【单片机C语言程序设计入门】:零基础快速掌握单片机开发
发布时间: 2024-07-09 03:06:16 阅读量: 74 订阅数: 32 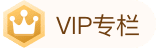
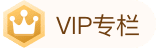
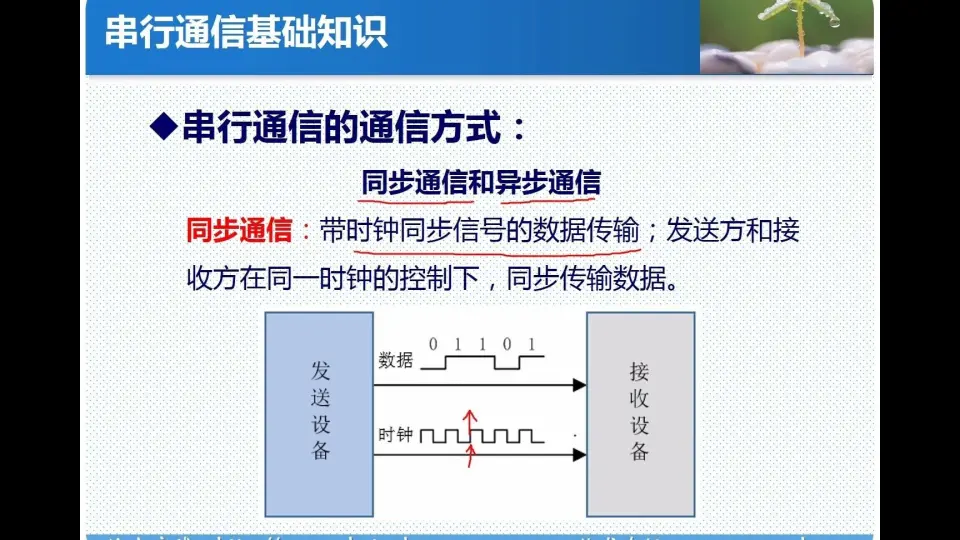
# 1. 单片机C语言简介**
单片机C语言是一种嵌入式系统编程语言,专为资源受限的单片机系统而设计。它是一种高级语言,具有结构化、模块化和可移植性等特点,可以有效提高单片机系统的开发效率和代码质量。
单片机C语言基于ANSI C标准,但针对单片机的特点进行了优化,增加了对寄存器、中断和低级硬件操作的支持。它提供了丰富的库函数和宏定义,方便开发者快速开发应用程序。
与汇编语言相比,单片机C语言具有更高的抽象性,更接近自然语言,更容易理解和维护。同时,它还支持结构化编程和模块化设计,可以有效降低程序的复杂度和提高可读性。
# 2. 单片机C语言基础语法
### 2.1 数据类型和变量
#### 2.1.1 基本数据类型
单片机C语言中提供了多种基本数据类型,用于表示不同的数据。主要包括:
- **整型:**int、short、long,用于表示整数。
- **浮点型:**float、double,用于表示小数。
- **字符型:**char,用于表示单个字符。
**代码块:**
```c
int age = 25; // 声明一个整型变量 age 并初始化为 25
float temperature = 37.5; // 声明一个浮点型变量 temperature 并初始化为 37.5
char letter = 'A'; // 声明一个字符型变量 letter 并初始化为 'A'
```
**逻辑分析:**
- `age` 变量存储了一个整数值 25,表示年龄。
- `temperature` 变量存储了一个浮点数值 37.5,表示温度。
- `letter` 变量存储了一个字符值 'A',表示字母 A。
#### 2.1.2 变量的定义和使用
变量用于存储数据,其定义语法为:
```c
数据类型 变量名;
```
**代码块:**
```c
int number; // 定义一个整型变量 number
float price; // 定义一个浮点型变量 price
char name[20]; // 定义一个字符数组 name,最多可存储 20 个字符
```
**逻辑分析:**
- `number` 变量可以存储整数值。
- `price` 变量可以存储浮点数值。
- `name` 变量可以存储一个字符串,最多包含 20 个字符。
### 2.2 运算符和表达式
#### 2.2.1 算术运算符
算术运算符用于执行算术运算,包括:
- `+`:加法
- `-`:减法
- `*`:乘法
- `/`:除法
- `%`:取模
**代码块:**
```c
int result = 10 + 5; // 加法
int difference = 15 - 7; // 减法
int product = 3 * 4; // 乘法
int quotient = 12 / 3; // 除法
int remainder = 10 % 3; // 取模
```
**逻辑分析:**
- `result` 变量存储了 10 和 5 的和,即 15。
- `difference` 变量存储了 15 和 7 的差,即 8。
- `product` 变量存储了 3 和 4 的积,即 12。
- `quotient` 变量存储了 12 除以 3 的商,即 4。
- `remainder` 变量存储了 10 除以 3 的余数,即 1。
#### 2.2.2 关系运算符
关系运算符用于比较两个表达式的值,包括:
- `==`:等于
- `!=`:不等于
- `<`:小于
- `>`:大于
- `<=`:小于等于
- `>=`:大于等于
**代码块:**
```c
int a = 10;
int b = 5;
bool equal = (a == b); // 比较 a 和 b 是否相等
bool notEqual = (a != b); // 比较 a 和 b 是否不相等
bool lessThan = (a < b); // 比较 a 是否小于 b
bool greaterThan = (a > b); // 比较 a 是否大于 b
bool lessThanOrEqual = (a <= b); // 比较 a 是否小于或等于 b
bool greaterThanOrEqual = (a >= b); // 比较 a 是否大于或等于 b
```
**逻辑分析:**
- `equal` 变量存储了 `a` 和 `b` 是否相等的布尔值,即 `false`。
- `notEqual` 变量存储了 `a` 和 `b` 是否不相等的布尔值,即 `true`。
- `lessThan` 变量存储了 `a` 是否小于 `b` 的布尔值,即 `false`。
- `greaterThan` 变量存储了 `a` 是否大于 `b` 的布尔值,即 `true`。
- `lessThanOrEqual` 变量存储了 `a` 是否小于或等于 `b` 的布尔值,即 `false`。
- `greaterThanOrEqual` 变量存储了 `a` 是否大于或等于 `b` 的布尔值,即 `true`。
### 2.3 流程控制语句
#### 2.3.1 条件语句
条件语句用于根据条件执行不同的代码块,包括:
- `if` 语句:如果条件为真,则执行代码块。
- `else` 语句:如果条件为假,则执行代码块。
- `else if` 语句:如果条件为真,则执行代码块,否则执行 `else` 语句。
**代码块:**
```c
int age = 18;
if (age >= 18) {
// 如果 age 大于或等于 18,则执行此代码块
printf("您已成年。\n");
} else {
// 如果 age 小于 18,则执行此代码块
printf("您未成年。\n");
}
```
**逻辑分析:**
- 如果 `age` 变量的值大于或等于 18,则打印 "您已成年。"。
- 否则,打印 "您未成年。"。
#### 2.3.2 循环语句
循环语句用于重复执行代码块,包括:
- `while` 循环:只要条件为真,就重复执行代码块。
- `do-while` 循环:先执行代码块,然后检查条件是否为真,如果为真,则重复执行代码块。
- `for` 循环:使用一个计数器变量来控制循环的次数。
**代码块:**
```c
int i = 0;
while (i < 10) {
// 只要 i 小于 10,就重复执行此代码块
printf("%d\n", i);
i++;
}
```
**逻辑分析:**
- 初始化一个计数器变量 `i` 为 0。
- 只要 `i` 小于 10,就打印 `i` 的值并将其加 1。
- 循环将重复 10 次,打印从 0 到 9 的数字。
# 3.1 函数和数组
#### 3.1.1 函数的定义和调用
**函数定义**
函数是代码的模块化单元,它可以接受输入参数,执行特定任务,并返回结果。在单片机C语言中,函数的定义语法如下:
```c
return_type function_name(parameter_list) {
// 函数体
}
```
* **return_type:**指定函数返回的值的类型。如果函数不返回任何值,则使用`void`。
* **function_name:**函数的名称。
* **parameter_list:**函数的参数列表,可以为空。
**函数调用**
要调用函数,只需使用其名称并传递参数即可。函数调用语法如下:
```c
function_name(argument_list);
```
* **argument_list:**函数调用的参数列表,可以为空。
**示例:**
```c
// 定义一个计算两数和的函数
int sum(int a, int b) {
return a + b;
}
// 调用sum函数
int result = sum(5, 10); // result = 15
```
#### 3.1.2 数组的定义和使用
**数组定义**
数组是一种数据结构,它存储同一类型的一组元素。在单片机C语言中,数组的定义语法如下:
```c
data_type array_name[size];
```
* **data_type:**数组元素的类型。
* **array_name:**数组的名称。
* **size:**数组的大小,指定数组中元素的数量。
**数组使用**
数组元素可以通过索引访问,索引从0开始。数组使用语法如下:
```c
array_name[index];
```
* **index:**数组元素的索引。
**示例:**
```c
// 定义一个存储5个整数的数组
int numbers[5];
// 访问数组的第一个元素
int first_number = numbers[0];
```
**数组与函数结合使用**
数组和函数可以结合使用,以实现更复杂的功能。例如,我们可以定义一个函数来查找数组中的最大值:
```c
// 定义一个查找数组中最大值的函数
int find_max(int array[], int size) {
int max = array[0];
for (int i = 1; i < size; i++) {
if (array[i] > max) {
max = array[i];
}
}
return max;
}
// 使用find_max函数
int numbers[] = {1, 2, 3, 4, 5};
int max_value = find_max(numbers, 5); // max_value = 5
```
# 4. 单片机C语言实践应用
### 4.1 I/O端口编程
#### 4.1.1 I/O端口的配置和操作
单片机通过I/O端口与外界进行数据交换,I/O端口可以配置为输入或输出模式。
**配置I/O端口为输入模式**
```c
DDRx &= ~(1 << Px); // Px为端口引脚号,x为端口号
```
**配置I/O端口为输出模式**
```c
DDRx |= (1 << Px); // Px为端口引脚号,x为端口号
```
**读取I/O端口输入数据**
```c
uint8_t data = PINx & (1 << Px); // Px为端口引脚号,x为端口号
```
**向I/O端口输出数据**
```c
PORTx |= (1 << Px); // Px为端口引脚号,x为端口号
```
#### 4.1.2 I/O端口的应用示例
**LED灯控制**
```c
// 定义LED灯连接的端口和引脚
#define LED_PORT PORTB
#define LED_PIN 5
// 初始化LED灯
void led_init() {
// 配置LED灯端口为输出模式
DDRB |= (1 << LED_PIN);
}
// 打开LED灯
void led_on() {
// 将LED灯端口置高
LED_PORT |= (1 << LED_PIN);
}
// 关闭LED灯
void led_off() {
// 将LED灯端口置低
LED_PORT &= ~(1 << LED_PIN);
}
```
### 4.2 定时器编程
#### 4.2.1 定时器的配置和操作
单片机定时器用于产生定时中断或产生脉冲波形。
**配置定时器**
```c
// 定义定时器寄存器
#define TCNT0 TCNT1
#define TCCR0B TCCR1B
#define OCR0A OCR1A
// 配置定时器为CTC模式
TCCR0B |= (1 << WGM02);
// 设置定时器时钟源为内部时钟
TCCR0B |= (1 << CS00);
// 设置定时器比较值
OCR0A = 255;
```
**启动定时器**
```c
// 启动定时器
TCCR0B |= (1 << CS00);
```
**停止定时器**
```c
// 停止定时器
TCCR0B &= ~(1 << CS00);
```
#### 4.2.2 定时器的应用示例
**产生方波**
```c
// 定义定时器寄存器
#define TCNT0 TCNT1
#define TCCR0B TCCR1B
#define OCR0A OCR1A
// 配置定时器为CTC模式
TCCR0B |= (1 << WGM02);
// 设置定时器时钟源为内部时钟
TCCR0B |= (1 << CS00);
// 设置定时器比较值
OCR0A = 255;
// 启用定时器中断
TIMSK0 |= (1 << OCIE0A);
// 定义中断服务函数
ISR(TIMER0_COMPA_vect) {
// 翻转端口输出
PORTB ^= (1 << PB0);
}
```
# 5. 单片机C语言项目实战**
**5.1 LED闪烁程序**
**5.1.1 程序的实现**
```c
#include <reg51.h>
void main() {
while (1) {
P1 = 0x01; // LED1亮
delay(1000); // 延时1s
P1 = 0x00; // LED1灭
delay(1000); // 延时1s
}
}
void delay(unsigned int ms) {
unsigned int i, j;
for (i = 0; i < ms; i++) {
for (j = 0; j < 120; j++);
}
}
```
**5.1.2 程序的分析**
该程序是一个简单的LED闪烁程序。它通过循环地设置P1端口为高电平(LED亮)和低电平(LED灭)来实现LED闪烁的效果。
* `main()`函数是程序的入口点。它包含一个无限循环,该循环不断地执行LED闪烁操作。
* `delay()`函数是一个延时函数,它通过循环执行空操作来实现延时。
* `P1`端口用于控制LED。当`P1`端口为高电平时,LED亮;当`P1`端口为低电平时,LED灭。
**5.2 温度检测程序**
**5.2.1 程序的实现**
```c
#include <reg51.h>
#include <intrins.h>
#define TEMP_SENSOR_PIN P0_0
void main() {
unsigned char temp;
while (1) {
temp = TEMP_SENSOR_PIN; // 读取温度传感器数据
P2 = temp; // 显示温度数据
}
}
```
**5.2.2 程序的分析**
该程序是一个简单的温度检测程序。它通过读取温度传感器的数据并将其显示在P2端口上,来实现温度检测的功能。
* `main()`函数是程序的入口点。它包含一个无限循环,该循环不断地执行温度检测操作。
* `TEMP_SENSOR_PIN`宏定义了温度传感器引脚。
* `P2`端口用于显示温度数据。
0
0
相关推荐




