配置Spring Security:认证与授权
发布时间: 2024-02-22 06:13:52 阅读量: 42 订阅数: 21 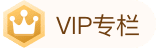
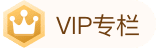
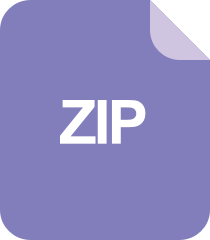
spring security认证和授权
# 1. Spring Security简介
## 1.1 Spring Security简介和背景
Spring Security是一个强大且高度可定制的身份验证和访问控制框架,它是基于Spring框架的一个扩展,用于帮助开发人员构建安全的应用程序。
## 1.2 Spring Security的核心功能和优势
Spring Security提供了诸如认证、授权、会话管理等核心功能,通过集成Spring Security,开发人员可以轻松地增强应用程序的安全性,保护用户的敏感数据。
## 1.3 Spring Security在现代Web应用中的重要性
随着Web应用程序的普及,安全性变得尤为重要。Spring Security提供了一种可靠的方式来保护应用程序免受各种安全威胁,如跨站脚本攻击(XSS)、跨站请求伪造(CSRF)等。
接下来,我们将深入探讨如何配置Spring Security来实现认证与授权。
# 2. 认证的配置与实现
认证是确保用户是谁的过程,是保护系统免受未经授权的访问的重要一环。在Spring Security中,我们可以通过不同方式实现认证,包括基于内存、基于数据库、使用LDAP以及自定义认证提供者。接下来将介绍这些认证方式的配置与实现。
### 2.1 用户认证的基本概念
在进行认证配置之前,首先要了解用户认证的基本概念。用户认证是确认用户身份的过程,一般通过用户名和密码来进行验证。Spring Security提供了多种方式来支持用户认证,确保系统的安全性。
### 2.2 基于内存的认证
基于内存的认证是最简单的一种认证方式,适用于小型应用或者测试环境。通过在配置文件中配置用户名、密码和角色信息来实现认证。
```java
@Configuration
@EnableWebSecurity
public class MemoryAuthenticationConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user")
.password("{noop}password")
.roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
```
代码解析:
- `configure(AuthenticationManagerBuilder auth)`: 配置用户名、密码和角色信息。
- `configure(HttpSecurity http)`: 配置允许所有用户访问,同时启用基于表单的登录验证。
运行代码后,用户可以使用用户名"user"和密码"password"来登录系统,并被授予"USER"角色。
### 2.3 基于数据库的认证
基于数据库的认证是更常见的一种方式,用户信息存储在数据库中,通过查询数据库来验证用户身份。
```java
@Configuration
@EnableWebSecurity
public class DatabaseAuthenticationConfig extends WebSecurityConfigurerAdapter {
@Autowired
private DataSource dataSource;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.jdbcAuthentication().dataSource(dataSource)
.usersByUsernameQuery("SELECT username, password, enabled FROM users WHERE username=?")
.authoritiesByUsernameQuery("SELECT username, authority FROM authorities WHERE username=?");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
```
代码解析:
- 通过`dataSource`注入数据源。
- `configure(AuthenticationManagerBuilder auth)`: 配置查询用户信息和权限信息的SQL语句。
- `configure(HttpSecurity http)`: 配置允许所有用户访问,同时启用基于表单的登录验证。
通过配置数据库认证,系统将会根据数据库中存储的用户信息进行认证。
### 2.4 使用LDAP进行认证
LDAP是一种常用的轻量级目录访问协议,通过LDAP进行认证可以方便地对大量用户进行管理和认证。
```java
@Configuration
@EnableWebSecurity
public class LdapAuthenticationConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.ldapAuthentication()
.userDnPatterns("uid={0},ou=people")
.groupSearchBase("ou=groups")
.contextSource()
.url("ldap://localhost:389/dc=springframework,dc=org")
.managerDn("cn=admin")
.managerPassword("password");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin();
}
}
```
代码解析:
- `configure(AuthenticationManagerBuilder auth)`: 配置LDAP服务器的连接信息和用户、组信息的查找规则。
- `configure(HttpSecurity http)`: 配置允许所有用户访问,同时启用基于表单的登录验证。
通过LDAP认证,系统可以实现与LDAP服务器的集成认证功能。
### 2.5 自定义认证提供者
除了上述方式外,我们还可以自定义认证提供者来满足特定的认证需求。自定义认证提供者需要实现`AuthenticationProvider`接口。
```java
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
// 自定义认证逻辑
return new UsernamePasswordAuthenticationToken(username, password, new ArrayList<>());
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
代码解析:
- 实现了`AuthenticationProvider`接口,并重写了`authenticate`和`supports`方法。
- 可以在`authenticate`方法中实现自定义的认证逻辑。
通过自定义认证提供者,我们可以自定义认证过程,满足特定的认证需求。
这些是配置Spring Security中认证的几种常见方式,根据实际需求选择合适的认证方式,并结合章节二中的配置方法进行实现。
# 3. 授权的配置与实现
在本章中,我们将深入探讨如何配置和实现Spring Security中的授权功能。授权是指确定用户是否有权限执行特定操作或访问特定资源的过程。在现代Web应用中,良好的授权机制是确保系统安全性的关键。
#### 3.1 授权概念和权限管理
授权是指验证用户是否有权执行某个操作或访问某个资源。权限管理是指对用户所具有的权限进行管理和控制。在Spring Security中,授权可以基于角色、权限或表达式进行配置。合理的权限管理可以有效地保护系统的安全性和数据的完整性,避免未经授权的用户访问敏感资源。
#### 3.2 基于角色的授权
基于角色的授权是指通过为用户分配特定角色来控制其权限。在Spring Security中,可以使用`hasRole()`方法进行基于角色的授权配置。例如,通过配置`hasRole("ADMIN")`来确保只有拥有"ADMIN"角色的用户才能执行特定操作。
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
```
#### 3.3 基于权限的授权
基于权限的授权是指直接针对用户的具体权限进行控制。在Spring Security中,可以使用`hasAuthority()`方法进行基于权限的授权配置。例如,通过配置`hasAuthority("READ_WRITE")`来确保只有具有"READ_WRITE"权限的用户才能执行特定操作。
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasAuthority("ADMIN_READ_WRITE")
.antMatchers("/user/**").hasAuthority("USER_READ")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
```
#### 3.4 表达式授权
Spring Security还支持使用表达式进行细粒度的授权配置。可以通过`access`关键字和表达式来实现更加灵活和动态的授权控制。例如,可以通过配置`access("hasRole('ADMIN') and hasIpAddress('192.168.1.0/24')")`来实现同时需要"ADMIN"角色和特定IP地址才能访问的授权规则。
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").access("hasRole('ADMIN')")
.antMatchers("/user/**").access("hasRole('USER')")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
```
#### 3.5 动态权限配置与管理
在实际应用中,权限管理通常是动态的,用户的权限可能会根据角色变化、业务流程变化等动态变化。Spring Security提供了基于方法的安全性和动态权限配置的支持,可以实现更加灵活和动态的权限管理。
以上是关于Spring Security授权配置与实现的内容,合理的授权配置可以帮助我们更好地保护系统资源,确保系统安全性。
# 4. 安全配置
在构建和配置Spring Security时,保障应用程序的安全性是至关重要的。本章将重点介绍如何进行安全配置,包括配置HTTP请求权限、Session管理、CSRF保护以及使用Stateless Token认证等内容。
### 4.1 安全配置类介绍
在Spring Security中,安全配置类起着至关重要的作用。通过安全配置类,我们可以对认证、授权和其他安全机制进行定义和定制。一般来说,我们需要继承`WebSecurityConfigurerAdapter`类,并重写`configure`方法来进行安全配置。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
```
### 4.2 配置HTTP请求权限
通过配置HTTP请求权限,我们可以精细地控制哪些请求需要进行认证,哪些请求不需要认证即可访问。在上面的示例中,我们使用`.antMatchers()`来指定不同的URL路径需要不同的权限控制。
### 4.3 配置Session管理
Spring Security也提供了Session管理的功能,可以帮助我们管理用户的Session状态,防止Session Fixation攻击等。可以通过在`configure`方法中进行配置,例如限制用户在同一时间只能有一个活跃会话。
### 4.4 CSRF保护
跨站请求伪造(Cross-Site Request Forgery,CSRF)是常见的安全威胁之一。Spring Security提供了CSRF保护功能,可以防止CSRF攻击。在`configure`方法中,我们可以配置CSRF保护的相关属性,Spring Security会帮助我们处理CSRF Token。
### 4.5 使用Stateless Token认证
除了Session认证方式外,还可以使用Stateless Token认证的方式来实现认证。Stateless Token认证不保存用户状态在服务端,每次请求都需要携带Token进行认证,适用于分布式系统和前后端分离的应用。
总的来说,在安全配置中,我们需要综合考虑应用程序的特点和安全需求,结合Spring Security提供的功能进行配置,以实现全面的安全保护和控制。
通过以上内容,您可以更深入地了解如何配置Spring Security中的安全功能,从而保障应用程序的安全性。
# 5. Spring Security与第三方认证
在现代Web应用程序中,越来越多的应用需要与第三方身份验证提供者集成,以实现单点登录和更强大的身份验证功能。Spring Security提供了与各种第三方认证服务集成的功能,使开发人员能够轻松地实现这些功能。
### 5.1 集成OAuth2
OAuth2是一种用于授权的开放标准,允许用户授权第三方应用访问他们存储在授权服务器上的资源,而无需将用户名和密码分享给第三方应用。Spring Security提供了OAuth2客户端和资源服务器支持,可以帮助开发人员快速集成OAuth2认证。
下面是一个使用Spring Security OAuth2客户端的示例代码:
```java
@EnableOAuth2Sso
@Configuration
public class OAuth2Configuration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.antMatcher("/**")
.authorizeRequests()
.antMatchers("/", "/login**")
.permitAll()
.anyRequest()
.authenticated();
}
}
```
在上面的代码中,我们通过@EnableOAuth2Sso注解启用了OAuth2单点登录,并配置了应用程序的安全规则。
### 5.2 集成OpenID Connect
OpenID Connect是建立在OAuth2之上的一种身份验证协议,它为用户提供了身份验证和基本资料的验证。Spring Security提供了对OpenID Connect的支持,使开发人员能够轻松地将OpenID Connect集成到他们的应用程序中。
以下是一个使用Spring Security集成OpenID Connect的示例代码:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login", "/error")
.permitAll()
.anyRequest()
.authenticated()
.and()
.oauth2Login();
}
}
```
在上面的代码中,我们配置了对/login和/error路径的开放访问,并启用了OAuth2登录功能。
### 5.3 集成SAML
Security Assertion Markup Language(SAML)是一种用于基于Web的单点登录(SSO)的开放标准。Spring Security提供了对SAML的支持,使开发人员能够将SAML集成到他们的应用程序中,实现跨组织的SSO功能。
以下是一个简单的Spring Security配置示例,用于集成SAML:
```java
@EnableWebSecurity
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home")
.permitAll()
.anyRequest()
.authenticated()
.and()
.saml2Login();
}
}
```
通过上面的配置,我们启用了SAML2单点登录,并配置了应用程序的安全规则。
### 5.4 集成JWT
JSON Web Token(JWT)是一种用于在网络应用之间安全地传输信息的开放标准。Spring Security提供了对JWT的支持,使开发人员能够使用JWT来实现身份验证和授权。
以下是一个简单的Spring Security JWT集成示例代码:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public-api/**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.addFilter(new JwtAuthenticationFilter(authenticationManager()));
}
}
```
在上面的代码中,我们配置了对“/public-api/**”路径的开放访问,并添加了一个JWT身份验证过滤器。
通过以上介绍,我们可以看到Spring Security提供了丰富的功能和支持,使开发人员能够轻松集成各种第三方身份验证服务,增强应用程序的安全性和用户体验。
# 6. 最佳实践与故障排除
在配置Spring Security时,遵循最佳实践并及时排除故障是至关重要的。本章将介绍一些实践经验和故障排除建议,帮助您更好地保护应用程序和数据。
### 6.1 安全配置最佳实践
在配置Spring Security时,有几项安全配置最佳实践值得注意:
- **定期更新依赖项**:保持Spring Security及相关依赖项的最新版本,以获取最新的安全补丁和功能更新。
- **使用强密码**:配置密码策略,要求用户使用强密码,包括长度、混合字符和特殊符号等。
- **限制登录尝试次数**:为防止暴力破解,限制用户登录尝试次数并实施锁定账户功能。
- **启用HTTPS**:使用HTTPS协议传输敏感数据,确保数据在传输过程中的安全性。
- **最小化权限**:最小化每个用户和角色的权限,避免赋予过多权限而导致安全风险。
### 6.2 防止安全漏洞的常见措施
在开发和配置过程中,需要注意一些常见的安全漏洞,以及相应的防范措施:
- **跨站脚本攻击(XSS)**:对用户输入数据进行验证和转义,避免恶意脚本注入。
- **跨站请求伪造(CSRF)**:实施CSRF Token来验证用户请求的合法性。
- **不安全的直接对象引用**:避免直接引用对象ID等敏感数据,使用间接引用或权限验证。
- **敏感信息泄露**:避免在错误消息或日志中暴露敏感信息,做好错误处理和日志记录。
### 6.3 针对常见问题的解决方案
在实际应用中,可能会遇到一些常见的问题,例如权限无效、认证失败等,针对这些问题可以采取以下解决方案:
- **检查配置**:仔细检查Spring Security的配置,确保配置正确无误。
- **查看日志**:查看应用程序日志,定位具体错误信息,排除问题根源。
- **调试代码**:在必要时添加日志输出或调试断点,逐步排查问题。
### 6.4 日常安全监控和日志记录建议
建议在生产环境中进行日常安全监控和日志记录,以及定期对安全配置进行审查和更新:
- **监控安全事件**:监控登录失败、权限访问异常等安全事件,及时发现并应对安全威胁。
- **记录安全日志**:记录安全事件日志,包括用户操作、异常情况等,便于事后分析和追踪。
- **定期审查配置**:定期审查Spring Security的配置,更新依赖项,并根据需要调整安全策略。
通过以上最佳实践和故障排除建议,可以有效增强应用程序的安全性,并在出现问题时能够及时做出反应和处理,保障系统和数据的安全。
0
0
相关推荐






