单片机汇编语言实时操作系统:管理并发和时间敏感任务
发布时间: 2024-07-07 08:37:50 阅读量: 57 订阅数: 30 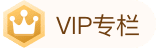
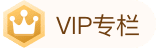
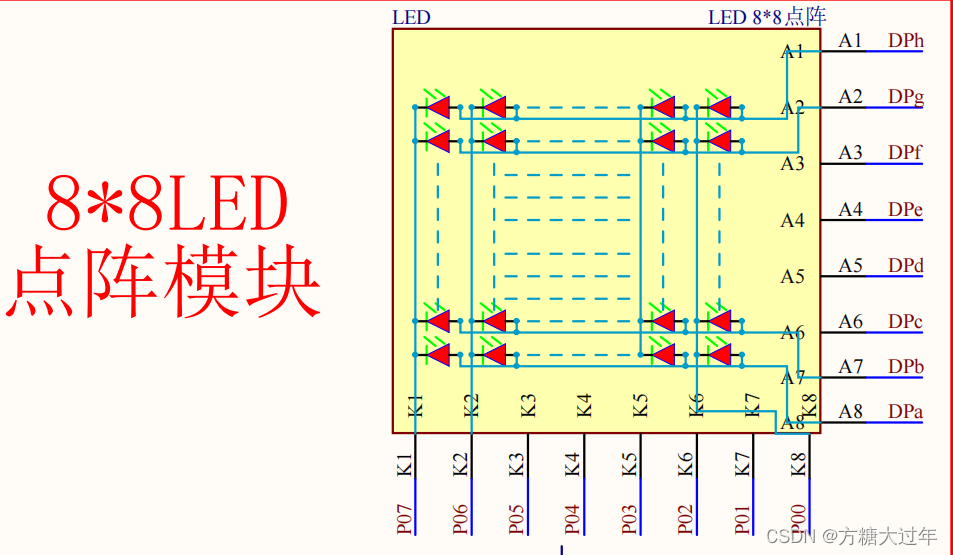
# 1. 单片机汇编语言基础**
汇编语言是一种低级编程语言,它直接与计算机的机器指令相对应。单片机汇编语言是专门为单片机设计的汇编语言,它具有以下特点:
- **紧凑性:**汇编语言代码体积小,执行效率高。
- **可移植性:**汇编语言代码可以移植到不同的单片机平台上。
- **实时性:**汇编语言可以实现精确的定时和中断处理,满足实时系统的要求。
汇编语言程序由以下部分组成:
- **指令:**执行特定操作的机器指令。
- **操作数:**指令操作的对象。
- **伪指令:**用于控制程序编译和链接过程的指令。
# 2. 实时操作系统的概念和原理
### 2.1 实时操作系统的特点和分类
实时操作系统(RTOS)是一种专门设计用于控制实时系统的操作系统,其特点如下:
- **实时性:**RTOS 能够在可预测的时间内对事件做出响应,确保系统在指定的时间限制内完成任务。
- **并发性:**RTOS 支持多个任务同时运行,允许系统处理来自不同来源的事件。
- **确定性:**RTOS 的行为是可预测的,可以保证任务在规定的时间内执行。
- **容错性:**RTOS 具有故障处理机制,可以防止系统崩溃或数据丢失。
根据不同的分类标准,RTOS 可以分为以下类型:
- **硬实时操作系统:**对时间要求非常严格,要求所有任务在指定的截止时间内完成,否则将导致系统故障。
- **软实时操作系统:**对时间要求不太严格,可以容忍某些任务偶尔错过截止时间,但需要保证关键任务的实时性。
- **微内核实时操作系统:**采用微内核架构,仅提供基本的操作系统服务,如任务调度和中断处理,其他功能通过模块化方式实现。
- **宏内核实时操作系统:**提供丰富的操作系统服务,包括文件系统、网络协议栈和图形用户界面。
### 2.2 实时操作系统中的任务和调度
**任务**
任务是 RTOS 中执行的基本单位,它是一个独立的执行线程,具有自己的代码、数据和堆栈空间。任务可以处于以下状态:
- **就绪:**任务已准备好执行,但等待 CPU 资源。
- **运行:**任务正在 CPU 上执行。
- **阻塞:**任务正在等待某个事件(如 I/O 操作完成)发生。
**调度**
调度是 RTOS 的核心功能,它负责决定哪个任务在特定时间点运行。常见的调度算法包括:
- **优先级调度:**根据任务的优先级分配 CPU 时间,优先级高的任务优先执行。
- **时间片轮转调度:**每个任务分配一个时间片,任务在时间片内执行,时间片结束后切换到下一个任务。
- **最短作业优先调度:**选择执行时间最短的任务,以提高系统吞吐量。
**代码块:任务调度算法示例**
```c
// 优先级调度算法
void priority_scheduler() {
while (true) {
// 获取优先级最高的就绪任务
Task* task = get_highest_priority_task();
// 将任务切换到运行状态
task->state = RUNNING;
// 执行任务
task->execute();
// 将任务切换到就绪状态
task->state = READY;
}
}
// 时间片轮转调度算法
void round_robin_scheduler() {
while (true) {
// 遍历所有就绪任务
for (Task* task in ready_queue) {
// 如果任务的时间片已到
if (task->time_slice == 0) {
// 将任务切换到就绪状态
task->state = READY;
// 重置任务的时间片
task->time_slice = TASK_TIME_SLICE;
}
// 如果任务的时间片未到
else {
// 将任务切换到运行状态
task->state = RUNNING;
// 执行任务
task->execute();
// 减少任务的时间片
task->time_slice--;
}
}
}
}
```
**逻辑分析:**
- 优先级调度算法根据任务的优先级分配 CPU 时间,确保高优先级任务优先执行。
- 时间片轮转调度算法为每个任务分配一个时间片,任务在时间片内执行,时间片结束后切换到下一个任务,从而保证所有任务公平地获得 CPU 时间。
# 3. 单片机汇编语言实时操作系统设计
### 3.1 任务管理和调度算法
**任务管理**
任务管理是实时操作系统中的一项核心功能,负责创建、管理和调度任务。任务是操作系统中执行的独立执行单元,每个任务都有自己的代码、数据和堆栈。任务管理模块负责创建和删除任务,分配资源,以及设置任务的优先级和调度策略。
**调度算法**
调度算法决定了任务的执行顺序。实时操作系统中常用的调度算法包括:
- **先来先服务 (FCFS)**:任务按照到达顺序执行。
- **最短作业优先 (SJF)**:优先执行执行时间最短的任务。
- **最高优先级优先 (HPF)**:优先执行优先级最高的任务。
- **轮转调度**:任务按照轮流的方式执行,每个任务执行一定的时间片后,切换到下一个任务。
### 3.2 中断处理和实时响应
**中断处理**
中断是硬件或软件事件导致程序执行流被暂停的情况。实时操作系统必须及时处理中断,以确保对时间敏感事件的快速响应。中断处理模块负责接收和处理中断请求,并根据中断源执行相应的操作。
**实时响应**
实时响应是实时操作系统的关键特性。实时操作系统必须能够在可预测的时间内对事件做出响应。这需要优化中断处理和调度算法,以确保任务能够在规定的时间限制内完成。
### 3.3 资源管理和同步机制
**资源管理**
资源管理模块负责管理系统中的资源,例如内存、外设和文件。资源管理模块确保任务能够安全地访问和使用这些资源,并防止资源冲突。
**同步机制**
同步机制用于协调多个任务对共享资源的访问。常见的同步机制包括:
- **互斥量**:确保一次只有一个任务可以访问临界区。
- **信号量**:用于
0
0
相关推荐
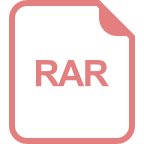
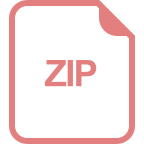
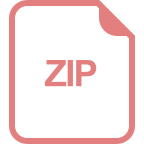
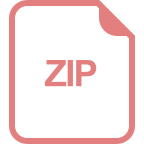
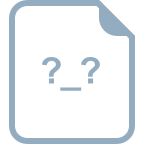