
发布时间: 2023-12-15 07:55:37 阅读量: 16 订阅数: 17 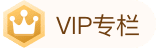
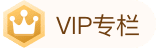
# 第一章:React Native中的动画基础
## 1.1 React Native中的动画简介
在React Native开发中,动画是提升用户体验和应用吸引力的重要组成部分。通过动画,可以让用户界面更加生动和富有交互性,提升用户的使用体验。React Native提供了丰富的动画API和组件,可以轻松实现各种动画效果。
## 1.2 动画库的选择
在React Native中,有多种动画库可供选择,例如Animated API、LayoutAnimation、React Native Reanimated等。不同的库适用于不同的场景和动画需求,开发者需要根据实际情况选择适合的动画库。
## 1.3 基本动画效果的实现
基本动画效果包括位移动画、缩放动画、旋转动画等,这些动画是React Native中常见的基础动画效果。在本节中,我们将介绍如何使用React Native提供的动画API和组件来实现这些基本动画效果。
## 第二章:动画效果的优化和性能
### 3. 第三章:基本动画类型的实现
在React Native中,实现基本的动画效果通常涉及到位移动画、缩放动画和旋转动画。这些动画类型是移动应用中常见的基本交互效果,下面将分别介绍它们的实现方法。
#### 3.1 位移动画
位移动画可以让UI元素在屏幕上沿着X轴或Y轴移动,为用户提供视觉上的反馈。在React Native中,可以使用Animated组件配合使用`Animated.timing()`或`Animated.spring()`来实现位移动画。下面是一个简单的例子,当点击按钮时,一个View组件会沿着Y轴进行位移动画:
```jsx
import React, { Component } from 'react';
import { View, Animated, Easing, TouchableOpacity } from 'react-native';
class MoveAnimationExample extends Component {
constructor(props) {
super(props);
this.state = {
translateY: new Animated.Value(0),
};
}
handleStartAnimation = () => {
Animated.timing(this.state.translateY, {
toValue: 200,
duration: 1000,
easing: Easing.linear,
}).start();
};
render() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Animated.View
style={{
width: 100,
height: 100,
backgroundColor: 'skyblue',
transform: [{ translateY: this.state.translateY }],
}}
/>
<TouchableOpacity onPress={this.handleStartAnimation}>
<View
style={{
width: 150,
height: 50,
backgroundColor: 'steelblue',
justifyContent: 'center',
alignItems: 'center',
marginTop: 20,
}}>
<Text style={{ color: 'white' }}>Start Animation</Text>
</View>
</TouchableOpacity>
</View>
);
}
}
export default MoveAnimationExample;
```
通过`Animated.timing()`定义了Y轴位移的动画效果,并在点击按钮后触发动画效果的开始。
#### 3.2 缩放动画
缩放动画可以让UI元素在屏幕上按比例进行缩放,从而产生放大或缩小的效果。在React Native中,同样可以使用`Animated.timing()`或`Animated.spring()`来实现缩放动画。以下是一个简单的例子,展示了一个View组件在缩放动画的效果:
```jsx
// 与代码相关的import语句
// ...
class ScaleAnimationExample extends Component {
constructor(props) {
super(props);
this.state = {
scale: new Animated.Value(1),
};
}
handleStartAnimation = () => {
Animated.timing(this.state.scale, {
toValue: 2,
duration: 1000,
easing: Easing.linear,
}).start();
};
render() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Animated.View
style={{
width: 100,
height: 100,
backgroundColor: 'lightgreen',
transform: [{ scale: this.state.scale }],
}}
/>
<TouchableOpacity onPress={this.handleStartAnimation}>
<View
style={{
width: 150,
height: 50,
backgroundColor: 'darkgreen',
justifyContent: 'center',
alignItems: 'center',
marginTop: 20,
}}>
<Text style={{ color: 'white' }}>Start Animation</Text>
</View>
</TouchableOpacity>
</View>
);
}
}
export default ScaleAnimationExample;
```
在上述示例中,`Animated.timing()`定义了View组件的缩放动画效果,点击按钮后触发动画效果的开始。
#### 3.3 旋转动画
旋转动画可以让UI元素围绕自身中心或特定点进行旋转,为用户带来视觉上的动感效果。在React Native中,同样可以使用`Animated.timing()`或`Animated.spring()`来实现旋转动画。以下是一个简单的例子,展示了一个View组件在旋转动画的效果:
```jsx
// 与代码相关的import语句
// ...
class RotateAnimationExample extends Component {
constructor(props) {
super(props);
this.state = {
rotate: new Animated.Value(0),
};
}
handleStartAnimation = () => {
Animated.timing(this.state.rotate, {
toValue: 360,
duration: 1000,
easing: Easing.linear,
}).start();
};
render() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Animated.View
style={{
width: 100,
height: 100,
```
0
0
相关推荐
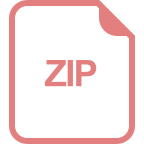
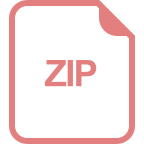
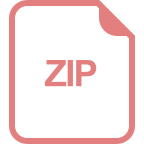
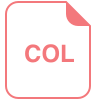
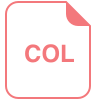
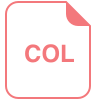
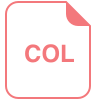

