Vue.js 中的组件化开发
发布时间: 2024-01-08 17:19:39 阅读量: 49 订阅数: 41 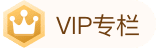
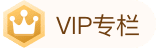
# 1. 介绍
## 1.1 Vue.js概述
Vue.js是一款流行的前端JavaScript框架,专注于实现响应式的数据驱动视图。它通过简单的API和灵活的组件化系统,能够帮助开发者快速构建交互式的界面。
## 1.2 组件化开发的概念及优势
组件化开发是一种通过将界面拆分为独立可复用的组件来构建用户界面的方法。Vue.js中,组件是可扩展的,能够封装自己的模板、样式和逻辑,并可以嵌套使用,这使得组件化开发成为Vue.js的一大特色。
组件化开发的优势包括:
- **复用性:** 可以在不同地方重复使用同一个组件,提高开发效率。
- **维护性:** 组件内部自成体系,易于维护和管理。
- **解耦:** 各个组件间相互独立,降低耦合性,便于团队协作开发。
- **可测试:** 每个组件都可以单独进行测试,提高代码的可测试性。
# 2. Vue组件基础
Vue组件是Vue.js中最强大的特性之一,它可以帮助我们构建大型、可复用、可维护的应用程序。在这一章节中,我们将会介绍Vue组件的基础知识,包括如何创建和注册Vue组件,以及组件的生命周期。
### 2.1 Vue组件的创建与注册
在Vue中,我们可以使用`Vue.component`方法来创建一个全局的Vue组件,也可以使用`components`选项来在一个Vue实例的选项中定义局部的组件。下面是一个简单的例子来说明如何创建和注册一个Vue组件:
```javascript
// 全局组件的创建
Vue.component('my-component', {
template: '<div>这是我的第一个Vue组件!</div>'
});
// 局部组件的注册
var app = new Vue({
el: '#app',
components: {
'my-local-component': {
template: '<div>这是一个局部的Vue组件!</div>'
}
}
});
```
上面的例子中,我们分别创建了一个全局组件和一个局部组件。全局组件可以在应用程序的任何地方使用,而局部组件只能在创建它的Vue实例范围内使用。
### 2.2 组件的生命周期
每个Vue组件实例都有一个完整的生命周期,包括创建、挂载、更新和销毁等阶段。在这些生命周期阶段中,我们可以利用特定的钩子函数来执行我们想要的操作。下面是Vue组件的生命周期钩子函数的一个简单示例:
```javascript
Vue.component('lifecycle-component', {
data: function() {
return {
message: 'Hello, Vue!'
};
},
created: function() {
console.log('组件被创建!');
},
mounted: function() {
console.log('组件被挂载!');
},
updated: function() {
console.log('组件更新完成!');
},
destroyed: function() {
console.log('组件被销毁!');
},
template: '<div>{{ message }}</div>'
});
```
在上述示例中,我们定义了一个名为`lifecycle-component`的Vue组件,同时定义了`created`、`mounted`、`updated`和`destroyed`等生命周期钩子函数,并在这些函数中输出相应的日志信息,以便我们能够了解组件生命周期中的各个阶段。
通过学习Vue组件的创建和注册以及生命周期的使用方法,我们可以更好地理解和使用Vue组件化开发的强大功能。
以上就是Vue组件基础的介绍,下一节我们将会学习组件间通信的方法。
# 3. 组件间通信
在Vue.js中,组件通信是非常重要的一部分,实现组件间的数据传递和交互是开发过程中必不可少的功能。组件间通信可以分为父子组件通信、兄弟组件通信以及跨级组件通信等几种情况。下面将分别介绍这几种常见的组件通信方式。
#### 3.1 父子组件通信
父子组件通信是最基本、最常见的组件通信方式。父组件通过props属性向子组件传递数据,子组件通过事件向父组件发送消息。
示例代码如下:
```vue
<template>
<div>
<h1>{{ message }}</h1> <!-- 父组件传递的数据 -->
<child-component @child-event="handleChildEvent"></child-component> <!-- 子组件触发的事件 -->
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
data() {
return {
message: 'Hello, Vue!'
};
},
components: {
ChildComponent
},
methods: {
handleChildEvent(data) {
console.log('Received message from child component:', data);
}
}
}
</script>
```
子组件可以通过emit方法触发自定义事件,并将需要传递的数据作为事件参数传递给父组件。
```vue
<template>
<button @click="handleClick">Click me</button>
</template>
<script>
export default {
methods: {
handleClick() {
this.$emit('child-event', 'Hello from child!'); // 触发自定义事件
}
}
}
</script>
```
#### 3.2 兄弟组件通信
兄弟组件通信是指处于同一层级的组件之间的通信。在Vue.js中,可以通过共享同一个父组件中的数据来实现兄弟组件之间的通信。
示例代码如下:
```vue
<template>
<div>
<component-a></component-a>
<component-b></component-b>
</div>
</template>
<script>
import ComponentA from './ComponentA.vue';
import ComponentB from './ComponentB.vue';
export default {
components: {
ComponentA,
ComponentB
}
}
</script>
```
ComponentA和ComponentB是兄弟组件,它们可以通过共享父组件的数据来进行通信。
```vue
// ComponentA.vue
<template>
<div>
<h2>{{ sharedData }}</h2>
<button @click="updateSharedData">Update</button>
</div>
</template>
<script>
export default {
data() {
return {
sharedData: 'Initial data'
}
},
methods: {
updateSharedData() {
this.sharedData = 'Updated data from ComponentA';
}
}
}
</script>
```
```vue
// ComponentB.vue
<template>
<div>
<h2>{{ sharedData }}</h2>
<button @click="updateSharedData">Update</button>
</div>
</template>
<script>
export default {
data() {
return {
sharedData: 'Initial data'
}
},
methods: {
updateSharedData() {
this.sharedData = 'Updated data from ComponentB';
}
}
}
</script>
```
在上述示例中,ComponentA和ComponentB都通过data属性定义了一个名为sharedData的数据。它们可以通过点击按钮来更新sharedData的值,从而实现兄弟组件之间的通信。
#### 3.3 跨级组件通信
在Vue.js中,跨级组件通信是指组件之间存在多级嵌套的情况下,如何实现跨级组件间的通信。
可以通过provide/inject实现跨级组件通信。provide用于在父级组件的上下文中设置数据,inject用于从父级组件的上下文中获取数据。
示例代码如下:
```vue
// ParentComponent.vue
<template>
<div>
<child-component></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
provide() {
return {
sharedData: 'This is shared data from ParentComponent'
};
},
components: {
ChildComponent
}
}
</script>
```
```vue
// ChildComponent.vue
<template>
<div>
<grandchild-component></grandchild-component>
</div>
</template>
<script>
import GrandchildComponent from './GrandchildComponent.vue';
export default {
components: {
GrandchildComponent
}
}
</script>
```
```vue
// GrandchildComponent.vue
<template>
<div>
<h2>{{ sharedData }}</h2>
</div>
</template>
<script>
export default {
inject: ['sharedData']
}
</script>
```
在上述示例中,ParentComponent通过provide方法提供了一个名为sharedData的数据,它的值是"This is shared data from ParentComponent"。然后,ChildComponent通过inject数组将sharedData注入到自己的上下文中。GrandchildComponent中可以直接使用sharedData来获取父级组件提供的共享数据。
这种方式可以在多级嵌套的组件中实现跨级通信,非常灵活和方便。
# 4. 动态组件
在Vue.js中,动态组件允许我们在不同的组件之间进行动态切换,以实现不同的功能展示需求。Vue.js提供了`<component>`标签来实现动态组件的切换。
#### 4.1 动态组件的动态切换
动态组件的切换通常是基于某个条件的变化来触发的,我们可以通过使用`v-bind:is`指令来实现动态组件的切换。
下面是一个示例的代码,用来展示不同的按钮组件:
```html
<template>
<div>
<div>
<button @click="currentComponent = 'Button1'">Button 1</button>
<button @click="currentComponent = 'Button2'">Button 2</button>
<button @click="currentComponent = 'Button3'">Button 3</button>
</div>
<component :is="currentComponent"></component>
</div>
</template>
<script>
import Button1 from './Button1.vue';
import Button2 from './Button2.vue';
import Button3 from './Button3.vue';
export default {
data() {
return {
currentComponent: 'Button1',
};
},
components: {
Button1,
Button2,
Button3,
},
};
</script>
```
在以上代码中,我们定义了三个不同的按钮组件Button1、Button2和Button3。通过点击不同的按钮,可以实现动态切换组件的功能。当点击不同的按钮时,`currentComponent`的值会改变,从而触发动态组件的切换。
#### 4.2 使用keep-alive组件缓存动态组件
在动态组件中,每次切换组件时,之前的组件都会被销毁重新创建,这可能会造成一些性能损耗。Vue.js提供了`<keep-alive>`组件来缓存动态组件,从而避免组件的重复渲染。
下面是一个使用`<keep-alive>`组件缓存动态组件的示例代码:
```html
<template>
<div>
<div>
<button @click="currentComponent = 'Button1'">Button 1</button>
<button @click="currentComponent = 'Button2'">Button 2</button>
<button @click="currentComponent = 'Button3'">Button 3</button>
</div>
<keep-alive>
<component :is="currentComponent"></component>
</keep-alive>
</div>
</template>
<script>
import Button1 from './Button1.vue';
import Button2 from './Button2.vue';
import Button3 from './Button3.vue';
export default {
data() {
return {
currentComponent: 'Button1',
};
},
components: {
Button1,
Button2,
Button3,
},
};
</script>
```
在以上代码中,我们将动态组件`<component>`标签放在`<keep-alive>`标签之内,这样在切换组件时,之前的组件不会被销毁,而是被缓存起来,从而提高了组件切换的性能。
总结:
- 动态组件允许我们在不同的组件之间进行动态切换。
- 使用`<component>`标签和`v-bind:is`指令可以实现动态组件的切换。
- 使用`<keep-alive>`组件可以缓存动态组件,避免重复渲染。
# 5. 插槽(Slot)
在Vue.js中,插槽(Slot)是一种用于组件间内容分发的机制。它允许我们将组件的内容进行分离,使得组件更加灵活和可复用。
### 5.1 插槽的基本用法
在组件中使用插槽非常简单,只需在组件的模板中加入`<slot></slot>`标签即可。例如,我们有一个名为`Card`的组件,它有一个标题和内容需要展示,我们可以在组件的模板中添加两个插槽:
```vue
<template>
<div class="card">
<h2><slot name="title"></slot></h2>
<div><slot name="content"></slot></div>
</div>
</template>
```
然后,在使用`Card`组件的地方,我们可以通过`<template>`标签内的内容来填充插槽:
```vue
<Card>
<template v-slot:title>
Card Title
</template>
<template v-slot:content>
This is the content of the card.
</template>
</Card>
```
这样,`Card`组件中的两个插槽就会被填充上相应的内容。
### 5.2 具名插槽与作用域插槽
除了基本的插槽用法,Vue.js还提供了具名插槽和作用域插槽来进一步增加灵活性。
具名插槽允许我们定义多个插槽,并通过`v-slot`指令来选择要填充的插槽。例如,在`Card`组件中,我们可以将标题和内容分别定义为具名插槽:
```vue
<template>
<div class="card">
<slot name="title"></slot>
<slot name="content"></slot>
</div>
</template>
```
然后,在使用`Card`组件时,我们可以通过`v-slot`指令来选择具体要填充的插槽:
```vue
<Card>
<template v-slot:title>
<h2>Card Title</h2>
</template>
<template v-slot:content>
<p>This is the content of the card.</p>
</template>
</Card>
```
作用域插槽则允许我们在插槽内部访问父组件的数据。我们可以通过在插槽的模板中使用`slot-scope`来声明作用域变量。例如,在`List`组件中,我们可以通过作用域插槽来展示列表的每一项:
```vue
<template>
<ul>
<slot name="item" v-for="item in list" :item="item"></slot>
</ul>
</template>
```
然后,在使用`List`组件时,我们可以在插槽内部访问父组件的数据:
```vue
<List>
<template v-slot:item="slotProps">
<li>{{ slotProps.item }}</li>
</template>
</List>
```
通过作用域插槽,我们可以方便地将父组件的数据传递给插槽内部,实现更加灵活的组件组合。
插槽是Vue.js中非常强大且有用的功能,它使得组件可以更加灵活地适应各种需求。掌握插槽的基本用法以及具名插槽和作用域插槽的高级用法,可以提升组件的复用性和可拓展性。
这里是一个基于Vue.js的插槽示例代码:
```vue
<template>
<div>
<h1>{{ title }}</h1>
<!-- 使用具名插槽 -->
<slot name="content" :content="content"></slot>
<!-- 使用作用域插槽 -->
<slot name="footer" v-bind="footerData"></slot>
</div>
</template>
<script>
export default {
name: 'MyComponent',
data() {
return {
title: 'Hello World',
content: 'This is the content of the component',
footerData: {
author: 'John Doe',
year: 2021
}
};
}
};
</script>
```
```html
<MyComponent>
<!-- 使用具名插槽 -->
<template v-slot:content="slotProps">
<p>{{ slotProps.content }}</p>
</template>
<!-- 使用作用域插槽 -->
<template v-slot:footer="slotProps">
<footer>{{ slotProps.author }} - {{ slotProps.year }}</footer>
</template>
</MyComponent>
```
代码解释:
- 在`MyComponent`组件的模板中,我们定义了一个默认插槽`<slot></slot>`和两个具名插槽`<slot name="content"></slot>`和`<slot name="footer"></slot>`。
- `MyComponent`组件中的`data`属性包含了标题、内容和页脚数据。
- 在使用`MyComponent`组件时,我们使用`<template>`标签来填充各个插槽。具名插槽中可以通过`v-slot`指令来为插槽指定名称,作用域插槽中可以通过`v-bind`指令将父组件的数据传递给插槽内部。
代码总结:
- 插槽是Vue.js中用于组件间内容分发的机制,可以提升组件的灵活性和可复用性。
- 使用`<slot></slot>`标签可以在组件中定义插槽,使用`<template>`标签来填充插槽。
- 具名插槽允许我们定义多个插槽,并使用`v-slot`指令来选择要填充的插槽。
- 作用域插槽允许我们在插槽内部访问父组件的数据,可以通过在插槽的模板中使用`slot-scope`来声明作用域变量。
结果说明:
- 在浏览器中运行上述示例代码,将会显示一个标题为"Hello World"的组件,组件中包含了内容和页脚。
- 具名插槽中的内容和作用域插槽中的数据都会被正确地填充到组件中相应的位置。
# 6. 组件化开发的实践
在Vue.js中,组件化开发不仅可以提高代码的复用性和可维护性,还可以实现更高效的团队协作。本章将介绍几种组件化开发的实践方法。
### 6.1 构建可复用的组件库
构建可复用的组件库是组件化开发的基础。通过封装通用的UI组件,我们可以在项目中反复使用这些组件,减少重复编写代码的工作量。
以下是一个示例的构建可复用的组件库的步骤和代码示例:
#### 步骤1:创建一个新的项目目录
在命令行中执行以下命令创建一个新的项目目录,用于存放组件库的代码。
```
mkdir my-component-library
cd my-component-library
```
#### 步骤2:初始化npm
执行以下命令初始化npm,并按照提示填写相关信息。
```
npm init
```
#### 步骤3:创建组件
在项目目录下创建一个名为`components`的文件夹,并在其中创建一个名为`Button.vue`的文件,用于封装一个按钮组件。
```html
<template>
<button class="button">
<slot></slot>
</button>
</template>
<script>
export default {
name: 'Button'
}
</script>
<style>
.button {
/* 按钮样式 */
}
</style>
```
#### 步骤4:打包组件
在项目目录下创建一个名为`webpack.config.js`的文件,并按以下配置进行配置。
```javascript
const path = require('path');
module.exports = {
entry: './components/Button.vue',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js',
library: 'my-component-library',
libraryTarget: 'umd',
umdNamedDefine: true
},
module: {
rules: [
{
test: /\.vue$/,
loader: 'vue-loader'
}
]
}
};
```
执行以下命令将组件打包成一个独立的文件。
```
npx webpack --config webpack.config.js
```
#### 步骤5:发布组件库
执行以下命令将组件库发布到npm上。
```
npm publish
```
通过以上步骤,我们成功构建了一个可复用的组件库。其他项目可以通过执行以下命令安装并使用该组件库。
```
npm install my-component-library
```
### 6.2 使用Vue CLI进行组件开发与发布
Vue CLI是一个提供了开发Vue.js项目的快速环境搭建和模板生成的工具。使用Vue CLI可以方便地进行组件开发和发布。
以下是使用Vue CLI进行组件开发与发布的步骤:
#### 步骤1:安装Vue CLI
在命令行中执行以下命令全局安装Vue CLI。
```
npm install -g @vue/cli
```
#### 步骤2:创建一个新的组件项目
执行以下命令创建一个新的组件项目。
```
vue create my-component-library
```
根据提示选择默认设置或自定义项目配置。
#### 步骤3:编写组件
在项目目录下的`src`文件夹中,创建一个名为`Button.vue`的文件,用于封装一个按钮组件。可以根据需要添加相关组件代码和样式。
#### 步骤4:打包组件
执行以下命令打包组件。
```
npm run build
```
#### 步骤5:发布组件库
执行以下命令将组件库发布到npm上。
```
npm publish
```
通过以上步骤,我们成功使用Vue CLI进行了组件开发与发布。
这些实践方法可以帮助我们更好地进行组件化开发,提高代码的复用性和开发效率。在实际项目中,根据具体需求和团队情况,可以结合其他工具和技术进一步优化组件化开发的流程和体验。
0
0
相关推荐
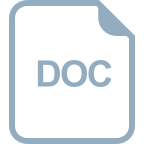
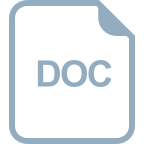
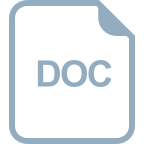
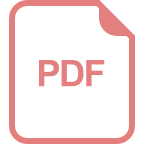
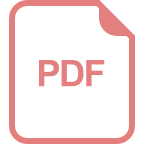
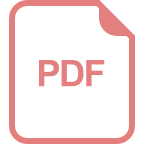
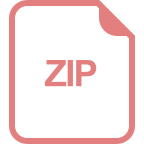
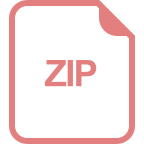
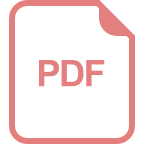