React组件通信与状态提升
发布时间: 2024-02-16 07:52:28 阅读量: 12 订阅数: 19 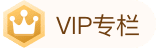
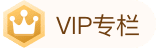
# 1. React 组件通信概述
## 1.1 React 组件之间为什么需要通信
React 应用程序通常由多个组件组成,而这些组件之间经常需要相互通信以实现协同工作和数据传递。理解组件通信的原因将有助于我们选择合适的通信方式,从而更好地组织和管理组件间的关系。
## 1.2 组件通信的类型与场景
在实际开发中,组件通信的方式多种多样,包括父子组件通信、兄弟组件通信以及跨层级组件通信等。每种通信方式都有其适用的场景,我们需要根据具体情况选择最合适的方式来进行组件之间的通信。
## 1.3 基于Props的单向数据流
React 的单向数据流使得组件之间的数据传递更加可控和易于维护。Props 作为组件间通信的主要方式之一,具有只读性,能够帮助我们实现数据的单向传递,从而更好地保持应用的可预测性和可维护性。
# 2. Props 和 State
Props 和 State 是 React 组件通信和状态管理的关键概念,理解和运用它们对于构建可维护和可扩展的 React 应用至关重要。
### 2.1 Props 和 State 的概念和区别
Props(属性)和 State(状态)是 React 组件的两个主要概念,它们都用于存储和管理组件的数据。
- Props:是由父组件传递给子组件的数据,是只读的,子组件不能直接修改 Props 的值。Props 可以包含任何类型的数据,包括基本数据类型、对象、函数等。父组件通过在子组件上指定属性并传递相应的值,来将数据传递给子组件。
- State:是组件内部管理的可变数据,可以通过 this.state 获取。与 Props 不同,组件可以通过 this.setState 方法来更新 State 的值,触发组件重新渲染。State 是组件的私有数据,只能在组件内部访问和修改。
Props 和 State 的区别在于:
- Props 是由父组件传递给子组件的,是只读的。
- State 是组件自身管理的内部状态,是可变的。
### 2.2 Props 的传递与接收
在 React 中,父组件可以通过在子组件上指定属性并传递相应的值,将数据传递给子组件。子组件可以通过 this.props 属性获取父组件传递的 Props。
下面是一个示例,展示了父组件向子组件传递 Props 的方法和子组件接收 Props 的方式:
```jsx
// 父组件
class ParentComponent extends React.Component {
render() {
const name = "Alice";
return (
<ChildComponent name={name} />
);
}
}
// 子组件
class ChildComponent extends React.Component {
render() {
const { name } = this.props;
return (
<p>Hello, {name}!</p>
);
}
}
```
在上述示例中,父组件通过在子组件上指定 name 属性并传递相应的值,将数据传递给子组件。子组件通过 this.props.name 获取父组件传递的 Props,并在组件的渲染中使用 Props 的值。
### 2.3 State 的管理与更新
在 React 组件中,可以通过构造函数的方式初始化组件的 State,然后通过 this.setState 方法来更新 State 的值。当 State 更新时,React 会自动触发组件的重新渲染,以反映更新后的 State。
下面是一个示例,展示了 State 的管理和更新的方法:
```jsx
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
}
incrementCount() {
this.setState({ count: this.state.count + 1 });
}
render() {
const { count } = this.state;
return (
<div>
<p>Count: {count}</p>
<button onClick={() => this.incrementCount()}>Increment</button>
</div>
);
}
}
```
在上述示例中,组件 MyComponent 初始化时设置 count 初始值为 0。通过调用 this.setState 方法并传入新的 State 值,可以更新组件的 State。在按钮的点击事件中,调用 incrementCount 方法来增加 count 值,并通过 this.setState 更新组件的 State。每次 State 更新后,React 会自动重新渲染组件,并在渲染中更新显示的 count 值。
通过 Props 和 State,我们可以实现 React 组件间的数据传递和状态管理。在后续章节中,我们将探讨更多高级的组件通信方式和解决方案。
# 3. 父子组件通信
在 React 应用中,父子组件通信是最常见的一种组件通信方式。父组件可以通过 Props 将数据传递给子组件,子组件也可以通过回调函数将数据传递回父组件。
#### 3.1 父组件向子组件传递 Props
父组件可以通过在 JSX 中的属性传递数据给子组件,子组件可以通过 `this.props` 访问父组件传递过来的数据。这种单向数据流的方式非常适合用于父子组件之间的简单通信。
```jsx
// ParentComponent.js
import React, { Component } from 'react';
import ChildComponent from './ChildComponent';
class ParentComponent extends Component {
render() {
return (
<div>
<ChildComponent name="Alice" />
</div>
);
}
}
export default ParentComponent;
```
```jsx
// ChildComponent.js
import React, { Component } from 'react';
class ChildComponent extends Component {
render() {
return (
<div>
<p>Hello, {this.props.name}!</p>
</div>
);
}
}
export default ChildComponent;
```
在这个例子中,`ParentComponent` 将 `name` 作为 Props 传递给 `ChildComponent`,`ChildComponent` 可以通过 `this.props.name` 访问并使用这个数据。
#### 3.2 子组件向父组件传递数据与回调函数
有时候子组件需要将数据传递给父组件,这时可以通过在子组件中定义一个回调函数,并将这个函数作为 Props 传递给子组件,子组件在合适的时机调用这个函数并传递数据。
```jsx
// ParentComponent.js
import React, { Component } from 'react';
import ChildComponent from './ChildComponent';
class ParentComponent extends Component {
handleDataFromChild = (data) => {
console.log("Data from child: " + data);
// 处理从子组件传递过来的数据
}
render() {
return (
<div>
<ChildComponent onDataReady={this.handleDataFromChild} />
</div>
);
}
}
export default ParentComponent;
```
```jsx
// ChildComponent.js
import React, { Component } from 'react';
class ChildComponent extends Component {
sendDataToParent = () => {
const data = "Hello, this is data from child!";
this.props.onDataReady(data);
}
render() {
return (
<div>
<
```
0
0
相关推荐
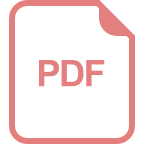





