多张图像合成的艺术:OpenCV图像融合技术,打造视觉盛宴
发布时间: 2024-08-07 18:11:41 阅读量: 24 订阅数: 13 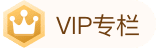
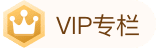
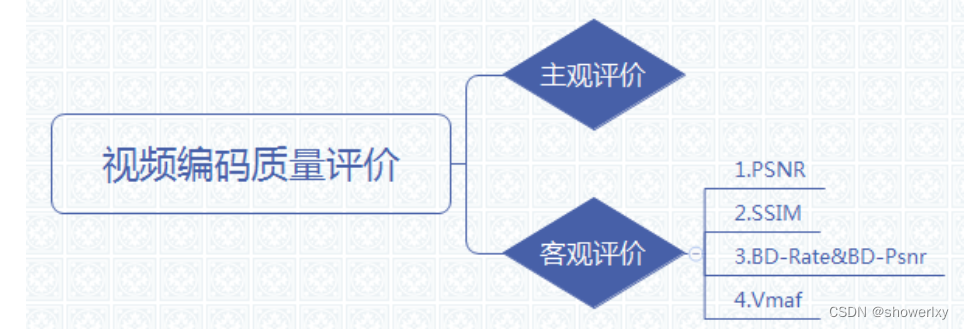
# 1. 图像融合概述**
图像融合是一种将来自不同来源的多个图像组合成单个图像的技术,以获得更完整、更准确的场景表示。它广泛应用于各种领域,包括计算机视觉、医学成像和遥感。
图像融合的类型包括:
- **像素级融合:**直接融合图像的像素值,例如加权平均法。
- **特征级融合:**提取图像的特征,然后融合这些特征,例如图像金字塔法。
- **决策级融合:**将每个图像的决策结果融合,例如多尺度分解法。
# 2. OpenCV图像融合技术
### 2.1 OpenCV图像融合基础
#### 2.1.1 图像融合的概念和类型
图像融合是指将两幅或多幅图像组合成一幅新图像,新图像保留了原始图像中互补的信息。图像融合技术广泛应用于计算机视觉、医学成像和遥感等领域。
图像融合的类型主要包括:
- **像素级融合:**将原始图像中的像素直接组合,生成融合图像。
- **特征级融合:**提取原始图像中的特征,如边缘、纹理和颜色,然后组合特征生成融合图像。
- **决策级融合:**对原始图像进行分析和决策,然后生成融合图像。
#### 2.1.2 OpenCV中的图像融合函数
OpenCV提供了丰富的图像融合函数,涵盖了像素级、特征级和决策级融合算法。常用的函数包括:
- `cv2.addWeighted()`:加权平均法,对原始图像进行线性加权组合。
- `cv2.pyrDown()` 和 `cv2.pyrUp()`:图像金字塔法,通过图像金字塔进行融合。
- `cv2.detailEnhance()`:多尺度分解法,通过多尺度分解和重构进行融合。
### 2.2 融合算法
#### 2.2.1 加权平均法
加权平均法是最简单的图像融合算法,通过对原始图像进行线性加权组合生成融合图像。权重值代表了原始图像在融合图像中的重要性。
```python
import cv2
# 读取两幅原始图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 设置权重值
alpha = 0.5
# 加权平均融合
fused_img = cv2.addWeighted(img1, alpha, img2, 1-alpha, 0)
```
**逻辑分析:**
- `cv2.addWeighted()`函数接收四个参数:原始图像1、权重1、原始图像2、权重2、伽马校正值。
- `alpha`代表原始图像1的权重,`1-alpha`代表原始图像2的权重。
- 融合图像`fused_img`是原始图像1和原始图像2的加权平均值。
#### 2.2.2 图像金字塔法
图像金字塔法通过构建图像金字塔进行融合。图像金字塔是一组不同尺度的图像,其中每一层图像都是上一层图像的缩小版本。
```python
import cv2
# 读取两幅原始图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 构建图像金字塔
pyramid1 = [img1]
pyramid2 = [img2]
for i in range(1, 5):
pyramid1.append(cv2.pyrDown(pyramid1[i-1]))
pyramid2.append(cv2.pyrDown(pyramid2[i-1]))
# 融合图像金字塔
fused_pyramid = []
for i in range(5):
fused_pyramid.append(cv2.addWeighted(pyramid1[i], 0.5, pyramid2[i], 0.5, 0))
# 重构融合图像
fused_img = fused_pyramid[0]
for i in range(1, 5):
fused_img = cv2.pyrUp(fused_img) + fused_pyramid[i]
```
**逻辑分析:**
- `cv2.pyrDown()`函数将图像缩小一半,生成图像金字塔。
- `cv2.pyrUp()`函数将图像放大一半,重构融合图像。
- 融合图像金字塔`fused_pyramid`是原始图像金字塔1和原始图像金字塔2的加权平均值。
- 融合图像`fused_img`是融合图像金字塔的重构结果。
#### 2.2.3 多尺度分解法
多尺度分解法通过对原始图像进行多尺度分解和重构进行融合。多尺度分解将图像分解成不同尺度的子带,重构将子带组合成融合图像。
```python
import cv2
# 读取两幅原始图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 多尺度分解
decomposed1 = cv2.detailEnhance(img1, sigma_s=10, sigma_r=0.15)
decomposed2 = cv2.detailEnhance(img2, sigma_s=10, sigma_r=0.15)
# 融合子带
fused_decomposed = []
for i in range(3):
fused_decomposed.append(cv2.addWeighted(decomposed1[i], 0.5, decomposed2[i], 0.5, 0))
# 重构融合图像
fused_img = cv2.detailEnhance(fused_decomposed, sigma_s=10, sigma_r=0.15)
```
**逻辑分析:**
- `cv2.detailEnhance()`函数进行多尺度分解,生成三组子带。
- 融合子带`fused_decomposed`是原始图像子带1和原始图像子带2的加权平均值。
- 融合图像`fused_img`是融合子带的重构结果。
# 3. 图像融合实践
### 3.1 图像读取和预处理
#### 3.1.1 图像读取和转换
图像融合的第一步是读取需要融合的图像。OpenCV提供了多种函数来读取图像,例如`imread()`和`imdecode()`。`imread()`函数从文件路径读取图像,而`imdecode()`函数从内存缓冲区读取图像。
```python
```
0
0
相关推荐
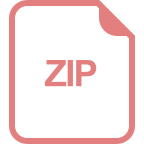
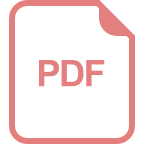
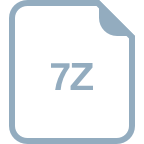





