【django.utils.text必备技能】:字符串长度控制与预处理技巧全面剖析
发布时间: 2024-10-06 20:09:45 阅读量: 24 订阅数: 14 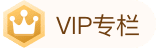
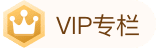
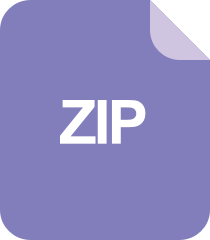
Java-美妆神域_3rm1m18i_221-wx.zip
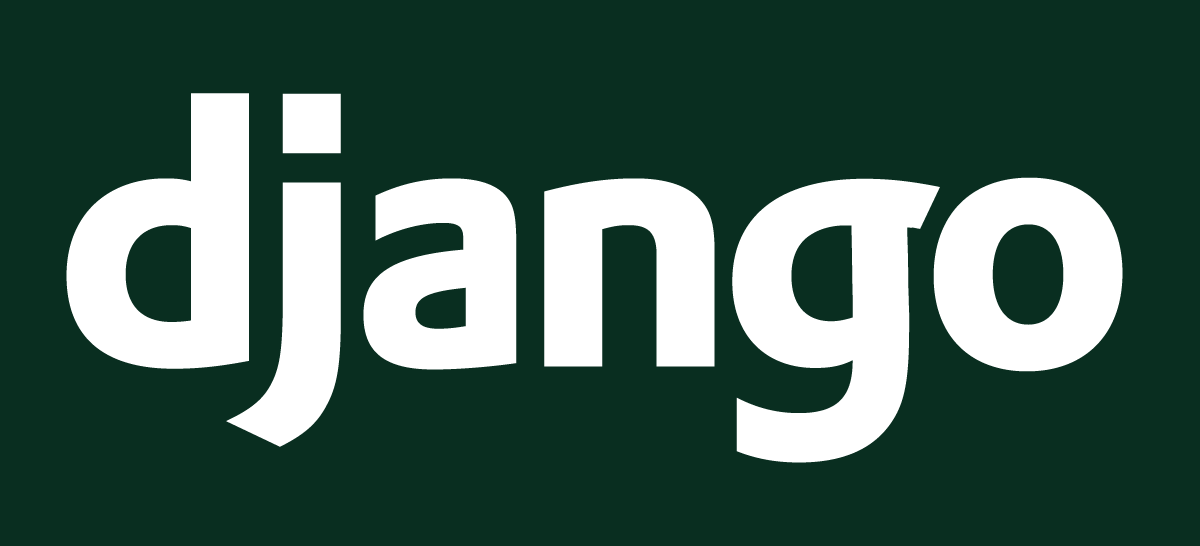
# 1. Django与django.utils.text模块简介
在Web开发的世界中,Django框架以其快速开发和安全性的特性,赢得了广泛的应用。而django.utils.text模块,作为Django框架的一部分,提供了一系列实用的文本处理工具,它们对于开发中的文本处理需求提供了解决方案,从而使得开发者可以更加专注于业务逻辑的实现。
本章将带领读者初步认识Django以及django.utils.text模块,重点介绍django.utils.text模块的基础功能和应用场景。我们不仅会概述Django框架,还会详细解析django.utils.text模块中一些核心函数的工作原理,为后续章节中深入探讨模块的高级应用打下坚实的基础。
```python
# 示例代码:Django与django.utils.text模块引入
import django
from django.utils.text import Truncator
# 输出Django版本信息
print(django.get_version())
# 创建一个Truncator对象,并演示基本使用
truncator = Truncator('This is a very long text that needs to be truncated.')
print(truncator.words(5))
```
在本章结束时,读者应能理解django.utils.text模块如何简化文本处理流程,以及如何在实际项目中应用其中的函数。接下来的章节,我们将进一步深入探讨字符串长度控制、预处理技巧和进阶操作等主题,以便充分掌握django.utils.text模块的全部潜力。
# 2. 字符串长度控制
在现代Web开发中,对数据的呈现往往需要进行严格的格式控制,特别是在文本内容展示上,我们需要确保用户界面的整洁性和可读性。字符串长度控制是确保内容展示得当的关键技术之一。在本章节中,我们将深入探讨Django框架提供的字符串长度控制方法以及django.utils.text模块中的相关工具。
## 2.1 Django中字符串长度的标准衡量
### 2.1.1 字符串长度的计算方法
字符串长度的计算是文本处理中最为基础的操作之一。在Python中,长度通常是指字符串中字符的个数。可以通过内置的`len()`函数来获取字符串的长度。该函数返回字符串中的字符总数,包括字母、数字、符号以及空格。例如:
```python
text = "Hello, Django!"
length = len(text)
print(length) # 输出: 14
```
在Web开发中,我们也经常需要按单词或按段落来计算长度。为了实现这一点,我们可以使用`split()`方法将字符串分割成单词列表,然后计算列表的长度。同样地,按段落计算时,可以使用`split('\n')`方法。
### 2.1.2 Django内置的字符串长度控制函数
Django提供了一些内置函数,以支持开发者对字符串长度进行便捷控制。这些函数有助于在不破坏内容语义的前提下,进行适当的裁剪和格式化。
在Django中,`truncate_words()`函数用于按单词数截断文本。例如:
```python
from django.utils.html import truncate_words
long_text = "This is an example of a long string that needs to be truncated."
truncated_text = truncate_words(long_text, 5)
print(truncated_text) # 输出: This is an example of a...
```
另一个有用的函数是`truncatechars()`, 它按照指定的字符数截断字符串。它确保即使截断的位置在字符的中间,也会从字符的开始处截断,以避免产生不完整字符。
```python
from django.utils.html import truncatechars
long_text = "This is an example of a long string that needs to be truncated."
truncated_text = truncatechars(long_text, 30)
print(truncated_text) # 输出: This is an example of a...
```
## 2.2 django.utils.text模块中的长度控制工具
### 2.2.1 Truncator类的使用技巧
django.utils.text模块中的Truncator类提供了灵活的字符串截断能力。与简单的函数相比,Truncator类不仅支持字符串长度的控制,还提供了额外的功能,如在截断位置添加省略标记。
创建Truncator实例后,可以使用`append()`方法添加省略标记,并使用`chars()`或`words()`方法进行截断:
```python
from django.utils.text import Truncator
text = "This is an example of a long string that needs to be truncated."
truncator = Truncator(text)
# 截断字符并添加省略标记
truncated_text = truncator.chars(30, "...")
print(truncated_text) # 输出: This is an example of...
# 截断单词并添加省略标记
truncated_text = truncator.words(5, "...")
print(truncated_text) # 输出: This is an example...
```
### 2.2.2 案例:动态文本摘要的实现
在实际项目中,动态生成文本摘要是一项常见的需求。例如,在一个博客平台上,当显示帖子列表时,我们希望每个帖子都能有适当的摘要来告知用户其主要内容。
利用Truncator类,我们可以轻松实现这一功能:
```python
from django.utils.text import Truncator
def generate_summary(post_content, word_limit=20, ellipsis="..."):
truncator = Truncator(post_content)
summary = truncator.words(word_limit, ellipsis)
return summary
post_content = """Django is a high-level Python Web framework that encourages rapid development and clean, pragmatic design.
Developed by a fast-moving online-news operation, it was released publicly in July 2005."""
summary = generate_summary(post_content)
print(summary) # 输出: Django is a high-level Python Web framework that...
```
## 2.3 实践:构建动态内容显示系统
### 2.3.1 系统需求分析
在构建一个动态内容显示系统时,首要的是需求分析。我们需要考虑以下几点:
1. **内容的动态性**:系统应能根据内容的长度,动态地展示不同的摘要或全部内容。
2. **可读性**:内容需要呈现得易于阅读,避免过长的文本导致界面杂乱。
3. **用户交互**:可能需要提供一个选项,让用户决定是否查看完整内容。
### 2.3.2 实现动态内容摘要功能
在Django框架中,结合模板语言和视图逻辑,可以实现一个动态内容摘要系统。以下是视图中处理内容摘要的示例:
```python
from django.shortcuts import render
from django.utils.text import Truncator
def post_list(request):
# 假设我们有一个post列表
posts = get_posts_from_database()
for post in posts:
post.summary = generate_summary(post.content)
return render(request, 'post_list.html', {'posts': posts})
```
在HTML模板中,我们可以展示文本摘要或完整内容,具体取决于内容的实际长度或用户的选择:
```html
{% for post in posts %}
<div class="post">
<h2>{{ post.title }}</h2>
<p>{{ post.summary }}</p>
<a href="{{ post.get_absolute_url }}">Read More...</a>
</div>
{% endfor %}
```
这种动态内容显示系统既满足了需求,也提升了用户体验。
以上便是第二章节的内容。在下一章节,我们将深入探讨字符串预处理技巧,这是确保Web应用中数据准确性和有效性的另一个重要话题。
# 3. 字符串预处理技巧
字符串预处理是编程中常见的步骤,尤其在处理用户输入或从外部数据源读取数据时。在本章节中,我们将深入了解如何使用Python以及django.utils.text模块来净化和准备字符串数据。
## 3.1 去除字符串中的空白字符
空白字符不仅包括空格,还包括制表符、换行符等。在处理文本数据时,这些空白字符可能会导致格式问题,因此,清除字符串中的空白字符变得尤为重要。
### 3.1.1 常用的去除空白的函数
Python标准库提供了一系列简单的字符串方法来去除空白字符,比如`strip()`, `rstrip()`, `lstrip()`。`strip()`方法能够去除字符串首尾的空白字符,而`rstrip()`和`lstrip()`则分别去除字符串尾部和首部的空白字符。
```python
text = " Hello, World! "
stripped_text = text.strip()
print(stripped_text) # 输出: "Hello, World!"
```
在django.utils.text模块中,虽然没有直接提供去除空白的函数,但是模块中提供了更加强大的文本处理工具,这些工具同样可以用于去除字符串中的空白字符。
### 3.1.2 去除字符串首尾空白的案例
在实际应用中,去除字符串首尾的空白字符是一个常
0
0
相关推荐
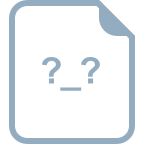
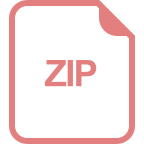
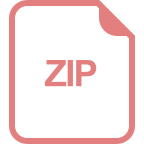
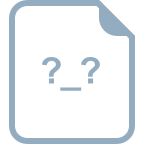
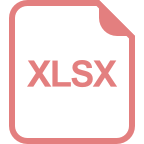