C#中安全认证与授权机制的实现与加固
发布时间: 2024-05-01 21:47:52 阅读量: 94 订阅数: 57 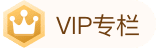
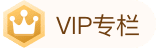
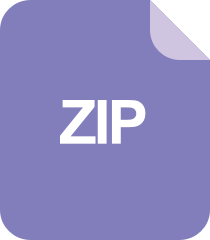
基于C#的软件加密、授权与注册
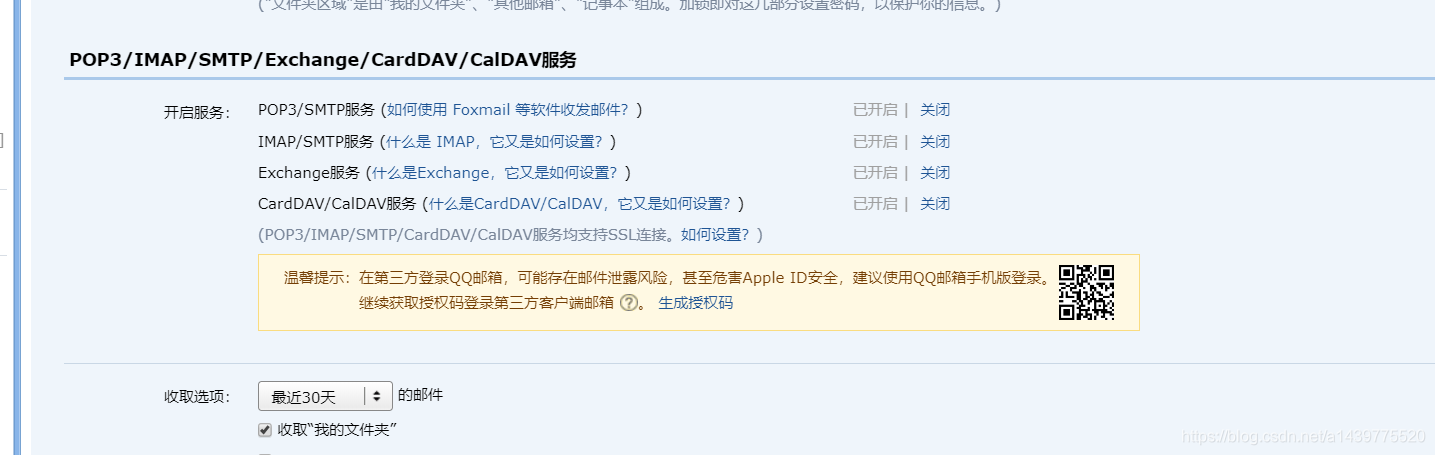
# 1.1 C#安全认证与授权机制概述
C#安全认证与授权机制是保护应用程序免受未经授权访问和操作的关键组成部分。认证是验证用户身份的过程,而授权是确定用户是否有权执行特定操作的过程。
C#中提供了各种认证和授权机制,包括:
- 表单身份验证:使用HTML表单收集用户凭据,并将其与存储在应用程序中的凭据进行比较。
- Cookie身份验证:在用户浏览器中存储加密的令牌,用于在后续请求中识别用户。
- OAuth身份验证:允许用户使用第三方服务(如Google或Facebook)登录应用程序,而无需创建单独的帐户。
# 2. C# 认证机制的实现
### 2.1 身份验证方法
身份验证是验证用户身份的过程,以确保只有授权用户才能访问系统。C# 中有几种身份验证方法可供选择:
#### 2.1.1 表单身份验证
表单身份验证是最简单的身份验证方法,它使用 HTML 表单收集用户的用户名和密码。服务器验证这些凭据,如果有效,则创建身份验证 cookie 并将其发送到客户端。
**代码块:**
```csharp
public ActionResult Login(LoginViewModel model)
{
if (ModelState.IsValid)
{
var user = _userService.Authenticate(model.Username, model.Password);
if (user != null)
{
FormsAuthentication.SetAuthCookie(user.Username, model.RememberMe);
return RedirectToAction("Index", "Home");
}
else
{
ModelState.AddModelError("", "Invalid username or password");
}
}
return View(model);
}
```
**逻辑分析:**
此代码块显示了表单身份验证的实现。它接收一个 LoginViewModel,其中包含用户名和密码。如果模型有效,它调用 _userService.Authenticate() 方法来验证凭据。如果凭据有效,则创建身份验证 cookie 并将其发送到客户端。
#### 2.1.2 Cookie 身份验证
Cookie 身份验证使用存储在客户端浏览器中的 cookie 来跟踪用户身份。当用户登录时,服务器会创建并发送一个身份验证 cookie。每次用户访问受保护的资源时,浏览器都会发送该 cookie,服务器会使用它来验证用户的身份。
**代码块:**
```csharp
public ActionResult Login(LoginViewModel model)
{
if (ModelState.IsValid)
{
var user = _userService.Authenticate(model.Username, model.Password);
if (user != null)
{
var identity = new ClaimsIdentity(new[] {
new Claim(ClaimTypes.Name, user.Username),
new Claim(ClaimTypes.Role, user.Role)
}, CookieAuthenticationDefaults.AuthenticationScheme);
var principal = new ClaimsPrincipal(identity);
var authProperties = new AuthenticationProperties
{
IsPersistent = model.RememberMe
};
HttpContext.SignInAsync(CookieAuthenticationDefaults.AuthenticationScheme, principal, authProperties);
return RedirectToAction("Index", "Home");
}
else
```
0
0
相关推荐







