TensorFlow基础概念解析与实践
发布时间: 2024-03-22 14:49:24 阅读量: 37 订阅数: 48 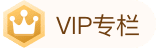
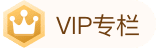
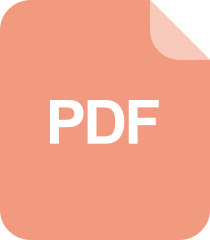
TensorFlow基础
# 1. TensorFlow简介
TensorFlow是一个由Google开发的开源机器学习框架,广泛应用于深度学习和人工智能领域。本章将介绍TensorFlow的基本概念、历史沿革以及应用领域。TensorFlow提供了一种灵活的架构,让用户可以方便地构建和训练各种机器学习模型,并且在各种硬件设备上运行,包括CPU、GPU甚至TPU(Tensor Processing Unit)等。TensorFlow的出现极大地推动了机器学习和深度学习技术的发展,成为当前人工智能领域的热门工具之一。
# 2. TensorFlow基本概念
TensorFlow作为一个强大的机器学习框架,其基本概念对于理解和应用 TensorFlow 是非常重要的。在本章中,我们将深入探讨 TensorFlow 的基本概念,包括张量、计算图、数据流图和会话的使用方法。
### 2.1 张量(Tensor)是什么
在 TensorFlow 中,张量(Tensor)是数据的基本单位。它可以看作是一个多维数组,可以是标量(0 维张量)、向量(1 维张量)、矩阵(2 维张量)或更高维的数据结构。在张量中,我们可以存储和处理各种数据类型,如整数、浮点数等。
```python
import tensorflow as tf
# 创建一个常量张量
tensor = tf.constant([[1, 2], [3, 4]])
print(tensor)
```
**代码总结:** 在 TensorFlow 中,张量是多维数组,是数据的基本单位。
**结果说明:** 打印张量 `[[1, 2], [3, 4]]`。
### 2.2 计算图(Computational Graph)的概念和作用
TensorFlow 使用计算图来描述计算任务,计算图是由节点(Nodes)和边(Edges)构成的有向图,节点表示操作(Operations),边表示数据流(Tensors)。通过在计算图中定义节点和边,我们可以实现各种复杂的计算任务。
```python
import tensorflow as tf
# 创建计算图
a = tf.constant(5)
b = tf.constant(3)
c = tf.add(a, b)
print(c)
```
**代码总结:** 在 TensorFlow 中,通过计算图定义节点和边来实现计算任务。以上代码实现了两个常量相加的操作。
**结果说明:** 打印节点 `c`,结果为 8。
### 2.3 TensorFlow中的数据流图(Data Flow Graph)
数据流图是一种特殊的计算图,描述了数据在各个操作之间的流动过程。TensorFlow 使用数据流图来表示整个计算过程,通过节点之间的边传递张量,实现数据的流动和计算。
```python
import tensorflow as tf
# 创建数据流图
a = tf.constant(2)
b = tf.constant(3)
c = tf.multiply(a, b)
with tf.Session() as sess:
result = sess.run(c)
print(result)
```
**代码总结:** 在 TensorFlow 中,数据流图描述了数据在操作之间的流动过程。以上代码实现了两个常量相乘的操作,并通过会话执行得到结果。
**结果说明:** 打印结果 6。
### 2.4 会话(Session)的使用方法
在 TensorFlow 中,会话(Session)负责执行操作和计算图中的节点。通过创建会话对象,并调用 `run()` 方法来启动计算图中的节点操作,实现计算任务的执行。
```python
import tensorflow as tf
# 创建会话
a = tf.constant(2)
b = tf.constant(3)
c = tf.add(a, b)
with tf.Session() as sess:
result = sess.run(c)
print(result)
```
**代码总结:** 在 TensorFlow 中,会话负责执行计算图中的节点操作。以上代码创建了一个会话对象,执行了两个常量相加的操作,并打印结果。
**结果说明:** 打印结果 5。
通过本章的学习,读者应该对 TensorFlow 的基本概念有了更深入的理解,包括张量、计算图、数据流图和会话的使用方法。在接下来的章节中,我们将继续深入探讨 TensorFlow 的核心组件和实践技巧。
# 3. TensorFlow核心组件深度解析
在这一章节中,我们将深入探讨TensorFlow的核心组件,包括变量(Variables)、占位符(Placeholders)和张量操作(Tensor Operations)。
#### 3.1 TensorFlow的变量(Variables)
TensorFlow中的变量是一种特殊的张量,用于存储持久化的状态,通常用于表示神经网络中的权重和偏置项。在使用变量之前,需要先对变量进行初始化操作。下面是一个使用变量的示例代码:
```python
import tensorflow as tf
# 定义一个变量
weight = tf.Variable(initial_value=tf.random_normal(shape=(5, 5), stddev=0.1), name='weight')
# 初始化变量
init = tf.global_variables_initializer()
# 创建会话并运行初始化操作
with tf.Session() as sess:
sess.run(init)
print(sess.run(weight))
```
#### 3.2 TensorFlow的占位符(Placeholders)
占位符用于在构建计算图时暂时存储数据,等到真正执行计算图时再传入具体数值。占位符在训练神经网络时非常有用。以下是一个简单的占位符示例:
```python
import tensorflow as tf
# 创建占位符
input_data = tf.placeholder(tf.float32, shape=(None, 784), name='input_data')
# 使用占位符进行计算
output = tf.reduce_sum(input_data, axis=1)
with tf.Session() as sess:
data = [[1, 2, 3, ...]] # 输入数据
result = sess.run(output, feed_dict={input_data: data})
print(result)
```
#### 3.3 TensorFlow的张量操作(Tensor Operations)
TensorFlow提供了丰富的张量操作函数,用于构建计算图并进行数值计算。常用的张量操作包括加法、乘法、矩阵乘法等。以下是一个简单的张量操作示例:
```python
import tensorflow as tf
# 定义两个常量张量
a = tf.constant([1, 2, 3])
b = tf.constant([4, 5, 6])
# 加法操作
c = tf.add(a, b)
with tf.Session() as sess:
result = sess.run(c)
print(result)
```
通过深入理解和使用TensorFlow的核心组件,可以更好地构建和优化神经网络模型。在下一章节中,我们将探讨如何使用TensorFlow构建模型并进行训练。
# 4. TensorFlow模型构建
在TensorFlow中构建模型是机器学习和深度学习应用的关键步骤。本章将介绍如何使用TensorFlow构建模型,包括简单的神经网络模型、层和模块的使用方法,以及训练模型的基本流程。
#### 4.1 如何构建简单的神经网络模型
在TensorFlow中构建神经网络模型的核心是定义网络结构、选择合适的激活函数和损失函数。以下是一个简单的全连接神经网络模型的构建示例:
```python
import tensorflow as tf
# 定义输入数据和标签
X = tf.placeholder(tf.float32, shape=(None, 784))
y_true = tf.placeholder(tf.float32, shape=(None, 10))
# 定义神经网络参数
W = tf.Variable(tf.random_normal([784, 10]))
b = tf.Variable(tf.zeros([10]))
# 构建模型
y_pred = tf.matmul(X, W) + b
# 定义损失函数
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=y_pred, labels=y_true))
# 定义优化器
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01)
train_step = optimizer.minimize(cross_entropy)
# 初始化变量
init = tf.global_variables_initializer()
# 训练模型
with tf.Session() as sess:
sess.run(init)
for i in range(1000):
batch_X, batch_y = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={X: batch_X, y_true: batch_y})
```
#### 4.2 TensorFlow中的层(Layers)和模块(Modules)使用方法
TensorFlow提供了丰富的层和模块,方便快速构建复杂的神经网络模型。以下是一个使用TensorFlow Layers构建卷积神经网络(CNN)的示例:
```python
import tensorflow as tf
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = tf.keras.Sequential([
Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=5)
```
#### 4.3 TensorFlow训练模型的基本流程
训练模型的基本流程包括定义模型、选择损失函数和优化器、设置训练参数、载入数据并进行训练。通过迭代多个epoch,不断调整模型参数来提高模型性能。
以上是TensorFlow模型构建的基本内容,通过学习和实践这些知识,可以帮助您更好地构建和优化深度学习模型。
# 5. TensorFlow模型优化与调参
在构建和训练TensorFlow模型后,优化和调参是提高模型性能和泛化能力的关键步骤。本章将深入探讨如何使用TensorFlow中的损失函数、优化器、正则化方法和学习率调整策略来优化模型。
#### 5.1 TensorFlow的损失函数(Loss Functions)及优化器(Optimizers)
在TensorFlow中,损失函数用于衡量模型预测输出与真实标签之间的差异,是模型优化的关键指标。常见的损失函数包括均方差误差(Mean Squared Error)、交叉熵误差(Cross Entropy Error)等。通过选择合适的损失函数可以更好地指导模型参数的更新方向。
优化器则用于根据损失函数计算的梯度来更新模型参数,常用的优化器有随机梯度下降(Stochastic Gradient Descent)、Adam、RMSprop等。不同的优化器对模型训练的速度和效果有着不同的影响,需要根据具体问题进行选择。
```python
# 示例:使用Adam优化器和交叉熵损失函数进行模型优化
import tensorflow as tf
# 定义模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(units=10, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(units=10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=5)
```
#### 5.2 TensorFlow中的正则化方法和学习率调整策略
为了缓解模型过拟合的问题,可以通过正则化方法来约束模型的复杂度,常见的正则化方法包括L1正则化、L2正则化和Dropout。这些方法可以有效提高模型的泛化能力,避免在训练集上表现良好但在测试集上表现较差的情况。
另外,在训练过程中动态调整学习率也是优化模型的重要手段。学习率过大会导致震荡或发散,学习率过小会导致训练过程缓慢。常见的学习率调整策略有指数衰减、学习率衰减和自适应学习率等。
```python
# 示例:使用L2正则化和指数衰减学习率优化模型
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', kernel_regularizer=tf.keras.regularizers.l2(0.01)),
tf.keras.layers.Dense(10, activation='softmax')
])
initial_learning_rate = 0.1
lr_schedule = tf.keras.optimizers.schedules.ExponentialDecay(
initial_learning_rate,
decay_steps=1000,
decay_rate=0.96,
staircase=True)
optimizer = tf.keras.optimizers.SGD(learning_rate=lr_schedule)
model.compile(optimizer=optimizer,
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
```
#### 5.3 使用TensorBoard进行模型性能可视化
TensorBoard是TensorFlow提供的强大可视化工具,可以帮助我们可视化模型训练过程中的指标、损失函数、权重分布等信息,帮助分析模型性能和调参效果。
```python
# 示例:使用TensorBoard可视化模型训练过程
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir=log_dir, histogram_freq=1)
model.fit(train_images, train_labels, epochs=5, callbacks=[tensorboard_callback])
```
通过以上优化和调参的方法,我们可以更好地提升模型的性能,逐步完善和优化我们的TensorFlow模型。
# 6. TensorFlow实践项目
在本章中,我们将探讨如何在TensorFlow中进行实际项目开发,包括构建图像分类模型、自然语言处理应用以及其他领域的实践案例分析。
#### 6.1 使用TensorFlow构建图像分类模型
首先,我们将介绍如何在TensorFlow中构建一个图像分类模型。以下是一个简单的示例代码,用于训练一个基本的卷积神经网络(CNN)进行图像分类。
```python
import tensorflow as tf
from tensorflow.keras import layers, models
# 构建CNN模型
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(10, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(train_images, train_labels, epochs=5)
# 评估模型
test_loss, test_acc = model.evaluate(test_images, test_labels)
print("Test accuracy:", test_acc)
```
通过上面的代码,我们可以看到如何使用TensorFlow构建一个简单的图像分类模型,并进行训练和评估。
#### 6.2 TensorFlow在自然语言处理中的应用
除了图像分类外,TensorFlow也广泛应用于自然语言处理(NLP)领域。以下是一个简单的情感分析模型示例代码,用于对文本进行情感分类。
```python
import tensorflow as tf
from tensorflow.keras import layers, models
# 构建情感分析模型
model = models.Sequential([
layers.Embedding(input_dim=1000, output_dim=64),
layers.LSTM(128),
layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.compile(optimizer='adam',
loss='binary_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(train_texts, train_labels, epochs=5)
# 评估模型
test_loss, test_acc = model.evaluate(test_texts, test_labels)
print("Test accuracy:", test_acc)
```
通过以上代码,我们展示了如何在TensorFlow中构建一个简单的情感分析模型,并进行训练和评估。
#### 6.3 TensorFlow在其他领域的实践案例分析
除了图像分类和自然语言处理,TensorFlow在许多其他领域也有着广泛的应用。例如,音频处理、推荐系统、强化学习等领域都可以看到TensorFlow的身影。在实际项目中,可以根据具体需求结合TensorFlow的强大功能进行开发,实现各种应用场景的解决方案。
0
0
相关推荐
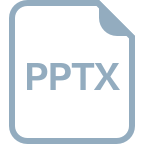
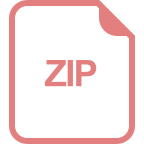
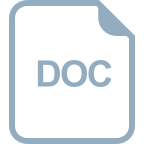
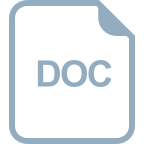
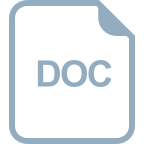
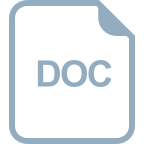
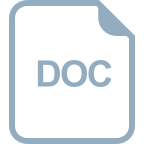