TypeScript中的装饰器与元数据
发布时间: 2024-02-22 04:37:54 阅读量: 10 订阅数: 19 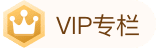
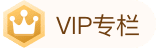
# 1. 介绍装饰器和元数据
## 1.1 什么是装饰器
装饰器是一种特殊的声明,它可以附加到类声明、方法、访问符、属性或参数上,可以用来修改类的行为。在面向对象的编程中,装饰器模式是一种结构型设计模式,它以对对象的功能进行动态添加的方式来扩展功能。
## 1.2 装饰器在TypeScript中的应用
在TypeScript中,装饰器是一种实验性功能,在ES6中引入了装饰器的语法,通过装饰器能够很好地实现AOP(面向切面编程),方便扩展和维护代码。
## 1.3 元数据的概念和作用
元数据是描述数据的数据,通过元数据可以更好地理解和操作数据。在TypeScript中,装饰器与元数据结合使用,可以实现更加灵活的功能扩展和数据处理。
# 2. TypeScript中装饰器的基本语法
装饰器是一种特殊类型的声明,它可以被附加到类声明、方法、访问符、属性或参数上,来修改类的行为。在TypeScript中,装饰器以`@decorator`的形式使用,可以根据需要传入参数。接下来将介绍装饰器的基本语法。
### 2.1 装饰器的定义和使用
装饰器是一个表达式,当装饰对象被声明时会被调用。装饰器函数的第一个参数是装饰的目标对象,第二个参数是装饰的属性名(可选)。下面是一个简单的类装饰器示例:
```typescript
function ClassDecorator(target: any) {
console.log("Class decorator invoked on", target);
}
@ClassDecorator
class ExampleClass {
// Class properties and methods
}
```
### 2.2 装饰器工厂
装饰器工厂是一个返回装饰器的函数,可以对装饰器进行参数化处理。以下是一个装饰器工厂的示例:
```typescript
function Logger(prefix: string) {
return function(target: any) {
console.log(`${prefix} - ${target}`);
}
}
@Logger("DEBUG")
class ExampleClass {
// Class properties and methods
}
```
### 2.3 装饰器组合
多个装饰器可以同时应用于同一个声明。当多个装饰器应用于一个声明时,它们的求值方式与复合函数相似。在TypeScript中,从上往下依次对装饰器表达式求值,然后将结果应用于声明。
```typescript
function Log(target: any) {
console.log("Log decorator invoked on", target);
}
function Measure(target: any) {
console.log("Measure decorator invoked on", target);
}
@Log
@Measure
class ExampleClass {
// Class properties and methods
}
```
以上是TypeScript中装饰器的基本语法及用法,通过灵活使用装饰器可以实现更多功能和增强类的行为。
# 3. 装饰器的应用场景
装饰器在TypeScript中有多种应用场景,包括类装饰器、方法装饰器、属性装饰器和参数装饰器。下面分别介绍它们的具体用法:
#### 3.1 类装饰器
类装饰器是应用于类构造函数的装饰器,可以用来添加类的元数据或静态成员。一个类装饰器的基本结构如下:
```typescript
function classDecorator<T extends {new(...args:any[]):{}}>(constructor:T) {
return class extends constructor {
newProperty = "new property";
hello = "override";
}
}
@classDecorator
class Greeter {
property = "property";
hello: string;
constructor(m: string) {
this.hello = m;
}
}
console.log(new Greeter("world"));
```
在上面的示例中,类装饰器`@classDecorator`通过添加新属性`newProperty`和修改`hello`值来装饰类`Greeter`。当实例化`Greeter`类时,输出的结果为`Greeter { property: 'property', hello: 'override', newProperty: 'new property' }`。
#### 3.2 方法装饰器
方法装饰器可以用来装饰类的方法,在方法执行前后进行一些操作。一个方法装饰器的基本结构如下:
```typescript
function methodDecorator(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
console.log("Method Decorator called on: ", target, propertyKey, descriptor);
}
class Greeter {
@methodDecorator
greet() {
return "Hello!";
}
}
new Greeter().greet();
```
上面的代码演示了如何使用方法装饰器`@methodDecorator`来在实例方法`greet`被调用时输出调用信息。
#### 3.3 属性装饰器
属性装饰器可以用来装饰类的属性,在属性定义时进行一些操作。一个属性装饰器的基本结构如下:
```typescript
function propertyDecorator(target: any, propertyKey: string) {
```
0
0
相关推荐
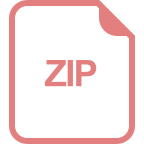
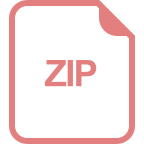
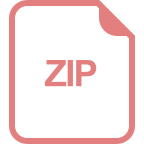





