集成Spring Security:保护Spring MVC应用的安全性
发布时间: 2024-03-25 18:22:52 阅读量: 37 订阅数: 44 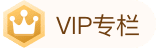
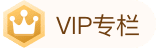
# 1. Spring Security简介
1.1 什么是Spring Security?
1.2 Spring Security的特性与优势
1.3 Spring Security在Web应用中的作用
# 2. 集成Spring Security到Spring MVC应用
在这一章中,我们将学习如何将Spring Security集成到Spring MVC应用中。首先,我们需要配置Spring Security的Maven依赖,然后设置基本的配置,最后可以根据需要进行自定义配置。
### 2.1 配置Spring Security依赖
首先,在项目的`pom.xml`文件中添加以下Spring Security的Maven依赖:
```xml
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-web</artifactId>
<version>5.4.2</version>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-config</artifactId>
<version>5.4.2</version>
</dependency>
```
### 2.2 配置Spring Security的基本设置
接着,在Spring配置文件(如`applicationContext.xml`)中配置Spring Security的基本设置,例如:
```xml
<beans:beans xmlns="http://www.springframework.org/schema/security"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/security
http://www.springframework.org/schema/security/spring-security.xsd">
<http auto-config="true">
<intercept-url pattern="/admin/**" access="hasRole('ROLE_ADMIN')" />
<form-login />
</http>
<authentication-manager>
<authentication-provider>
<user-service>
<user name="admin" password="admin" authorities="ROLE_ADMIN" />
</user-service>
</authentication-provider>
</authentication-manager>
</beans:beans>
```
### 2.3 自定义Spring Security配置
如果需要自定义Spring Security的配置,可以创建一个类继承`WebSecurityConfigurerAdapter`,并覆盖`configure()`方法,如下:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password("password").roles("USER")
.and()
.withUser("admin").password("admin").roles("USER", "ADMIN");
}
}
```
通过以上配置,您可以将Spring Security成功集成到Spring MVC应用中,并且可以根据需要进行个性化的定制和配置。
# 3. 认证与授权
在本章中,我们将深入探讨Spring Security中的认证与授权机制,这是保护Spring MVC应用安全性的核心部分。
#### 3.1 认证流程介绍
在一个Web应用中,用户需要通过认证来验证其身份。Spring Security使用AuthenticationManager来管理认证,并通过UserDetailsService来获取用户的认证信息。
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
}
```
#### 3.2 使用内存认证
对于简单的应用场景,可以使用内存认证来验证用户。
```java
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
```
0
0
相关推荐
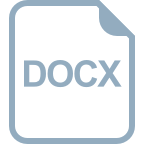
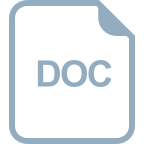
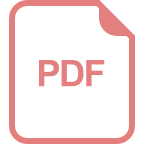
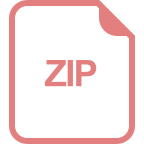
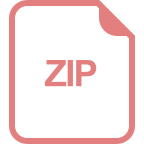
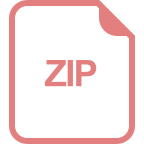
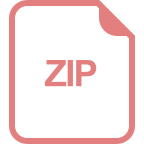
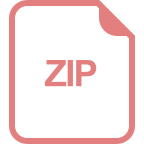
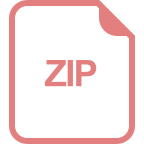