MySQL连接池与微服务架构:微服务场景下的连接管理
发布时间: 2024-08-05 06:18:31 阅读量: 11 订阅数: 16 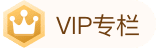
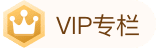
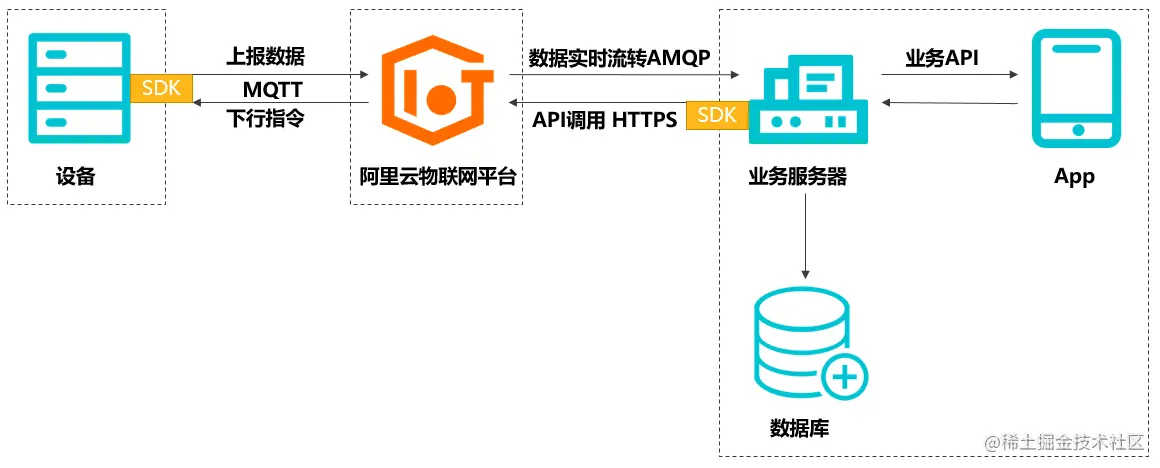
# 1. MySQL连接池概述**
MySQL连接池是一种管理和复用MySQL数据库连接的机制。它通过预先建立和维护一定数量的空闲连接,从而避免了频繁创建和销毁连接的开销,提高了数据库访问的性能。
连接池提供了许多优点,包括:
- 减少连接创建和销毁的开销,提高性能。
- 限制同时打开的连接数,防止数据库资源耗尽。
- 提高应用程序的可用性,通过自动重连和故障恢复机制。
# 2.1 连接池的概念和原理
### 2.1.1 连接池的优点和缺点
**优点:**
- **减少开销:**创建和销毁数据库连接是昂贵的操作。连接池通过重用现有的连接,减少了这些开销。
- **提高性能:**由于连接池避免了频繁创建和销毁连接,它可以显著提高数据库操作的性能。
- **可伸缩性:**连接池允许应用程序根据需要动态调整连接数,从而提高可伸缩性。
- **故障隔离:**连接池中的连接是独立的,因此一个连接的故障不会影响其他连接。
**缺点:**
- **资源消耗:**连接池需要维护一定数量的空闲连接,这会消耗系统资源。
- **连接泄漏:**如果应用程序不正确释放连接,可能会导致连接泄漏,从而耗尽系统资源。
- **安全性:**如果连接池配置不当,可能会导致安全漏洞,例如 SQL 注入攻击。
### 2.1.2 连接池的实现方式
连接池可以采用以下两种实现方式:
- **基于队列的连接池:**应用程序将连接请求放入队列中,连接池从队列中获取请求并分配连接。这种方式简单易用,但性能可能较低。
- **基于对象池的连接池:**连接池维护一个连接对象池,应用程序直接从对象池中获取连接。这种方式性能更高,但实现更复杂。
**代码块:**
```python
# 基于队列的连接池
from queue import Queue
from threading import Thread
class QueueBasedConnectionPool:
def __init__(self, max_connections):
self.max_connections = max_connections
self.queue = Queue()
self.connections = []
# 创建连接线程
for i in range(max_connections):
thread = Thread(target=self.create_connection)
thread.start()
def create_connection(self):
# 创建数据库连接
connection = create_connection()
self.connections.append(connection)
self.queue.put(connection)
def get_connection(self):
# 从队列中获取连接
connection = self.queue.get()
return connection
def release_connection(self, connection):
# 将连接放回队列
self.queue.put(connection)
# 基于对象池的连接池
from collections import deque
class ObjectBasedConnectionPool:
def __init__(self, max_connections):
self.max_connections = max_connections
self.connections = deque()
def get_connection(self):
# 从对象池中获取连接
if len(self.connections) == 0:
# 创建新连接
connection = create_connection()
self.connections.append(connection)
else:
# 重
```
0
0
相关推荐
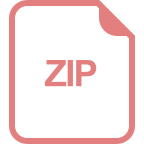
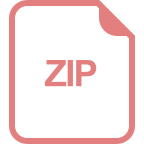
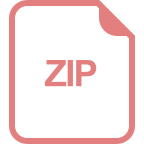





