Java顺序表在并发与大数据场景中的应用解析:内存管理与性能挑战
发布时间: 2024-09-10 21:11:11 阅读量: 69 订阅数: 24 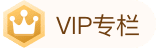
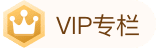
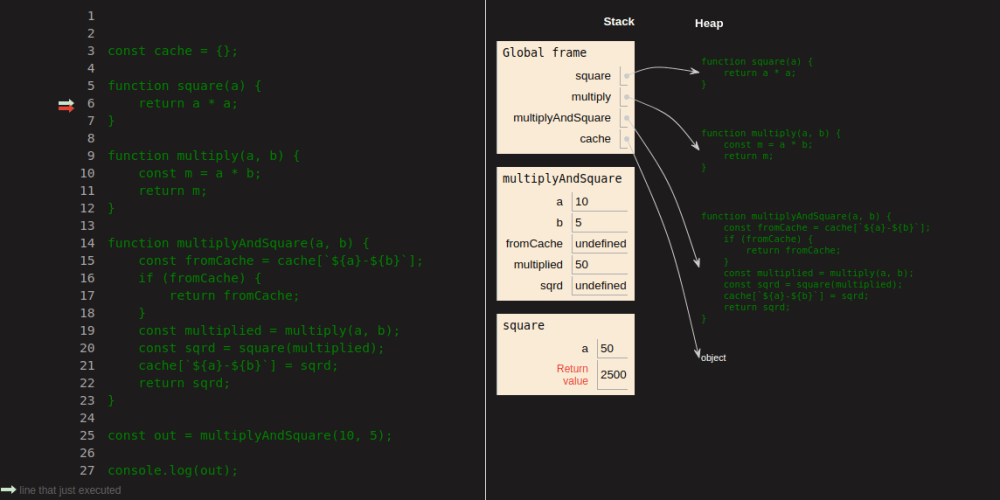
# 1. Java顺序表基础概述
在Java编程世界中,顺序表是一种常见的数据结构,它在内存中以连续的数组形式存在,通过索引即可快速访问任意元素。由于其数组的性质,顺序表的随机访问性能十分优越,但相对的,在进行插入或删除操作时,可能会导致数组元素的移动,这在大数据量操作时,效率较低。
顺序表主要由数组实现,它为开发者提供了简洁的API,如add、remove、get和set等方法,用于添加、删除、读取和更新元素。在理解顺序表的工作原理时,需要重视其容量和扩容机制,这直接关系到程序的性能表现。例如,当顺序表达到其容量上限时,通常需要创建一个新的、更大的数组,并将旧数组的内容复制到新数组中,这是一个耗费资源的操作,因此,合理预测并管理顺序表的容量是优化性能的重要环节。
此外,Java中的ArrayList类是顺序表的一个广泛使用的实现,其内部实现了动态数组的机制,使得开发者无需关注数组容量的管理。在本章接下来的内容中,我们将深入探讨顺序表的并发访问控制以及在大数据处理场景中的应用。通过本章的学习,读者将掌握顺序表的基本概念、特点及其在实际应用中的优势与挑战。
# 2. Java顺序表的并发访问控制
## 2.1 并发编程的基本概念
### 2.1.1 线程安全的基本原则
在多线程环境中,线程安全是一个核心问题。线程安全的代码能够确保在多线程环境下,对于共享资源的访问和修改是正确和同步的。理解线程安全的基本原则对于设计健壮的并发应用程序至关重要。
线程安全通常涉及到以下几个关键概念:
- **原子性**:指的是一个操作或者多个操作要么全部执行,要么全部不执行。
- **可见性**:一个线程对共享变量的修改,对其他线程是立即可见的。
- **有序性**:程序执行的顺序能够按照代码的先后顺序执行。
Java中的线程安全可以通过使用synchronized关键字、显式锁(如ReentrantLock)以及原子变量等机制来实现。在设计线程安全的代码时,应当尽量减少共享资源的范围,减少锁的竞争,提高程序的并发性能。
### 2.1.2 并发控制机制概述
并发控制机制是用来确保并发访问共享资源时数据的一致性和正确性。常见的并发控制机制包括互斥锁(Mutex)、读写锁(ReadWriteLock)、乐观锁和悲观锁等。每种机制都有其适用的场景,开发者需要根据具体需求选择合适的控制机制。
- **互斥锁**:确保多个线程在同一时间只有一个可以访问资源,适用于读写操作都需要保护的场景。
- **读写锁**:允许多个线程同时读取数据,但写入时必须独占访问。适用于读多写少的场景。
- **乐观锁与悲观锁**:乐观锁假设资源冲突较少,只有在提交更新时才检查数据是否被修改过。而悲观锁则假设资源冲突是常态,所以在数据访问时就加锁。
在选择并发控制机制时,应当考虑到性能、可伸缩性以及复杂性等多个因素,以达到最优的并发访问控制效果。
## 2.2 Java顺序表并发控制策略
### 2.2.1 锁的使用与优化
在Java中,锁是最常用的并发控制手段之一,它能够保证对共享资源的安全访问。synchronized关键字和显式锁(如ReentrantLock)是最常见的两种锁实现。
#### 使用synchronized关键字
synchronized关键字提供了一种简单的锁定机制,可以用来同步对方法或代码块的访问。下面是一个简单的示例代码:
```java
public class Counter {
private int count = 0;
public void increment() {
synchronized(this) {
count++;
}
}
public int getCount() {
synchronized(this) {
return count;
}
}
}
```
在这个例子中,increment方法和getCount方法都被synchronized关键字修饰,确保了同一时间只有一个线程可以执行。
#### 使用显式锁
显式锁ReentrantLock提供了比synchronized关键字更灵活的锁定机制,例如可以尝试获取锁但不阻塞线程。下面是一个使用ReentrantLock的示例:
```java
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class LockCounter {
private final Lock lock = new ReentrantLock();
private int count = 0;
public void increment() {
lock.lock();
try {
count++;
} finally {
lock.unlock();
}
}
public int getCount() {
lock.lock();
try {
return count;
} finally {
lock.unlock();
}
}
}
```
在上述代码中,通过调用lock.lock()和lock.unlock()来控制获取和释放锁。显式锁提供了更细粒度的控制,包括尝试锁定、非阻塞锁定和中断锁定等高级特性。
#### 锁优化技术
锁优化技术通常包括减少锁的持有时间、避免死锁和减少锁的竞争等。下面是一些常见的优化策略:
- **缩小锁的范围**:只在必要的代码块上加锁,减少锁的持有时间。
- **锁分离**:将不同功能的锁分离,如读写锁分离,允许读操作并行执行。
- **锁粗化**:对于频繁的临界区,将锁粗化可以减少锁切换的开销。
通过这些优化措施,可以在保证线程安全的同时,提高系统的并发性能。
### 2.2.2 无锁编程技术探索
无锁编程是一种实现线程安全的技术,它避免使用互斥锁,而是通过原子操作来保证数据的一致性。无锁编程的核心思想是使用原子操作来实现同步,常见的原子操作包括CAS(Compare-And-Swap)。
#### CAS操作
CAS是一种常见的无锁操作,它包含三个操作数:内存位置(V)、预期原值(A)和新值(B)。当且仅当内存位置的值与预期原值相匹配时,才会使用新值更新该位置的值。
Java中的AtomicInteger类就是利用CAS操作来实现线程安全的计数器:
```java
import java.util.concurrent.atomic.AtomicInteger;
public class NonBlockingCounter {
private final AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
```
在这个例子中,incrementAndGet()方法通过CAS来安全地增加计数器的值,而不需要使用传统的锁机制。
#### 无锁编程的挑战
虽然无锁编程可以提高性能,但也存在挑战:
- **ABA问题**:当一个值从A变成B,然后再变回A时,CAS操作无法感知中间的变化。
- **循环时间开销**:在高争用的情况下,不断尝试CAS操作可能会导致处理器时间开销增大。
- **无锁编程的适用性**:无锁编程通常适用于简单的原子操作,对于复杂的同步需求,可能难以实现或维护。
因此,在设计无锁结构时,需要权衡其优势和潜在的问题,以决定是否适用。
### 2.2.3 读写锁的应用场景
读写锁(ReadWriteLock)是一种特殊的锁,它允许多个读取操作并行执行,但在写入操作时,所有的读取和写入操作都必须等待。读写锁适用于读多写少的场景,能够显著提高系统的并发性能。
#### 使用ReadWriteLock
ReadWriteLock接口有两个主要实现:ReentrantReadWriteLock和StampedLock。下面是一个使用ReentrantReadWriteLock的例子:
```java
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class Cache {
private final ReadWriteLock readWriteLock = new ReentrantReadWriteLock();
private String cache;
public void readCache() {
readWriteLock.readLock().lock();
```
0
0
相关推荐
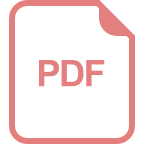
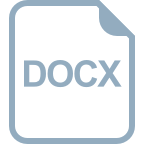
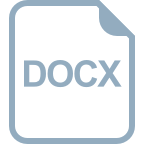
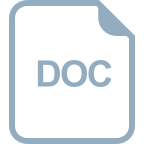
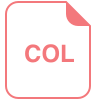
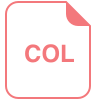
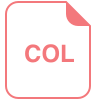
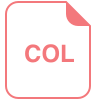
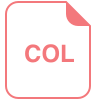