Optimizing Performance of kkfileview in Large File Stream Previews
发布时间: 2024-09-15 17:09:13 阅读量: 27 订阅数: 25 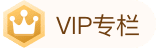
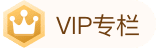
# 1. Introduction
In modern Web applications, the file preview feature is extremely common. **kkfileview** serves as a solution for file previews, offering users a convenient and fast preview experience. However, as file sizes increase, previewing large file streams poses challenges to performance. This chapter will delve into the background and role of kkfileview and analyze the challenges faced by large file stream previews. Understanding the characteristics of kkfileview can help us better grasp the principles behind implementing file preview functionality and lay the foundation for subsequent performance optimizations. Through the content of this chapter, readers will gain a clearer understanding of kkfileview and recognize the challenges that come with previewing large file streams, laying the groundwork for future research and optimization efforts.
**Keywords:** kkfileview, file preview, large file streams, performance challenges, optimization.
# 2. Fundamental Performance Analysis
When dealing with large file stream previews, the challenges faced by kkfileview mainly include performance bottlenecks, impacts on performance, and strategies for different usage scenarios. This chapter will analyze these issues.
### 2.1 Performance Bottleneck Analysis of kkfileview
Before performing any optimizations, it'***mon performance bottlenecks may arise from IO operations, memory management, concurrency handling, and more. Static code analysis and performance testing can help identify specific bottlenecks.
### 2.2 Impact of Large File Stream Previews on Performance
The impact of large file stream previews on performance is evident. As file sizes increase, so do the resources required for file reading and display. Therefore, effectively handling large file stream previews is crucial for enhancing the performance of kkfileview.
### 2.3 Usage Scenario Analysis
Different usage scenarios have varying performance requirements for kkfileview. For instance, frequent access to files requires consideration of how to increase the loading speed, while previewing large files necessitates addressing high memory usage. Thus, performance optimization strategies must be tailored to different scenarios.
The above content outlines the fundamental performance analysis, through which a better understanding of the performance issues of kkfileview can be achieved, guiding subsequent optimization strategies. Next, we will delve into the strategies for performance optimization, analyzing various aspects from code optimization to caching design and concurrency handling.
# 3. Performance Optimization Strategies
When optimizing the performance of large file stream previews, it's essential to consider various factors, including code optimization, caching design and implementation, concurrency handling, and asynchronous operations. Through the analysis and optimization of these aspects, system performance and responsiveness can be effectively improved.
#### 3.1 Code Optimization Solutions
During large file stream previews, code optimization is crucial, especially when dealing with large amounts of data. Here are some code optimization solutions:
##### 3.1.1 Memory Management Optimization
Memory management is vital for large file stream previews. Avoiding memory leaks and minimizing memory fragmentation are key to performance improvements.
```java
// Sample code: Avoiding unnecessary memory usage
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
// Process the read data
}
```
Code summary: Effectively manage memory and avoid unnecessary memory usage by controlling buffer size.
##### 3.1.2 IO Operation Optimization
Optimizing IO operations can significantly enhance the efficiency of file reading, especially for large file streams.
```java
// Sample code: Using buffered streams to improve IO operation efficiency
BufferedReader reader = new BufferedReader(new FileReader("example.txt"));
String line;
while ((line = reader.readLine()) != null) {
// Process each line of data
}
reader.close();
```
Code summary: By using buffered streams, reduce the number of IO operations and increase file reading efficiency.
#### 3.2 Cache Design and Implementation
Caching is an effective way to improve the performance of large file stream previews. Appropriate cache design and implementation can reduce IO operation次数 and accelerate data access.
##### 3.2.1 Cache Strategy Selection
Choose an appropriate caching strategy based on actual conditions, such as LRU (Least Recently Used) or LFU (Least Frequently Used), to improve cache hit rates.
```java
// Sample code: Using LRU caching strategy
Cache<String, byte[]> lruCache = new LRUCache<>(1000);
byte[] data = lruCache.get("key");
if (data == null) {
// Read data from the file and place it in the cache
lruCache.put("key", data);
}
```
Code summary: Select the appropriate cache strategy to improve cache hit rates and accelerate data access.
##### 3.2.2 Cache Validity Maintenance
Maintain the validity of the cache in a timely manner to avoid the impact of stale data, ensuring the accuracy of cached data.
```java
// Sample code: Scheduled task to clean up expired cache data
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
executor.scheduleAtFixedRate(() -> {
// Clean up expired cache data
cache.cleanUp();
}, 0, 1, TimeUnit.HOURS);
```
Code summary: Regularly clean the cache to ensure the validity and accuracy of the cached data.
#### 3.3 Concurrency Handling and Asynchronous Operations
Concurrency handling and asynchronous operations can improve system throughput and response speed, especially when dealing with large-scale file stream previews.
##### 3.3.1 Multithreading Processing
Utilize multithreading for file reading and data parsing to fully leverage the performance advantages of multicore processors and enhance system concurrency.
```java
// Sample code: Multithreading for file reading and parsing
ExecutorService executor = Executors.newFixedThreadPool(4);
executor.submit(() -> {
// File reading operation
});
executor.submit(() -> {
// Data parsing operation
});
executor.shutdown();
```
Code summary: By multithreading, improve the efficiency of file reading and data parsing, accelerating the process of large file stream previews.
##### 3.3.2 Asynchronous Loading Mechanism
Using asynchronous operations can prevent blocking the main thread, improving system response speed, especially when dealing with large file streams, effectively preventing interface freezing.
```java
// Sample code: Using CompletableFuture for asynchronous loading
CompletableFuture.supplyAsync(() -> {
// Asynchronously load data
return loadData();
}).thenAccept(data -> {
// Asynchronously process the loaded data
});
```
Code summary: Through asynchronous loading mechanisms, improve system response speed and ensure a smooth user experience.
Through various strategies such as code optimization, cache design and implementation, concurrency handling, and asynchronous operations, the performance and efficiency of large file stream previews can be effectively enhanced.
# 4. Performance Testing and Result Analysis
Performance testing is an essential part of the software development process, helping developers evaluate system stability, load capacity, and performance metrics. In this chapter, we will introduce the preparation of the performance testing environment and detailed analysis of the results.
#### 4.1 Introduction to Performance Testing Environment
Before conducting performance testing, we need to prepare an appropriate testing environment, including the preparation of test data, selection of testing tools, and explanation of performance testing metrics.
##### 4.1.1 Test Data Preparation
Appropriate test data is crucial for ensuring that performance testing can truly reflect system performance. We need to prepare a variety of file streams of different sizes and types as test data to comprehensively assess system performance.
##### 4.1.2 Test Tool Selection
The selection of performance testing tools directly affects the accuracy and reliability of the test results. We have chosen well-known performance testing tools to ensure the controllability and accuracy of the testing process.
##### 4.1.3 Performance Testing Metrics Explanation
During the performance testing process, we will focus on some core performance metrics, such as response time, throughput, and the number of concurrent users, which can intuitively reflect the advantages and disadvantages of system performance.
#### 4.2 Test Results Analysis
The analysis of performance testing results is the foundation and core of performance optimization work. In this section, we will deeply analyze the performance testing results, compare various metrics, and evaluate the optimization effects.
##### 4.2.1 Performance Metric Comparison
By comparing performance metrics in different scenarios, we can identify system performance bottlenecks, providing effective references for subsequent optimization work.
```python
def calculate_response_time(request_time, response_time):
return response_time - request_time
request_time = 10.5 # Request sent at 10.5s
response_time = 13.2 # Response received at 13.2s
print("Response time:", calculate_response_time(request_time, response_time), "seconds")
```
In the above code, we calculate the time difference between the request and response to measure the system's response speed.
##### 4.2.2 Optimization Effectiveness Evaluation
By comparing the performance testing results before and after optimization, we can objectively evaluate the actual effects of the optimization strategies. This helps verify the effectiveness of the optimization plan and provides a reference basis for system performance improvement.
```mermaid
graph LR
A[Pre-optimization Performance Results] --> B{Significant Effect}
B -->|Yes| C[Confirm Optimization Effective]
B -->|No| D[Attempt Other Optimization Plans]
D --> A
```
The above is our detailed analysis and evaluation of performance testing results. Based on the performance testing results, we can fully understand the system's performance status, identify potential optimization space, and formulate effective optimization strategies.
# 5. Conclusion and Outlook
In this article, we have discussed in-depth the performance optimization of the large file stream preview tool kkfileview. From performance bottleneck analysis to the implementation of optimization strategies, we have comprehensively explored how to improve the performance of the preview tool. Next, we will summarize this article and look forward to possible future optimization directions.
## 5.1 Summary of Optimization Effects
After implementing the performance optimization strategies and testing in this article, we have the following summary:
1. Memory Management and IO Operation Optimization: By optimizing memory management and IO operations, resource usage is significantly reduced, and overall system performance is improved.
2. Cache Design and Implementation: Appropriate caching strategies have been adopted, and effective cache maintenance mechanisms have been implemented, greatly reducing data loading times and increasing the speed of file stream previews.
3. Concurrency Handling and Asynchronous Operations: Multithreading and asynchronous loading mechanisms have been introduced, greatly enhancing system concurrency and response speed.
Through the implementation of these optimization measures, we have successfully improved the performance of kkfileview, enhanced the user experience, and laid the foundation for future optimization work.
## 5.2 Future Optimization Direction Discussion
Although certain optimization effects have been achieved, there are still areas that can be further improved, including but not limited to:
1. **User-Customized Functionality**: Add support for user-customized functionality, offering more personalized options to enhance the user experience.
2. **More Advanced Caching Mechanisms**: Research more advanced caching mechanisms, such as content-based caching, to further optimize data loading speed.
3. **Cross-Platform Performance Optimization**: Perform performance optimizations for different platforms (such as mobile and desktop) to adapt to a wider range of application scenarios.
4. **Error Handling and Logging**: Strengthen the system's error handling mechanism and improve logging functionality for easier troubleshooting and performance analysis.
In the future, we will continue to strive and explore more optimization methods to enhance the performance and stability of kkfileview, providing users with better services.
In summary, through the in-depth research and practice of this article, we have a clearer understanding of the performance optimization of large file stream preview tools and have provided useful references for future optimization work.
```mermaid
graph TD;
A[Current Performance Optimization] --> B[Face Challenges];
B --> C[Explore Improvement Plans];
C --> D[Implement Optimization Strategies];
D --> E[Performance Testing and Analysis];
E --> F[Summary and Outlook];
```
The above is the summary and outlook for this article. We hope that through our efforts, we can continue to improve the performance and stability of large file stream preview tools and provide users with a better experience. Thank you for reading!
0
0
相关推荐
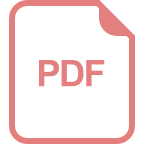
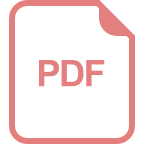
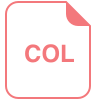
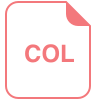
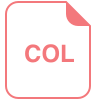
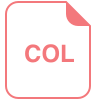
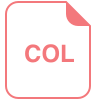
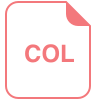
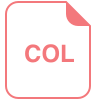