使用React Native进行布局设计
发布时间: 2024-02-22 04:31:39 阅读量: 45 订阅数: 17 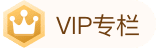
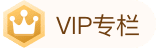
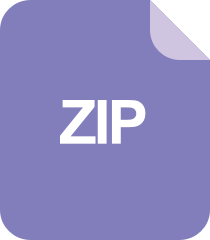
React Native的布局代码
# 1. React Native布局设计基础
## 1.1 React Native简介
React Native是一个由Facebook开源的跨平台移动应用开发框架,使用JavaScript和React来构建用户界面。它允许开发人员使用相同的代码库在iOS和Android平台上构建原生应用。
## 1.2 布局设计概述
在移动应用开发中,布局设计是非常重要的,它关乎用户界面的外观和响应性。在React Native中,布局设计涉及到组件的排列、尺寸和样式设置等方面。
## 1.3 Flex布局原理
Flex布局是React Native中常用的一种布局方式,它基于弹性盒子模型,通过弹性的方式来进行组件的布局排列。开发者可以使用Flexbox来实现灵活的布局设计,适配不同尺寸的屏幕和不同设备。
在接下来的内容中,我们将深入介绍React Native布局设计的基础知识以及灵活布局的实现方法。
# 2. React Native中的组件布局
在React Native开发中,组件布局是非常重要的一部分,通过合适的布局方式可以实现页面的灵活性和美观性。以下是一些关于React Native中组件布局的内容:
### 2.1 常用布局组件介绍
在React Native中,有许多常用的布局组件可以帮助我们实现不同类型的布局。其中,常见的布局组件包括:
- **View组件**:View是React Native中最基本的组件,用于包裹其他组件并控制它们的位置和样式。
- **Text组件**:Text用于显示文本内容,可以设置文字样式、字体大小等。
- **Image组件**:Image组件用于显示图片,可以设置图片源、尺寸等属性。
- **ScrollView组件**:ScrollView用于实现可滚动的视图,适用于长列表或大段文字显示。
### 2.2 使用Flexbox进行组件布局
在React Native中,Flexbox是一种弹性布局模型,通过在父容器上设置`flexDirection`、`justifyContent`、`alignItems`等样式属性,可以实现灵活的布局。下面是一个简单的Flex布局示例:
```jsx
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const FlexLayoutExample = () => {
return (
<View style={styles.container}>
<View style={styles.box1}>
<Text>Box 1</Text>
</View>
<View style={styles.box2}>
<Text>Box 2</Text>
</View>
<View style={styles.box3}>
<Text>Box 3</Text>
</View>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
justifyContent: 'space-between',
alignItems: 'center',
},
box1: {
flex: 1,
backgroundColor: 'red',
padding: 10,
},
box2: {
flex: 2,
backgroundColor: 'blue',
padding: 10,
},
box3: {
flex: 1,
backgroundColor: 'green',
padding: 10,
},
});
export default FlexLayoutExample;
```
在上面的示例中,我们使用Flexbox布局实现了一个横向排列的布局,其中三个盒子根据各自的`flex`值占据不同比例的空间。
### 2.3 网格布局的实现
在实际开发中,有时候我们需要实现网格布局,来展示多个数据项或图像等。在React Native中,可以通过计算每个格子的宽度和高度,以及使用`flexWrap`属性来实现简单的网格布局。下面是一个简单的网格布局示例:
```jsx
import React from 'react';
import { View, StyleSheet } from 'react-native';
const GridLayoutExample = () => {
return (
<View style={styles.gridContainer}>
<View style={styles.gridItem}></View>
<View style={styles.gridItem}></View>
<View style={styles.gridItem}></View>
<View style={styles.gridItem}></View>
</View>
);
};
const styles = StyleSheet.create({
gridContainer: {
flexDirection: 'row',
flexWrap: 'wrap',
justifyContent: 'space-between',
},
gridItem: {
width: '45%',
aspectRatio: 1, // 1:1宽高比
backgroundColor: 'lightgray',
marginVertical: 5,
},
});
export default GridLayoutExample;
```
在上面的示例中,我们通过设置`flexWrap: 'wrap'`来实现了四个等宽的正方形格子,它们会根据容器宽度自动换行。
通过灵活运用Flexbox和样式属性,我们可以实现各种类型的组件布局,让页面展现出我们想要的效果。
# 3. 响应式设计和多设备适配
在移动应用开发中,响应式设计和多设备适配是非常重要的,因为用户可能使用不同大小和分辨率的设备访问您的应用。React Native提供了一些机制来帮助开发人员创建适应不同设备的布局。
#### 3.1 响应式设计原理
响应式设计的核心原理是使应用能够根据设备的属性(如屏幕尺寸、方向等)以及用户行为(如旋转设备、调整窗口大小)做出相应的布局调整。在React Native中,可以通过Flex布局和Media Queries等方式实现响应式设计。
#### 3.2 使用Media Queries实现多设备适配
Media Queries是一种CSS3特性,可以根据不同的媒体类型和属性条件应用样式。在React Native中,并没有直接支持Media Queries,但可以通过Dimensions API获取设备的宽度和高度,然后根据具体情况动态设置组件的样式。
```javascript
import {Dimensions, StyleSheet} from 'react-native';
const {width} = Dimensions.get('window');
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'lightblue',
padding: 10,
// 根据不同设备宽度动态调整样式
width: width > 600 ? '50%' : '80%',
},
text: {
fontSize: 16,
color: 'white',
},
});
```
#### 3.3 适配不同屏幕尺寸的布局策略
针对不同的屏幕尺寸和分辨率,可以采取以下策略来实现布局适配:
- 使用Flex布局和相对单位(如百分比)来设计布局,使组件能够根据可用空间自动调整大小和位置。
- 根据设备宽度动态设置组件样式,以适配不同尺寸的屏幕。
- 使用ScrollView等组件来处理大量内容和滚动视图,确保内容可以在各种屏幕上正确显示。
通过以上策略,可以实现对不同屏幕尺寸设备的良好适配,提高用户体验和应用的可用性。
# 4. Style布局设计
在React Native中,样式是布局设计中至关重要的一部分。本章将介绍如何使用StyleSheet创建样式,以及样式的复用、继承和最佳实践。
#### 4.1 使用StyleSheet创建样式
在React Native中,可以使用StyleSheet.create方法创建样式对象,以确保高效的样式定义和管理。以下是一个简单的示例:
```javascript
import React, { Component } from 'react';
import { View, Text, StyleSheet } from 'react-native';
export default class StyleExample extends Component {
render() {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, World!</Text>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
color: 'blue',
},
});
```
#### 4.2 样式的复用和继承
为了避免代码重复,可以将样式定义为常量,并在需要的地方进行引用。另外,React Native也支持样式的继承,可以通过扩展已有样式来创建新的样式。
```javascript
import React, { Component } from 'react';
import { View, Text, StyleSheet } from 'react-native';
const commonStyles = {
text: {
fontSize: 16,
color: 'black',
},
title: {
fontSize: 24,
fontWeight: 'bold',
},
};
export default class StyleReuseExample extends Component {
render() {
return (
<View style={styles.container}>
<Text style={commonStyles.text}>Regular Text</Text>
<Text style={[commonStyles.text, commonStyles.title]}>Title Text</Text>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
```
#### 4.3 在React Native中使用样式表的最佳实践
- 使用StyleSheet.create方法创建样式对象,以提高性能和可读性。
- 将重复使用的样式定义为常量,并在需要的地方进行引用。
- 善用样式的继承,避免重复定义相似的样式。
通过本章的学习,读者将掌握如何在React Native中高效地创建、复用和继承样式,以实现灵活且易于维护的布局设计。
# 5. 布局性能优化
在使用React Native进行布局设计时,除了实现功能需求,我们也需要重视布局性能优化,以提升用户体验和应用性能。以下是一些布局性能优化的技巧:
### 5.1 避免布局抖动
布局抖动是指由于频繁触发布局重绘导致界面元素不断变化位置的现象,会影响用户体验。为了避免布局抖动,可以采取以下措施:
```javascript
// 不推荐的写法,会导致布局抖动
state = {
width: 100
}
// 在render函数中避免频繁改变布局
render() {
return (
<View style={{ width: this.state.width }}>
<Text>Content</Text>
</View>
);
}
```
### 5.2 避免不必要的布局计算
在布局设计中,避免进行不必要的布局计算可以提升性能。比如,在布局中避免使用`measure`、`onLayout`等会触发布局计算的属性和方法,除非必要。
```javascript
// 避免频繁触发布局计算
<View onLayout={this.handleLayout}>
<Text>Content</Text>
</View>
```
### 5.3 使用PureComponent优化布局组件
React Native中的`PureComponent`可以帮助我们优化布局组件的性能,避免不必要的渲染。
```javascript
import React, { PureComponent } from 'react';
class OptimizedComponent extends PureComponent {
render() {
return (
<View>
<Text>Optimized Content</Text>
</View>
);
}
}
```
通过以上布局性能优化的技巧,我们可以更好地提升React Native应用的性能表现,让用户体验更加流畅。
# 6. 实际案例分析与实践
在本章节中,我们将深入探讨使用React Native进行布局设计的实际案例和实践经验,通过具体的项目案例和实际经验分享,帮助读者更好地理解布局设计的应用和技巧。
#### 6.1 实际项目布局设计案例
在这一部分,我们将以一个简单的实际项目示例来演示如何使用React Native进行布局设计。假设我们需要创建一个登录页面,包括输入用户名、密码和登录按钮,并且要求在不同屏幕尺寸上都能良好展示。
```javascript
import React from 'react';
import { View, TextInput, Button } from 'react-native';
const LoginScreen = () => {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<TextInput
placeholder="Enter your username"
style={{ height: 40, borderColor: 'gray', borderWidth: 1, width: '80%', marginBottom: 20, padding: 10 }}
/>
<TextInput
placeholder="Enter your password"
style={{ height: 40, borderColor: 'gray', borderWidth: 1, width: '80%', marginBottom: 20, padding: 10 }}
secureTextEntry={true}
/>
<Button
title="Login"
onPress={() => { /* 登录逻辑 */ }}
/>
</View>
);
};
export default LoginScreen;
```
在上面的代码中,我们使用了`View`、`TextInput`和`Button`等React Native组件来实现一个简单的登录页面布局。通过`flex`布局和样式设置,我们使得页面在不同设备上都能够居中显示,并且输入框和按钮大小适中。
#### 6.2 布局设计实践经验分享
在布局设计的实践过程中,我们还可以根据具体项目情况进行布局设计的优化和调整。例如,可以根据实际需求添加动画效果、定时刷新等功能,提升用户体验和页面性能。
在React Native中,还可以使用第三方库如`Styled Components`、`UI Kitten`等来简化样式的管理和布局设计,提高开发效率。同时,及时对布局进行测试和调试,保证页面在不同屏幕尺寸和设备上都有良好的展示效果。
#### 6.3 React Native布局设计的进阶技巧
最后,在进阶章节中,我们将介绍一些React Native布局设计的高级技巧,如使用`Dimensions`获取设备屏幕尺寸、使用`React Navigation`实现页面导航等。这些技巧可以帮助开发者更加灵活地设计和控制页面布局,满足不同项目的需求。
通过对实际案例分析和实践经验分享,读者将更好地掌握React Native布局设计的应用技巧和优化策略,为项目开发提供帮助和指导。
0
0
相关推荐
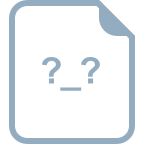
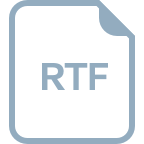
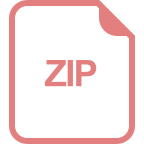
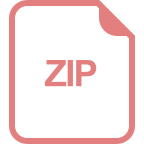
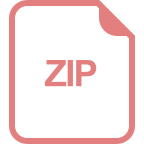
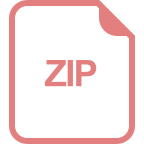
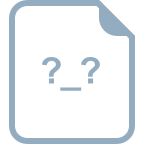
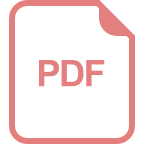