掌握Spring Security的基本配置与使用
发布时间: 2024-01-08 21:19:09 阅读量: 59 订阅数: 45 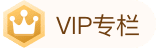
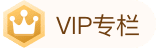
# 1. 理解Spring Security
## 1.1 什么是Spring Security
Spring Security是一个功能强大且灵活的框架,用于在Spring应用程序中实现身份验证和授权。它提供了一套全面的安全性解决方案,可帮助我们保护我们的应用程序免受各种安全威胁。
## 1.2 Spring Security的作用和优势
Spring Security可以用于以下几方面:
- 身份验证:验证用户的凭据(如用户名和密码)以允许或拒绝访问应用程序的特定部分。
- 授权:通过分配给用户角色和权限来控制他们可以访问的资源。
- 安全性保护:保护应用程序免受威胁,如跨站点脚本(XSS)、令牌伪造攻击(CSRF)、会话劫持等。
- 单点登录(SSO):允许用户使用一组凭据访问多个关联的应用程序。
Spring Security的优势包括:
- 简化安全性实现:Spring Security提供了许多内置的功能和工具,可以轻松实现各种安全性需求。
- 细粒度的控制:可以根据角色和权限来定义访问控制,实现更细粒度的安全性。
- 可扩展性:Spring Security具有高度可扩展性,可以根据需要定制和扩展各种功能。
- 社区支持:Spring Security由一个庞大的社区提供支持,可以获得高质量的文档、教程和解决方案。
## 1.3 Spring Security的基本原理
在Spring Security中,身份验证和授权是基于一套定义良好的核心原则和概念完成的。主要包括以下几个要点:
- 用户认证:通过验证用户的身份来确认其真实性。可以使用各种方式进行用户认证,如基于内存的认证、基于数据库的认证、第三方身份认证等。
- 授权管理:确定哪些用户有权限访问应用程序的特定资源。可以基于角色或权限来定义授权策略。
- 过滤器链:Spring Security通过一系列的过滤器来拦截用户请求,并依次进行身份验证和授权处理。
- 安全上下文:用于在整个应用程序中存储和传递当前用户的安全相关信息。
理解这些基本原理将有助于我们更好地配置和使用Spring Security来保护我们的应用程序。
在接下来的章节中,我们将深入探讨Spring Security的基本配置和使用。请继续阅读第二章节以了解如何配置Spring Security的基本设置。
# 2. Spring Security基本配置
在本章中,我们将介绍如何配置Spring Security的基本设置,并定义用户认证和授权信息。
#### 2.1 添加Spring Security依赖
首先,我们需要在项目中添加Spring Security的依赖。可以在项目的构建配置文件(如pom.xml)中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
#### 2.2 配置Spring Security的基本设置
接下来,我们需要配置Spring Security的基本设置。可以创建一个继承自WebSecurityConfigurerAdapter的配置类,并重写configure方法,如下所示:
```java
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
public void configure(WebSecurity web) throws Exception {
web.ignoring().antMatchers("/resources/**");
}
}
```
以上配置中,我们定义了以下内容:
- `/public/**`路径下的请求允许所有用户访问。
- 其他路径的请求需要经过认证后才能访问。
- 使用自定义的登录页面`/login`。
- 允许所有用户注销。
另外,通过重写`configure(WebSecurity web)`方法,我们可以配置哪些静态资源不需要进行安全验证。
#### 2.3 定义用户认证和授权信息
接下来,我们需要定义用户认证和授权信息。可以创建一个实现UserDetailsService接口的类,并重写loadUserByUsername方法,如下所示:
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 根据用户名从数据库或其他数据源中获取用户信息
// ...
// 返回Spring Security提供的UserDetails实现类
return User.builder()
.username(username)
.password("password") // replace with hashed password
.roles("USER")
.build();
}
}
```
以上代码中,我们通过实现UserDetailsService接口并重写loadUserByUsername方法,可以根据用户名从数据库或其他数据源中获取用户信息,并返回Spring Security提供的UserDetails实现类。在示例中,我们使用了User.builder()方法创建一个UserDetails对象,并设置了用户名、密码(需替换为哈希密码)和角色。
这样,我们就完成了Spring Security的基本配置和用户认证信息的定义。
在下一章节中,我们将详细介绍用户认证的几种方式:基于内存的用户认证、基于数据库的用户认证和自定义用户认证。敬请期待!
**代码总结:**
在本章中,我们介绍了如何配置Spring Security的基本设置,并定义了用户认证和授权信息。我们通过添加依赖、配置基本设置和定义UserDetailsService来完成这些操作。
**结果说明:**
通过以上的配置和定义,我们可以实现基本的用户认证和授权功能,保护我们的应用程序免受未授权访问。在下一章节中,我们将进一步探讨用户认证的几种方式,以及如何自定义认证逻辑。
# 3. 用户认证
### 3.1 基于内存的用户认证
在Spring Security中,我们可以通过不同的方式来进行用户认证。其中一种简单的方式是将用户信息存储在内存中,并使用基于内存的用户认证机制。
首先,我们需要在Spring Security配置文件中添加如下代码来启用基于内存的用户认证:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}admin123").roles("ADMIN")
.and()
.withUser("user").password("{noop}user123").roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
```
上述代码中,我们通过`auth.inMemoryAuthentication()`创建了一个基于内存的认证机制。然后,我们使用`withUser`方法指定了两个用户,分别为admin和user,以及它们的密码和角色。`{noop}`表示密码的加密方式为空,也就是不进行密码加密。
接下来,我们在`configure(HttpSecurity http)`方法中配置了访问权限。对于以`/admin/`开头的URL,需要拥有ADMIN角色才能访问;而以`/user/`开头的URL,需要拥有ADMIN或USER角色才能访问。其他未匹配的URL则需要进行认证。
最后,我们还配置了表单登录和注销功能。通过`formLogin().permitAll()`指定了登录页面可以被所有人访问,而`logout().permitAll()`表示注销功能也可以被所有人访问。
### 3.2 基于数据库的用户认证
除了基于内存的用户认证,我们还可以使用基于数据库的用户认证。下面是一个使用JDBC和MySQL的例子。
首先,我们需要添加相关依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
接下来,我们需要配置数据库连接信息和JPA实体类。假设我们有一个名为"users"的表用于存储用户信息,表结构如下:
```sql
CREATE TABLE users (
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(255) UNIQUE,
password VARCHAR(255),
enabled BOOLEAN
);
```
然后,我们创建一个用户实体类`User`,并使用JPA注解将它映射到数据库中的"users"表:
```java
@Entity
@Table(name = "users")
public class User implements UserDetails {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(unique = true)
private String username;
private String password;
private boolean enabled;
// ... 省略其他属性和方法
}
```
接下来,我们需要创建一个继承自`UserDetailsService`的类`UserDetailsServiceImpl`,并实现其中的方法。该类负责从数据库中加载用户信息:
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
private final UserRepository userRepository;
public UserDetailsServiceImpl(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Override
@Transactional(readOnly = true)
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return user;
}
}
```
在上述代码中,我们使用`UserRepository`来查询数据库并返回一个`UserDetails`对象。
最后,在Spring Security配置文件中添加如下代码:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private final UserDetailsService userDetailsService;
public SecurityConfig(UserDetailsService userDetailsService) {
this.userDetailsService = userDetailsService;
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
```
在上述代码中,我们注入了`UserDetailsService`并通过`auth.userDetailsService(userDetailsService)`将其设置为认证管理器中使用的服务。
### 3.3 自定义用户认证
有时候我们需要使用自定义的用户认证逻辑,而不仅仅是从内存或数据库中读取用户信息。对于这种情况,我们可以创建一个实现了`AuthenticationProvider`接口的类来实现自定义的用户认证逻辑。
下面是一个简单示例:
```java
@Component
public class CustomAuthenticationProvider implements AuthenticationProvider {
@Override
public Authentication authenticate(Authentication authentication) throws AuthenticationException {
String username = authentication.getName();
String password = authentication.getCredentials().toString();
// 验证用户名和密码的逻辑
if (username.equals("admin") && password.equals("admin123")) {
List<GrantedAuthority> authorities = new ArrayList<>();
authorities.add(new SimpleGrantedAuthority("ROLE_ADMIN"));
return new UsernamePasswordAuthenticationToken(username, password, authorities);
} else {
throw new BadCredentialsException("Authentication failed");
}
}
@Override
public boolean supports(Class<?> authentication) {
return authentication.equals(UsernamePasswordAuthenticationToken.class);
}
}
```
在上述代码中,我们首先从`Authentication`对象中获取用户名和密码,然后根据自定义的逻辑进行认证。如果认证通过,我们创建一个`UsernamePasswordAuthenticationToken`对象来表示已认证的用户,并设置角色为"ROLE_ADMIN";否则,我们抛出`BadCredentialsException`表示认证失败。
最后,在Spring Security配置文件中进行如下配置:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
private final CustomAuthenticationProvider authenticationProvider;
public SecurityConfig(CustomAuthenticationProvider authenticationProvider) {
this.authenticationProvider = authenticationProvider;
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.authenticationProvider(authenticationProvider);
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin().permitAll()
.and()
.logout().permitAll();
}
}
```
在上述代码中,我们注入了`CustomAuthenticationProvider`并通过`auth.authenticationProvider(authenticationProvider)`将其配置为认证管理器中使用的提供者。
# 4. 用户授权
在本章中,我们将深入探讨Spring Security中的用户授权相关内容。用户授权是指确定用户是否有权限执行特定操作的过程,包括基于角色的授权管理、基于权限的授权管理以及自定义访问控制。
#### 4.1 基于角色的授权管理
基于角色的授权是一种常见的授权管理方式,通过为用户分配特定的角色,来控制其在系统中的操作权限。
##### 场景:
假设我们有一个在线商城系统,分为普通用户和管理员两种角色,普通用户具有查看商品和下单的权限,而管理员具有商品管理和订单管理的权限。
##### 代码示例:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/products").hasAnyRole("USER", "ADMIN")
.antMatchers("/orders").hasAnyRole("ADMIN")
.and()
.formLogin();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("USER", "ADMIN");
}
}
```
##### 代码解释:
- `configure(HttpSecurity http)`方法配置了访问权限规则,使用`hasAnyRole()`方法指定不同URL需要的角色权限。
- `configure(AuthenticationManagerBuilder auth)`方法配置了基于内存的用户认证信息,分别创建了用户"user"和"admin",并分配了对应的角色。
##### 代码总结:
通过以上配置,实现了基于角色的授权管理,普通用户和管理员在访问不同URL时将根据其角色权限进行控制。
##### 结果说明:
- 用户访问`/products`页面时需要具有"USER"或"ADMIN"角色。
- 用户访问`/orders`页面时需要具有"ADMIN"角色。
#### 4.2 基于权限的授权管理
除了基于角色的授权管理外,Spring Security还支持基于权限的细粒度控制,允许对用户进行更加精细化的授权管理。
##### 场景:
在之前的在线商城系统中,除了角色管理外,我们还希望对某些特定操作进行权限控制,比如只有管理员可以添加商品或管理订单。
##### 代码示例:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/products/add").hasAuthority("ADD_PRODUCT")
.antMatchers("/orders/manage").hasAuthority("MANAGE_ORDER")
.and()
.formLogin();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}password").authorities("ADD_PRODUCT", "MANAGE_ORDER");
}
}
```
##### 代码解释:
- `configure(HttpSecurity http)`方法中使用`hasAuthority()`方法指定URL需要具备的权限。
- `configure(AuthenticationManagerBuilder auth)`方法中创建了用户"admin",并分配了"ADD_PRODUCT"和"MANAGE_ORDER"两个权限。
##### 代码总结:
上述配置实现了基于权限的授权管理,只有具备对应权限的用户才能访问特定URL。
##### 结果说明:
- 用户访问`/products/add`页面时需要具备"ADD_PRODUCT"权限。
- 用户访问`/orders/manage`页面时需要具备"MANAGE_ORDER"权限。
#### 4.3 自定义访问控制
除了基于角色和权限的授权管理外,Spring Security还支持自定义访问控制,允许开发人员根据具体的业务需求对用户的访问进行更加个性化的控制。
##### 场景:
在在线商城系统中,我们希望对VIP用户开放特定的折扣商品页面,普通用户无法访问。
##### 代码示例:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/products/discount").access("hasRole('VIP') and isAuthenticated()")
.and()
.formLogin();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("vipuser").password("{noop}password").roles("VIP")
.and()
.withUser("normaluser").password("{noop}password").roles("USER");
}
}
```
##### 代码解释:
- `configure(HttpSecurity http)`方法中使用`access()`方法自定义访问表达式,要求用户具备"VIP"角色并已认证。
- `configure(AuthenticationManagerBuilder auth)`方法中创建了"vipuser"和"normaluser"两个用户,分别具备不同的角色权限。
##### 代码总结:
以上配置实现了自定义访问控制,只有具备"VIP"角色且已认证的用户才能访问折扣商品页面。
##### 结果说明:
- 用户访问`/products/discount`页面时需要具备"VIP"角色并已认证。
本章内容介绍了Spring Security中用户授权的基本配置和使用,包括基于角色的授权管理、基于权限的授权管理以及自定义访问控制,希朿能够对读者有所帮助。
# 5. Spring Security与Web应用集成
#### 5.1 配置Spring Security与Spring MVC集成
在Spring Security中,与Spring MVC集成是非常常见的需求,通过配置可以实现对URL的访问控制和权限管理。
##### 场景
假设我们有一个基于Spring MVC的Web应用,我们希望通过Spring Security来管理用户的登录和权限控制。
##### 代码示例
首先,添加Spring Security和Spring MVC的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
然后,配置Spring Security:
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
}
```
接着,配置Spring MVC,让它与Spring Security集成:
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.web.servlet.config.annotation.ViewControllerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class MvcConfig implements WebMvcConfigurer {
@Override
public void addViewControllers(ViewControllerRegistry registry) {
registry.addViewController("/login").setViewName("login");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
##### 代码总结
1. 通过@EnableWebSecurity注解和WebSecurityConfigurerAdapter的配置,实现对URL的访问控制和权限管理。
2. 通过addViewControllers方法,指定登录页面的URL与实际视图的映射关系。
3. 通过PasswordEncoder,对用户密码进行加密和验证。
##### 结果说明
经过以上配置,我们实现了Spring Security与Spring MVC的集成,可以对不同URL进行权限控制,并且实现了自定义的登录页面。
#### 5.2 自定义登录页面和错误处理
在实际应用中,我们可能需要自定义登录页面和错误处理,以提升用户体验和安全性。
##### 场景
假设我们需要自定义登录页面,并且在登录失败时显示自定义的错误信息。
##### 代码示例
首先,创建自定义的登录页面login.html:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h2>Login</h2>
<form action="/login" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username"/>
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password"/>
</div>
<button type="submit">Login</button>
</form>
</body>
</html>
```
然后,配置自定义的登录页面和错误处理:
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/custom-login")
.permitAll()
.failureUrl("/custom-login?error=true")
.and()
.logout()
.permitAll();
}
}
```
##### 代码总结
1. 创建自定义的登录页面login.html,并通过配置指定登录页面的URL。
2. 在配置中指定登录失败时显示自定义的错误信息。
##### 结果说明
通过以上配置,我们实现了自定义的登录页面和错误处理,提升了用户体验和安全性。
#### 5.3 CSRF保护和Session管理
在实际应用中,CSRF(Cross-Site Request Forgery)保护和Session管理是非常重要的安全考虑因素。
##### 场景
我们需要对Web应用进行CSRF保护,并且对Session进行有效管理。
##### 代码示例
首先,配置CSRF保护和Session管理:
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf().disable() // 禁用CSRF保护
.authorizeRequests()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.sessionManagement()
.invalidSessionUrl("/session-expired")
.maximumSessions(1)
.maxSessionsPreventsLogin(true);
}
}
```
然后,配置Session过期处理:
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.web.session.HttpSessionEventPublisher;
@Configuration
public class SessionConfig {
@Bean
public HttpSessionEventPublisher httpSessionEventPublisher() {
return new HttpSessionEventPublisher();
}
}
```
##### 代码总结
1. 通过禁用CSRF保护,实现对跨站请求伪造的保护。
2. 通过sessionManagement配置,对Session进行有效管理,并指定Session过期处理的URL。
##### 结果说明
通过以上配置,我们实现了对Web应用的CSRF保护和Session管理,提升了安全性和用户体验。
以上是第五章关于Spring Security与Web应用集成的内容,涵盖了与Spring MVC的集成、自定义登录页面和错误处理、以及CSRF保护和Session管理。
# 6. 高级主题和使用技巧
在本章中,我们将介绍一些Spring Security的高级主题和使用技巧,包括单点登录、OAuth2.0集成以及保护RESTful API的方法。
#### 6.1 使用Spring Security实现单点登录
单点登录(Single Sign-On, SSO)是一种允许用户使用单组凭据(例如用户名和密码)访问多个相关但独立的软件系统的技术,我们可以使用Spring Security来实现单点登录,简化用户的认证过程。
##### 场景
假设我们有多个子系统,例如电子商城、论坛和博客系统,用户只需要登录一次,即可访问所有子系统而无需重新认证。
##### 代码示例
```java
// 在Spring Security配置中添加单点登录的配置
@Override
protected void configure(HttpSecurity http) throws Exception {
http
// ... 其他配置
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/dashboard")
.permitAll()
.and()
.logout()
.permitAll()
.and()
.csrf().disable()
.and()
.addFilterBefore(ssoFilter(), BasicAuthenticationFilter.class);
}
@Bean
public FilterRegistrationBean ssoFilter() {
// 配置单点登录过滤器
// ...
}
```
##### 代码总结
上述代码通过添加单点登录的过滤器来实现SSO,同时配置了其他的Spring Security基本设置。
##### 结果说明
用户在任意一个子系统登录后,即可访问其他子系统而无需重新登录,实现了单点登录的效果。
#### 6.2 集成OAuth2.0
OAuth2.0是一种用于授权的开放标准,允许用户授权第三方应用访问他们存储在授权服务器上的信息,Spring Security提供了与OAuth2.0的集成支持。
##### 场景
假设我们需要让用户使用他们的社交媒体账号(如Google、Facebook或GitHub)登录我们的应用,我们可以使用OAuth2.0来实现这一功能。
##### 代码示例
```java
// 配置OAuth2登录
@EnableOAuth2Sso
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/login**", "/callback/", "/webjars/**")
.permitAll()
.anyRequest()
.authenticated();
}
}
```
##### 代码总结
通过@EnableOAuth2Sso注解来启用OAuth2.0单点登录功能,配置了允许匿名访问的URL,以及需要认证的URL。
##### 结果说明
用户可以使用他们的第三方账号直接登录我们的应用,无需注册新的账号,简化了用户的注册和登录流程。
#### 6.3 保护RESTful API
在实际开发中,我们通常需要保护RESTful API,以确保只有经过授权的用户可以访问特定的API端点,Spring Security可以很好地支持这一功能。
##### 场景
假设我们有一些敏感的数据需要通过RESTful API提供给移动端或前端应用,我们希望只有经过认证和授权的用户才能访问这些API。
##### 代码示例
```java
// 配置Spring Security保护RESTful API
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/**").authenticated()
.anyRequest().permitAll()
.and()
.httpBasic();
}
```
##### 代码总结
通过配置antMatchers来指定需要认证的API端点,同时配置其他API端点为允许匿名访问。
##### 结果说明
只有经过认证的用户才能访问被保护的RESTful API,确保了API的安全性和数据的隐私。
以上就是关于Spring Security的高级主题和使用技巧的内容,包括单点登录、OAuth2.0集成以及保护RESTful API的方法。希望本章内容能帮助你更深入地理解和应用Spring Security。
0
0
相关推荐
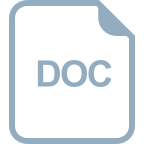
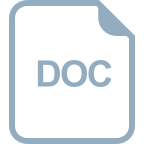
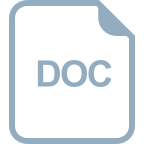
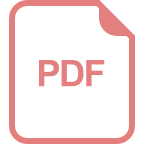
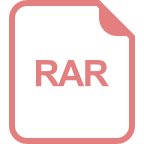
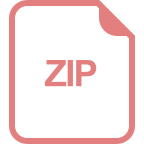
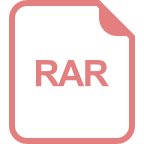
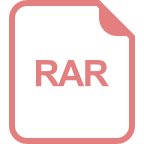
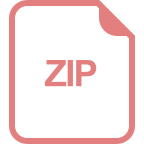