如何使用Spring Security4保护RESTful API
发布时间: 2023-12-16 21:05:07 阅读量: 31 订阅数: 36 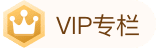
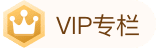
# 1. 介绍Spring Security4和RESTful API
#### 1.1 什么是Spring Security4?
Spring Security是一个功能强大且高度可定制的身份验证和访问控制框架。它提供了全面的安全性解决方案,包括身份验证、授权、会话管理以及防止常见的攻击。Spring Security4是Spring Security的最新版本,为RESTful API提供了更加灵活和强大的安全性支持。
#### 1.2 什么是RESTful API?
RESTful API是一种基于REST架构风格设计的API。它使用统一的接口,通过HTTP协议传输数据,包括GET、POST、PUT和DELETE等操作。RESTful API的设计原则包括无状态、可缓存、统一接口等,使得它成为了现代Web应用程序的常见API设计风格。
#### 1.3 Spring Security4在保护RESTful API中的作用
Spring Security4提供了一套完善的身份验证和授权机制,可以轻松地集成到RESTful API中,保护API的安全性。它支持多种认证方式、各种授权策略,并且提供了丰富的扩展点,适用于各种复杂的场景下。在RESTful API开发中,Spring Security4能够有效地保护API免受恶意攻击和未授权访问。
接下来,我们将深入探讨如何配置和使用Spring Security4来保护RESTful API,以及最佳实践和推荐的安全性策略。
# 2. 配置Spring Security4
在这一章节中,我们将介绍如何配置Spring Security4来保护RESTful API。我们将重点讨论添加Spring Security4依赖、配置Spring Security4以及自定义安全配置的具体步骤。
### 2.1 添加Spring Security4依赖
首先,我们需要在项目的Maven或Gradle配置文件中添加Spring Security4的依赖。在Maven项目中,可以像下面这样添加依赖:
```xml
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-core</artifactId>
<version>4.2.3.RELEASE</version>
</dependency>
```
在Gradle项目中,可以通过以下方式添加依赖:
```gradle
compile group: 'org.springframework.security', name: 'spring-security-core', version: '4.2.3.RELEASE'
```
### 2.2 配置Spring Security4
接下来,我们需要配置Spring Security4来定义安全规则和访问控制策略。可以创建一个类继承自`WebSecurityConfigurerAdapter`,并重写`configure`方法来进行配置:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
}
```
在上面的例子中,我们定义了一个基本的安全配置,允许对`/public/**`路径的请求进行匿名访问,其它请求需要进行认证。
### 2.3 自定义安全配置
除了使用默认的安全配置外,我们还可以根据项目的实际需求进行自定义安全配置。可以通过创建自定义的`UserDetailsService`、`AuthenticationProvider`和`AuthenticationEntryPoint`等来实现更复杂的安全机制。
```java
@Configuration
@EnableWebSecurity
public class CustomSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService userDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService)
.passwordEncoder(new BCryptPasswordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
}
```
在上面的例子中,我们使用自定义的`UserDetailsService`来实现用户认证,并定义了基于角色的访问控制规则。
通过上面这些配置,我们就可以基本的保护我们的RESTful API了。接下来,我们将会讨论更多高级的认证和授权机制。
0
0
相关推荐
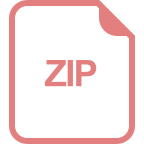
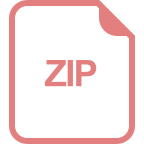
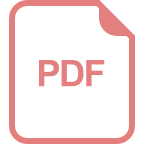
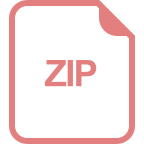
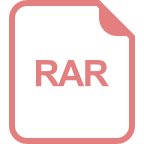
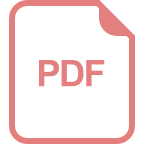
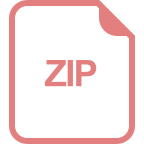
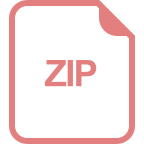
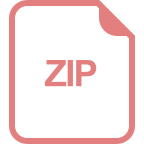