C#中的数据访问技术: ADO.NET实践
发布时间: 2024-04-07 22:18:05 阅读量: 53 订阅数: 22 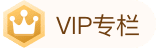
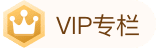
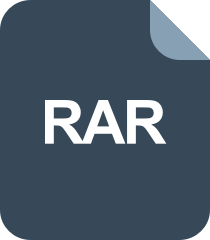
C#数据库访问技术ADO.net
# 1. 理解ADO.NET
- 1.1 什么是ADO.NET?
- 1.2 ADO.NET的优势和用途
# 2. 连接数据库
- 2.1 数据库连接串的配置
- 2.2 建立与数据库的连接
# 3. 执行SQL查询
#### 3.1 使用SqlCommand对象执行查询
在本章节中,我们将学习如何使用SqlCommand对象来执行SQL查询操作。SqlCommand是ADO.NET中与数据库交互的重要组件之一,通过它我们可以向数据库发送SQL查询语句并获取结果。
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
namespace DataAccessDemo
{
class Program
{
static void Main(string[] args)
{
string connStr = "Data Source=YourServer;Initial Catalog=YourDatabase;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connStr))
{
string query = "SELECT * FROM Customers";
SqlCommand command = new SqlCommand(query, connection);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
while (reader.Read())
{
Console.WriteLine($"Customer Name: {reader["Name"]}, Email: {reader["Email"]}");
}
}
reader.Close();
}
}
}
}
```
**代码总结**:以上代码演示了如何使用SqlCommand对象执行查询操作,并通过SqlDataReader读取返回的数据结果。
**结果说明**:执行以上代码将查询数据库表"Customers"并输出每个客户的姓名和邮箱信息。
#### 3.2 执行存储过程
另一个重要的SQL查询方式是执行存储过程,存储过程可以预先在数据库中编译和存储,提供了更好的性能和安全性。
```csharp
using System;
using System.Data;
using System.Data.SqlClient;
namespace DataAccessDemo
{
class Program
{
static void Main(string[] args)
{
string connStr = "Data Source=YourServer;Initial Catalog=YourDatabase;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connStr))
{
SqlCommand command = new SqlCommand("sp_GetAllCustomers", connection);
command.CommandType = CommandType.StoredProcedur
```
0
0
相关推荐

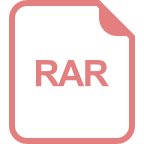
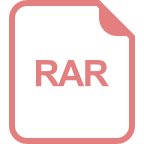
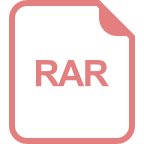
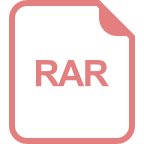
