事件驱动编程中的补偿机制:处理失败事件和回滚操作,保障数据完整性
发布时间: 2024-08-26 13:04:01 阅读量: 43 订阅数: 28 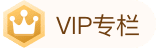
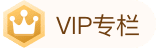
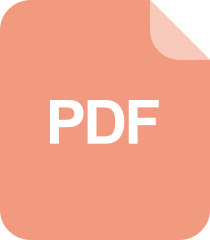
解析php mysql 事务处理回滚操作(附实例)
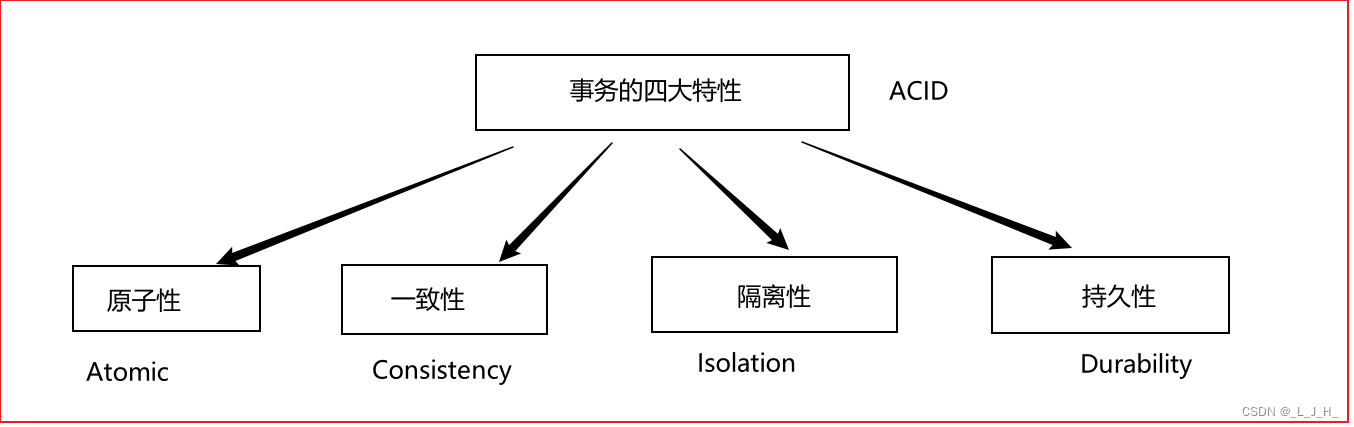
# 1. 事件驱动编程中的补偿机制概述
事件驱动编程(EDP)是一种软件设计模式,它使用事件来触发动作。补偿机制是 EDP 中的关键概念,它允许系统在发生故障时恢复到一致状态。
补偿机制是一种机制,它允许系统在执行操作后撤销或补偿该操作。它通过执行一个相反的操作来实现,该操作将系统恢复到操作前的状态。补偿机制对于确保系统在发生故障时保持数据完整性和业务连续性至关重要。
# 2. 补偿机制的理论基础
### 2.1 补偿机制的定义和分类
**定义:**
补偿机制是一种软件设计模式,用于在发生错误或故障时,将系统恢复到一致状态。它通过执行与原始操作相反的操作来实现,从而抵消原始操作的影响。
**分类:**
补偿机制可以根据其实现方式进行分类:
- **基于消息队列:**使用消息队列来存储和处理补偿操作。
- **基于数据库事务:**使用数据库事务来实现补偿操作。
- **基于业务代码:**直接在业务代码中实现补偿操作。
### 2.2 补偿机制的优点和局限性
**优点:**
- **保证系统一致性:**补偿机制可以确保在发生错误时,系统仍能恢复到一致状态。
- **提高系统可用性:**通过处理错误并恢复系统,补偿机制可以提高系统的可用性。
- **简化错误处理:**补偿机制将错误处理从业务逻辑中分离出来,使其更容易管理和维护。
**局限性:**
- **性能开销:**补偿机制需要执行额外的操作,这可能会增加系统的性能开销。
- **复杂性:**实现和维护补偿机制可能比较复杂,尤其是对于复杂的业务逻辑。
- **潜在的死锁:**如果补偿操作与原始操作涉及相同的资源,可能会导致死锁。
### 2.3 补偿机制的实现原理
补偿机制的实现原理是基于以下步骤:
1. **执行原始操作:**系统执行正常的业务操作。
2. **保存补偿信息:**系统将补偿操作的信息(例如,操作参数、状态等)保存到一个持久化存储中。
3. **发生错误:**如果原始操作发生错误,系统将回滚该操作。
4. **执行补偿操作:**系统从持久化存储中获取补偿信息,并执行与原始操作相反的补偿操作。
5. **恢复系统一致性:**补偿操作完成后,系统将恢复到一致状态。
**代码示例:**
```python
# 基于消息队列的补偿机制
import json
import pika
# 创建消息队列连接
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# 定义队列名称
queue_name = 'compensation_queue'
# 创建队列
channel.queue_declare(queue=queue_name)
# 定义补偿操作
def compensate(data):
# 解析补偿信息
data = json.loads(data)
# 执行补偿操作
# ...
# 监听补偿队列
def callback(ch, method, properties, body):
# 处理补偿信息
data = body.decode('utf-8')
compensate(data)
channel.basic_consume(queue=queue_name, on_message_callback=callback, auto_ack=True)
# 启动消息队列监听
channel.start_consuming()
```
**逻辑分析:**
这段代码实现了基于消息队列的补偿机制。当原始操作发生错误时,补偿信息会被保存到消息队列中。补偿机制会持续监听消息队列,当收到补偿信息时,会执行与原始操作相反的补偿操作,从而恢复系统一致性。
**参数说明:**
- `queue_name`:消息队列名称。
- `callback`:消息队列处理函数。
- `data`:补偿信息。
# 3. 补偿机制的实践应用
### 3.1 基于消息队列的补偿机制
#### 3.1.1 消息队列的原理和应用场景
消息队列是一种异步通信机制,它允许应用程序在不直接通信的情况下交换消息。消息队列充当消息的中间人,将发送方和接收方解耦。
消息队列的典型应用场景包括:
- **异步处理:**将耗时的任务从主流程中分离出来,提高系统响应速度。
- **解耦通信:**允许应用程序在不了解彼此的情况下进行通信,提高系统可扩展性和灵活性。
- **可靠性保证:**通过消息持久化和重试机制,确保消息即使在系统故障的情况下也能被传递。
#### 3.1.2 基于消息队列的补偿机制实现
基于消息队列的补偿机制是一种异步补偿机制,它利用消息队列来传递补偿消息。补偿消息包含了需要执行的补偿操作的信息。
实现基于消息队列的补偿机制的步骤如下:
1. **发送补偿消息:**当发生需要补偿的事件时,发送一个补偿消息到消息队列。
2. **消费补偿消息:**补偿消费者从消息队列中消费补偿消息,并执行相应的补偿操作。
3. **确认补偿:**补偿消费者在执行补偿操作后,向消息队列发送确认消息。
基于消息队列的补偿机制的优点:
- **异步执行:**补偿操作与主流程异步执行,不会影响主流程的性能。
- **高可靠性:**消息队列提供消息持久化和重试机制,确保补偿消息即使在系统故障的情况下也能被传递。
- **可扩展性:**消息队列可以轻松扩展,以满足不断增长的补偿需求。
### 3.2 基于数据库事务的补偿机制
#### 3.2.1 数据库事务的特性和隔离级别
数据库事务是一组原子操作的集合,要么全部成功,要么全部失败。数据库事务具有以下特性:
- **原子性:**事务中的所有操作要么全部成功,要么全部失败,不会出现部分成功的情况。
- **一致性:**事务完成后,数据库必须处于一致的状态,即满足所有业务规则。
- **隔离性:**一个事务对其他事务的影响是隔离的,即一个事务的操作不会被其他事务看到。
- **持久性:**一旦事务提交,其对数据库所做的修改将永久保存。
数据库事务的隔离级别决定了不同事务之间的隔离程度,常见的隔离级别有:
- **读未提交:**一个事务可以读取另一个事务未提交的数据。
- **读已提交:**一个事务只能读取另一个事务已提交的数据。
- **可重复读:**一个事务在执行过程中,不会看到其他事务对同一数据的修改。
- **串行化:**
0
0
相关推荐







