揭秘图像处理算法原理:OpenCV图像处理背后的秘密
发布时间: 2024-08-13 23:36:12 阅读量: 22 订阅数: 25 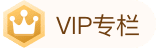
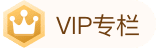
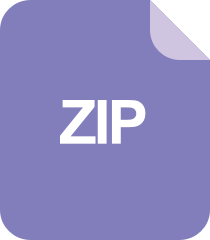
基于OPenCV实现图像处理各种常用算法.zip
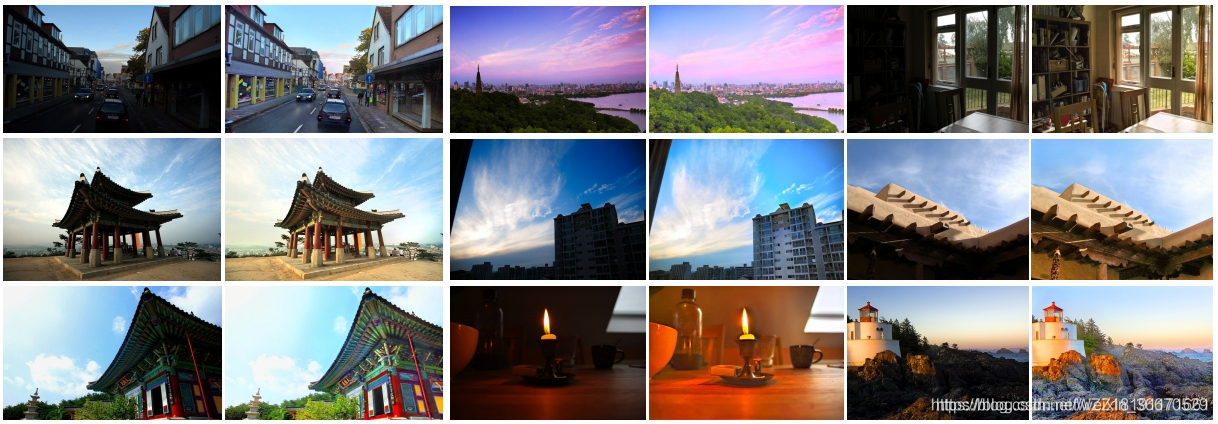
# 1. 图像处理基础**
图像处理是利用计算机对图像进行分析、处理和修改的技术。其基本原理是将图像数字化,并使用数学算法对其进行操作。图像处理广泛应用于计算机视觉、医学成像、遥感等领域。
图像处理涉及图像增强、图像分割、图像分析等多个方面。图像增强旨在改善图像的视觉效果,例如调整对比度和亮度。图像分割将图像分解为不同的区域,以提取感兴趣的对象。图像分析则用于从图像中提取特征和信息,为进一步的处理和决策提供依据。
# 2. OpenCV图像处理库简介
### 2.1 OpenCV的安装和配置
#### 2.1.1 Windows系统安装
1. 下载OpenCV安装包:前往OpenCV官方网站(https://opencv.org/)下载适用于Windows系统的安装包。
2. 运行安装程序:双击下载的安装包,按照提示完成安装。
3. 配置环境变量:在系统环境变量中添加OpenCV的bin目录,例如:C:\opencv\build\x64\vc15\bin。
#### 2.1.2 Linux系统安装
1. 依赖库安装:安装OpenCV所需的依赖库,例如:sudo apt-get install build-essential cmake git libgtk2.0-dev pkg-config libavcodec-dev libavformat-dev libswscale-dev
2. 下载OpenCV源码:使用git命令克隆OpenCV源码仓库:git clone https://github.com/opencv/opencv.git
3. 编译安装:进入OpenCV源码目录,执行以下命令进行编译安装:cmake -B build && make -j4 && sudo make install
### 2.2 OpenCV图像数据结构和操作
#### 2.2.1 图像数据结构
OpenCV中图像数据结构使用Mat类表示,Mat是一个多维数组,可以存储不同类型的数据,例如:
```cpp
Mat image = Mat::zeros(height, width, CV_8UC3);
```
* `height`:图像高度
* `width`:图像宽度
* `CV_8UC3`:图像数据类型,表示8位无符号3通道图像
#### 2.2.2 图像操作
OpenCV提供了丰富的图像操作函数,包括:
* **读取和显示图像:**
```cpp
Mat image = imread("image.jpg");
imshow("Image", image);
```
* **图像转换和格式转换:**
```cpp
Mat grayImage;
cvtColor(image, grayImage, COLOR_BGR2GRAY);
```
* **图像滤波和降噪:**
```cpp
Mat blurredImage;
GaussianBlur(image, blurredImage, Size(5, 5), 0);
```
# 3. 图像处理理论
图像处理理论是图像处理的基础,它为图像处理算法和技术提供了理论支持。图像处理理论包括图像增强、图像分割、图像特征提取和图像识别等内容。
### 3.1 图像增强
图像增强是通过对图像进行处理,提高图像的视觉效果和信息内容。图像增强技术有很多种,常用的包括直方图均衡化和图像锐化。
#### 3.1.1 直方图均衡化
直方图均衡化是一种图像增强技术,它通过调整图像的直方图,使图像的灰度分布更加均匀。直方图均衡化可以提高图像的对比度,增强图像的细节信息。
**代码块:**
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 计算直方图
hist = cv2.calcHist([image], [0], None, [256], [0, 256])
# 归一化直方图
hist = hist / np.sum(hist)
# 累积直方图
cdf = np.cumsum(hist)
# 映射图像灰度值
image_eq = np.interp(image, np.arange(256), cdf * 255).astype(np.uint8)
# 显示原图和增强后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Equalized Image', image_eq)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.calcHist` 函数计算图像的直方图。
* `np.cumsum` 函数计算直方图的累积分布函数 (CDF)。
* `np.interp` 函数使用 CDF 将图像的灰度值映射到新的灰度值。
* `astype(np.uint8)` 函数将映射后的灰度值转换为无符号 8 位整数。
#### 3.1.2 图像锐化
图像锐化是通过增强图像边缘来提高图像的清晰度。图像锐化技术有很多种,常用的包括拉普拉斯算子和 Sobel 算子。
**代码块:**
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg')
# 拉普拉斯算子锐化
laplacian = cv2.Laplacian(image, cv2.CV_64F)
laplacian = np.uint8(np.absolute(laplacian))
# Sobel 算子锐化
sobelx = cv2.Sobel(image, cv2.CV_64F, 1, 0, ksize=5)
sobely = cv2.Sobel(image, cv2.CV_64F, 0, 1, ksize=5)
sobel = np.hypot(sobelx, sobely)
sobel = np.uint8(sobel)
# 显示原图和锐化后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Laplacian Sharpened Image', laplacian)
cv2.imshow('Sobel Sharpened Image', sobel)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.Laplacian` 函数使用拉普拉斯算子对图像进行锐化。
* `cv2.Sobel` 函数使用 Sobel 算子对图像进行锐化。
* `np.hypot` 函数计算两个数组的平方和的平方根。
* `np.uint8` 函数将锐化后的图像转换为无符号 8 位整数。
# 4.1 图像读取和显示
### 4.1.1 图像读取
OpenCV提供了`cv2.imread()`函数读取图像文件。该函数接受两个参数:
- `filename`: 图像文件的路径
- `flags`: 指定图像读取方式的标志,可选值有:
- `cv2.IMREAD_COLOR`: 读取彩色图像(默认)
- `cv2.IMREAD_GRAYSCALE`: 读取灰度图像
- `cv2.IMREAD_UNCHANGED`: 读取图像而不进行任何转换
**代码块:**
```python
import cv2
# 读取彩色图像
image = cv2.imread('image.jpg')
# 读取灰度图像
gray_image = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
```
**逻辑分析:**
* `cv2.imread()`函数读取图像文件并返回一个NumPy数组,其中包含图像数据。
* `filename`参数指定图像文件的路径。
* `flags`参数指定图像读取方式。
### 4.1.2 图像显示
读取图像后,可以使用`cv2.imshow()`函数显示图像。该函数接受两个参数:
- `window_name`: 图像显示窗口的名称
- `image`: 要显示的图像
**代码块:**
```python
# 显示彩色图像
cv2.imshow('Color Image', image)
# 显示灰度图像
cv2.imshow('Gray Image', gray_image)
```
**逻辑分析:**
* `cv2.imshow()`函数创建一个窗口并显示图像。
* `window_name`参数指定窗口的名称。
* `image`参数指定要显示的图像。
* 窗口保持打开状态,直到用户按任意键。
### 4.1.3 等待用户输入
在显示图像后,可以使用`cv2.waitKey()`函数等待用户输入。该函数接受一个参数:
- `delay`: 等待用户输入的毫秒数(0表示无限等待)
**代码块:**
```python
# 等待用户按任意键
cv2.waitKey(0)
```
**逻辑分析:**
* `cv2.waitKey()`函数等待用户按任意键。
* `delay`参数指定等待时间(以毫秒为单位)。
* 如果`delay`为0,函数将无限期等待用户输入。
### 4.1.4 销毁窗口
用户按任意键后,可以使用`cv2.destroyAllWindows()`函数销毁所有打开的窗口。
**代码块:**
```python
# 销毁所有打开的窗口
cv2.destroyAllWindows()
```
**逻辑分析:**
* `cv2.destroyAllWindows()`函数销毁所有打开的窗口。
# 5. OpenCV图像处理进阶**
**5.1 图像特征提取**
图像特征提取是识别和分类图像的关键步骤。OpenCV提供了一系列强大的算法来提取图像中的特征。
**5.1.1 边缘检测**
边缘检测算法识别图像中像素之间的剧烈变化,从而突出物体和区域的边界。OpenCV提供多种边缘检测算法,包括:
- **Canny边缘检测:**一种广泛使用的算法,可检测图像中强边缘,同时抑制噪声。
- **Sobel边缘检测:**一种基于梯度的算法,可计算图像中像素的水平和垂直梯度。
- **Laplacian边缘检测:**一种二阶导数算法,可检测图像中像素的曲率变化。
**5.1.2 角点检测**
角点检测算法识别图像中像素的局部极值点,这些点通常对应于图像中的特征点。OpenCV提供多种角点检测算法,包括:
- **Harris角点检测:**一种基于局部自相关矩阵的算法,可检测图像中角点和边缘。
- **Shi-Tomasi角点检测:**一种基于最小特征值算法,可检测图像中稳定且明显的角点。
- **FAST角点检测:**一种快速且鲁棒的算法,可检测图像中圆形角点。
**5.2 图像识别和分类**
图像识别和分类是将图像分配到特定类别的过程。OpenCV提供了一系列算法来执行此任务。
**5.2.1 模板匹配**
模板匹配算法将图像与模板图像进行比较,以查找模板图像在图像中的位置。它通常用于检测已知对象的出现。
**5.2.2 机器学习分类**
机器学习分类算法使用训练数据来学习图像的特征,并将其分配到不同的类别。OpenCV支持多种机器学习算法,包括:
- **支持向量机(SVM):**一种二分类算法,可将数据点划分为不同的类。
- **决策树:**一种基于规则的算法,可通过一系列决策将数据点分配到不同的类。
- **神经网络:**一种受生物神经系统启发的算法,可学习图像中的复杂特征。
0
0
相关推荐
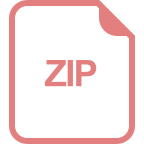
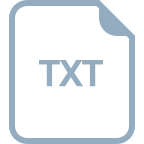





