单片机C语言程序设计:深入理解数据结构与算法,提升你的编程思维
发布时间: 2024-07-06 21:54:21 阅读量: 39 订阅数: 46 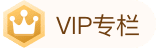
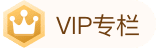
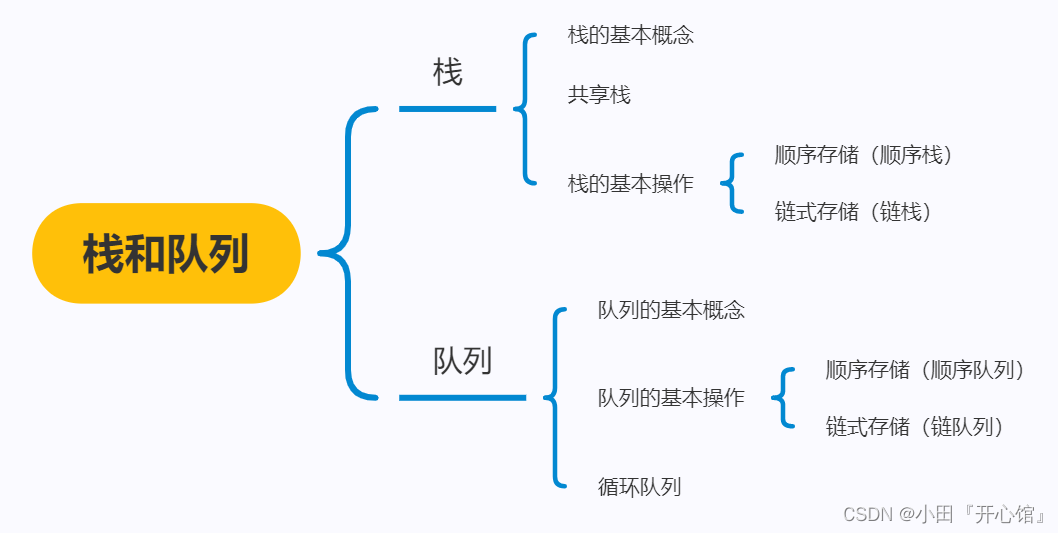
# 1. 单片机C语言程序设计概述**
单片机C语言程序设计是嵌入式系统开发中的核心技术,它是一种基于C语言的、面向单片机的编程语言。单片机C语言具有简洁高效、可移植性强等优点,广泛应用于各种嵌入式系统中。
单片机C语言程序设计的基本流程包括:需求分析、程序设计、代码编写、编译调试、下载烧录。在需求分析阶段,需要明确系统功能、性能要求和硬件资源限制。程序设计阶段,需要设计程序结构、算法和数据结构。代码编写阶段,使用单片机C语言编写程序代码。编译调试阶段,使用编译器将代码编译成可执行文件,并进行调试。下载烧录阶段,将可执行文件下载到单片机中并烧录到芯片上。
单片机C语言程序设计中常用的数据结构包括数组、链表、栈和队列。数组是一种线性数据结构,元素按顺序存储在连续的内存空间中。链表是一种非线性数据结构,元素存储在不连续的内存空间中,通过指针连接。栈和队列是两种特殊的线性数据结构,栈遵循后进先出(LIFO)原则,队列遵循先进先出(FIFO)原则。
# 2. 数据结构与算法基础
### 2.1 数据结构的概念和分类
数据结构是组织和存储数据的抽象方法,它决定了数据的存储方式和访问方式,影响着程序的效率和可维护性。数据结构主要分为以下几类:
#### 2.1.1 数组
数组是一种最基本的线性数据结构,它由一系列相同类型的数据元素组成,每个元素都有一个唯一的索引。数组的优点是访问元素简单高效,但插入和删除元素需要移动大量数据,效率较低。
```c
int arr[10]; // 声明一个包含 10 个整数的数组
```
#### 2.1.2 链表
链表是一种非线性数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。链表的优点是插入和删除元素方便,但查找元素需要遍历整个链表,效率较低。
```c
struct node {
int data;
struct node *next;
};
```
#### 2.1.3 栈和队列
栈和队列都是基于先进先出(FIFO)和后进先出(LIFO)原则的线性数据结构。栈用于存储临时数据,如函数调用时的局部变量,而队列用于处理等待任务,如打印队列。
```c
// 栈的实现
struct stack {
int *data;
int top;
};
// 队列的实现
struct queue {
int *data;
int front, rear;
};
```
### 2.2 算法的复杂度分析
算法的复杂度分析是评估算法效率的一种方法,它描述了算法在不同输入规模下的时间和空间消耗。算法的复杂度通常用大 O 符号表示,表示算法在最坏情况下的复杂度。
#### 2.2.1 时间复杂度
时间复杂度表示算法执行所需的时间,通常用输入规模 n 的函数表示。常见的复杂度等级包括:
- O(1):常数时间复杂度,无论输入规模如何,算法执行时间都为常数。
- O(n):线性时间复杂度,算法执行时间与输入规模 n 成正比。
- O(n^2):平方时间复杂度,算法执行时间与输入规模 n 的平方成正比。
#### 2.2.2 空间复杂度
空间复杂度表示算法执行所需的内存空间,通常用输入规模 n 的函数表示。常见的复杂度等级包括:
- O(1):常数空间复杂度,无论输入规模如何,算法所需的内存空间都为常数。
- O(n):线性空间复杂度,算法所需的内存空间与输入规模 n 成正比。
- O(n^2):平方空间复杂度,算法所需的内存空间与输入规模 n 的平方成正比。
# 3. 单片机C语言中的数据结构应用
### 3.1 数组在单片机中的应用
数组是一种线性数据结构,它由一系列具有相同数据类型且连续存储的元素组成。在单片机C语言中,数组可以用于存储各种类型的数据,如整数、浮点数、字符等。
#### 3.1.1 存储数据
数组最基本的应用之一是存储数据。例如,我们可以使用数组来存储一组传感器采集到的数据,或者存储一个字符串。
```c
int data[10]; // 声明一个存储10个整数的数组
// 将数据存储到数组中
for (int i = 0; i < 10; i++) {
data[i] = i;
}
```
#### 3.1.2
0
0
相关推荐
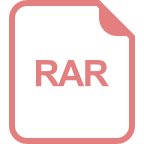
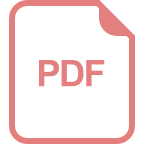






