NIO中的数据加密与解密
发布时间: 2024-01-09 12:13:04 阅读量: 33 订阅数: 34 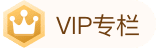
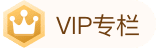
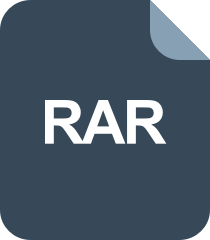
加密和解密
# 1. 简介
## 1.1 什么是NIO
NIO(New Input/Output)是Java中的非阻塞式IO。传统的Java IO是基于流(stream)的,每次读取或写入数据都会阻塞线程,直到完成操作。而NIO基于缓冲区(buffer)和通道(channel)的概念,通过选择器(selector)实现了多路复用。这样可以在一个线程中处理多个客户端连接,并提高IO操作的效率。
NIO有许多优点,例如更高的性能、更少的线程需求和更好的扩展性。因此,它在网络编程、服务器编程等领域得到了广泛应用。
## 1.2 数据加密与解密的重要性
数据加密和解密是信息安全领域的关键技术。随着互联网的发展,我们越来越需要保护数据的机密性和完整性,以防止数据被窃取、篡改或伪造。
加密是指将原始数据转化为一段看似随机的密文,只有持有密钥的人才能解密还原成原始数据。解密则是将密文还原为原始数据。通过使用加密算法,我们可以确保数据在传输过程中不被非法访问和篡改。
在本篇文章中,我们将介绍一些常用的加密算法,以及如何使用这些算法来实现NIO中的数据加密与解密。同时,我们还会探讨数字签名的概念以及加密传输协议的使用方法。
# 2. 对称加密算法
对称加密算法是一种使用相同的密钥对数据进行加密和解密的算法。在NIO中,我们可以使用AES算法来实现数据加密与解密。
### 2.1 AES算法原理
AES(Advanced Encryption Standard)是一种对称加密算法,它可以使用128位、192位或256位的密钥来加密数据。AES算法使用替代和置换操作,以及逐位行变换,来实现高级的数据加密。
AES算法的加密过程如下:
1. 将明文按照128位进行分块。
2. 将密钥扩展到和明文分块相同的长度。
3. 进行多轮的替代、置换和行变换操作。
4. 输出密文。
### 2.2 使用AES实现NIO数据加密与解密
下面是使用AES算法在Java中实现NIO数据加密与解密的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.security.Key;
public class AESExample {
private static final String ALGORITHM = "AES";
private static final String KEY = "ThisIsASecretKey";
public static void main(String[] args) throws Exception {
// 加密文件
encryptFile("plain.txt", "encrypted.txt");
// 解密文件
decryptFile("encrypted.txt", "decrypted.txt");
}
public static void encryptFile(String inputFile, String outputFile) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
Key secretKey = new SecretKeySpec(KEY.getBytes(), ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
FileChannel inputChannel = FileChannel.open(Paths.get(inputFile), StandardOpenOption.READ);
FileChannel outputChannel = FileChannel.open(Paths.get(outputFile), StandardOpenOption.CREATE, StandardOpenOption.WRITE);
ByteBuffer buffer = ByteBuffer.allocate(1024);
while (inputChannel.read(buffer) > 0) {
buffer.flip();
byte[] encryptedBytes = cipher.update(buffer.array());
outputChannel.write(ByteBuffer.wrap(encryptedBytes));
buffer.clear();
}
byte[] finalBytes = cipher.doFinal();
outputChannel.write(ByteBuffer.wrap(finalBytes));
inputChannel.close();
outputChannel.close();
}
public static void decryptFile(String inputFile, String outputFile) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
Key secretKey = new SecretKeySpec(KEY.getBytes(), ALGORITHM);
cipher.ini
```
0
0
相关推荐
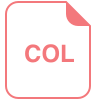
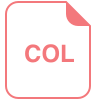
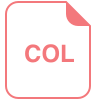
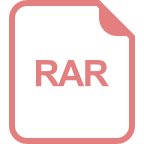
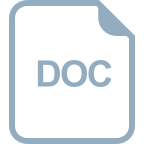
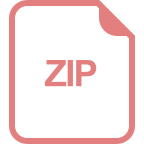