如何在Spring框架中使用注解
发布时间: 2024-02-21 16:25:19 阅读量: 13 订阅数: 16 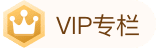
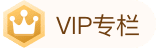
# 1. Spring框架简介
## 1.1 Spring框架概述
Spring是一个轻量级的开源框架,用于构建企业级应用程序。它提供了全面的基础设施支持,包括依赖注入、面向切面编程、事务管理等功能,使开发人员能够专注于业务逻辑的开发,而不是处理底层的应用程序配置。
## 1.2 Spring框架的核心功能
Spring框架的核心功能包括:
- **依赖注入(Dependency Injection)**:将对象之间的依赖关系交给容器管理,降低组件之间的耦合度。
- **面向切面编程(Aspect Oriented Programming)**:通过AOP实现横切关注点,例如日志、事务管理等。
- **事务管理**:Spring提供了声明式的事务管理,简化了事务管理的操作。
- **模块化**:Spring框架是模块化的,可以根据需要选择使用的模块,如Spring MVC、Spring Boot等。
## 1.3 Spring框架中注解的作用
在Spring框架中,注解是一种使用频率非常高的特性,通过注解能够简化开发过程,使得代码更加清晰易懂。注解可以用来替代XML配置文件,使得配置更加便捷灵活。
接下来我们将深入探讨Spring框架中各种常用注解的用途和具体应用场景。
# 2. Spring框架中的常用注解
### 2.1 @Component注解
在Spring框架中,`@Component`注解用于将类标识为Spring组件,让Spring能够扫描到并将其实例化为bean。这样我们就可以使用`@Autowired`注解进行自动注入。
```java
@Component
public class MyComponent {
// Class implementation
}
```
### 2.2 @Autowired注解
`@Autowired`注解可以用来自动装配bean,它可以在属性、构造函数或方法上使用。以下是一个示例,其中`MyComponent`会被自动注入到`myService`中。
```java
@Service
public class MyService {
@Autowired
private MyComponent myComponent;
// Class implementation
}
```
### 2.3 @Controller、@Service、@Repository注解
这些注解是`@Component`的特化,用于分别标识控制器、服务、数据访问对象。它们和`@Component`的作用是一样的,只不过是为了更好地表达组件的用途,使代码更加清晰。
```java
@Controller
public class MyController {
// Class implementation
}
@Service
public class MyService {
// Class implementation
}
@Repository
public class MyRepository {
// Class implementation
}
```
### 2.4 @Configuration注解
`@Configuration`注解用于标识配置类,它是Spring容器中的一种特殊的bean,用来配置`@Bean`实例。我们可以使用`@Bean`注解来注册bean,Spring容器会根据这个配置类来加载bean定义。
```java
@Configuration
public class MyConfig {
@Bean
public MyService myService() {
return new MyService();
}
}
```
这是Spring框架中常用注解的简要介绍。在下一章节中,我们将详细讲解如何在Spring中使用这些注解。
# 3. 如何在Spring中使用注解
在Spring框架中,注解是一种方便快捷的方式来配置和管理应用程序组件。通过注解,可以简化配置文件并提高代码的可读性和可维护性。本章将介绍如何在Spring中使用注解来完成各种任务。
#### 3.1 基本的注解使用方法
在Spring中,使用注解需要先开启注解扫描功能,通常在配置文件中添加以下配置:
```xml
<context:component-scan base-package="com.example"/>
```
然后就可以在组件上使用注解了,比如@Component、@Service、@Controller等。基本的注解使用方法如下:
```java
@Component
public class UserService {
// 类代码
}
@Service
public class UserRepository {
// 类代码
}
```
#### 3.2 在类、属性、方法上使用注解
除了在类上使用注解外,还可以在属性和方法上使用注解来完成更细粒度的配置,比如@Autowired、@Value等。示例代码如下:
```java
@Component
public class UserController {
@Autowired
private UserService userService;
@
```
0
0
相关推荐
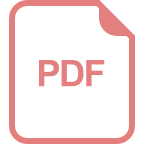
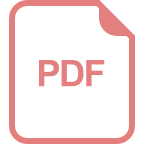
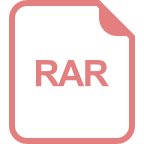





