关系的特性
发布时间: 2024-01-29 11:33:55 阅读量: 31 订阅数: 22 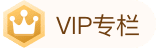
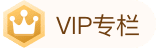
# 1. 关系数据库的特性
## 1.1 数据的结构化
关系数据库是以表格形式存储和管理数据的系统,其中数据以结构化的形式存储,以便有效地组织和访问。数据的结构化使得数据项之间存在明确的关系和约束,可以方便地进行增删改查操作。
```python
# 示例代码:创建关系数据库表
CREATE TABLE students (
id INT PRIMARY KEY,
name VARCHAR(100),
age INT,
course VARCHAR(100)
);
```
在上述示例中,我们创建了一个名为students的数据表,其中包含id、name、age和course等列,每个列都有其特定的数据类型。这种结构化的数据模型使得数据的存储和管理更加高效。
## 1.2 数据一致性
关系数据库具有数据一致性的特性,即数据的变动应该符合数据库中定义的各种规则和约束条件。当对数据库进行更新操作时,系统会自动检查数据的一致性,确保数据的完整性和正确性。
```java
// 示例代码:插入数据并保持一致性
INSERT INTO students (id, name, age, course)
VALUES (1, 'Alice', 20, 'Math');
// 更新数据,并保持一致性
UPDATE students
SET age = 21
WHERE id = 1;
```
在上述示例中,我们插入了一条学生数据,并执行了更新操作。数据库会自动检查约束条件,例如主键的唯一性、字段类型的正确性等,确保数据的一致性。
## 1.3 数据的索引和查询
关系数据库支持数据的索引和查询操作,以提高数据检索的效率。通过在表格的某个列上创建索引,可以快速地定位目标数据,而不需要遍历整个表格。
```sql
-- 示例代码:创建索引
CREATE INDEX idx_students_name ON students (name);
-- 查询操作
SELECT * FROM students WHERE name = 'Alice';
```
在上述示例中,我们创建了一个名为idx_students_name的索引,以加快对name字段的查询速度。当查询时,数据库会利用该索引直接定位到满足条件的数据,提高查询的效率。
## 1.4 数据完整性和安全性
关系数据库具有数据的完整性和安全性保障。通过定义约束条件和权限控制机制,可以保证数据的完整性,防止数据的意外修改和删除。同时,数据库也支持数据的备份和恢复,以应对意外情况。
```sql
-- 示例代码:定义约束条件和权限控制
ALTER TABLE students
ADD CONSTRAINT age_check CHECK (age >= 0);
-- 备份和恢复
BACKUP DATABASE students TO 'backup/students.bak';
RESTORE DATABASE students FROM 'backup/students.bak';
```
在上述示例中,我们定义了一个名为age_check的约束条件,确保学生的年龄大于等于0。同时,我们还展示了如何进行数据库的备份和恢复操作,以保证数据的安全性。
以上是关系数据库的一些特性,尽管现在有许多新型数据库的出现,但关系数据库仍然是广泛应用的一种数据存储和管理方式。
# 2. 网络关系的特性
在IT领域中,网络是一个至关重要的组成部分。网络关系的特性对于数据传输、安全性和性能都有着重要的影响。本章将介绍网络关系的一些重要特性。
### 2.1 网络拓扑结构
网络拓扑结构是指网络中各个节点之间连接的方式。常见的网络拓扑结构包括星型、总线型、环型、树型和网状型等。不同的拓扑结构具有不同的特点和适用场景。
在此,我们以Python代码演示一个简单的星型拓扑结构的创建和数据传输:
```python
import socket
# 创建一个服务器Socket对象
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_address = ('localhost', 8888)
# 绑定服务器地址
server_socket.bind(server_address)
# 监听客户端连接
server_socket.listen(1)
print('服务器已启动,等待客户端连接...')
while True:
# 接受客户端连接
client_socket, client_address = server_socket.accept()
print('客户端已连接:', client_address)
# 接收客户端发送的数据
data = client_socket.recv(1024)
print('接收到客户端的数据:', data.decode('utf-8'))
# 发送响应给客户端
response = 'Hello, client!'
client_socket.send(response.encode('utf-8'))
# 关闭客户端连接
client_socket.close()
```
这段代码创建了一个简单的服务器Socket对象,使用星型拓扑结构监听客户端连接,接收客户端发送的数据并发送响应。通过运行代码,可以建立起一个简单的星型网络结构。
### 2.2 数据传输的可靠性
在网络中,数据传输的可靠性是指数据能否按照正确的顺序和完整的传输到目的地。为了保证数据传输的可靠性,常用的技术包括错误校验、重传机制和流量控制等。
以下是一个基于Java的简单数据传输代码示例,使用TCP协议保证数据传输的可靠性:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.net.Socket;
public class TCPClient {
private static final String SERVER_IP = "localhost";
private static final int SERVER_PORT = 8888;
public static void main(String[] args) {
try {
// 创建客户端Socket对象
Socket clientSocket = new Socket(SERVER_IP, SERVER_PORT);
// 创建输入流和输出流
BufferedReader inFromServer = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
PrintWriter outToServer = new PrintWriter(clientSocket.getOutputStream(), true);
// 发送数据给服务器
outToServer.println("Hello, server!");
// 接收服务器端响应数据
String response = inFromServer.readLine();
System.out.println("接收到服务器的数据:" + response);
// 关闭连接
clientSocket.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,客户端通过Socket连接到服务器,并发送数据给服务器。服务器接收到数据后进行响应,客户端再次接收响应数据。这个过程通过TCP协议保证了数据传输的可靠性。
### 2.3 网络性能和带宽
网络性能是指在一定时间内网络传输的数据量和速度。而带宽是指网络传输的最大数据量。网络性能和带宽对于数据传输的效率和用户体验都至关重要。
以下是一个使用Go语言进行网络性能测试的示例代码:
```go
package main
import (
"fmt"
"net/http"
)
func main() {
url := "https://www.example.com"
// 发送HTTP GET请求
response, err := http.Get(url)
if err != nil {
fmt.Println("请求失败:", err)
return
}
defer response.Body.Close()
// 输出响应状态码和内容长度
fmt.Println("状态码:", response.StatusCode)
fmt.Println("内容长度:", response.ContentLength)
}
```
通过这段代码,我们可以发送HTTP GET请求来测试指定URL的网络性能,可以获取到响应的状态码和内容长度,从而了解网络传输的相关性能指标。
0
0
相关推荐
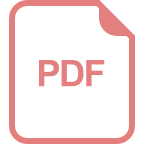
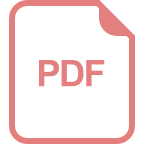
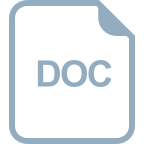
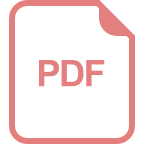
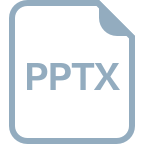