点与圆、球的位置关系解析
发布时间: 2024-03-03 11:48:21 阅读量: 37 订阅数: 24 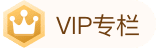
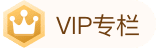
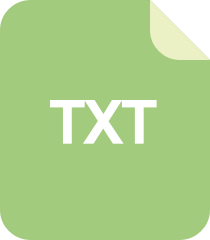
用C++判断直线和圆的位置关系
# 1. 点和圆的基本概念
## 1.1 圆的定义
在平面几何中,圆是由平面上距离一个固定点距离相等的所有点组成的几何图形。这个固定点称为圆心,距离称为半径。圆可以用圆心坐标和半径来表示。
```python
# Python代码示例
class Circle:
def __init__(self, center, radius):
self.center = center # 圆心坐标
self.radius = radius # 半径
def area(self):
return 3.14 * self.radius * self.radius # 圆的面积
def circumference(self):
return 2 * 3.14 * self.radius # 圆的周长
circle1 = Circle((0, 0), 5) # 创建一个圆,圆心坐标为(0, 0),半径为5
print("圆的面积为:", circle1.area())
print("圆的周长为:", circle1.circumference())
```
## 1.2 点的坐标表示
在二维坐标系中,点可以用(x, y)的坐标表示,其中x和y分别代表点在水平和垂直方向上的位置。
```java
// Java代码示例
public class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
Point point1 = new Point(3, 4); // 创建一个点,坐标为(3, 4)
System.out.println("点的坐标为:(" + point1.getX() + ", " + point1.getY() + ")");
```
## 1.3 点到圆的距离公式
设圆的圆心坐标为(x0, y0),点的坐标为(x, y),则点到圆心的距离d可通过以下公式计算:
$d = \sqrt{(x - x0)^2 + (y - y0)^2}$
```go
// Go代码示例
package main
import (
"fmt
"math"
)
func distanceToCircle(centerX, centerY, x, y, radius float64) float64 {
return math.Sqrt(math.Pow(x-centerX, 2) + math.Pow(y-centerY, 2)) - radius
}
func main() {
centerX, centerY := 1.0, 1.0 // 圆心坐标
x, y := 3.0, 4.0 // 点的坐标
radius := 5.0 // 圆的半径
distance := distanceToCircle(centerX, centerY, x, y, radius)
fmt.Println("点到圆的
```
0
0
相关推荐
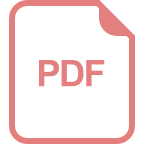
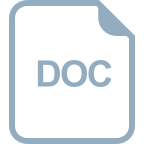





