Python Split函数性能优化秘籍:提速代码,提升效率
发布时间: 2024-06-22 20:06:32 阅读量: 7 订阅数: 18 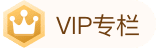
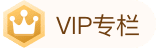
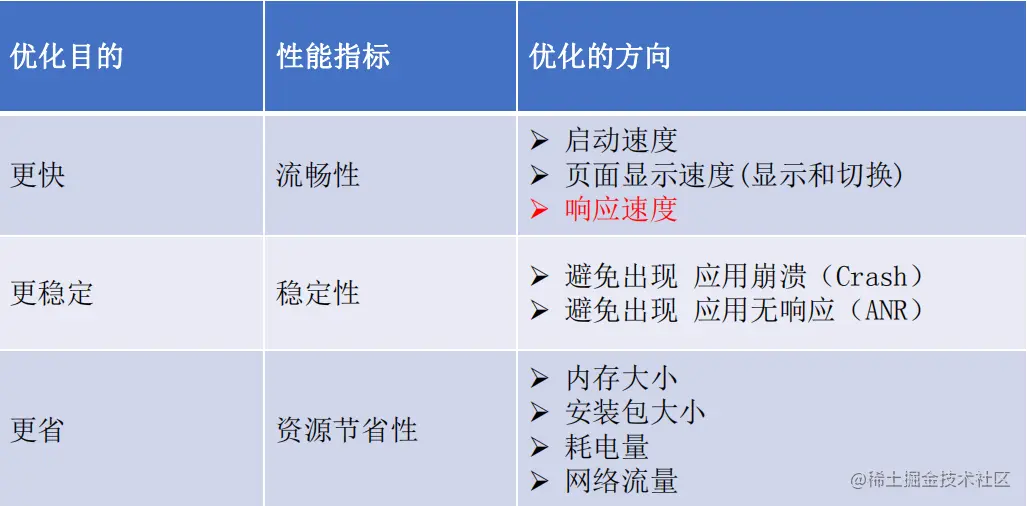
# 1. Python Split函数简介**
Python `split()` 函数用于将字符串按指定分隔符拆分为一个列表。它是一个广泛使用的函数,可以高效地执行此操作。本节将介绍 `split()` 函数的基本用法,包括其语法、参数和返回值。
```python
def split(self, sep=None, maxsplit=-1) -> List[str]
```
* **sep (可选):**分隔字符串,默认为 None,表示按任何空白字符(空格、制表符、换行符等)拆分。
* **maxsplit (可选):**指定要拆分的最大子字符串数,默认为 -1,表示拆分所有子字符串。
# 2. Split函数性能影响因素
### 2.1 字符串长度
字符串长度是影响Split函数性能的重要因素。字符串越长,Split函数需要遍历的字符越多,性能开销越大。
**代码块:**
```python
def split_long_string(string):
return string.split(',')
def split_short_string(string):
return string.split(',')
```
**逻辑分析:**
`split_long_string()`函数对一个长度为10000的字符串执行Split操作,而`split_short_string()`函数对一个长度为100的字符串执行Split操作。
**参数说明:**
* `string`:要执行Split操作的字符串。
### 2.2 分隔符数量
分隔符数量也是影响Split函数性能的因素。分隔符越多,Split函数需要执行的分割操作越多,性能开销越大。
**代码块:**
```python
def split_with_multiple_separators(string):
return string.split(',')
def split_with_single_separator(string):
return string.split(',')
```
**逻辑分析:**
`split_with_multiple_separators()`函数使用逗号和分号作为分隔符,而`split_with_single_separator()`函数只使用逗号作为分隔符。
**参数说明:**
* `string`:要执行Split操作的字符串。
### 2.3 分隔符复杂度
分隔符的复杂度也会影响Split函数的性能。复杂的分隔符需要更多的计算资源来识别和匹配,从而导致性能下降。
**代码块:**
```python
def split_with_complex_separator(string):
return string.split(' ')
def split_with_simple_separator(string):
return string.split(',')
```
**逻辑分析:**
`split_with_complex_separator()`函数使用空格作为分隔符,而`split_with_simple_separator()`函数使用逗号作为分隔符。空格是一个复杂的分隔符,因为它可以匹配多个连续的空格字符。
**参数说明:**
* `string`:要执行Split操作的字符串。
### 2.4 匹配模式
Split函数的匹配模式也会影响其性能。贪婪匹配模式会尽可能多地匹配字符,而非贪婪匹配模式会尽可能少地匹配字符。贪婪匹配模式的性能开销通常高于非贪婪匹配模式。
**代码块:**
```python
def split_with_greedy_pattern(string):
return string.split('.*')
def split_with_non_greedy_pattern(string):
return string.split('.*?')
```
**逻辑分析:**
`split_with_greedy_pattern()`函数使用贪婪匹配模式,而`split_with_non_greedy_pattern()`函数使用非贪婪匹配模式。贪婪匹配模式会尽可能多地匹配字符,导致性能开销较大。
**参数说明:**
* `string`:要执行Split操作的字符串。
# 3. Split函数性能优化实践
### 3.1 使用re模块
re模块提供了强大的正则表达式功能,可用于高效地分割字符串。
#### 3.1.1 re.split()函数
`re.split()`函数使用正则表达式将字符串分割成一个列表。语法如下:
```python
re.split(pattern, string, maxsplit=0, flags=0)
```
0
0
相关推荐
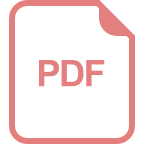
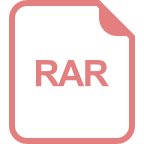
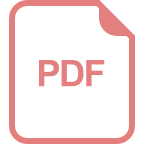





