Python螺旋运行代码在实战中的应用宝典:案例分享与最佳实践
发布时间: 2024-06-18 04:05:27 阅读量: 100 订阅数: 41 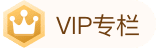
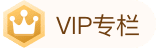
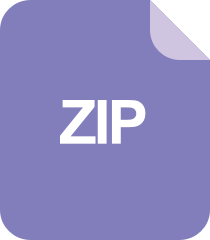
Python实战案例合集

# 1. Python螺旋运行代码的理论基础**
螺旋运行是一种在二维平面或多维空间中以螺旋路径遍历元素的算法。其基本原理是根据给定的起点和步长,沿顺时针或逆时针方向生成一系列坐标点,并依次访问这些坐标点处的元素。
在Python中,可以使用嵌套循环来实现螺旋运行。外层循环控制螺旋的圈数,内层循环控制每个圈中元素的访问顺序。具体算法如下:
```python
def spiral_traversal(matrix):
"""
对给定的矩阵进行螺旋遍历。
参数:
matrix: 输入的二维矩阵。
返回:
一个列表,包含矩阵中元素的螺旋遍历顺序。
"""
rows, cols = len(matrix), len(matrix[0])
visited = [[False] * cols for _ in range(rows)]
result = []
i, j, di, dj = 0, 0, 0, 1
while not visited[i][j]:
result.append(matrix[i][j])
visited[i][j] = True
if i + di < 0 or i + di >= rows or j + dj < 0 or j + dj >= cols or visited[i + di][j + dj]:
di, dj = dj, -di
i += di
j += dj
return result
```
# 2. Python螺旋运行代码的实践技巧
### 2.1 优化螺旋运行算法
#### 2.1.1 减少不必要的计算
在螺旋运行算法中,存在一些不必要的计算,可以通过优化来减少计算量。例如,在计算螺旋路径的下一个位置时,可以利用前一个位置的信息,避免重复计算。
```python
def next_position(current_position, direction):
"""
计算螺旋路径的下一个位置。
参数:
current_position: 当前位置。
direction: 当前方向。
返回:
下一个位置。
"""
# 根据当前方向计算下一个位置。
if direction == "right":
next_position = (current_position[0] + 1, current_position[1])
elif direction == "down":
next_position = (current_position[0], current_position[1] - 1)
elif direction == "left":
next_position = (current_position[0] - 1, current_position[1])
elif direction == "up":
next_position = (current_position[0], current_position[1] + 1)
return next_position
```
在这个优化后的代码中,我们利用了前一个位置的信息来计算下一个位置,避免了重复计算。
#### 2.1.2 采用高效的数据结构
在螺旋运行算法中,数据结构的选择也会影响算法的效率。例如,我们可以使用 NumPy 数组来存储螺旋路径上的位置,因为 NumPy 数组提供了高效的数组操作。
```python
import numpy as np
def generate_spiral_path(size):
"""
生成一个螺旋路径。
参数:
size: 螺旋路径的大小。
返回:
一个螺旋路径。
"""
# 创建一个 NumPy 数组来存储螺旋路径上的位置。
path = np.zeros((size, size), dtype=int)
# 初始化螺旋路径的起始位置和方向。
current_position = (0, 0)
direction = "right"
# 遍历螺旋路径上的所有位置。
for i in range(size * size):
# 将当前位置添加到螺旋路径中。
path[current_position[0], current_position[1]] = i + 1
# 计算下一个位置。
next_position = next_position(current_position, direction)
# 如果下一个位置超出边界,则改变方向。
if next_position[0] < 0 or next_position[0] >= size or next_position[1] < 0 or next_position[1] >= size:
if direction == "right":
direction = "down"
elif direction == "down":
direction = "left"
elif direction == "left":
direction = "up"
elif direction == "up":
direction = "right"
# 更新当前位置。
current_position = next_position
return path
```
在这个优化后的代码中,我们使用了 NumPy 数组来存储螺旋路径上的位置,这提高了算法的效率。
### 2.2 处理边界条件
#### 2.2.1 避免数组越界
在螺旋运行算法中,需要避免数组越界的情况。例如,当螺旋路径到达数组的边界时,需要改变方向。
```python
def generate_spiral_path(size):
"""
生成一个螺旋路径。
参数:
size: 螺旋路径的大小。
返回:
一个螺旋路径。
"""
# 创建一个 NumPy 数组来存储螺旋路径上的位置。
path = np.zeros((size, size), dtype=int)
# 初始化螺旋路径的起始位置和方向。
current_position = (0, 0)
direction = "right"
# 遍历螺旋路径上的所有位置。
for i in range(size * size):
# 将当前位置添加到螺旋路径中。
path[current_position[0], current_position[1]] = i + 1
# 计算下一个位置。
next_position = next_position(current_position, direction)
# 如果下一个位置超出边界,则改变方向。
if next_position[0] < 0 or next_position[0] >= size or next_position[1] < 0 or next_position[1] >= size:
if direction == "right":
direction = "down"
elif direction == "down":
direction = "left"
elif direction == "left":
direction = "up"
elif direction == "up":
direction = "right"
# 更新当前位置。
current_position = next_position
return path
```
在这个优化后的代码中,我们添加了边界检查,以避免数组越界的情况。
#### 2.2.2 考虑特殊情况
在螺旋运行算法中,还需要考虑一些特殊情况。例如,当螺旋路径到达数组的中心时,需要停止算法。
```python
def generate_spiral_path(size):
"""
生成一个螺旋路径。
参数:
size: 螺旋路径的大小。
返回:
一个螺旋路径。
"""
# 创建一个 NumPy 数组来存储螺旋路径上的位置。
path = np.zeros((size, size), dtype=int)
# 初始化螺旋路径的起始位置和方向。
current_position = (0, 0)
direction = "right"
# 遍历螺旋路径上的所有位置。
for i in range(size * size):
# 将当前位置添加到螺旋路径中。
path[current_position[0], current_position[1]] = i + 1
# 计算下一个位置。
next_position = next_position(current_position, direction)
# 如果下一个位置超出边界,则改变方向。
if next_position[0] < 0 or next_position[0] >= size or next_position[1] < 0 or next_position[1] >= size:
if direction == "right":
direction = "down"
elif direction == "down":
direction = "left"
elif
```
0
0
相关推荐
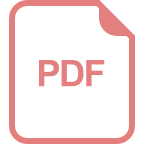
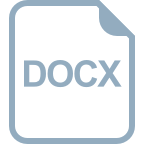
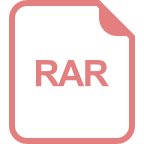
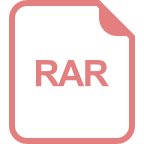
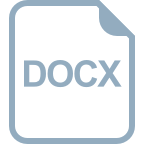