Python执行Linux命令的10大最佳实践:提升效率和可靠性
发布时间: 2024-06-22 15:40:17 阅读量: 113 订阅数: 32 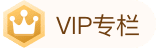
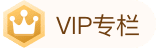
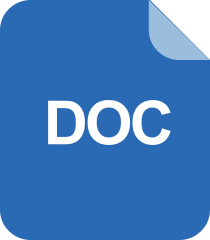
提高linux命令行下工作效率.

# 1. Python执行Linux命令的基础知识
Python提供了一个强大的生态系统,用于与Linux操作系统交互,其中包括执行Linux命令。本章将介绍Python执行Linux命令的基础知识,包括:
- 使用`subprocess`模块执行Linux命令
- 捕获命令输出并处理错误
- 优化命令执行以提高效率
# 2. Python执行Linux命令的最佳实践
在掌握了Python执行Linux命令的基础知识后,让我们深入探讨一些最佳实践,以提高代码的健壮性、效率和可维护性。
### 2.1 确保命令可用性
#### 2.1.1 验证命令路径
在执行命令之前,验证命令路径至关重要,以避免"命令未找到"错误。可以使用`which`命令来检查命令是否存在于系统路径中:
```python
import subprocess
def check_command_path(command):
"""验证命令是否存在于系统路径中。
Args:
command (str): 要检查的命令。
Returns:
bool: 如果命令存在,返回True;否则返回False。
"""
try:
subprocess.run(['which', command], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
return True
except subprocess.CalledProcessError:
return False
```
#### 2.1.2 处理命令不存在的情况
如果命令不存在,可以使用`raise`语句引发异常,并提供有意义的错误消息:
```python
def execute_command(command):
"""执行命令并处理命令不存在的情况。
Args:
command (str): 要执行的命令。
Raises:
subprocess.CalledProcessError: 如果命令不存在。
"""
if not check_command_path(command):
raise subprocess.CalledProcessError(1, command, output="Command not found")
else:
subprocess.run(command, shell=True)
```
### 2.2 捕获命令输出
#### 2.2.1 使用subprocess.Popen()
`subprocess.Popen()`允许我们捕获命令的输出,以便进一步处理或分析:
```python
import subprocess
def capture_output(command):
"""捕获命令的输出。
Args:
command (str): 要执行的命令。
Returns:
tuple: 命令的输出和错误信息。
"""
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
output, error = process.communicate()
return output, error
```
#### 2.2.2 处理输出缓冲区
默认情况下,`subprocess.Popen()`会将输出缓冲到内存中。如果输出很大,这可能会导致内存不足。我们可以使用`stdout=subprocess.PIPE`和`stderr=subprocess.PIPE`参数将输出重定向到管道,以逐行处理输出:
```python
def process_output(command):
"""逐行处理命令的输出。
Args:
command (str): 要执行的命令。
"""
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
for line in process.stdout:
# 处理每行输出
pass
for line in process.stderr:
# 处理每行错误信息
pass
```
### 2.3 处理命令错误
#### 2.3.1 检查返回码
每个命令都会返回一个返回码,指示命令的执行状态。我们可以使用`returncode`属性来检查返回码:
```python
def check_return_code(command):
"""检查命令的返回码。
Args:
command (str): 要执行的命令。
Returns:
int: 命令的返回码。
"""
process = subprocess.run(command)
return process.returncode
```
#### 2.3.2 捕获错误信息
如果命令执行失败,我们可以使用`stderr`属性来捕获错误信息:
```python
def capture_error(command):
"""捕获命令的错误信息。
Args:
command (str): 要执行的命令。
Returns:
str: 命令的错误信息。
"""
process = subprocess.run(command, stderr=subprocess.PIPE)
return process.stderr.decode('utf-8')
```
### 2.4 优化命令执行
#### 2.4.1 使用Shell脚本
对于复杂或多命令的场景,使用Shell脚本可以提高效率。Python可以使用`subprocess.call()`或`subprocess.check_call()`来执行Shell脚本:
```python
import subprocess
def execute_shell_script(script_path):
"""执行Shell脚本。
Args:
script_path (str): Shell脚本的路径。
"""
subprocess.call(script_path)
```
#### 2.4.2 并行执行命令
对于需要并行执行多个命令的情况,我们可以使用`concurrent.futures`模块:
```python
import concurrent.futures
def parallel_execute(commands):
"""并行执行多个命令。
Args:
commands (list): 要执行的命令列表。
"""
with concurrent.futures.ThreadPoolExecutor() as executor:
executor.map(subprocess.run, commands)
```
# 3.1 使用交互式Shell
#### 3.1.1 使用subprocess.run()
`subprocess.run()` 函数提供了一种更高级别的接口来执行命令,它可以处理交互式命令和捕获输出。
```python
import subprocess
# 执行交互式命令
result = subprocess.run("echo Hello World!", shell=True, capture_output=True)
# 捕获输出
output = result.stdout.decode("utf-8")
print(output) # 输出:Hello World!
```
**参数说明:**
- `shell=True`: 指定使用系统shell执行命令。
- `capture_output=True`: 捕获命令输出并返回在 `stdout` 和 `stderr` 属性中。
#### 3.1.2 处理交互式命令
交互式命令需要用户输入才能执行。`subprocess.run()` 函数可以通过 `input` 参数提供输入。
```python
import subprocess
# 执行交互式命令
result = subprocess.run("read -p 'Enter your name: ' name; echo Hello $name!", shell=True, capture_output=True, input="John")
# 捕获输出
output = result.stdout.decode("utf-8")
print(output) # 输出:Hello John!
```
**参数说明:**
- `input="John"`: 提供给交互式命令的输入。
# 4. Python执行Linux命令的特殊场景
### 4.1 处理特殊字符
在执行Linux命令时,某些特殊字符具有特殊含义,可能会导致命令执行出现问题。常见的特殊字符包括:
- 空格:空格用于分隔命令和参数,但如果参数中包含空格,则需要使用引号或转义字符来将其括起来。
- 引号:引号用于将参数括起来,防止其被解释为多个参数。
- 转义字符:转义字符用于转义特殊字符,使其失去特殊含义。常见的转义字符包括反斜杠(\)和单引号(')。
#### 4.1.1 转义特殊字符
为了避免特殊字符导致命令执行问题,可以通过转义字符将其转义。例如:
```python
import subprocess
# 转义空格
command = "echo hello world"
subprocess.run(command, shell=True)
# 转义引号
command = 'echo "hello world"'
subprocess.run(command, shell=True)
# 转义转义字符
command = r"echo \"hello world\""
subprocess.run(command, shell=True)
```
#### 4.1.2 使用引号和转义序列
除了转义字符,还可以使用引号将参数括起来,防止其被解释为多个参数。常见的引号类型包括单引号(')和双引号(")。
- 单引号:单引号内的所有字符都被视为普通字符,不会被解释为特殊字符。
- 双引号:双引号内的字符会被解释为特殊字符,但可以转义特殊字符使其失去特殊含义。
例如:
```python
# 使用单引号
command = 'echo hello "world"'
subprocess.run(command, shell=True)
# 使用双引号
command = "echo hello \"world\""
subprocess.run(command, shell=True)
```
### 4.2 处理环境变量
环境变量是存储在操作系统中的键值对,用于配置系统和应用程序。在执行Linux命令时,可以获取和设置环境变量,以影响命令的执行。
#### 4.2.1 获取环境变量
可以通过`os.environ`字典获取当前环境变量。例如:
```python
import os
# 获取环境变量
env_var = os.environ.get("PATH")
print(env_var)
```
#### 4.2.2 设置环境变量
可以通过`os.environ`字典设置环境变量。例如:
```python
# 设置环境变量
os.environ["MY_VAR"] = "my_value"
# 执行命令
command = "echo $MY_VAR"
subprocess.run(command, shell=True)
```
### 4.3 处理文件路径
在执行Linux命令时,经常需要处理文件路径。文件路径可以是绝对路径或相对路径。
#### 4.3.1 规范化文件路径
规范化文件路径是指将文件路径转换为绝对路径并简化其结构。例如:
```python
import os
# 规范化文件路径
path = os.path.normpath("/home/user/Documents/file.txt")
print(path)
```
#### 4.3.2 处理相对路径和绝对路径
相对路径相对于当前工作目录,而绝对路径从根目录开始。在执行Linux命令时,需要根据命令的语义选择合适的路径类型。例如:
```python
# 相对路径
command = "ls Documents"
subprocess.run(command, shell=True)
# 绝对路径
command = "ls /home/user/Documents"
subprocess.run(command, shell=True)
```
# 5. Python执行Linux命令的扩展应用
### 5.1 构建命令行界面
#### 5.1.1 使用argparse模块
argparse模块提供了构建命令行界面的工具,允许用户指定命令行参数及其类型、帮助文本和默认值。
```python
import argparse
parser = argparse.ArgumentParser(description="Example command line interface")
parser.add_argument("-f", "--file", type=str, help="Input file path")
parser.add_argument("-o", "--output", type=str, help="Output file path", default="output.txt")
args = parser.parse_args()
print(args.file)
print(args.output)
```
### 5.1.2 处理命令行参数
解析命令行参数后,可以通过`args`对象访问参数值。
```python
# 获取输入文件路径
input_file = args.file
# 获取输出文件路径,如果未指定则使用默认值
output_file = args.output if args.output else "output.txt"
```
### 5.2 编写系统脚本
#### 5.2.1 创建系统服务
Python脚本可以通过创建systemd服务单元来作为系统服务运行。
```python
# 创建systemd服务单元文件
with open("/etc/systemd/system/my_service.service", "w") as f:
f.write("[Unit]\nDescription=My Service\nAfter=network.target\n\n[Service]\nType=simple\nExecStart=/usr/bin/python /path/to/my_script.py\n\n[Install]\nWantedBy=multi-user.target")
# 启用并启动服务
os.system("systemctl daemon-reload")
os.system("systemctl enable my_service")
os.system("systemctl start my_service")
```
#### 5.2.2 编写启动脚本
Python脚本可以通过编写init脚本在系统启动时运行。
```python
# 创建init脚本
with open("/etc/init.d/my_script", "w") as f:
f.write("#!/bin/bash\n\n# Start script\nstart() {\n /usr/bin/python /path/to/my_script.py\n}\n\n# Stop script\nstop() {\n killall my_script.py\n}\n\n# Main\ncase "$1" in\n start)\n start\n ;;\n stop)\n stop\n ;;\n *)\n echo "Usage: /etc/init.d/my_script {start|stop}" >&2\n exit 1\n ;;\nesac\nexit 0")
# 使脚本可执行
os.chmod("/etc/init.d/my_script", 0o755)
# 添加脚本到启动序列
os.system("update-rc.d my_script defaults")
```
0
0
相关推荐
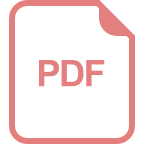
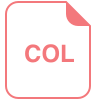
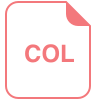
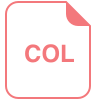
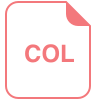
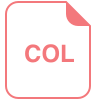
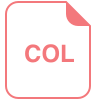