Go语言中的并发编程与数据持久化
发布时间: 2024-02-22 05:44:11 阅读量: 35 订阅数: 23 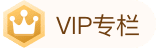
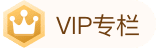
# 1. Go语言中的并发编程简介
并发编程是当今软件开发中的重要话题,尤其是在多核处理器成为主流的今天。Go语言作为一门支持并发编程的优秀语言,提供了丰富的并发编程机制,其中最为突出的就是goroutines和channel。本章将介绍Go语言中的并发编程概念、机制以及goroutines与channel的基本用法。让我们一起深入探讨吧。
## 1.1 并发编程的概念
在计算机科学中,并发是指多个独立的执行流同时存在,并发的各个执行单元之间可以相互交错执行。并发编程是利用计算机系统的多个计算资源(如CPU核心)同时处理多个任务的编程方式,以提高程序的性能和效率。
## 1.2 Go语言中的并发机制
Go语言通过goroutines和channel提供了原生的支持,使并发编程变得简单而又强大。goroutine是由Go语言运行时负责调度的轻量级线程,相比传统的线程消耗更少的资源。而channel则是用来在goroutines之间进行通信和同步的数据结构。
## 1.3 Goroutines与Channel的基本用法
在Go语言中,我们可以使用关键字`go`来创建一个goroutine,简单地将一个函数调用前加上`go`即可让这个函数在一个新的goroutine中执行。而channel则可以通过`make(chan 类型)`来创建,用于在goroutines之间传递数据。
```go
package main
import "fmt"
func sayHello(s string) {
fmt.Println("Hello,", s)
}
func main() {
// 创建一个goroutine
go sayHello("world")
// 等待程序在子goroutine执行完毕后再退出
var input string
fmt.Scanln(&input)
}
```
在上面的示例中,`sayHello`函数被放在一个新的goroutine中执行,主程序会等待用户输入后再退出,从而保证`sayHello`函数有足够的时间执行。
以上就是Go语言中并发编程的基础知识,接下来我们将进一步探讨并发编程的实践应用。
# 2. Go语言中的并发编程实践
在Go语言中,利用并发编程可以更有效地利用CPU资源,提高程序的性能。本章将介绍如何在Go语言中进行并发编程的实践,包括使用goroutines实现并发任务、利用channel进行数据交换与同步以及避免并发问题的最佳实践。
### 2.1 使用goroutines实现并发任务
在Go语言中,可以通过goroutines实现并发任务。goroutine是一种轻量级的线程,由Go语言的运行时管理。使用goroutines可以很容易地实现并发任务的执行,下面是一个简单的示例:
```go
package main
import (
"fmt"
"time"
)
func task(id int) {
for i := 0; i < 3; i++ {
fmt.Printf("Task %d: %d\n", id, i)
time.Sleep(time.Second)
}
}
func main() {
for i := 0; i < 3; i++ {
go task(i)
}
time.Sleep(4 * time.Second)
}
```
在上面的示例中,我们定义了一个`task`函数,会打印任务ID和任务执行的次数,然后使用`go task(i)`开启了3个goroutines来执行任务。最后通过`time.Sleep`等待所有任务执行完成。运行该程序可以看到各个任务交替执行的结果。
### 2.2 利用channel进行数据交换与同步
在并发编程中,为了避免竞态条件以及协调不同goroutines之间的操作,可以使用channel进行数据交换与同步。下面是一个使用channel的示例:
```go
package main
import "fmt"
func producer(ch chan int) {
for i := 0; i < 5; i++ {
ch <- i
}
close(ch)
}
func consumer(ch chan int) {
for val := range ch {
fmt.Println("Consumed:", val)
}
}
func main() {
ch := make(chan int)
go producer(ch)
go consumer(ch)
// 等待goroutines执行完毕
var input string
fmt.Scanln(&input)
}
```
上面的示例中,我们定义了一个`producer`函数往channel中发送数据,定义了一个`consumer`函数从channel中接收数据。在`main`函数中创建了一个channel,然后启动了两个goroutines分别执行生产者和消费者任务,并通过`range ch`从channel中读取数据。最后通过`fmt.Scanln`等待程序执行完成。
### 2.3 避免并发问题的最佳实践
在Go语言中,为了避免并发问题(如竞态条件、死锁等),可以采用一些最佳实践,如避免使用全局变量、使用`sync`包的锁、避免goroutines泄露等。在编写并发程序时,务必考虑数据的安全访问,保证程序的正确性和稳定性。
通过本章的学习,您可以更好地理解如何在Go语言中进行并发编程的实践,提高程序的性能和效率。在下一章中,我们将深入探讨Go语言中的数据持久化介绍。
# 3. Go语言中的数据持久化介绍
在本章中,我们将介绍Go语言中数据持久化的相关概念,不同的数据持久化方案以及数据库选择
0
0
相关推荐
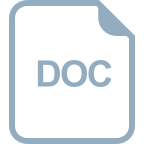
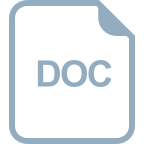
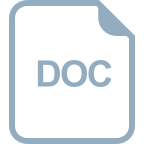
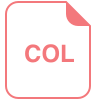
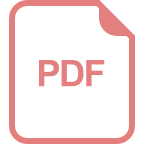
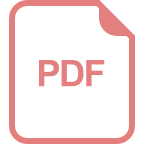
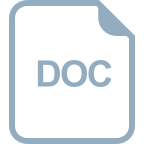
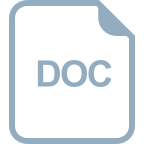
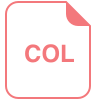