使用Spring Boot 2.0实现身份验证和授权
发布时间: 2023-12-20 13:19:40 阅读量: 10 订阅数: 11 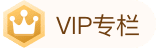
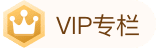
# 第一章:介绍Spring Boot 2.0和身份验证授权
## 1.1 什么是Spring Boot 2.0
Spring Boot 2.0是一个用于构建独立的、生产级的Spring应用程序的框架。它采用“约定优于配置”的原则,可以快速启动和运行应用程序,并集成了大量常用的功能,包括对身份验证和授权的支持。
## 1.2 身份验证和授权的重要性
身份验证和授权是现代应用程序不可或缺的部分。身份验证用于确认用户的身份,而授权则确定用户可以访问的资源和操作。良好的身份验证和授权机制可以保护应用程序免受未经授权的访问和恶意攻击。
## 1.3 Spring Boot 2.0对身份验证和授权的改进
### 2. 第二章:配置Spring Security实现基本身份验证
在本章中,我们将介绍如何配置Spring Security来实现基本的身份验证。身份验证是应用程序安全的基础,它可以确保只有经过授权的用户可以访问受保护的资源。通过Spring Security,我们可以轻松地实现基本的用户名和密码验证,并且可以根据需要进行自定义用户存储和认证配置。让我们一起来看看如何配置Spring Security来保护我们的应用程序。
#### 2.1 添加Spring Security依赖
首先,我们需要在我们的Spring Boot项目中添加Spring Security的依赖。在`pom.xml`文件中,添加以下依赖项:
```xml
<dependencies>
<!-- 其他依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
</dependencies>
```
这将引入Spring Security starter依赖,使我们能够轻松地集成Spring Security到我们的应用程序中。
#### 2.2 配置基本的身份验证
接下来,我们需要配置Spring Security来实现基本的身份验证。在我们的Spring Boot应用程序的主配置类中,添加`@EnableWebSecurity`注解,并继承`WebSecurityConfigurerAdapter`类。然后,重写`configure(HttpSecurity http)`方法来配置基本的身份验证。
```java
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
```
在上面的示例中,我们启用了基本的Web安全功能,并且要求所有请求都需要经过身份验证。我们还配置了使用HTTP基本认证(Basic Authentication)来进行身份验证。
#### 2.3 自定义用户存储和认证配置
如果我们想要使用自定义的用户存储和认证配置,我们可以扩展`WebSecurityConfigurerAdapter`类,并重写`configure(AuthenticationManagerBuilder auth)`方法来配置认证管理器。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class CustomSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("USER", "ADMIN");
}
}
```
在上述示例中,我们使用了内存中的用户存储,并为用户“user”和“admin”设置了密码和角色。在实际应用中,我们可以使用JDBC、LDAP等实际的用户存储方式,并根据需求进行配置。
### 第三章:使用JWT实现身份验证和授权
在本章中,我们将探讨如何使用JWT(JSON Web Token)实现身份验证和授权。我们将介绍JWT的基本概念,讨论如何将JWT集成到Spring Boot应用程序中,并演示如何实现基于角色的访问控制。
#### 3.1 什么是JWT(JSON Web Token)
0
0
相关推荐
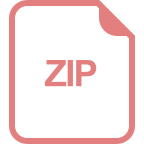
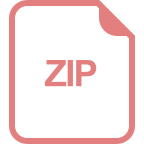
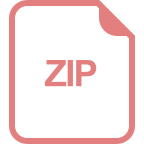
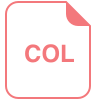
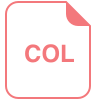
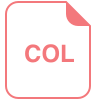
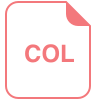

