Spring中的Bean生命周期管理与扩展点
发布时间: 2023-12-21 08:28:28 阅读量: 39 订阅数: 33 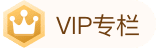
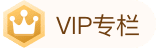
## 一、Spring框架中Bean的生命周期管理
### 二、Spring框架中Bean的生命周期扩展方式
在Spring框架中,Bean的生命周期可以通过多种方式进行扩展,包括BeanPostProcessor接口、InitializingBean和DisposableBean接口以及@PostConstruct和@PreDestroy注解等。下面将详细介绍这些扩展方式。
#### 2.1 BeanPostProcessor接口
BeanPostProcessor接口是用来在Bean初始化前后进行一些自定义处理的接口。通过实现BeanPostProcessor接口,可以在Bean的实例化、依赖注入和初始化等步骤前后插入自定义逻辑。
```java
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
public class CustomBeanPostProcessor implements BeanPostProcessor {
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
// 在初始化之前进行自定义处理
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
// 在初始化之后进行自定义处理
return bean;
}
}
```
#### 2.2 InitializingBean和DisposableBean接口
InitializingBean和DisposableBean接口分别定义了在Bean初始化之后和销毁之前的自定义逻辑。通过实现这两个接口,可以在Bean的初始化和销毁阶段执行一些特定操作。
```java
import org.springframework.beans.factory.DisposableBean;
import org.springframework.beans.factory.InitializingBean;
public class CustomBean implements InitializingBean, DisposableBean {
@Override
public void afterPropertiesSet() throws Exception {
// 在初始化之后进行自定义逻辑
}
@Override
public void destroy() throws Exception {
// 在销毁之前进行自定义逻辑
}
}
```
#### 2.3 @PostConstruct和@PreDestroy注解
@PostConstruct和@PreDestroy注解分别用于标记初始化和销毁方法。通过在Bean的初始化方法上标记@PostConstruct注解,在销毁方法上标记@PreDestroy注解,可以达到和InitializingBean和DisposableBean接口相同的效果。
```java
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
public class CustomBean {
@PostConstruct
public void customInit() {
// 自定义初始化逻辑
}
@PreDestroy
public void customDestroy() {
// 自定义销毁逻辑
}
}
```
### 三、Bean生命周期中的Aware接口
在Spring框架中,Bean的生命周期中存在着一些特殊的接口,它们可以让Bean在特定阶段感知到容器的存在或者自身的状态,这些接口被统称为Aware接口。通过实现Aware接口,我们可以在Bean的创建和初始化阶段获取到更多关于容器和Bean自身的信息,从而实现更精细化的控制和定制化操作。
#### 3.1 BeanNameAware接口
BeanNameAware接口是一个简单的接口,它只包含了一个方法`setBeanName`。当一个Bean实现了BeanNameAware接口时,在Bean被容器实例化之后,容器会调用`setBeanName`方法将Bean的名字传递给Bean,从而让Bean知道自己在容器中的名字。通过实现BeanNameAware接口,我们可以在Bean初始化之后获取到Bean在容器中的名称,用于记录日志、打印调试信息等操作。
```java
import org.springframework.beans.factory.BeanNameAware;
public class MyBean implements BeanNameAware {
private String beanName;
@Override
public void setBeanName(String name) {
this.beanName = name;
System.out.println("MyBean的名字是:" + beanName);
}
}
```
#### 3.2 BeanFactoryAware接口
BeanFactoryAware接口是用来让Bean获取所属的BeanFactory实例的接口,它包含了`setBeanFactory`方法。当一个Bean实现了BeanFactoryAware接口时,在Bean被容器实例化之后,容器会调用`setBeanFactory`方法将BeanFactory的实例传递给Bean,从而让Bean知道所属的BeanFactory。通过实现BeanFactoryAware接口,我们可以在Bean初始化之后获取到所属的BeanFactory实例,从而实现一些动态的Bean操作或者资源管理。
```java
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.BeanFactoryAware;
public class MyBean implements BeanFactoryAware {
private BeanFactory beanFactory;
@Override
public void setBeanFactory(BeanFactory beanFactory) {
this.beanFactory = beanFactory;
System.out.println("MyBean所属的BeanFactory是:" + beanFactory);
}
}
```
#### 3.3 ApplicationContextAware接口
ApplicationContextAware接口是用来让Bean获取所属的ApplicationContext实例的接口,它也包含了`setApplicationContext`方法。当一个Bean实现了ApplicationContextAware接口时,在Bean被容器实例化之后,容器会调用`setApplicationContext`方法将ApplicationContext的实例传递给Bean,从而让Bean知道所属的ApplicationContext。通过实现ApplicationContextAware接口,我们可以在Bean初始化之后获取到所属的ApplicationContext实例,从而实现一些更高级的Bean操作或者资源管理。
```java
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
public class MyBean implements ApplicationContextAware {
private ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) {
this.applicationContext = applicationContext;
System.out.println("MyBean所属的ApplicationContext是:" + applicationContext);
}
}
```
### 四、Bean生命周期中的事件处理
在Spring框架中,Bean的生命周期中还涉及到事件的处理。Spring提供了多种方式来处理Bean生命周期中的事件,包括使用ApplicationEvent及ApplicationListener处理事件、使用@EventListener注解处理事件以及使用BeanPostProcessor处理事件。
#### 4.1 使用ApplicationEvent及ApplicationListener处理事件
在Spring框架中,可以通过定义自定义的事件,然后通过ApplicationListener接口来监听和处理这些事件。以下是事件处理的具体步骤。
1. 定义自定义事件类,继承自ApplicationEvent:
```java
import org.springframework.context.ApplicationEvent;
public class CustomEvent extends ApplicationEvent {
public CustomEvent(Object source) {
super(source);
}
@Override
public String toString() {
return "CustomEvent Occurred";
}
}
```
2. 创建事件监听器,实现ApplicationListener接口,并处理自定义事件:
```java
import org.springframework.context.ApplicationListener;
public class CustomEventListener implements ApplicationListener<CustomEvent> {
@Override
public void onApplicationEvent(CustomEvent event) {
System.out.println("Received custom event: " + event.toString());
}
}
```
3. 在配置文件中注册事件监听器:
```xml
<bean class="com.example.CustomEventListener"/>
```
4. 发布自定义事件:
```java
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.context.ApplicationEventPublisherAware;
public class CustomEventPublisher implements ApplicationEventPublisherAware {
private ApplicationEventPublisher publisher;
public void publishEvent() {
CustomEvent event = new CustomEvent(this);
publisher.publishEvent(event);
}
@Override
public void setApplicationEventPublisher(ApplicationEventPublisher publisher) {
this.publisher = publisher;
}
}
```
#### 4.2 使用@EventListener注解处理事件
除了使用ApplicationEvent及ApplicationListener处理事件外,Spring还提供了使用@EventListener注解来处理事件的方式。使用@EventListener注解的方法将会成为事件的处理方法,当事件发生时自动被调用。
1. 创建事件发布类,用于发布事件:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.stereotype.Component;
@Component
public class MyEventPublisher {
@Autowired
private ApplicationEventPublisher publisher;
public void publishEvent(String message) {
MyEvent event = new MyEvent(this, message);
publisher.publishEvent(event);
}
}
```
2. 创建事件类,继承自ApplicationEvent:
```java
import org.springframework.context.ApplicationEvent;
public class MyEvent extends ApplicationEvent {
private String message;
public MyEvent(Object source, String message) {
super(source);
this.message = message;
}
public String getMessage() {
return message;
}
}
```
3. 创建事件监听方法,并使用@EventListener注解处理事件:
```java
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
@Component
public class MyEventListener {
@EventListener
public void handleContextStart(MyEvent event) {
System.out.println("Received MyEvent: " + event.getMessage());
}
}
```
#### 4.3 使用BeanPostProcessor处理事件
除了通过自定义事件和@EventListener注解处理事件外,还可以使用BeanPostProcessor处理事件。BeanPostProcessor是一个接口,它允许在Bean初始化前后进行一些定制操作。
1. 创建自定义的BeanPostProcessor:
```java
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
public class CustomBeanPostProcessor implements BeanPostProcessor {
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
System.out.println("Before Initialization : " + beanName);
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
System.out.println("After Initialization : " + beanName);
return bean;
}
}
```
2. 在配置文件中注册自定义的BeanPostProcessor:
```xml
<bean class="com.example.CustomBeanPostProcessor"/>
```
通过以上方式,可以在Bean的生命周期中进行事件的处理,包括自定义事件发布与监听、@EventListener注解处理事件以及使用BeanPostProcessor进行定制操作。
## 五、Spring框架中Bean的生命周期实例
在Spring框架中,我们可以通过编写自定义的类来扩展Bean的生命周期,并对Bean的创建、初始化和销毁过程进行定制化操作。接下来,我们将通过实际的代码示例来展示如何编写自定义的BeanPostProcessor、InitializingBean和DisposableBean,以及如何编写自定义的Aware接口和事件处理。
### 5.1 编写自定义的BeanPostProcessor
```java
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.config.BeanPostProcessor;
public class CustomBeanPostProcessor implements BeanPostProcessor {
@Override
public Object postProcessBeforeInitialization(Object bean, String beanName) throws BeansException {
System.out.println("Before initialization: " + beanName);
return bean;
}
@Override
public Object postProcessAfterInitialization(Object bean, String beanName) throws BeansException {
System.out.println("After initialization: " + beanName);
return bean;
}
}
```
在上面的代码中,我们编写了一个自定义的BeanPostProcessor实现类CustomBeanPostProcessor,重写了postProcessBeforeInitialization和postProcessAfterInitialization方法,在Bean初始化之前和之后分别进行了输出操作。
### 5.2 编写自定义的InitializingBean和DisposableBean
```java
import org.springframework.beans.factory.DisposableBean;
import org.springframework.beans.factory.InitializingBean;
public class CustomInitializingBean implements InitializingBean, DisposableBean {
@Override
public void afterPropertiesSet() throws Exception {
System.out.println("CustomInitializingBean: InitializingBean's afterPropertiesSet method");
}
@Override
public void destroy() throws Exception {
System.out.println("CustomInitializingBean: DisposableBean's destroy method");
}
}
```
上面的代码展示了一个自定义的InitializingBean和DisposableBean实现类CustomInitializingBean,分别重写了afterPropertiesSet和destroy方法,在Bean初始化和销毁时进行了输出操作。
### 5.3 编写自定义的Aware接口和事件处理
```java
import org.springframework.beans.factory.BeanNameAware;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.ApplicationListener;
public class CustomAwareAndEvent implements BeanNameAware, ApplicationListener<ApplicationEvent> {
@Override
public void setBeanName(String name) {
System.out.println("CustomAwareAndEvent: Bean name is " + name);
}
@Override
public void onApplicationEvent(ApplicationEvent event) {
System.out.println("CustomAwareAndEvent: Received custom event - " + event.toString());
}
}
```
上面的代码中,我们编写了一个自定义的Aware接口实现类CustomAwareAndEvent,实现了BeanNameAware和ApplicationListener接口,在Bean初始化时获取Bean的名称,并监听并处理自定义的事件。
通过以上示例,我们可以看到在Spring框架中如何编写自定义的BeanPostProcessor、InitializingBean和DisposableBean,以及如何编写自定义的Aware接口和事件处理,从而对Bean的生命周期进行进一步的定制化操作。
### 六、Spring框架中Bean生命周期管理的最佳实践
在Spring框架中,Bean的生命周期管理是非常重要的,合理的管理Bean的生命周期可以提高系统的性能和可维护性。下面将介绍一些在实际开发中的最佳实践。
#### 6.1 如何合理选择Bean的创建方式
在选择Bean的创建方式时,可以根据具体的需求和情况来选择合适的创建方式:
- **构造方法注入**:当Bean被创建时需要初始化一些必要的属性,可以使用构造方法注入来保证Bean在实例化时就拥有必要的属性。
- **工厂方法创建**:通过工厂方法创建Bean可以实现更灵活的对象创建过程,可以根据需要对对象的创建过程进行定制。
- **静态工厂方法创建**:通过静态工厂方法创建Bean可以在不实例化对象的情况下获取需要的对象实例。
#### 6.2 如何避免内存泄漏和资源泄漏
在Spring框架中,由于Bean的生命周期管理交给Spring容器来管理,如果不注意一些细节就有可能导致内存泄漏和资源泄漏的问题。
- **合理使用Bean的作用域**:根据实际情况选择合适的Bean作用域,避免使用过多的单例模式Bean导致内存占用过高。
- **注意手动资源释放**:对于一些需要手动释放资源的Bean,需要在Bean销毁的时候手动释放资源,避免资源泄漏。
#### 6.3 如何灵活应用Bean生命周期管理的扩展点
Spring框架提供了丰富的Bean生命周期扩展方式,合理地使用这些扩展点可以实现更多的定制化需求。
- **实现BeanPostProcessor接口**:可以在Bean的初始化前后执行一些自定义逻辑,例如数据校验、权限检查等。
- **使用@PostConstruct和@PreDestroy注解**:可以使用注解的方式在Bean的初始化和销毁时执行特定的方法,简化了Bean的生命周期管理逻辑。
- **使用事件处理机制**:通过使用事件监听机制,可以在Bean生命周期的各个阶段执行相应的逻辑,实现更加灵活的扩展。
0
0
相关推荐
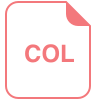
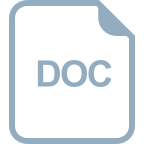
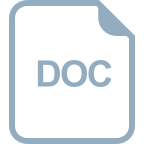
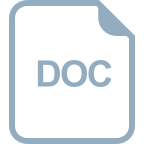
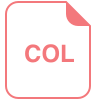
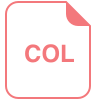