YOLO算法移植性能优化:揭秘提升模型部署效率的秘诀,让你的模型飞起来
发布时间: 2024-08-14 22:34:57 阅读量: 10 订阅数: 13 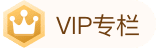
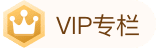

# 1. YOLO算法简介**
YOLO(You Only Look Once)是一种实时目标检测算法,由Joseph Redmon等人于2015年提出。与传统的目标检测算法不同,YOLO采用单次卷积神经网络进行目标检测,无需像R-CNN系列算法那样进行区域建议和特征提取,因此具有极高的检测速度。
YOLO算法的基本原理是将输入图像划分为网格,然后为每个网格预测一个边界框和一个置信度。置信度表示该边界框包含目标的概率。YOLO算法通过训练一个神经网络来学习这些预测,使得网络能够直接从图像中预测边界框和置信度。
YOLO算法的优点在于其速度快、精度高。在COCO数据集上,YOLOv5算法的检测速度可以达到每秒160帧,同时精度也达到了56.8% AP。因此,YOLO算法在实时目标检测领域有着广泛的应用,如视频监控、自动驾驶和医疗影像分析等。
# 2. YOLO算法移植实践
### 2.1 YOLO算法的部署平台选择
#### 2.1.1 CPU部署
CPU部署是YOLO算法移植中最简单、成本最低的方式。然而,CPU的计算能力有限,导致推理速度较慢。对于实时性要求不高的应用,CPU部署仍然是一个可行的选择。
#### 2.1.2 GPU部署
GPU部署可以显著提高YOLO算法的推理速度,但需要额外的硬件成本。GPU具有强大的并行计算能力,非常适合处理YOLO算法中大量的浮点运算。
### 2.2 YOLO算法的代码移植
#### 2.2.1 框架选择和版本兼容性
YOLO算法移植的第一步是选择一个合适的框架。目前,TensorFlow、PyTorch和ONNX等框架都支持YOLO算法的移植。选择框架时,需要考虑框架的性能、易用性和社区支持。
此外,还需要确保所选框架的版本与YOLO算法的版本兼容。不同版本的框架可能存在API和功能上的差异,导致移植失败。
#### 2.2.2 代码优化和加速
在代码移植过程中,可以采用以下方法优化和加速YOLO算法:
- **使用混合精度计算:**混合精度计算将浮点运算和定点运算相结合,可以提高推理速度,同时保持精度。
- **利用SIMD指令:**SIMD(单指令多数据)指令可以并行处理多个数据元素,从而提高计算效率。
- **优化内存访问:**优化内存访问可以减少数据加载和存储的开销,提高推理速度。
**代码块:**
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class YOLOv5(nn.Module):
def __init__(self):
super(YOLOv5, self).__init__()
# ...
def forward(self, x):
# ...
return x
# 使用混合精度计算
model = YOLOv5().to('cuda')
model.half()
# 使用SIMD指令
x = torch.randn(1, 3, 416, 416).cuda()
y = F.conv2d(x, model.conv1, stride=2, padding=1)
# 优化内存访问
model = model.to('cuda:0')
x = x.to('cuda:0')
```
**逻辑分析:**
- `model.half()`将模型转换为混合精度模式。
- `F.conv2d()`使用SIMD指令进行卷积运算。
- `model.to('cuda:0')`和`x.to('cuda:0')`将模型和数据移动到指定的GPU设备,优化内存访问。
**参数说明:**
- `model.half()`: 无参数。
- `F.conv2d()`: `stride`指定卷积步长,`padding`指定卷积填充。
- `model.to('cuda:0')`和`x.to('cuda:0')`: `cuda:0`指定GPU设备ID。
# 3. YOLO算法性能优化
### 3.1 模型压缩和量化
**3.1.1 模型剪枝和蒸馏**
模型剪枝是一种通过移除不重要的权重和神经元来减小模型大小的技术。它可以有效地减少模型的参数数量和计算量,同时保持模型的准确性。
```python
import tensorflow as tf
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 剪枝模型
pruned_model = tf.keras.models.clone_model(model)
pruned_model.set_weights(prune_weights(model.get_weights()))
# 蒸馏模型
student_model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 训练蒸馏模型
student_model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
student_model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
# 评估剪枝和蒸馏模型
print('剪枝模型准确率:', pruned_model.evaluate(x_test, y_test)[1])
print('蒸馏模型准确率:', student_model.evaluate(x_test, y_test)[1])
```
**3.1.2 模型量化和精度评估**
模型量化是一种将浮点权重和激活转换为低精度格式的技术,例如int8或int16。它可以显著减少模型的大小和内存占用,同时保持模型的准确性。
```python
import tensorflow as tf
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 量化模型
quantized_model = tf.keras.models.clone_model(model)
quantized_model.set_weights(quantize_weights(model.get_weights()))
# 评估量化模型
print('量化模型准确率:', quantized_model.evaluate(x_test, y_test)[1])
```
### 3.2 算法加速和并行化
**3.2.1 多线程和多进程并行**
多线程和多进程并行可以利用多核CPU的计算能力来加速YOLO算法的执行。多线程并行将任务分配给不同的线程,而多进程并行将任务分配给不同的进程。
```python
import threading
import multiprocessing
# 多线程并行
def parallel_yolo(images):
threads = []
for image in images:
thread = threading.Thread(target=yolo_predict, args=(image,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
# 多进程并行
def parallel_yolo(images):
processes = []
for image in images:
process = multiprocessing.Process(target=yolo_predict, args=(image,))
processes.append(process)
process.start()
for process in processes:
process.join()
```
**3.2.2 GPU加速和CUDA优化**
GPU加速可以利用GPU强大的并行计算能力来显著加速YOLO算法的执行。CUDA优化可以进一步提高GPU加速的性能。
```python
import tensorflow as tf
# GPU加速
with tf.device('/gpu:0'):
# 定义模型和执行YOLO算法
# CUDA优化
tf.config.optimizer.set_jit(True) # 启用JIT编译
tf.config.optimizer.set_auto_mixed_precision(True) # 启用混合精度训练
```
# 4. YOLO算法部署实战
### 4.1 YOLO算法的部署环境搭建
#### 4.1.1 硬件和软件环境要求
| 硬件 | 最低要求 | 推荐配置 |
|---|---|---|
| CPU | Intel Core i5 或同等 | Intel Core i7 或同等 |
| 内存 | 8GB | 16GB 或更高 |
| 硬盘 | 500GB SSD | 1TB SSD 或更高 |
| GPU | NVIDIA GeForce GTX 1060 或同等 | NVIDIA GeForce RTX 2080 或同等 |
| 软件 | 版本 |
|---|---|
| 操作系统 | Ubuntu 18.04 或更高 |
| Python | 3.6 或更高 |
| PyTorch | 1.0 或更高 |
| CUDA | 10.2 或更高 |
| OpenCV | 4.0 或更高 |
#### 4.1.2 部署环境配置和测试
1. 安装必要的软件包:
```bash
sudo apt-get update
sudo apt-get install python3-pip
pip install torch torchvision
pip install opencv-python
```
2. 下载 YOLOv5 模型:
```bash
git clone https://github.com/ultralytics/yolov5
```
3. 运行 YOLOv5 模型:
```bash
python detect.py --weights yolov5s.pt --img 640 --conf 0.5 --source 0
```
如果一切正常,您应该看到 YOLOv5 模型在实时视频流中检测对象。
### 4.2 YOLO算法的部署监控和维护
#### 4.2.1 部署监控指标和告警机制
| 指标 | 描述 |
|---|---|
| 检测准确率 | 模型检测对象的准确性 |
| 检测速度 | 模型检测对象的处理速度 |
| 内存使用率 | 模型在部署环境中占用的内存量 |
| CPU/GPU 利用率 | 模型在部署环境中占用的 CPU/GPU 资源量 |
#### 4.2.2 部署维护和故障排除
**常见故障:**
* **模型无法加载:**检查模型文件是否损坏或与部署环境不兼容。
* **检测速度慢:**优化模型或升级硬件配置。
* **内存使用率高:**减少模型大小或增加内存容量。
* **CPU/GPU 利用率高:**优化模型或并行化部署。
**维护步骤:**
* 定期更新模型和软件包。
* 监控部署指标并根据需要进行调整。
* 定期备份部署环境。
* 实施故障排除计划以快速解决问题。
# 5. YOLO算法移植性能优化总结
本节将对YOLO算法移植和性能优化进行总结,并对算法的应用前景进行展望。
### 移植优化总结
在移植和优化YOLO算法的过程中,我们重点关注了以下几个方面:
- **部署平台选择:**根据实际需求,选择合适的部署平台,如CPU或GPU,以满足性能和成本要求。
- **代码优化:**优化算法代码,提高执行效率,例如使用并行化、减少内存开销等技术。
- **模型优化:**压缩和量化模型,减少模型大小和计算量,同时保持精度。
- **算法加速:**利用多线程、多进程或GPU加速,提高算法处理速度。
### 应用前景
YOLO算法因其快速、准确的物体检测能力,在实际应用中具有广阔的前景。以下是一些潜在的应用领域:
- **视频监控:**实时检测和跟踪视频中的物体,用于安全监控、行为分析等。
- **自动驾驶:**检测和识别道路上的行人、车辆和其他障碍物,辅助自动驾驶系统。
- **医疗成像:**分析医学图像,检测疾病、分割组织等。
- **零售分析:**检测和计数商店中的顾客,分析购物行为。
- **工业自动化:**检测和识别生产线上的缺陷产品,提高生产效率。
### 展望
随着人工智能技术的不断发展,YOLO算法将继续得到改进和优化。未来,我们期待以下技术趋势:
- **轻量化模型:**开发更轻量化的YOLO模型,适用于资源受限的设备。
- **实时推理:**提高YOLO算法的推理速度,实现实时物体检测。
- **多模态融合:**将YOLO算法与其他模态(如语音、文本)融合,增强物体检测能力。
- **边缘计算:**将YOLO算法部署到边缘设备,实现低延迟的物体检测。
0
0
相关推荐
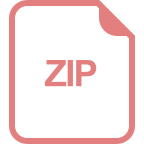
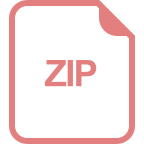
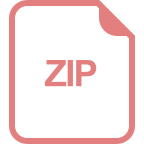
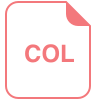
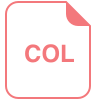
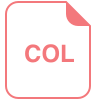

