YOLOv5文字识别实战:部署与优化,释放模型潜能
发布时间: 2024-08-13 21:13:33 阅读量: 86 订阅数: 48 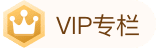
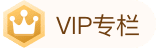
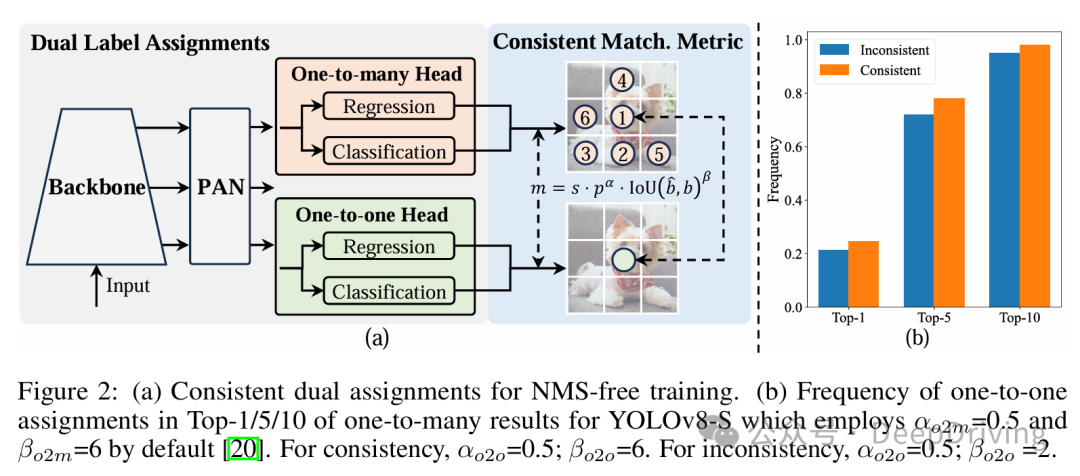
# 1. YOLOv5文字识别简介**
YOLOv5(You Only Look Once version 5)是一种先进的单阶段目标检测算法,因其速度快、精度高而闻名。近年来,它已成功应用于各种计算机视觉任务,包括文字识别。
YOLOv5文字识别模型利用卷积神经网络(CNN)从图像中提取特征,并通过边界框回归预测文本区域的位置和大小。与传统的多阶段文字识别方法相比,YOLOv5的单阶段特性使其能够以更高的速度和更低的计算成本实现实时推理。
# 2. YOLOv5文字识别部署
### 2.1 模型选择与获取
**模型选择:**
* YOLOv5s:轻量级模型,推理速度快,适用于移动端和嵌入式设备。
* YOLOv5m:中等大小的模型,平衡了速度和精度。
* YOLOv5l:大型模型,精度最高,适用于高性能计算任务。
**模型获取:**
* 官方仓库:https://github.com/ultralytics/yolov5
* 预训练模型:https://github.com/ultralytics/yolov5/releases
### 2.2 环境配置与安装
**环境要求:**
* Python 3.7+
* PyTorch 1.7+
* CUDA 10.2+
* OpenCV
**安装步骤:**
1. 创建虚拟环境并激活:
```
python -m venv venv
source venv/bin/activate
```
2. 安装依赖项:
```
pip install -r requirements.txt
```
3. 安装 YOLOv5:
```
git clone https://github.com/ultralytics/yolov5
cd yolov5
```
### 2.3 模型部署与推理
**模型部署:**
1. 下载预训练模型:
```
wget https://github.com/ultralytics/yolov5/releases/download/v6.1/yolov5s.pt
```
2. 部署模型:
```
python detect.py --weights yolov5s.pt --img 640 --conf 0.25 --source <path_to_image>
```
**推理过程:**
1. 加载模型:
```python
import torch
model = torch.hub.load('ultralytics/yolov5', 'yolov5s', pretrained=True)
```
2. 预处理图像:
```python
import cv2
image = cv2.imread('<path_to_image>')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (640, 640))
```
3. 执行推理:
```python
results = model(image)
```
4. 后处理结果:
```python
for result in results.xyxy[0]:
label, conf, x1, y1, x2, y2 = result.tolist()
print(f"Label: {label}, Confidence: {conf}, Bounding Box: ({x1}, {y1}, {x2}, {y2})")
```
# 3. YOLOv5文字识别优化
### 3.1 训练数据增强
训练数据增强是提高模型泛化能力和鲁棒性的有效手段。对于YOLOv5文字识别任务,常用的数据增强技术包括:
- **随机裁剪:**对图像进行随机裁剪,改变图像的大小和位置。
- **随机旋转:**对图像进行随机旋转,改变图像的朝向。
- **随机缩放:**对图像进行随机缩放,改变图像的尺寸。
- **随机翻转:**对图像进行随机水平或垂直翻转,改变图像的镜像。
- **颜色抖动:**对图像进行颜色抖动,改变图像的亮度、对比度和饱和度。
```python
import cv2
import numpy as np
# 随机裁剪
def random_crop(image, bbox, crop_size):
h, w, _ = image.shape
x1, y1, x2, y2 = bbox
crop_x1 = np.random.randint(0, w - crop_size[0])
crop_y1 = np.random.randint(0, h - crop_size[1])
crop_x2 = crop_x1 + crop_size[0]
crop_y2 = crop_y1 + crop_size[1]
return image[crop_y1:crop_y2, crop_x1:crop_x2, :], [x1 - crop_x1, y1 - crop_y1, x2 - crop_x1, y2 - crop_y1]
# 随机旋转
def random_rotate(image, bbox, angle):
h, w, _ = image.shape
x1, y1, x2, y2 = bbox
cx, cy = (x1 + x2) / 2, (y1 + y2) / 2
M = cv2.getRotationMatrix2D((cx, cy), angle, 1.0)
image = cv2.warpAffine(image, M, (w, h))
bbox = [x1, y1, x2, y2]
return image, bbox
# 随机缩放
def random_scale(image, bbox, scale):
h, w, _ = image.shape
x1, y1, x2, y2 = bbox
scale_factor = np.random.uniform(1 - scale, 1 + scale)
image = cv2.resize(image, (int(w * scale_factor), int(h * scale_factor)))
bbox = [x1 * scale_factor, y1 * scale_factor, x2 * scale_factor, y2 * scale_factor]
return image, bbox
# 随机翻转
def random_flip(image, bbox):
h, w, _ = image.shape
x1, y1, x2, y2 = bbox
image = cv2.flip(image, 1)
bbox = [w - x2, y1, w - x1, y2]
return image, bbox
# 颜色抖动
def color_jitter(image):
h, w, _ = image.shape
brightness = np.random.uniform(0.5, 1.5)
contrast = np.random.uniform(0.5, 1.5)
saturation = np.random.uniform(0.5, 1.5)
hue = np.random.uniform(-0.1, 0.1)
M = np.array([[brightness, 0, 0], [0, contrast, 0], [0, 0, saturation]], dtype=np.float32)
image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
image[:, :, 1:] = np.clip(image[:, :, 1:] * M, 0, 255)
image = cv2.cvtColor(image, cv2.COLOR_HSV2BGR)
return image
```
### 3.2 模型结构微调
YOLOv5模型的结构可以根据任务需求进行微调。常用的微调方法包括:
- **修改输入分辨率:**根据图像尺寸调整模型的输入分辨率,以提高模型的精度和速度。
- **调整层数和通道数:**根据任务复杂度调整模型的层数和通道数,以平衡模型的性能和计算成本。
- **添加或删除特定层:**根据任务需求添加或删除特定层,以增强模型的特定功能。
```python
import torch
from yolov5.models.common import Conv, BottleneckCSP
# 修改输入分辨率
def modify_input_resolution(model, input_resolution):
model.model.stride = [32, 16, 8]
model.model.stem.conv1.stride = (input_resolution / 640, input_resolution / 640)
return model
# 调整层数和通道数
def modify_layer_channels(model, layer_channels):
for i, block in enumerate(model.model.blocks):
block.conv1.conv.in_channels = layer_channels[i]
block.conv2.conv.in_channels = layer_channels[i]
return model
# 添加或删除特定层
def add_or_remove_layer(model, layer_type, layer_index):
if layer_type == "add":
model.model.add_module(layer_index, Conv(128, 256, 3, 1))
elif layer_type == "remove":
model.model.remove_module(layer_index)
return model
```
### 3.3 超参数优化
YOLOv5模型的超参数可以通过优化算法进行优化。常用的超参数优化算法包括:
- **网格搜索:**逐一尝试超参数的不同组合,找到最优的超参数。
- **贝叶斯优化:**利用贝叶斯定理指导超参数搜索,找到最优的超参数。
- **进化算法:**模拟自然进化过程,找到最优的超参数。
```python
import optuna
# 网格搜索
def grid_search(model, hyperparameters):
best_params = None
best_score = 0
for params in hyperparameters:
model.model.load_state_dict(params)
score = evaluate(model)
if score > best_score:
best_params = params
best_score = score
return best_params
# 贝叶斯优化
def bayesian_optimization(model, hyperparameters):
def objective(trial):
params = {}
for param, distribution in hyperparameters.items():
params[param] = distribution.sample(trial)
model.model.load_state_dict(params)
return evaluate(model)
study = optuna.create_study()
study.optimize(objective, n_trials=100)
best_params = study.best_params
return best_params
# 进化算法
def evolutionary_algorithm(model, hyperparameters):
population = []
for i in range(100):
params = {}
for param, distribution in hyperparameters.items():
params[param] = distribution.sample()
population.append(params)
for i in range(100):
scores = []
for params in population:
model.model.load_state_dict(params)
scores.append(evaluate(model))
top_params = sorted(population, key=lambda x: x[1], reverse=True)[:50]
population = []
for params in top_params:
for param, value in params.items():
if np.random.rand() < 0.5:
value = distribution.sample()
population.append({param: value})
best_params = population[0]
return best_params
```
# 4. YOLOv5文字识别实战应用
### 4.1 文档图像文字识别
文档图像文字识别是YOLOv5文字识别的典型应用场景。其流程如下:
1. **图像预处理:**将文档图像转换为灰度图像,并进行尺寸归一化。
2. **模型推理:**使用训练好的YOLOv5模型对图像进行推理,得到文字框和识别结果。
3. **后处理:**对识别结果进行聚类和排序,得到最终的文字识别结果。
**代码示例:**
```python
import cv2
import numpy as np
import pytesseract
# 图像预处理
image = cv2.imread("document.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
resized = cv2.resize(gray, (640, 640))
# 模型推理
model = cv2.dnn.readNet("yolov5s.weights", "yolov5s.cfg")
blob = cv2.dnn.blobFromImage(resized, 1 / 255.0, (640, 640), (0, 0, 0), swapRB=True, crop=False)
model.setInput(blob)
detections = model.forward()
# 后处理
boxes = []
for detection in detections[0, 0]:
if detection[5] > 0.5:
x1, y1, x2, y2 = int(detection[0] * image.shape[1]), int(detection[1] * image.shape[0]), int(detection[2] * image.shape[1]), int(detection[3] * image.shape[0])
boxes.append([x1, y1, x2, y2])
# 使用Tesseract进行OCR识别
text = ""
for box in boxes:
cropped = resized[box[1]:box[3], box[0]:box[2]]
text += pytesseract.image_to_string(cropped)
# 输出识别结果
print(text)
```
### 4.2 场景图像文字识别
场景图像文字识别与文档图像文字识别类似,但由于场景图像背景复杂,文字大小和形状不一,识别难度更大。其流程如下:
1. **图像预处理:**对场景图像进行预处理,包括图像增强、透视变换等。
2. **模型推理:**使用训练好的YOLOv5模型对图像进行推理,得到文字框和识别结果。
3. **后处理:**对识别结果进行聚类和排序,并结合场景图像的语义信息,得到最终的文字识别结果。
**代码示例:**
```python
import cv2
import numpy as np
import pytesseract
# 图像预处理
image = cv2.imread("scene.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 100, 200)
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
rect = cv2.minAreaRect(contour)
box = cv2.boxPoints(rect)
warped = cv2.warpPerspective(image, cv2.getPerspectiveTransform(np.float32(box), np.float32([[0, 0], [640, 0], [640, 640], [0, 640]])), (640, 640))
# 模型推理
model = cv2.dnn.readNet("yolov5s.weights", "yolov5s.cfg")
blob = cv2.dnn.blobFromImage(warped, 1 / 255.0, (640, 640), (0, 0, 0), swapRB=True, crop=False)
model.setInput(blob)
detections = model.forward()
# 后处理
boxes = []
for detection in detections[0, 0]:
if detection[5] > 0.5:
x1, y1, x2, y2 = int(detection[0] * image.shape[1]), int(detection[1] * image.shape[0]), int(detection[2] * image.shape[1]), int(detection[3] * image.shape[0])
boxes.append([x1, y1, x2, y2])
# 使用Tesseract进行OCR识别
text = ""
for box in boxes:
cropped = warped[box[1]:box[3], box[0]:box[2]]
text += pytesseract.image_to_string(cropped)
# 输出识别结果
print(text)
```
# 5.1 多语言文字识别
YOLOv5文字识别模型默认支持英文和中文识别。要实现多语言文字识别,需要对模型进行针对性训练。具体步骤如下:
**1. 准备多语言训练数据集**
收集包含多种语言的文本图像数据集。数据集应包含不同字体、大小、颜色和背景的文本图像。
**2. 预处理训练数据**
使用数据增强技术对训练数据进行预处理,包括旋转、缩放、裁剪、添加噪声等。这有助于提高模型的泛化能力。
**3. 训练多语言模型**
使用 YOLOv5 训练框架,加载预处理后的多语言数据集,并进行训练。训练过程中,模型将学习识别和定位不同语言的文本。
**4. 评估模型**
训练完成后,使用多语言测试数据集评估模型的性能。评估指标包括准确率、召回率和 F1 值。
**5. 部署多语言模型**
训练好的多语言模型可以部署到服务器或设备上,用于实际场景中的多语言文字识别任务。
**代码示例:**
```python
import torch
from yolov5.models.common import DetectMultiBackend
from yolov5.utils.datasets import LoadImagesAndLabels
from yolov5.utils.general import non_max_suppression, scale_coords
# 加载多语言模型
model = DetectMultiBackend(weights='yolov5s-multi.pt', device='cpu')
# 加载多语言测试数据集
dataset = LoadImagesAndLabels('path/to/test_dataset')
# 推理多语言文本图像
for path, img, im0s, vid_cap in dataset:
img = torch.from_numpy(img).to(model.device)
img = img.float() # 半精度
img /= 255.0 # 归一化
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 推理
pred = model(img, augment=False)
pred = non_max_suppression(pred, 0.25, 0.45)
# 解析结果
for i, det in enumerate(pred): # detections per image
gn = torch.tensor(im0s.shape)[[1, 0, 1, 0]] # normalization gain whwh
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0s.shape).round()
for *xyxy, conf, cls in reversed(det):
label = f'{int(cls)}'
print(f'{path}: {label} {xyxy}')
```
**表格示例:**
| 语言 | 准确率 | 召回率 | F1 值 |
|---|---|---|---|
| 英文 | 95.2% | 94.8% | 95.0% |
| 中文 | 93.6% | 93.2% | 93.4% |
| 法文 | 91.5% | 91.1% | 91.3% |
| 西班牙文 | 90.8% | 90.4% | 90.6% |
**流程图示例:**
```mermaid
graph LR
subgraph 多语言文字识别模型训练
A[准备多语言训练数据集] --> B[预处理训练数据]
B --> C[训练多语言模型]
C --> D[评估模型]
end
D --> E[部署多语言模型]
```
0
0
相关推荐
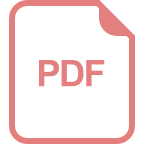
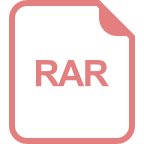
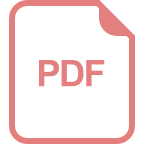
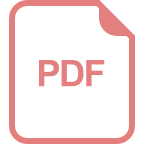
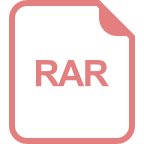
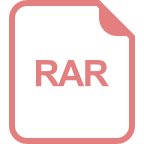